Ruby on Rails Developer Interview Questions & Answers Download PDF
Elevate your Ruby on Rails Developer interview readiness with our detailed compilation of 30 questions. These questions will test your expertise and readiness for any Ruby on Rails Developer interview scenario. Ideal for candidates of all levels, this collection is a must-have for your study plan. Get the free PDF download to access all 30 questions and excel in your Ruby on Rails Developer interview. This comprehensive guide is essential for effective study and confidence building.
30 Ruby on Rails Developer Questions and Answers:
Ruby on Rails Developer Job Interview Questions Table of Contents:
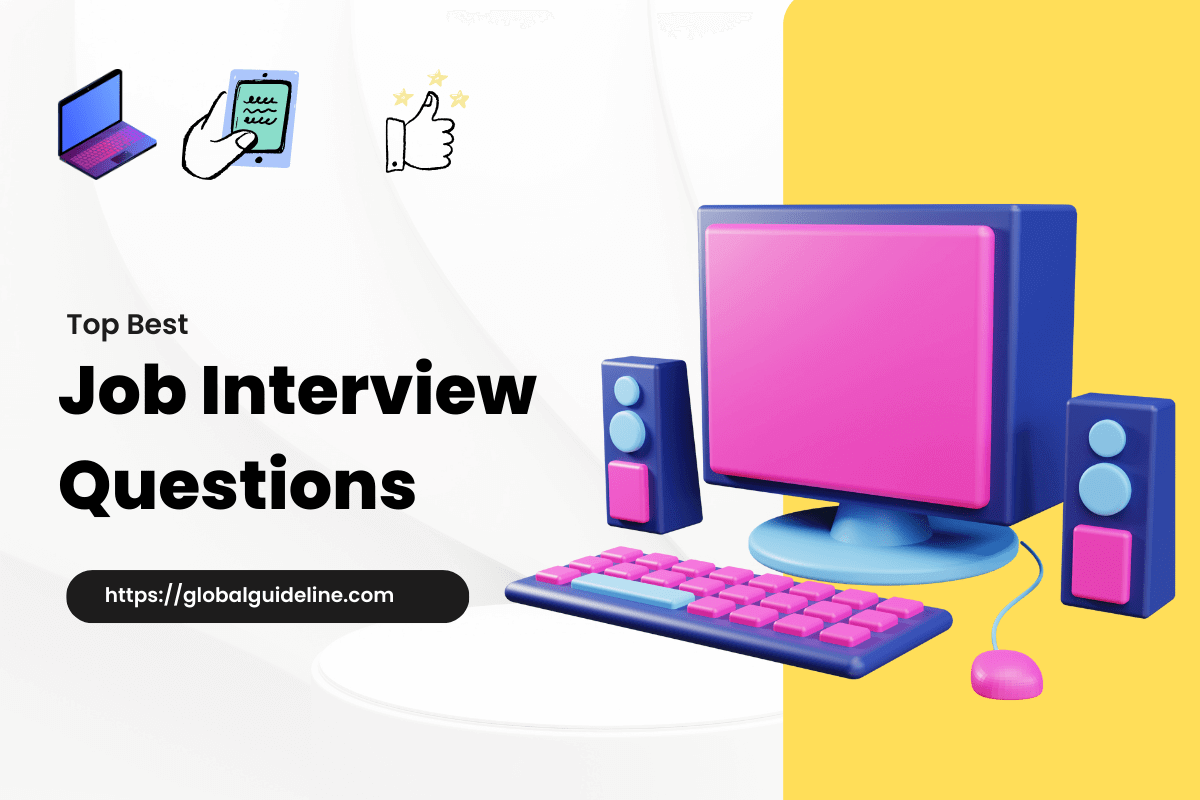
1 :: What is Rails Router?
The Rails router recognizes URLs and dispatches them to a controller’s action. It also generate paths and URLs. Rails router deals URLs in a different way from other language routers. It determines controller, parameters and action for the request.
2 :: Tell us what is the use of super function in Ruby on Rails?
The super function in Ruby is used to invoke the original method. It calls the super class implementation of the current method.
3 :: Tell us what’s the difference between new, save and create?
new – makes a new object but doesn’t save it to the database
save – saves the model. If the model is new a record gets created in the database, otherwise the existing record gets updated.
create – makes a new object and saves it to the database in one go
save – saves the model. If the model is new a record gets created in the database, otherwise the existing record gets updated.
create – makes a new object and saves it to the database in one go
4 :: Tell us what are benefits of using Active Record as opposed to raw SQL queries?
Here are the benefits:
☛ it’s really easy to go wrong during writing SQL queries
☛ each different type of database usually has a slightly different flavour of SQL, so it could be really painful if, during work on a big app, you decide to switch to a different database
☛ using Active Record reduces code significantly and makes it easier to read
☛ using Active Record makes common operations simpler and easier than writing your own SQL statements
☛ it’s really easy to go wrong during writing SQL queries
☛ each different type of database usually has a slightly different flavour of SQL, so it could be really painful if, during work on a big app, you decide to switch to a different database
☛ using Active Record reduces code significantly and makes it easier to read
☛ using Active Record makes common operations simpler and easier than writing your own SQL statements
5 :: Explain me what is Testing in Rails?
Rails test is very simple to write and run for your application. As Rails script generates models and controllers, in the same way test files are also generated. Rails also uses a separate database for testing. Test database in an application is rebuilt each time the application’s test run, and hence you always have a consistent database when your tests are run.
6 :: Do you know the role of the subdirectories app/controllers and app/helpers in Rails?
The app/controllers subdirectory holds all the controller classes for the app. Controllers handle web requests from the user. The app/helpers subdirectory holds helper classes, which are used to assist the model, view, and controller classes. By keeping helper classes in a separate subdirectory, the model, view, and controller classes can remain lean and uncluttered.
7 :: Do you know what is Rails Caching?
Caching is used in any web technologies. It speeds up the performance by storing previous results for subsequent requests. By default, cahcing is disabled in Rails.
Rails caching is available at three levels of granularity:
☛ Page
☛ Action
☛ Fragment
Rails caching is available at three levels of granularity:
☛ Page
☛ Action
☛ Fragment
8 :: Please explain what is the purpose of the rakefile available in the demo directory in Ruby?
The purpose of this simple question is to make sure a developer is familiar with a test-driven development. A beginner may not have dealt with this file yet. The rakefile is similar to the makefile in Unix, and assists with packaging and testing Rails code. It’s used by the rake utility, which ships natively with the Ruby installation.
9 :: Explain me what Are The Servers Supported By Ruby On Rails?
RoR was generally preferred over WEBrick server at the time of writing, but it can also be run by:
Lighttpd (pronounced ‘lighty’) is an open-source web server more optimized for speed-critical environments.
Abyss Web Server- is a compact web server available for windows, Mac osX and Linux operating system. Apache and nginx
Lighttpd (pronounced ‘lighty’) is an open-source web server more optimized for speed-critical environments.
Abyss Web Server- is a compact web server available for windows, Mac osX and Linux operating system. Apache and nginx
10 :: Do you know what are rails filters?
Rails filters are methods that run before or after a controller’s action method is executed. They are helpful when you want to ensure that a given block of code runs with whatever action method is called.
Rails support three types of filter methods:
☛ Before filters
☛ After filters
☛ Around filters
Rails support three types of filter methods:
☛ Before filters
☛ After filters
☛ Around filters
11 :: Tell us how Many Types Of Callbacks Available In Ror?
☛ before_validation
☛ before_validation_on_create
☛ validate_on_create
☛ after_validation
☛ after_validation_on_create
☛ before_save
☛ before_create
☛ after_create
☛ after_save
☛ before_validation_on_create
☛ validate_on_create
☛ after_validation
☛ after_validation_on_create
☛ before_save
☛ before_create
☛ after_create
☛ after_save
12 :: Tell us what are some advantages of using Ruby on Rails?
Famed coder Yukihiro “Matz” Matsumoto designed Ruby to make programmers “happy”—Rails affords you all the advantages of Ruby, including simple syntax, an extensive library, and a quickly growing community. Here are some advantages to look for in the developer’s answer.
☛ Programmer Productivity: The Ruby framework isn’t called “Rails” for no reason—testimonies abound around the web on how Rails can quickly carry an app from conception, through development, and into production in record speed.
☛ Built-In Testing: Rails enables developers to use supporting code called harnesses and fixtures to quickly draft simple extendable automated tests.
☛ Open-Source: Rails is open-source and 100% free, and its compatibility with Linux means there are many open-source options available when constructing your solution stack.
☛ Metaprogramming: The ability to write code that acts on code rather than data can be a huge advantage, and Rails makes it easy.
☛ Programmer Productivity: The Ruby framework isn’t called “Rails” for no reason—testimonies abound around the web on how Rails can quickly carry an app from conception, through development, and into production in record speed.
☛ Built-In Testing: Rails enables developers to use supporting code called harnesses and fixtures to quickly draft simple extendable automated tests.
☛ Open-Source: Rails is open-source and 100% free, and its compatibility with Linux means there are many open-source options available when constructing your solution stack.
☛ Metaprogramming: The ability to write code that acts on code rather than data can be a huge advantage, and Rails makes it easy.
13 :: Tell us what is the purpose of the resources method in the code snippet below?
resources :posts do
member do
get ‘messages’
end
collection do
post ‘bulk_upload'
end
end
Defining routes with the “resources” method automatically generates routes for the seven standard RESTful actions:
☛ GET /messages
☛ POST /messages
☛ GET /messages/new
☛ GET /messages/:id/edit
☛ GET /messages/:id
☛ PATCH/PUT /messages/:id
☛ DELETE /messages/:id
member do
get ‘messages’
end
collection do
post ‘bulk_upload'
end
end
Defining routes with the “resources” method automatically generates routes for the seven standard RESTful actions:
☛ GET /messages
☛ POST /messages
☛ GET /messages/new
☛ GET /messages/:id/edit
☛ GET /messages/:id
☛ PATCH/PUT /messages/:id
☛ DELETE /messages/:id
14 :: Tell me what’s the difference between destroy and delete?
Both of these methods delete the record in the database. The different between them is that:
☛ destroy – checks and deletes connected records if necessary and calls callbacks i.e. before_destroy, after_destroy etc.
☛ delete – doesn’t call callbacks and removes an object directly from the database.
☛ destroy – checks and deletes connected records if necessary and calls callbacks i.e. before_destroy, after_destroy etc.
☛ delete – doesn’t call callbacks and removes an object directly from the database.
15 :: Do you know who developed Rails?
Ruby on Rails was created by David Heinemeier Hansson (DHH).
16 :: Tell us ruby Supports Single Inheritance/multiple Inheritance Or Both?
Ruby Supports only Single Inheritance
17 :: Please explain what is a Rails Migration? Write up a short example of a simple Rails Migration with a table called customers, a string column called name, and a text column called description.?
Initiating the command c:rubyapplication>ruby script/generate migration table_name will create a Rails Migration. A Rails Migration can be used to create, drop, or remove tables and columns. A potential solution is provided below.
class CreateCustomers < ActiveRecord::Migration
def up
create_table :customers do |t|
t.string :name
t.text :description
t.timestamps
end
end
def down
drop_table :customers
end
end
class CreateCustomers < ActiveRecord::Migration
def up
create_table :customers do |t|
t.string :name
t.text :description
t.timestamps
end
end
def down
drop_table :customers
end
end
18 :: Tell me what is CoC in Rails?
DRY stands for Convention over Configuration. It provides different opinions for the best way to do many things in a web application.
19 :: Tell me the difference between ActiveSupport’s “HashWithIndifferentAccess” and Ruby’s “Hash”?
The “HashWithIndifferentAccess” class will treat symbol keys and string keys as equivalent while the “Hash” class in Ruby will use the stricter = = comparison on keys—an equivalent string key will not retrieve the value for a given symbol key.
20 :: Tell me how does Ruby on Rails use the Model View Controller (MVC) framework?
Web development can often be divided into three separate but closely integrated subsystems:
☛ Model (Active Record): The model handles all the data logic of the application. In Rails, this is handled by the Active Record library, which forms the bridge between the Ruby program code and the relational database.
☛ View (Action View): The view is the part of the application that the end user sees. In Rails, this is implemented by the Action View library, which is based on Embedded Ruby (ERB) and determines how data will be presented.
☛ Controller (Action Controller): The controller is like the data broker of an application, handling the logic that allows the model and view to communicate with one another. This is called the Action Controller in Rails.
☛ Model (Active Record): The model handles all the data logic of the application. In Rails, this is handled by the Active Record library, which forms the bridge between the Ruby program code and the relational database.
☛ View (Action View): The view is the part of the application that the end user sees. In Rails, this is implemented by the Action View library, which is based on Embedded Ruby (ERB) and determines how data will be presented.
☛ Controller (Action Controller): The controller is like the data broker of an application, handling the logic that allows the model and view to communicate with one another. This is called the Action Controller in Rails.
21 :: Tell us what Is The Log That Has To Seen To Check For An Error In Ruby Rails?
Rails will report errors from Apache in log/apache.log and errors from the ruby code in log/development.log. If you having a problem, do have a look at what these log are saying.
22 :: Tell us what Is Active Record?
Active Record are like Object Relational Mapping(ORM), where classes are mapped to table and objects are mapped to columns in the table.
23 :: What is Rails Migrations?
Migrations are a way to alter database schema over time in a consistent and organized manner. They use a Ruby DSL through which there is no need to write SQL by hand.
24 :: Do you know the role of garbage collection in Ruby on Rails?
Garbage collection frees up memory for other processes by removing pointer programs and inaccessible objects left behind after a program has executed. This frees the programmer from having to track objects created dynamically during runtime.
25 :: Tell me how does Rails implement AJAX?
Asynchronous JavaScript and XML (AJAX) is a suite of technologies used to retrieve data for a webpage without having to refresh the page itself. This is how modern websites are able to cultivate a “desktop-like” user experience. The Rails method of implementing AJAX operations is short and simple.
☛ First, a trigger is fired. The trigger can be something as simple as a user clicking on a call to action.
☛ Next, the web client uses JavaScript to send data via an XMLHttpRequest from the trigger to an action handler on the server.
☛ On the server-side, a Rails controller action receives the data and returns the corresponding HTML fragment to the client.
☛ The client receives the fragment and updates the view accordingly.
☛ First, a trigger is fired. The trigger can be something as simple as a user clicking on a call to action.
☛ Next, the web client uses JavaScript to send data via an XMLHttpRequest from the trigger to an action handler on the server.
☛ On the server-side, a Rails controller action receives the data and returns the corresponding HTML fragment to the client.
☛ The client receives the fragment and updates the view accordingly.
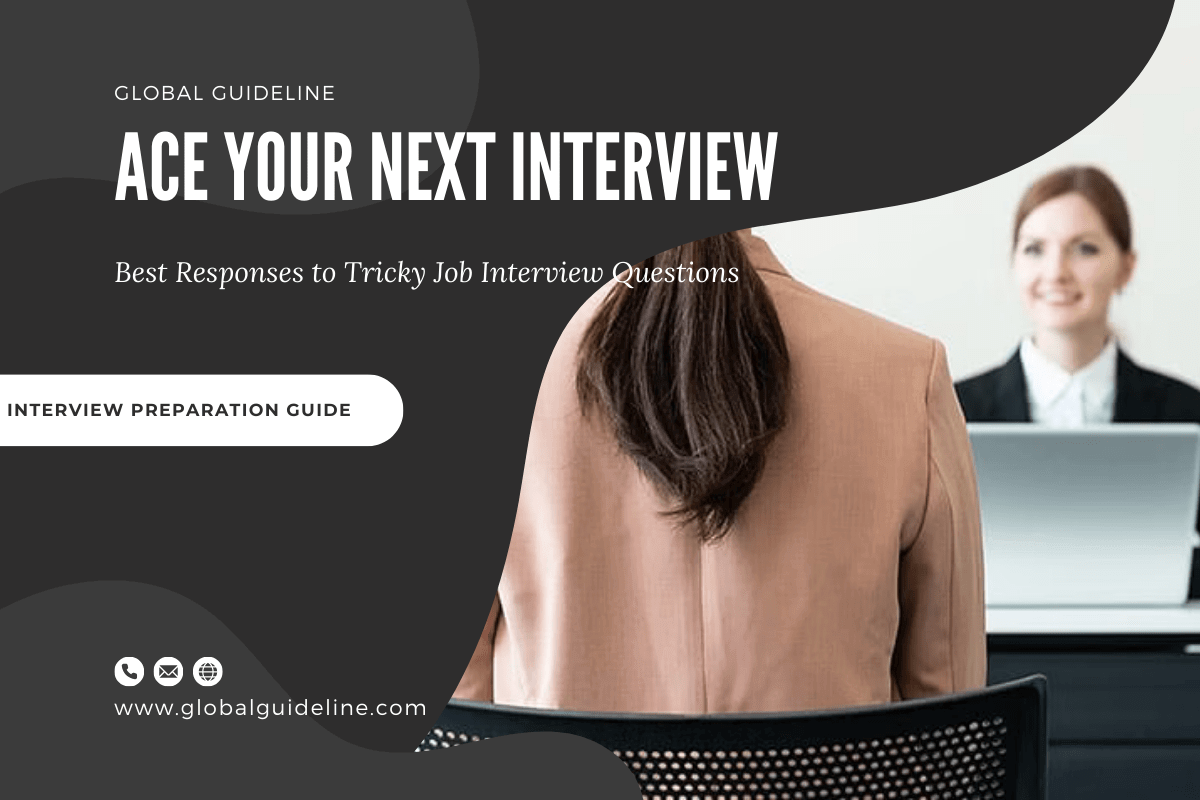