CGI Perl Interview Questions And Answers
Optimize your CGI Perl interview preparation with our curated set of 18 questions. Each question is designed to test and expand your CGI Perl expertise. Suitable for all experience levels, these questions will help you prepare thoroughly. Download the free PDF now to get all 18 questions and ensure you're well-prepared for your CGI Perl interview. This resource is perfect for in-depth preparation and boosting your confidence.
18 CGI Perl Questions and Answers:
CGI Perl Job Interview Questions Table of Contents:
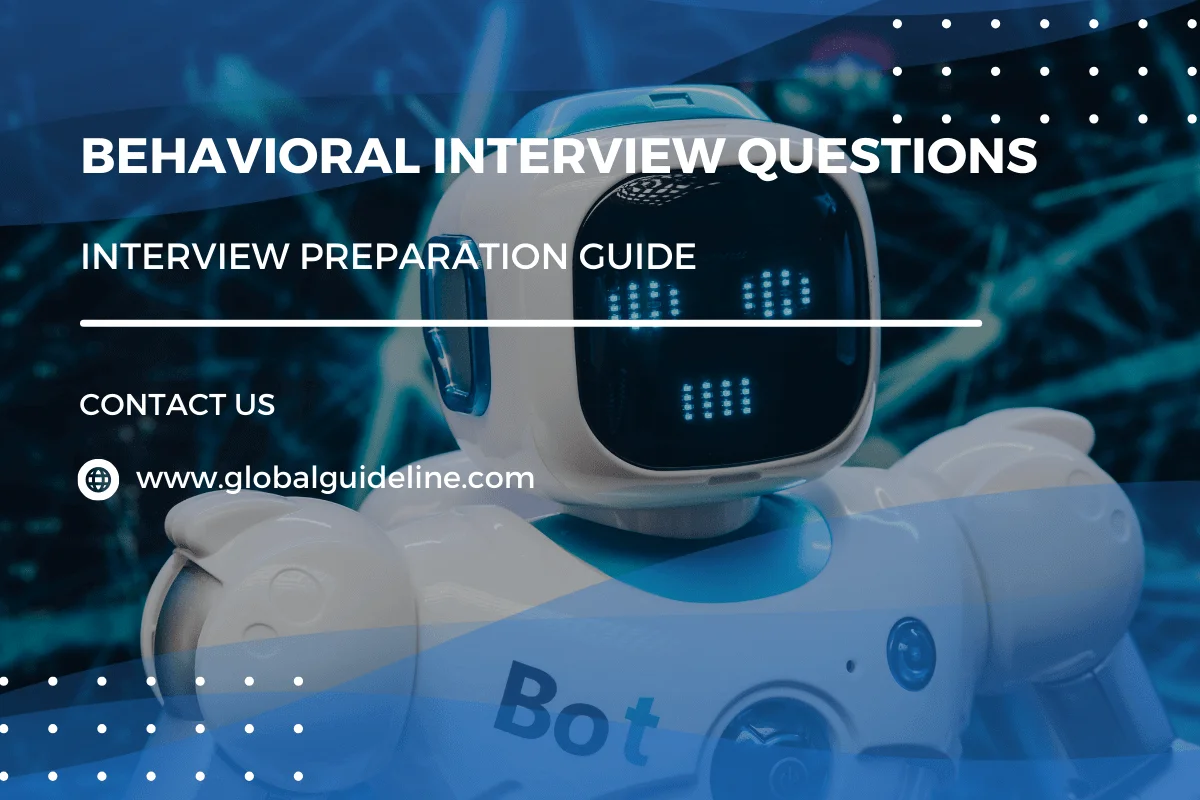
1 :: Write an expression or Perl script to identify the entered IP address is valid or not?
#! /usr/bin/per
use strict;
#- Use Input
#---------------
my $ip_add = "";
#- Script start
#---------------
print"Enter the IP address\n";
$ip_add = <STDIN>;
chomp($ip_add); # Remove the last "\n" character
$ip_add=$ip_add."\."; # Append . at the end of IP address
#-------Expression to check IP address is valid or not-----#
if($ip_add =~ m/^[0-2]?[0-5]?[0-5]?\.){4}$){
print" Valid IP Address\n";
}else{
print" Invalid IP Address\n"
}
Read Moreuse strict;
#- Use Input
#---------------
my $ip_add = "";
#- Script start
#---------------
print"Enter the IP address\n";
$ip_add = <STDIN>;
chomp($ip_add); # Remove the last "\n" character
$ip_add=$ip_add."\."; # Append . at the end of IP address
#-------Expression to check IP address is valid or not-----#
if($ip_add =~ m/^[0-2]?[0-5]?[0-5]?\.){4}$){
print" Valid IP Address\n";
}else{
print" Invalid IP Address\n"
}
2 :: Packing and Unpacking.
Hi, I want to get output as 0x23400000345....
in the below example How to get?
i tried out, but unable to get the answer
$r=0x234;
$t=0x345;
$y=pack(L L,$t,$r);
$x1=unpack(L!,pack(P,$y));
printf("\nThe value is $x1");
I didnt get constant output?
use the following code.
<code>
use bigint;
my $r = 0x234;
my $t = 0x345;
my $x1 = $r << 32 | $t;
print $x1->as_hex;
</code>
Read More<code>
use bigint;
my $r = 0x234;
my $t = 0x345;
my $x1 = $r << 32 | $t;
print $x1->as_hex;
</code>
3 :: How to sort dates in Perl?
Example
-------
use Data::Dumper;
my @dates = ( "02/11/2009" , "20/12/2001" , "21/11/2010" ) ;
@dates = sort { join( '', (split '/', $a)[2,1,0] ) cmp
join( '', (split '/', $b)[2,1,0]) } @dates;
print Dumper \@dates;
Read More-------
use Data::Dumper;
my @dates = ( "02/11/2009" , "20/12/2001" , "21/11/2010" ) ;
@dates = sort { join( '', (split '/', $a)[2,1,0] ) cmp
join( '', (split '/', $b)[2,1,0]) } @dates;
print Dumper \@dates;
4 :: Sort a word "system" in perl/shell without using built in functions output should be emssty?
#!/usr/bin/perl
my $word = $ARGV[0];
$sortword = "";
$lastchar = "";
while($word =~ /(.)/g)
{
$lastchar = $1;
if( $sortword ) {
$flag = "";
$newsortword = "";
while($sortword =~ /(.)/g) {
if( $lastchar gt $1 || $flag
eq "charcovered") {
$newsortword =
$newsortword.$1;
$flag = "greater" if($flag
ne "charcovered")
}
else {
$newsortword =
$newsortword.$lastchar.$1;
$flag = "charcovered";
}
}
if( $flag ne "charcovered" ) {
$newsortword =
$newsortword.$lastchar;
}
$sortword = $newsortword;
}
else {
$sortword = $lastchar;
}
}
print $sortword."\n";
Read Moremy $word = $ARGV[0];
$sortword = "";
$lastchar = "";
while($word =~ /(.)/g)
{
$lastchar = $1;
if( $sortword ) {
$flag = "";
$newsortword = "";
while($sortword =~ /(.)/g) {
if( $lastchar gt $1 || $flag
eq "charcovered") {
$newsortword =
$newsortword.$1;
$flag = "greater" if($flag
ne "charcovered")
}
else {
$newsortword =
$newsortword.$lastchar.$1;
$flag = "charcovered";
}
}
if( $flag ne "charcovered" ) {
$newsortword =
$newsortword.$lastchar;
}
$sortword = $newsortword;
}
else {
$sortword = $lastchar;
}
}
print $sortword."\n";
5 :: How to find a substring in a string without using substr built in functions, and print the substring found?
$r="YASHWANTH";
@n=split (//,$r);
print "@n\n";
@t=splice(@n,0,3);
print "@t\n";
$r=join("",@t);
print "$r\n";
~
~
Read More@n=split (//,$r);
print "@n\n";
@t=splice(@n,0,3);
print "@t\n";
$r=join("",@t);
print "$r\n";
~
~
6 :: Write a script to reverse a string without using Perls built in function?
my $i;
my $str="hello";
my @str=split('',$str);
for($i=$#str;$i>=0;$i--)
{
print $str[$i];
}
Read Moremy $str="hello";
my @str=split('',$str);
for($i=$#str;$i>=0;$i--)
{
print $str[$i];
}
7 :: Try pattern matching for the following:
1) 10.111.23.11
2) /root/abc/cde/fgg/ac.xml --> Get file name without extention.
3) /root/abc/ac.xml/fgg/ac.xml --> Get file name without extention.
4) What does "DIE" meant in PERL?
5) chomp
6) "This is saturday" --> Print the weekday number.
7) 11-2-2009 --> Print the name of the month.
8) Reverse the string without using func in C.?
my $str="This is testing of string reverse";
print scalar reverse $s
chomp is used to remove the trailing new line.
If we give chomp list then it will remove the trailing new
line in all the elements of the list.
die function is used for handling the errors in Perl.
It terminates the program immediately after printing the
message passed to the die function.
die("testing of die");
It will print the message "testing of die" on screen and
terminates the program.
Pattern Matching
----------------
[code]
my $str="10.111.23.11";
my $file="/root/abc/cde/fgg/ac.xml";
my $file1="/root/abc/ac.xml/fgg/ac.xml";
if($str=~/[0-9]{2}\.[0-9]{3}\.[0-9]{2}\.[0-9]{2}/)
{
print "Pattern $& get matched\n";
}
if($file1=~s/\/(.*)\/(.*)\.(.*)/$2/)
{
print "File Name is $file1\n";
}
print strftime("%B",0,0,0,11,2-1,2009);
[/code]
Read Moreprint scalar reverse $s
chomp is used to remove the trailing new line.
If we give chomp list then it will remove the trailing new
line in all the elements of the list.
die function is used for handling the errors in Perl.
It terminates the program immediately after printing the
message passed to the die function.
die("testing of die");
It will print the message "testing of die" on screen and
terminates the program.
Pattern Matching
----------------
[code]
my $str="10.111.23.11";
my $file="/root/abc/cde/fgg/ac.xml";
my $file1="/root/abc/ac.xml/fgg/ac.xml";
if($str=~/[0-9]{2}\.[0-9]{3}\.[0-9]{2}\.[0-9]{2}/)
{
print "Pattern $& get matched\n";
}
if($file1=~s/\/(.*)\/(.*)\.(.*)/$2/)
{
print "File Name is $file1\n";
}
print strftime("%B",0,0,0,11,2-1,2009);
[/code]
8 :: Why do you use only Perl when there a lot of more languages available in market like C, Java?
compare to other c and java perl is having strong regular
expression concept so data extraction will be easier and
complition will be faster too
Read Moreexpression concept so data extraction will be easier and
complition will be faster too
9 :: What is the meaning of rigging?
Rigging is use for if we want to give animation for any
object or character then we apply to character or object
internel bone setting(like our bones).that is called
rigging. when apply rigging, then we can give proper
animation.
Read Moreobject or character then we apply to character or object
internel bone setting(like our bones).that is called
rigging. when apply rigging, then we can give proper
animation.
10 :: What is the difference between having a parenthesis after module name and without parenthsis after module name?
i.e Package::Module();
and Package::Module;
Package::Module(); This will throw as error,
I think,the question should be as: What is the difference
between,
Package::MyModule qw(); # FIRST
and
Package::MyModule; # SECOND
# FIRST :- This will not import any subroutine from MyModule.
# SECOND :- This will import all the subroutine from the
MyModule.
Read MoreI think,the question should be as: What is the difference
between,
Package::MyModule qw(); # FIRST
and
Package::MyModule; # SECOND
# FIRST :- This will not import any subroutine from MyModule.
# SECOND :- This will import all the subroutine from the
MyModule.
11 :: Write a Perl script to find a particular word in a paragraph?
[code]
my $pat;
$pat='Using push we can add multiple items into an array in
a single instance.
If we are trying to add a module or library files in
our program using require or use statement then it will
search that module or library files in the Perl\'s default
search path.
The statement use lib is used to add the directories
to default search path.
So if the module or library file is not located in
the Perl\'s default search path then it will find the
library files in the path we have given with the use lib
$path.';
if($pat=~/push/)
{
print "Pattern push get matched\n";
}
[/code]
Read Moremy $pat;
$pat='Using push we can add multiple items into an array in
a single instance.
If we are trying to add a module or library files in
our program using require or use statement then it will
search that module or library files in the Perl\'s default
search path.
The statement use lib is used to add the directories
to default search path.
So if the module or library file is not located in
the Perl\'s default search path then it will find the
library files in the path we have given with the use lib
$path.';
if($pat=~/push/)
{
print "Pattern push get matched\n";
}
[/code]
12 :: Write a perl script to find whether a given line of text is starting and ending with same word or not?
Lets assume that the text to match is present in a file
say "data.txt".
Following program will print the line containing same
starting and ending word.
open(FILE,"data.txt") or die "cannot open file : $!";
while(<FILE>) {
if($_ =~ /^(\w+)\s+.*?\1$/) {
print "the line is $_ \n";
}
}
Read Moresay "data.txt".
Following program will print the line containing same
starting and ending word.
open(FILE,"data.txt") or die "cannot open file : $!";
while(<FILE>) {
if($_ =~ /^(\w+)\s+.*?\1$/) {
print "the line is $_ \n";
}
}
14 :: How to make the following assignment, as arrayreference assignment?
my $arr_ref=[1,2,3,4,4,elem];
my $ref=[1,2,3,4];
print ref $ref;
ref will return the type of reference.
In this case ref will return as 'ARRAY'.
Read Moreprint ref $ref;
ref will return the type of reference.
In this case ref will return as 'ARRAY'.
15 :: Difference between Perl and Mod_perl?
Perl is a language and MOD_PERL is a module of Apache used
to enhance the performance of the application.
Read Moreto enhance the performance of the application.
16 :: Why we use "use lib $path"?
If we are trying to add a module or library files in our
program using require or use statement then it will search
that module or library files in the Perl's default search path.
The statement use lib is used to add the directories to
default search path.
So if the module or library file is not located in the
Perl's default search path then it will find the library
files in the path we have given with the use lib $path.
Read Moreprogram using require or use statement then it will search
that module or library files in the Perl's default search path.
The statement use lib is used to add the directories to
default search path.
So if the module or library file is not located in the
Perl's default search path then it will find the library
files in the path we have given with the use lib $path.
17 :: Write a script to generate n prime no.s?
#!c:\perl\bin\perl
$Count = 0;
$pt = 2;
while ( $Count < @ARGV[0] )
{
if (isPrimary($pt))
{
print "$pt\n";
$Count++;
}
$pt++;
}
sub isPrimary
{
$flag = 1;
for ($i=2; $i<=$_[0]/2; $i++)
{
if ($_[0] % $i == 0)
{
$flag = 0;
}
}
return $flag;
}
Read More$Count = 0;
$pt = 2;
while ( $Count < @ARGV[0] )
{
if (isPrimary($pt))
{
print "$pt\n";
$Count++;
}
$pt++;
}
sub isPrimary
{
$flag = 1;
for ($i=2; $i<=$_[0]/2; $i++)
{
if ($_[0] % $i == 0)
{
$flag = 0;
}
}
return $flag;
}
18 :: Write a script to display mirror image of a entered value and also check whether Palindrome?
print("Enter the no. to check for Palindrome : ");
$no = <STDIN>;
chop($no);
$i = 0;
# Store into a array
while($no != 0)
{
@array[$i] = $no % 10;
$no = int($no / 10);
$i++;
}
$i--;
$j=0;
$flag = "true";
# Check for Palindrome
while( ($flag eq "true" )&& ( $j < @array/2) ){
if (@array[$j] != @array[$i])
{
$flag = "false"
}
$i--;
$j++;
}
# Print the result
if( $flag eq "true")
{
print("It is a Palindrome\n");
}
else
{
print("It is NOT a Palindrome\n");
}
Read More$no = <STDIN>;
chop($no);
$i = 0;
# Store into a array
while($no != 0)
{
@array[$i] = $no % 10;
$no = int($no / 10);
$i++;
}
$i--;
$j=0;
$flag = "true";
# Check for Palindrome
while( ($flag eq "true" )&& ( $j < @array/2) ){
if (@array[$j] != @array[$i])
{
$flag = "false"
}
$i--;
$j++;
}
# Print the result
if( $flag eq "true")
{
print("It is a Palindrome\n");
}
else
{
print("It is NOT a Palindrome\n");
}