Basic PHP Programming Interview Questions And Answers
Optimize your PHP interview preparation with our curated set of 139 questions. Each question is crafted to challenge your understanding and proficiency in PHP. Suitable for all skill levels, these questions are essential for effective preparation. Download the free PDF now to get all 139 questions and ensure you're well-prepared for your PHP interview. This resource is perfect for in-depth preparation and boosting your confidence.
139 PHP Questions and Answers:
PHP Job Interview Questions Table of Contents:
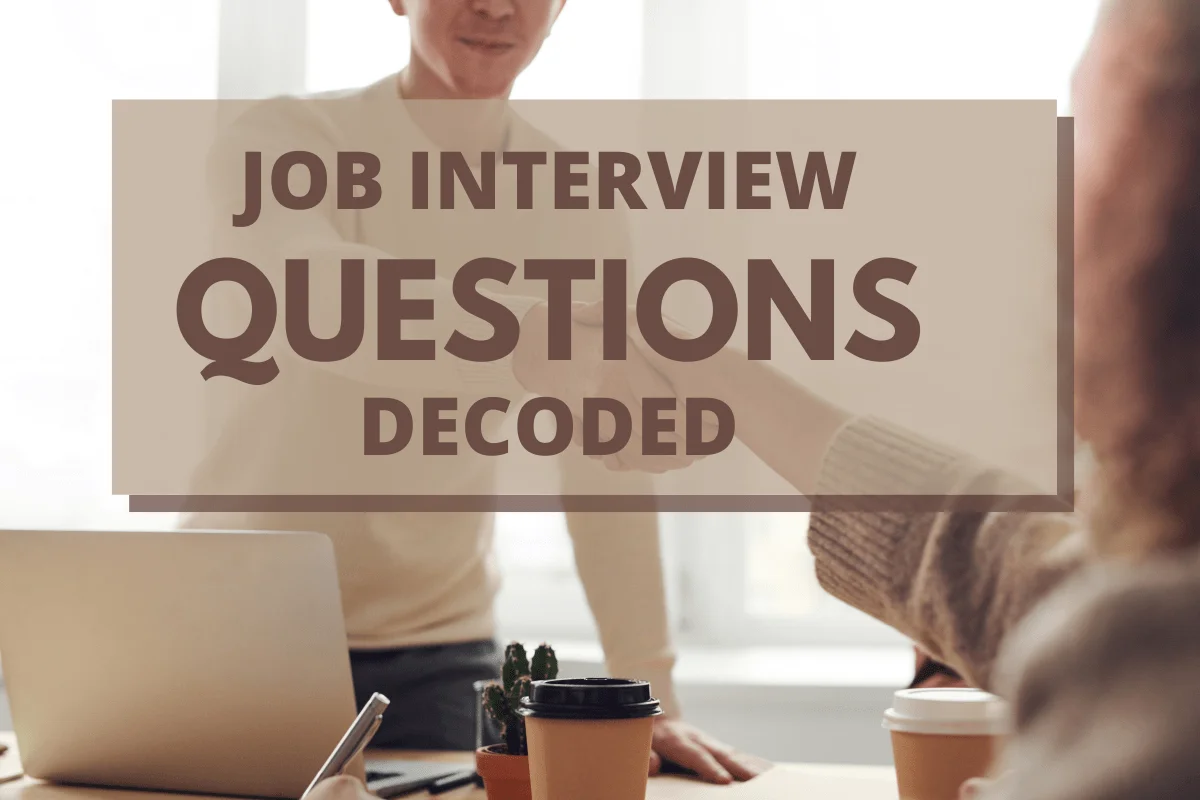
1 :: How To Download and Install PHP on Windows?
PHP is one of the most popular languages to develop dynamic Web pages. It supports all major database servers, including: MySQL, MS SQL Server, Oracle, mSQL, Sybase, etc.
If you are developing a Web application that uses PHP and needs to access MS SQL Server, you should go download and install PHP to your local machine to practice PHP and SQL Server connection. The best way to download and install PHP on Windows systems is to:
* Go to http://www.php.net, which is the official Web site for PHP.
* Click the Downloads menu link. You will see the PHP download page.
* Go to the "Windows Binaries" section, and click "PHP 5.2.3 zip package" link to download PHP binary version for Windows in ZIP format.
* Save the downloaded file, php-5.2.3-Win32.zip with 9,847,499 bytes, in C:Temp directory.
* Unzip the downloaded file into directory C:php.
You are done. No need to run any installation program.
Read MoreIf you are developing a Web application that uses PHP and needs to access MS SQL Server, you should go download and install PHP to your local machine to practice PHP and SQL Server connection. The best way to download and install PHP on Windows systems is to:
* Go to http://www.php.net, which is the official Web site for PHP.
* Click the Downloads menu link. You will see the PHP download page.
* Go to the "Windows Binaries" section, and click "PHP 5.2.3 zip package" link to download PHP binary version for Windows in ZIP format.
* Save the downloaded file, php-5.2.3-Win32.zip with 9,847,499 bytes, in C:Temp directory.
* Unzip the downloaded file into directory C:php.
You are done. No need to run any installation program.
2 :: How To Check Your PHP Installation?
PHP provides two execution interfaces: Command Line Interface (CLI) and Common Gateway Interface (CGI). If PHP is installed in the php directory on your system, you can try this to check your installation:
* Run "phpphp -v" command to check the Command Line Interface (CLI).
* Run "phpphp-cgi -v" command to check the Common Gateway Interface (CGI).
If you see PHP printing the version information on your screen for both commands, your installation is ok. Open a command window and run the commands below:
C:>phpphp -v
PHP 5.2.2 (cli) (built: May 2 2007 19:18:26)
Copyright (c) 1997-2007 The PHP Group
Zend Engine v2.2.0 Copyright (c) 1998-2007 Zend Technologies
C:>phpphp-cgi -v
PHP 5.2.2 (cgi-fcgi) (built: May 2 2007 19:18:25)
Copyright (c) 1997-2007 The PHP Group
Zend Engine v2.2.0 Copyright (c) 1998-2007 Zend Technologies
Read More* Run "phpphp -v" command to check the Command Line Interface (CLI).
* Run "phpphp-cgi -v" command to check the Common Gateway Interface (CGI).
If you see PHP printing the version information on your screen for both commands, your installation is ok. Open a command window and run the commands below:
C:>phpphp -v
PHP 5.2.2 (cli) (built: May 2 2007 19:18:26)
Copyright (c) 1997-2007 The PHP Group
Zend Engine v2.2.0 Copyright (c) 1998-2007 Zend Technologies
C:>phpphp-cgi -v
PHP 5.2.2 (cgi-fcgi) (built: May 2 2007 19:18:25)
Copyright (c) 1997-2007 The PHP Group
Zend Engine v2.2.0 Copyright (c) 1998-2007 Zend Technologies
3 :: How To Download and Install PHP for Windows?
The best way to download and install PHP on Windows systems is to:
► Go to http://www.php.net, which is the official Web site for PHP.
► Download PHP binary version for Windows in ZIP format.
► Unzip the downloaded file into a directory.
You are done. No need to run any install program.
Read More► Go to http://www.php.net, which is the official Web site for PHP.
► Download PHP binary version for Windows in ZIP format.
► Unzip the downloaded file into a directory.
You are done. No need to run any install program.
4 :: Where Are PHP Configuration Settings Stored?
PHP stores configuration settings in a file called php.ini in PHP home directory. You can open it with any text editor to your settings.
Read More5 :: How To Run a PHP Script?
A standard alone PHP script can be executed directly with the PHP Command Line Interface (CLI). Write the following script in a file called hello.php:
<?php echo "Hello world!"; ?>
This script can be executed by CLI interface like this:
phpphp hello.php
You should see the "Hello world!" message printed on your screen.
Read More<?php echo "Hello world!"; ?>
This script can be executed by CLI interface like this:
phpphp hello.php
You should see the "Hello world!" message printed on your screen.
6 :: What Are the Special Characters You Need to Escape in Single-Quoted Stings?
There are two special characters you need to escape in a single-quote string: the single quote (') and the back slash (). Here is a PHP script example of single-quoted strings:
<?php
echo 'Hello world!';
echo 'It's Friday!';
echo ' represents an operator.';
?>
This script will print:
Hello world!It's Friday! represents an operator.
Read More<?php
echo 'Hello world!';
echo 'It's Friday!';
echo ' represents an operator.';
?>
This script will print:
Hello world!It's Friday! represents an operator.
7 :: Can You Specify the "new line" Character in Single-Quoted Strings?
You can not specify the "new line" character in a single-quoted string. If you don't believe, try this script:
<?php
echo ' will not work in single quoted strings.';
?>
This script will print:
will not work in single quoted strings.
How Many Escape Sequences Are Recognized in Single-Quoted Strings?
There are 2 escape sequences you can use in single-quoted strings:
► - Represents the back slash character.
► ' - Represents the single quote character.
Read More<?php
echo ' will not work in single quoted strings.';
?>
This script will print:
will not work in single quoted strings.
How Many Escape Sequences Are Recognized in Single-Quoted Strings?
There are 2 escape sequences you can use in single-quoted strings:
► - Represents the back slash character.
► ' - Represents the single quote character.
8 :: What Are the Special Characters You Need to Escape in Double-Quoted Stings?
There are two special characters you need to escape in a double-quote string: the double quote (") and the back slash (). Here is a PHP script example of double-quoted strings:
<?php
echo "Hello world!";
echo "Tom said: "Who's there?"";
echo " represents an operator.";
?>
This script will print:
Hello world!Tom said: "Who's there?" represents an operator.
Read More<?php
echo "Hello world!";
echo "Tom said: "Who's there?"";
echo " represents an operator.";
?>
This script will print:
Hello world!Tom said: "Who's there?" represents an operator.
9 :: How Many Escape Sequences Are Recognized in Double-Quoted Strings in PHP?
There are 12 escape sequences you can use in double-quoted strings:
► - Represents the back slash character.
► " - Represents the double quote character.
► $ - Represents the dollar sign.
► - Represents the new line character (ASCII code 10).
► - Represents the carriage return character (ASCII code 13).
► - Represents the tab character (ASCII code 9).
► { - Represents the open brace character.
► } - Represents the close brace character.
► [ - Represents the open bracket character.
► ] - Represents the close bracket character.
► nn - Represents a character as an octal value.
► xnn - Represents a character as a hex value.
Read More► - Represents the back slash character.
► " - Represents the double quote character.
► $ - Represents the dollar sign.
► - Represents the new line character (ASCII code 10).
► - Represents the carriage return character (ASCII code 13).
► - Represents the tab character (ASCII code 9).
► { - Represents the open brace character.
► } - Represents the close brace character.
► [ - Represents the open bracket character.
► ] - Represents the close bracket character.
► nn - Represents a character as an octal value.
► xnn - Represents a character as a hex value.
10 :: How To Include Variables in Double-Quoted Strings in PHP?
Variables included in double-quoted strings will be interpolated. Their values will be concatenated into the enclosing strings. For example, two statements in the following PHP script will print out the same string:
<?php
$variable = "and";
echo "part 1 $variable part 2 ";
echo "part 1 ".$variable." part 2 ";
?>
This script will print:
part 1 and part 2
part 1 and part 2
Read More<?php
$variable = "and";
echo "part 1 $variable part 2 ";
echo "part 1 ".$variable." part 2 ";
?>
This script will print:
part 1 and part 2
part 1 and part 2
11 :: How Many Ways to Include Variables in Double-Quoted Strings in PHP?
There are 3 formats to include variables in double-quoted strings:
► "part 1 $variable part 2" - This is the simplest format to include a variable in a string. The variable name starts with the dollar sign and ends at the first character that can not be used in variable name. Space is good character to end a variable name.
► "part 1${variable}part 2" - This format helps you to clearly end the variable name. The variable name starts at dollar sign before the open brace (${) and ends at the close brace (}).
► "part 1{$variable}part 2" - This format is also called complex format. You use this format to specify any complex variable expression in the same way as in a normal statement. The variable expression starts at ({$) followed by a variable name and ends at (}).
Here is a PHP script example of different ways to include variables in double-quoted strings:
<?php
$beer = 'Heineken';
echo "$beer's taste is great. ";
echo "He drank some ${beer}s and water. ";
echo "She drank some {$beer}s and water. ";
?>
This script will print:
Heineken's taste is great.
He drank some Heinekens and water.
She drank some Heinekens and water.
Read More► "part 1 $variable part 2" - This is the simplest format to include a variable in a string. The variable name starts with the dollar sign and ends at the first character that can not be used in variable name. Space is good character to end a variable name.
► "part 1${variable}part 2" - This format helps you to clearly end the variable name. The variable name starts at dollar sign before the open brace (${) and ends at the close brace (}).
► "part 1{$variable}part 2" - This format is also called complex format. You use this format to specify any complex variable expression in the same way as in a normal statement. The variable expression starts at ({$) followed by a variable name and ends at (}).
Here is a PHP script example of different ways to include variables in double-quoted strings:
<?php
$beer = 'Heineken';
echo "$beer's taste is great. ";
echo "He drank some ${beer}s and water. ";
echo "She drank some {$beer}s and water. ";
?>
This script will print:
Heineken's taste is great.
He drank some Heinekens and water.
She drank some Heinekens and water.
12 :: How Many Ways to Include Array Elements in Double-Quoted Strings using PHP?
There are 2 formats to include array elements in double-quoted strings in PHP:
► "part 1 $array[key] part 2" - This is called simple format. In this format, you can not specify the element key in quotes.
► "part 1 {$array['key']} part 2" - This is called complex format. In this format, the array element expression is specified in the same way as in a normal statement.
Here is a PHP script example of different ways to include variables in double-quoted strings:
<?php
$fruits = array('strawberry' => 'red', 'banana' => 'yellow');
echo "A banana is $fruits[banana]. ";
echo "A banana is {$fruits['banana']}. ";
?>
This script will print:
A banana is yellow.
A banana is yellow.
"A banana is $fruits['banana']. " will give you a syntax error.
Read More► "part 1 $array[key] part 2" - This is called simple format. In this format, you can not specify the element key in quotes.
► "part 1 {$array['key']} part 2" - This is called complex format. In this format, the array element expression is specified in the same way as in a normal statement.
Here is a PHP script example of different ways to include variables in double-quoted strings:
<?php
$fruits = array('strawberry' => 'red', 'banana' => 'yellow');
echo "A banana is $fruits[banana]. ";
echo "A banana is {$fruits['banana']}. ";
?>
This script will print:
A banana is yellow.
A banana is yellow.
"A banana is $fruits['banana']. " will give you a syntax error.
13 :: How To Access a Specific Character in a String using PHP?
Any character in a string can be accessed by a special string element expression:
► $string{index} - The index is the position of the character counted from left and starting from 0.
Here is a PHP script example:
<?php
$string = 'It's Friday!';
echo "The first character is $string{0} ";
echo "The first character is {$string{0}} ";
?>
This script will print:
The first character is It's Friday!{0}
The first character is I
Read More► $string{index} - The index is the position of the character counted from left and starting from 0.
Here is a PHP script example:
<?php
$string = 'It's Friday!';
echo "The first character is $string{0} ";
echo "The first character is {$string{0}} ";
?>
This script will print:
The first character is It's Friday!{0}
The first character is I
14 :: How To Assigning a New Character in a String using PHP?
The string element expression, $string{index}, can also be used at the left side of an assignment statement. This allows you to assign a new character to any position in a string. Here is a PHP script example:
<?php
$string = 'It's Friday?';
echo "$string ";
$string{11} = '!';
echo "$string ";
?>
This script will print:
It's Friday?
It's Friday!
Read More<?php
$string = 'It's Friday?';
echo "$string ";
$string{11} = '!';
echo "$string ";
?>
This script will print:
It's Friday?
It's Friday!
15 :: How To Concatenate Two Strings Together in PHP?
You can use the string concatenation operator (.) to join two strings into one. Here is a PHP script example of string concatenation:
<?php
echo 'Hello ' . "world! ";
?>
This script will print:
Hello world!
Read More<?php
echo 'Hello ' . "world! ";
?>
This script will print:
Hello world!
16 :: How To Compare Two Strings with Comparison Operators in PHP?
PHP supports 3 string comparison operators, <, ==, and >, that generates Boolean values. Those operators use ASCII values of characters from both strings to determine the comparison results. Here is a PHP script on how to use comparison operators:
<?php
$a = "PHP is a scripting language.";
$b = "PHP is a general-purpose language.";
if ($a > $b) {
print('$a > $b is true.'." ");
} else {
print('$a > $b is false.'." ");
}
if ($a == $b) {
print('$a == $b is true.'." ");
} else {
print('$a == $b is false.'." ");
}
if ($a < $b) {
print('$a < $b is true.'." ");
} else {
print('$a < $b is false.'." ");
}
?>
This script will print:
$a > $b is true.
$a == $b is false.
$a < $b is false.
Read More<?php
$a = "PHP is a scripting language.";
$b = "PHP is a general-purpose language.";
if ($a > $b) {
print('$a > $b is true.'." ");
} else {
print('$a > $b is false.'." ");
}
if ($a == $b) {
print('$a == $b is true.'." ");
} else {
print('$a == $b is false.'." ");
}
if ($a < $b) {
print('$a < $b is true.'." ");
} else {
print('$a < $b is false.'." ");
}
?>
This script will print:
$a > $b is true.
$a == $b is false.
$a < $b is false.
17 :: How To Convert Numbers to Strings in PHP?
In a string context, PHP will automatically convert any numeric value to a string. Here is a PHP script examples:
<?php
print(-1.3e3);
print(" ");
print(strlen(-1.3e3));
print(" ");
print("Price = $" . 99.99 . " ");
print(1 . " + " . 2 . " = " . 1+2 . " ");
print(1 . " + " . 2 . " = " . (1+2) . " ");
print(1 . " + " . 2 . " = 3 ");
print(" ");
?>
This script will print:
-1300
5
Price = $99.99
3
1 + 2 = 3
1 + 2 = 3
The print() function requires a string, so numeric value -1.3e3 is automatically converted to a string "-1300". The concatenation operator (.) also requires a string, so numeric value 99.99 is automatically converted to a string "99.99". Expression (1 . " + " . 2 . " = " . 1+2 . " ") is a little bit interesting. The result is "3 " because concatenation operations and addition operation are carried out from left to right. So when the addition operation is reached, we have "1 + 2 = 1"+2, which will cause the string to be converted to a value 1.
Read More<?php
print(-1.3e3);
print(" ");
print(strlen(-1.3e3));
print(" ");
print("Price = $" . 99.99 . " ");
print(1 . " + " . 2 . " = " . 1+2 . " ");
print(1 . " + " . 2 . " = " . (1+2) . " ");
print(1 . " + " . 2 . " = 3 ");
print(" ");
?>
This script will print:
-1300
5
Price = $99.99
3
1 + 2 = 3
1 + 2 = 3
The print() function requires a string, so numeric value -1.3e3 is automatically converted to a string "-1300". The concatenation operator (.) also requires a string, so numeric value 99.99 is automatically converted to a string "99.99". Expression (1 . " + " . 2 . " = " . 1+2 . " ") is a little bit interesting. The result is "3 " because concatenation operations and addition operation are carried out from left to right. So when the addition operation is reached, we have "1 + 2 = 1"+2, which will cause the string to be converted to a value 1.
18 :: How To Convert Strings to Numbers in PHP?
In a numeric context, PHP will automatically convert any string to a numeric value. Strings will be converted into two types of numeric values, double floating number and integer, based on the following rules:
► The value is given by the initial portion of the string. If the string starts with valid numeric data, this will be the value used. Otherwise, the value will be 0 (zero).
► If the valid numeric data contains '.', 'e', or 'E', it will be converted to a double floating number. Otherwise, it will be converted to an integer.
Read More► The value is given by the initial portion of the string. If the string starts with valid numeric data, this will be the value used. Otherwise, the value will be 0 (zero).
► If the valid numeric data contains '.', 'e', or 'E', it will be converted to a double floating number. Otherwise, it will be converted to an integer.
19 :: How To Get the Number of Characters in a String?
You can use the "strlen()" function to get the number of characters in a string. Here is a PHP script example of strlen():
<?php
print(strlen('It's Friday!'));
?>
This script will print:
12
Read More<?php
print(strlen('It's Friday!'));
?>
This script will print:
12
20 :: How To Remove White Spaces from the Beginning and/or the End of a String in PHP?
There are 4 PHP functions you can use remove white space characters from the beginning and/or the end of a string:
► trim() - Remove white space characters from the beginning and the end of a string.
► ltrim() - Remove white space characters from the beginning of a string.
► rtrim() - Remove white space characters from the end of a string.
► chop() - Same as rtrim().
White space characters are defined as:
► " " (ASCII 32 (0x20)), an ordinary space.
► " " (ASCII 9 (0x09)), a tab.
► " " (ASCII 10 (0x0A)), a new line (line feed).
► " " (ASCII 13 (0x0D)), a carriage return.
► "" (ASCII 0 (0x00)), the NULL-byte.
► "x0B" (ASCII 11 (0x0B)), a vertical tab.
Here is a PHP script example of trimming strings:
<?php
$text = " Hello world! ";
$leftTrimmed = ltrim($text);
$rightTrimmed = rtrim($text);
$bothTrimmed = trim($text);
print("leftTrimmed = ($leftTrimmed) ");
print("rightTrimmed = ($rightTrimmed) ");
print("bothTrimmed = ($bothTrimmed) ");
?>
This script will print:
<pre>leftTrimmed = (Hello world! )
rightTrimmed = ( Hello world!)
bothTrimmed = (Hello world!)</pre>
Read More► trim() - Remove white space characters from the beginning and the end of a string.
► ltrim() - Remove white space characters from the beginning of a string.
► rtrim() - Remove white space characters from the end of a string.
► chop() - Same as rtrim().
White space characters are defined as:
► " " (ASCII 32 (0x20)), an ordinary space.
► " " (ASCII 9 (0x09)), a tab.
► " " (ASCII 10 (0x0A)), a new line (line feed).
► " " (ASCII 13 (0x0D)), a carriage return.
► "" (ASCII 0 (0x00)), the NULL-byte.
► "x0B" (ASCII 11 (0x0B)), a vertical tab.
Here is a PHP script example of trimming strings:
<?php
$text = " Hello world! ";
$leftTrimmed = ltrim($text);
$rightTrimmed = rtrim($text);
$bothTrimmed = trim($text);
print("leftTrimmed = ($leftTrimmed) ");
print("rightTrimmed = ($rightTrimmed) ");
print("bothTrimmed = ($bothTrimmed) ");
?>
This script will print:
<pre>leftTrimmed = (Hello world! )
rightTrimmed = ( Hello world!)
bothTrimmed = (Hello world!)</pre>
21 :: How To Remove the New Line Character from the End of a Text Line in PHP?
If you are using fgets() to read a line from a text file, you may want to use the chop() function to remove the new line character from the end of the line as shown in this PHP script:
<?php
$handle = fopen("/tmp/inputfile.txt", "r");
while ($line=fgets()) {
$line = chop($line);
# process $line here...
}
fclose($handle);
?>
Read More<?php
$handle = fopen("/tmp/inputfile.txt", "r");
while ($line=fgets()) {
$line = chop($line);
# process $line here...
}
fclose($handle);
?>
22 :: How To Remove Leading and Trailing Spaces from User Input Values in PHP?
If you are taking input values from users with a Web form, users may enter extra spaces at the beginning and/or the end of the input values. You should always use the trim() function to remove those extra spaces as shown in this PHP script:
<?php
$name = $_REQUEST("name");
$name = trim($name);
# $name is ready to be used...
?>
Read More<?php
$name = $_REQUEST("name");
$name = trim($name);
# $name is ready to be used...
?>
23 :: How to Find a Substring from a Given String in PHP?
To find a substring in a given string, you can use the strpos() function. If you call strpos($haystack, $needle), it will try to find the position of the first occurrence of the $needle string in the $haystack string. If found, it will return a non-negative integer represents the position of $needle. Othewise, it will return a Boolean false. Here is a PHP script example of strpos():
<?php
$haystack1 = "2349534134345globalguideline16504381640386488129";
$haystack2 = "globalguideline234953413434516504381640386488129";
$haystack3 = "guideline234953413434516504381640386488129ggl";
$pos1 = strpos($haystack1, "globalguideline");
$pos2 = strpos($haystack2, "globalguideline");
$pos3 = strpos($haystack3, "globalguideline");
print("pos1 = ($pos1); type is " . gettype($pos1) . " ");
print("pos2 = ($pos2); type is " . gettype($pos2) . " ");
print("pos3 = ($pos3); type is " . gettype($pos3) . " ");
?>
This script will print:
pos1 = (13); type is integer
pos2 = (0); type is integer
pos3 = (); type is boolean
"pos3" shows strpos() can return a Boolean value
Read More<?php
$haystack1 = "2349534134345globalguideline16504381640386488129";
$haystack2 = "globalguideline234953413434516504381640386488129";
$haystack3 = "guideline234953413434516504381640386488129ggl";
$pos1 = strpos($haystack1, "globalguideline");
$pos2 = strpos($haystack2, "globalguideline");
$pos3 = strpos($haystack3, "globalguideline");
print("pos1 = ($pos1); type is " . gettype($pos1) . " ");
print("pos2 = ($pos2); type is " . gettype($pos2) . " ");
print("pos3 = ($pos3); type is " . gettype($pos3) . " ");
?>
This script will print:
pos1 = (13); type is integer
pos2 = (0); type is integer
pos3 = (); type is boolean
"pos3" shows strpos() can return a Boolean value
24 :: What Is the Best Way to Test the strpos() Return Value in PHP?
Because strpos() could two types of values, Integer and Boolean, you need to be careful about testing the return value. The best way is to use the "Identical(===)" operator. Do not use the "Equal(==)" operator, because it does not differentiate "0" and "false". Check out this PHP script on how to use strpos():
<?php
$haystack = "needle234953413434516504381640386488129";
$pos = strpos($haystack, "needle");
if ($pos==false) {
print("Not found based (==) test ");
} else {
print("Found based (==) test ");
}
if ($pos===false) {
print("Not found based (===) test ");
} else {
print("Found based (===) test ");
}
?>
This script will print:
Not found based (==) test
Found based (===) test
Of course, (===) test is correct.
Read More<?php
$haystack = "needle234953413434516504381640386488129";
$pos = strpos($haystack, "needle");
if ($pos==false) {
print("Not found based (==) test ");
} else {
print("Found based (==) test ");
}
if ($pos===false) {
print("Not found based (===) test ");
} else {
print("Found based (===) test ");
}
?>
This script will print:
Not found based (==) test
Found based (===) test
Of course, (===) test is correct.
25 :: How To Take a Substring from a Given String in PHP?
If you know the position of a substring in a given string, you can take the substring out by the substr() function. Here is a PHP script on how to use substr():
<?php
$string = "beginning";
print("Position counted from left: ".substr($string,0,5)." ");
print("Position counted form right: ".substr($string,-7,3)." ");
?>
This script will print:
Position counted from left: begin
Position counted form right: gin
substr() can take negative starting position counted from the end of the string.
Read More<?php
$string = "beginning";
print("Position counted from left: ".substr($string,0,5)." ");
print("Position counted form right: ".substr($string,-7,3)." ");
?>
This script will print:
Position counted from left: begin
Position counted form right: gin
substr() can take negative starting position counted from the end of the string.