Unity 3D Developer Question: Download Questions PDF
Tell me the Issue With The Code Below And Provide An Alternative Implementation That Would Correct The Problem.
Using Unityengine;
Using System.collections;
Public Class Test : Monobehaviour {
Void Start () {
Transform.position.x = 10;
}
}?
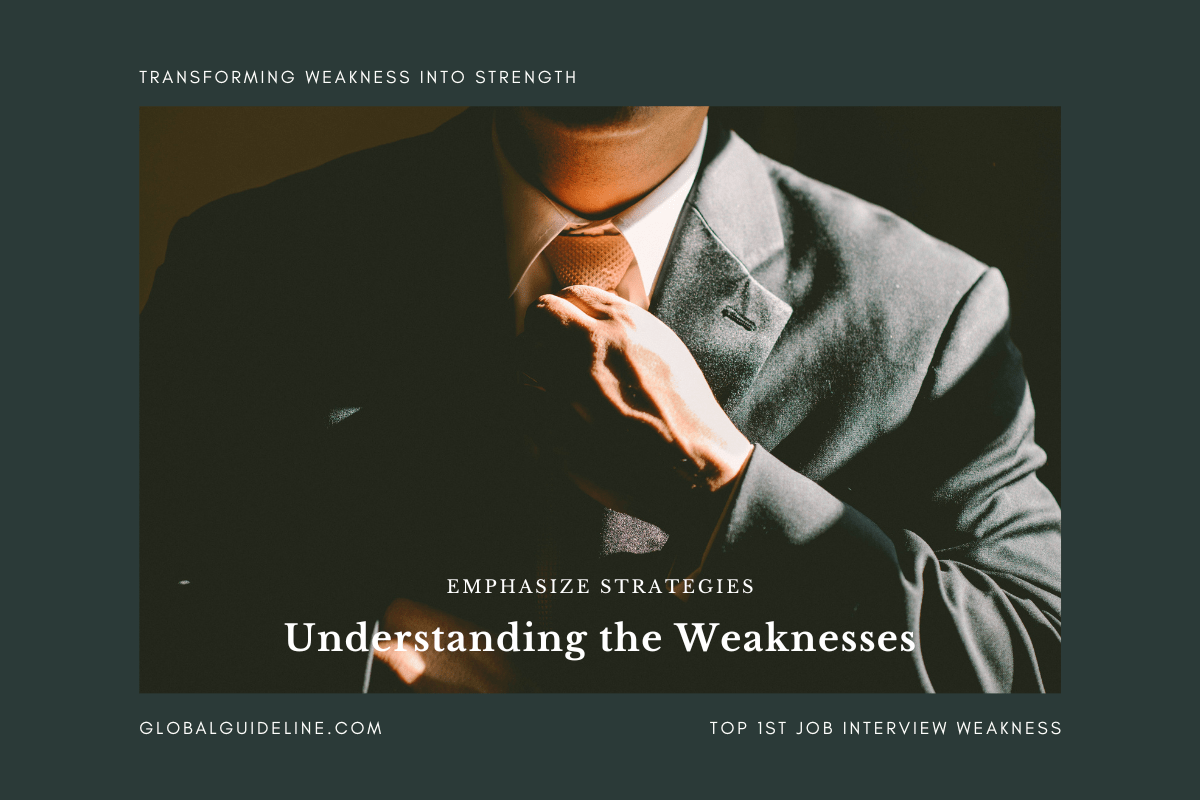
Answer:
The issue is that you can't modify the position from a transform directly. This is because the position is actually a property (not a field). Therefore, when a getter is called, it invokes a method which returns a Vector3 copy which it places into the stack.
So basically what you are doing in the code above is assigning a member of the struct a value that is in the stack and that is later removed.
Instead, the proper solution is to replace the whole property; e.g.:
using UnityEngine;
using System.Collections;
public class TEST : MonoBehaviour {
void Start () {
Vector3 newPos = new Vector3(10, transform.position.y, transform.position.z);
transform.position = newPos;
}
}
So basically what you are doing in the code above is assigning a member of the struct a value that is in the stack and that is later removed.
Instead, the proper solution is to replace the whole property; e.g.:
using UnityEngine;
using System.Collections;
public class TEST : MonoBehaviour {
void Start () {
Vector3 newPos = new Vector3(10, transform.position.y, transform.position.z);
transform.position = newPos;
}
}
Download Unity 3D Developer Interview Questions And Answers
PDF