Basic Analyst Integration Interview Preparation Guide Download PDF
Analyst Integration related Frequently Asked Questions in various Integration Programmer job Interviews by interviewer. The set of questions here ensures that you offer a perfect answer posed to you. So get preparation for your new job hunting
41 Integration Programmer Questions and Answers:
Table of Contents:
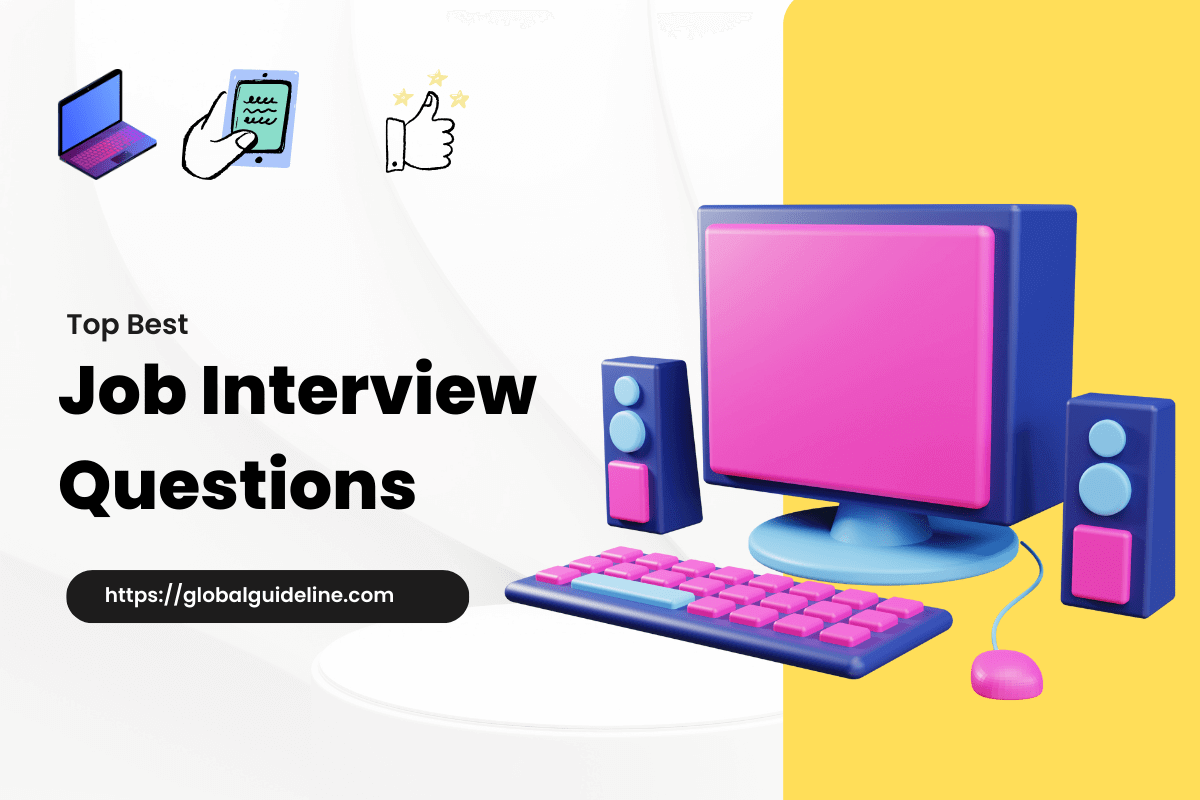
1 :: Explain regular expression?
Regular expression is a way to perform pattern matching on text data. It's very powerful tool to find something e.g. some character in a long string e.g. finding if a book contains some word or not. Almost all major programming language supports regular expression but Perl has been renowned for its enormous capability. Java also supports Perl like regular expression using java.util.regex package. You can use regular expression to check if a email is valid or not, if a phone number is valid, or if a zip code is valid, or even a SSN number is valid or not. One of the simplest example of regular expression is to check if a String is number or not.
2 :: Can you please explain the difference between linked list and an array?
linked list and array are two of the most important data structure in programming world. Most significant difference between them is that array stores its element at contiguous location while linked list stores its data anywhere in memory. This gives linked list enormous flexibility to expand itself because memory is always scattered. It's always possible that you wouldn't be able to create an array to store 1M integers but can do by using linked list because space is available but not as contiguous chunk. All other differences are result of this fact. For example you can search an element in array with O(1) time if you know the index but searching will take O(n) time in linked list. For more differences see the detailed answer.
3 :: Explain shell script?
shell script is set of shell commands with some programming constructs e.g. if and for loop, which allow you to automate some repetitive task. For example, you can write shell script to daily cleanup of logs files, for backing up data for historical use and for other housekeeping jobs, releases and monitoring.
4 :: How to find large files in UNIX e.g. more than 1GB?
You can easily find big files by using find command because it provides option to search files based upon there size. Use this if your file system is full and your Java process is crashing with no more space. This command will list all files which is more than 1GB. You can tweak the size easily e.g. to find all files with more than 100 MB just use +100M.
find . - type f -size +1G -print
find . - type f -size +1G -print
5 :: Can you please explain the difference between correlated and non-correlated subquery?
In correlated sub-query, inner query depends upon outer query and executes for each row in outer query. While non-correlated sub query doesn't depend upon outer query and can be executed independently. Due to this reason former is slow and later is fast. BTW, correlated subquery has some nice application, one of them is finding Nth highest salary in Employee table, as seen on previous SQL question as well.
6 :: How to find a running Java process on UNIX?
You can use combination of 'ps' and 'grep' command to find any process running on UNIX machine. Suppose your Java process has a name or any text which you can use to match against just use following command.
ps -ef | grep "myJavaApp"
ps -e will list every process i.e. process from all user not just you and ps -f will give you full details including PID, which will be required if you want to investigate more or would like to kill this process using kill command.
ps -ef | grep "myJavaApp"
ps -e will list every process i.e. process from all user not just you and ps -f will give you full details including PID, which will be required if you want to investigate more or would like to kill this process using kill command.
7 :: How to find if a number is power of two, without using arithmetic operator?
Assume its a question about using bitwise operator as soon as you hear restriction about not allowed to use arithmetic operator. If that restriction is not in place then you can easily check if a number is power of two by using modulus and division operator. By the using bitwise operator, there is a nice trick to do this. You can use following code to check if a number if power of two or no.
8 :: Write an SQL query to find second highest salary in employee table?
This is one of the classic question from SQL interviews, event it's quite old it is still interesting and has lots of follow-up you can use to check depth of candidate's knowledge. You can find second highest salary by using correlated and non-correlated sub query. You can also use keyword's like TOP or LIMIT if you are using SQL Server or MySQL, given Interviewer allows you. The simplest way to find 2nd highest salary is following :
SELECT MAX(Salary) FROM Employee WHERE Salary NOT IN (SELECT MAX(Salary) FROM Employee)
This query first find maximum salary and then exclude that from list and again finds maximum salary. Obviously second time, it would be second highest salary.
SELECT MAX(Salary) FROM Employee WHERE Salary NOT IN (SELECT MAX(Salary) FROM Employee)
This query first find maximum salary and then exclude that from list and again finds maximum salary. Obviously second time, it would be second highest salary.
9 :: Explain stateless system?
A stateless system is a system which doesn't maintain any internal state. Such system will produce same output for same input at any point of time. It's always easier to code and optimize a stateless system, so you should always strive for one if possible.
10 :: Explain couple of ways to resolve collision in hash table?
Linear probing, double hashing, and chaining. In linear probing, if bucket is already occupied then function check next bucket linearly until it find an empty one, while in chaining, multiple elements are stored in same bucket location.
11 :: Explain about unit testing?
Unit testing is way to test individual unit for their functionality instead of testing whole application. There are lot of tools to do the unit testing in different programming language e.g. in Java, you can use JUnit or TestNG to write unit tests. It is often run automatically during build process or in a continuous environment like Jenkins.
12 :: Explain time complexity of an algorithm?
Time complexity specify the ratio of time to the input. It shows how much time an algorithm will take to complete for a given number of input. It's approximated valued but enough to give you an indication that how your algorithm will perform if number of input is increased from 10 to 10 million.
13 :: Can you please explain the difference between binary tree and a binary search tree?
Binary search tree is an ordered binary tree, where value of all nodes in left tree are less than or equal to node and values of all nodes in right sub tree is greater than or equal to node (e.g. root). It's an important data structure and can be used to represent a sorted structure.
14 :: Explain recursive algorithm?
There are lots of places where recursive algorithm fits e.g. algorithm related to binary and linked list. Couple of examples of recursive algorithm is reversing String and calculating Fibonacci series. Other examples include reversing linked list, tree traversal, and quick sort algorithm.
15 :: Explain Open closed design principle?
Open closed is another principle from SOLID, which asserts that a system should be open for extension but close for modification. Which means if a new functionality is required in a stable system then your tried and tested code should not be touched and new functionality should be provided by adding new classes only.
16 :: Explain Liskov substitution principle?
Liskov substitution principle is one of the five principle introduced by Uncle Bob as SOLID design principles. It's the 'L' in SOLID. Liskov substitution principle asserts that every sub type should be able to work as proxy for parent type. For example, if a method except object of Parent class then it should work as expected if you pass an object of Child class. Any class which cannot stand in place of its parent violate LSP or Liskov substitution principle. This is actually a tough question to answer and if you does that you end up with creating a good impression on interviewers mind.
17 :: Explain test-driven development?
Test driven is one of the popular development methodology in which tests are written before writing any function code. In fact, test drives the structure of your program. Purists never wrote a single line of application code without writing test for that. It greatly improve code quality and often attributed as a quality of rockstar developers.
18 :: Explain the result of 1 XOR 1?
Answer is zero, because XOR returns 1 if two operands are distinct and zero if two operands are same, for example 0 XOR 0 is also zero, but 0 XOR 1 or 1 XOR 0 is always 1.
19 :: How to get the last digit of an integer?
By using modulus operator, number % 10 returns the last digit of the number, for example 2345%10 will return 5 and 567%10 will return 7. Similarly division operator can be used to get rid of last digit of a number e.g. 2345/10 will give 234 and 567/10 will return 56. This is an important technique to know and useful to solve problems like number palindrome or reversing numbers.
20 :: Can you please explain the difference between iteration and recursion?
Iteration uses loop to perform same step again and again while recursion calls function itself to do the repetitive task. Many times recursion result in a clear and concise solution of complex problem e.g. tower of Hanoi, reversing a linked list or reversing a String itself. One drawback of recursion is depth, since recursion stores intermediate result in stack you can only go upto certain depth, after that your program will die with StackOverFlowError, this is why iteration is preferred over recursion in production code.
21 :: Describe three different kinds of testing that might be performed on an application before it goes live?
Unit testing, integration testing and smoke testing. Unit testing is used to test individual units to verify whether they are working as expected, integration testing is done to verify whether individually tested module can work together or not and smoke testing is a way to test whether most common functionality of software is working properly or not e.g. in a flight booking website, you should be able to book, cancel or change flights.
22 :: Explain inner join and a left join in SQL?
In SQL, there are mainly two types of joins, inner join and outer join. Again outer joins can be two types right and left outer join. Main difference between inner join and left join is that in case of former only matching records from both tables are selected while in case of left join, all records from left table is selected in addition to matching records from both tables. Always watch out for queries which has "all" in it, they usually require left join e.g. write sql query to find all departments and number of employees on it. If you use inner join to solve this query, you will missed empty departments where no one works.
23 :: Can you please explain the difference between an interface and an abstract class?
This is the most classical question of all programming interviews. An interface is the purest form of abstraction with nothing concrete in place, while an abstract class is a combination of some abstraction and concrete things. The difference may vary depending upon language e.g. in Java you can extend multiple interface but you can only extend on abstract class. For a more comprehensive discussion see the detailed answer.
24 :: Can you please explain the difference between composition, aggregation and association?
Association means two objects are related to each other but can exists without each other, Composition is a form of association where one object is composed of multiple object, but they only exists together e.g. human body is composition of organs, individual organs cannot live they only useful in body. Aggregation is collection of object e.g. city is aggregation of citizens.
25 :: Explain loose-coupling?
Loose coupling is a desirable quality of software, which allows one part of software to modify without affecting other part of software. For example in a loosely coupled software a change in UI layout should not affect the back-end class structure.
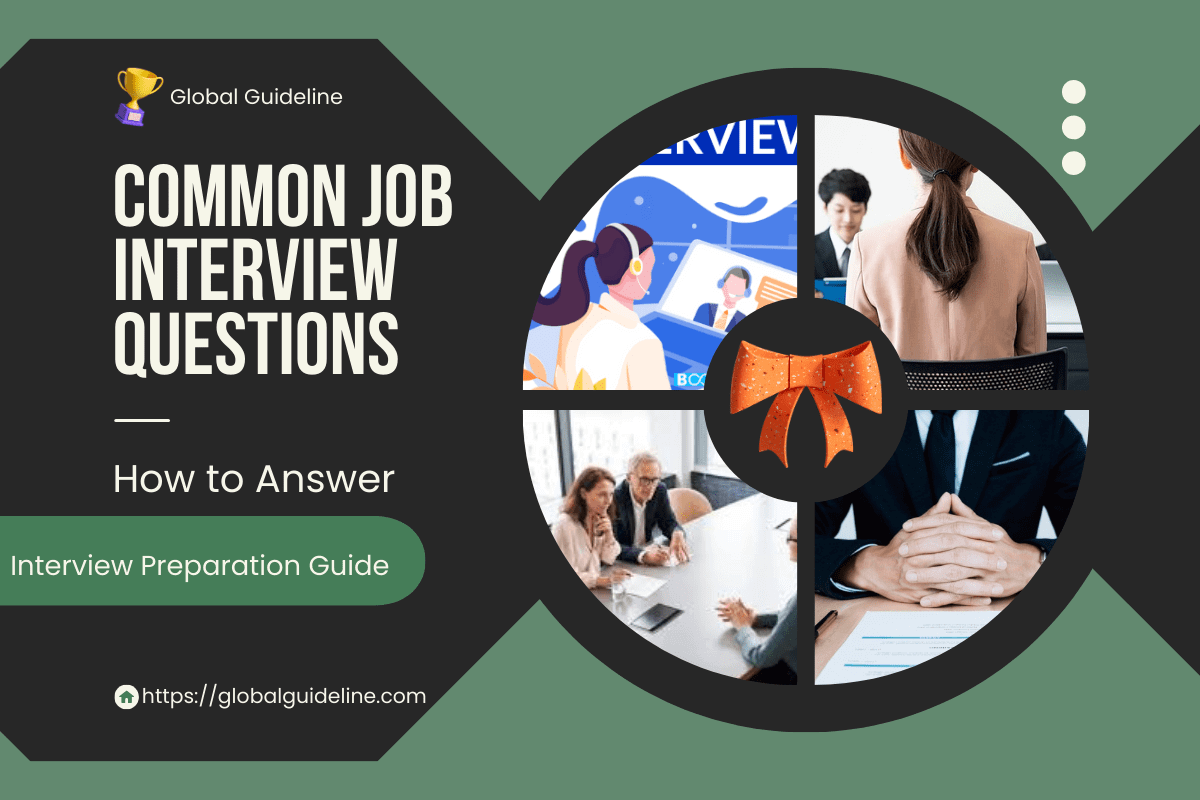