Ruby Developer Interview Questions And Answers
Prepare comprehensively for your Ruby Developer interview with our extensive list of 74 questions. Each question is crafted to challenge your understanding and proficiency in Ruby Developer. Suitable for all skill levels, these questions are essential for effective preparation. Access the free PDF to get all 74 questions and give yourself the best chance of acing your Ruby Developer interview. This resource is perfect for thorough preparation and confidence building.
74 Ruby Developer Questions and Answers:
Ruby Developer Job Interview Questions Table of Contents:
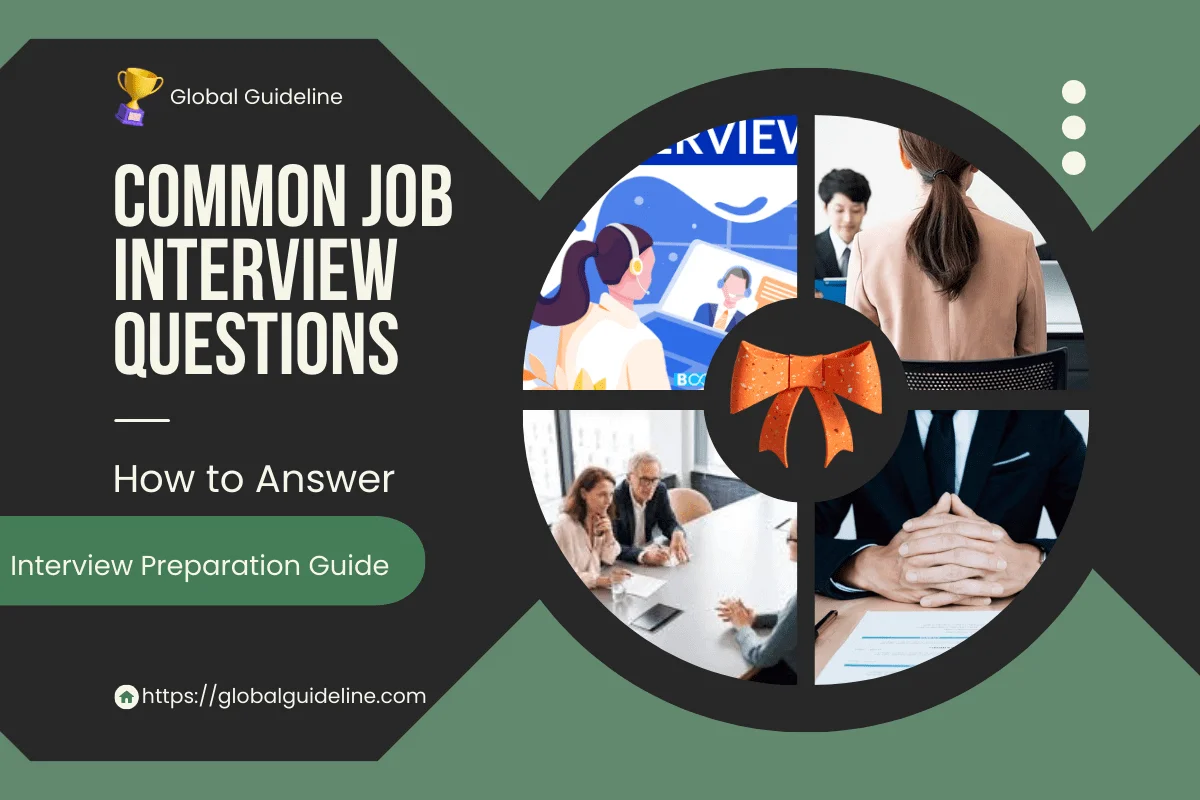
1 :: Tell us what is class libraries in Ruby?
Class libraries in Ruby consist of a variety of domains, such as data types, thread programming, various domains, etc.
Read More2 :: Tell me can you call a private method outside a Ruby class using its object?
Yes, with the help of the send method.
Given the class Test:
class Test
private
def method
p "I am a private method"
end
end
We can execute the private method using send:
>> Test.new.send(:method)
"I am a private method"
Read MoreGiven the class Test:
class Test
private
def method
p "I am a private method"
end
end
We can execute the private method using send:
>> Test.new.send(:method)
"I am a private method"
3 :: Explain me what is the function of ORM in Ruby on Rails?
ORM stands for Object Relationship Model that models the classes and helps in setting a relationship between the existing models.
Functions:
1. It allows the classes to be mapped to the table that is present in the database and objects get mapped to the rows in database table.
2. It shows the relationship that exists between the object and frame it using the model to display the output to the users.
3. It keeps the data in the database according to its relationships and performs the functions accordingly.
Read MoreFunctions:
1. It allows the classes to be mapped to the table that is present in the database and objects get mapped to the rows in database table.
2. It shows the relationship that exists between the object and frame it using the model to display the output to the users.
3. It keeps the data in the database according to its relationships and performs the functions accordingly.
4 :: Tell me the role of modules and mixins in Ruby?
Modules are Ruby’s way of grouping methods, classes, and constants together to provide a namespace for preventing name clashes. The second purpose of modules is to use them as mixins. Technically, Ruby only supports single inheritance, but by using modules as mixins, it is possible to share code among different classes—a key advantage of multiple inheritance—without having to give up the simplicity of the single inheritance paradigm.
Read More5 :: What is the difference extend and include?
The “include” makes the module’s methods available to the instance of a class, while “extend” makes these methods available to the class itself.
Read More6 :: What is the syntax for Ruby collect Iterator?
The syntax for Ruby collect Iterator collection = collection.collect.
Read More7 :: What is the difference between put and putc statement?
Unlike the puts statement, which outputs the entire string onto the screen. The Putc statement can be used to output one character at a time.
Read More8 :: Tell me how can we define Ruby regular expressions?
Ruby regular expression is a special sequence of characters that helps you match or find other strings. A regular expression literal is a pattern between arbitrary delimiters or slashes followed by %r.
Read More9 :: Tell us what is the difference between Dynamic and Static Scaffolding?
Dynamic Scaffolding:
It automatically creates the entire content and user interface at runtime
It enables to generation of new, delete, edit methods for the use in application
It does not need a database to be synchronized
Static Scaffolding:
It requires manual entry in the command to create the data with their fields
It does not require any such generation to take place
It requires the database to be migrated
Read MoreIt automatically creates the entire content and user interface at runtime
It enables to generation of new, delete, edit methods for the use in application
It does not need a database to be synchronized
Static Scaffolding:
It requires manual entry in the command to create the data with their fields
It does not require any such generation to take place
It requires the database to be migrated
10 :: Do you know what is rake in Rails?
Rake is a Ruby Make; it is a Ruby utility that substitutes the Unix utility ‘make’, and uses a ‘Rakefile’ and ‘.rake files’ to build up a list of tasks. In Rails, Rake is used for normal administration tasks like migrating the database through scripts, loading a schema into the database, etc.
Read More11 :: Tell me what is the difference between Active support’s “HashWithIndifferent” and Ruby’s “Hash” ?
The Hash class in Ruby’s core library returns value by using a standard “= =” comparison on the keys. It means that the value stored for a symbol key cannot be retrieved using the equivalent string. While the HashWithIndifferentAccess treats Symbol keys and String keys as equivalent.
Read More12 :: Can you list out what can Rails Migration do?
Rails Migration can do following things
☛ Create table
☛ Drop table
☛ Rename table
☛ Add column
☛ Rename column
☛ Change column
☛ Remove column and so on
Read More☛ Create table
☛ Drop table
☛ Rename table
☛ Add column
☛ Rename column
☛ Change column
☛ Remove column and so on
13 :: Tell me how Symbol is different from variables?
Symbol is different from variables in following aspects
☛ It is more like a string than variable
☛ In Ruby string is mutable but a Symbol is immutable
☛ Only one copy of the symbol requires to be created
☛ Symbols are often used as the corresponding to enums in Ruby
Read More☛ It is more like a string than variable
☛ In Ruby string is mutable but a Symbol is immutable
☛ Only one copy of the symbol requires to be created
☛ Symbols are often used as the corresponding to enums in Ruby
14 :: Explain me what the difference is between false and nil in Ruby?
In Ruby False indicates a Boolean datatype, while Nil is not a data type, it have an object_id 4.
Read More15 :: Tell me what is Ruby on Rails?
Ruby: It is an object oriented programming language inspired by PERL and PYTHON.
Rails: It is a framework used for building web application
Read MoreRails: It is a framework used for building web application
16 :: Tell me what is the role of sub-directory app/controllers and app/helpers?
☛ App/controllers: A web request from the user is handled by the Controller. The controller sub-directory is where Rails looks to find controller classes
☛ App/helpers: The helper’s sub-directory holds any helper classes used to assist the view, model and controller classes.
Read More☛ App/helpers: The helper’s sub-directory holds any helper classes used to assist the view, model and controller classes.
17 :: Tell me what is the command to create a migration?
To create migration command includes
C:rubyapplication>ruby script/generate migration table_name
Read MoreC:rubyapplication>ruby script/generate migration table_name
18 :: Explain how you can run Rails application without creating databases?
You can execute your application by uncommenting the line in environment.rb
path=> rootpath conf/environment.rb
config.frameworks = [ action_web_service, :action_mailer, :active_record]
Read Morepath=> rootpath conf/environment.rb
config.frameworks = [ action_web_service, :action_mailer, :active_record]
19 :: Tell me what is the difference between calling super() and super call?
☛ super(): A call to super() invokes the parent method without any arguments, as presumably expected. As always, being explicit in your code is a good thing.
☛ super call: A call to super invokes the parent method with the same arguments that were passed to the child method. An error will therefore occur if the arguments passed to the child method don’t match what the parent is expecting.
Read More☛ super call: A call to super invokes the parent method with the same arguments that were passed to the child method. An error will therefore occur if the arguments passed to the child method don’t match what the parent is expecting.
20 :: What is the Notation used for denoting class variables in Ruby?
In Ruby,
☛ A constant should begin with an uppercase letter, and it should not be defined inside a method
☛ A local must begin with the _ underscore sign or a lowercase letter
☛ A global variable should begin with the $ sign. An uninitialized global has the value of “nil” and it should raise a warning. It can be referred anywhere in the program.
☛ A class variable should begin with double @@ and have to be first initialized before being used in a method definition
Read More☛ A constant should begin with an uppercase letter, and it should not be defined inside a method
☛ A local must begin with the _ underscore sign or a lowercase letter
☛ A global variable should begin with the $ sign. An uninitialized global has the value of “nil” and it should raise a warning. It can be referred anywhere in the program.
☛ A class variable should begin with double @@ and have to be first initialized before being used in a method definition
21 :: As you know ruby provides four types of variables. List them and provide a brief explanation for each?
The four types of variables in Ruby are as follows:
☛ Global variables begin with $ and are accessible from anywhere within the Ruby program regardless of where they are declared—it stands to reason that they must be handled with care.
☛ Local variables begin with a lowercase letter or an underscore. The scope of a local variable is confined to the code construct within which it is declared.
☛ Class variables begin with @@ and are shared by all instances of the class that it is defined in.
☛ Instance variables begin with @ and are similar to class variables except that they are local to a single instance of a class in which they are instantiated.
Read More☛ Global variables begin with $ and are accessible from anywhere within the Ruby program regardless of where they are declared—it stands to reason that they must be handled with care.
☛ Local variables begin with a lowercase letter or an underscore. The scope of a local variable is confined to the code construct within which it is declared.
☛ Class variables begin with @@ and are shared by all instances of the class that it is defined in.
☛ Instance variables begin with @ and are similar to class variables except that they are local to a single instance of a class in which they are instantiated.
22 :: Please explain what is MVC and why do we use it?
MVC stands for Model View Controller.
Model is the data structure that your program uses.
The View is the part that interacts with the screen or the next level up.
The Controller generally processes data between the model and view.
For example, a model will define your table in the database, the controller will dictate what to do behind the scenes and what to display in the view next. The view part generates the user interface which presents data to the user.
Let’s take an example of creating a form. The submit form will be a view file, from that view file, when you click on the submit button it goes to the respective controller where you have written your business code about what happens on submitting the application/form, and from that controller the data will be saved in the database.
Thus MVC helps to split up the the business logic, database access and presentation. This is very useful in most web applications, and now lately into software/desktop applications.
Read MoreModel is the data structure that your program uses.
The View is the part that interacts with the screen or the next level up.
The Controller generally processes data between the model and view.
For example, a model will define your table in the database, the controller will dictate what to do behind the scenes and what to display in the view next. The view part generates the user interface which presents data to the user.
Let’s take an example of creating a form. The submit form will be a view file, from that view file, when you click on the submit button it goes to the respective controller where you have written your business code about what happens on submitting the application/form, and from that controller the data will be saved in the database.
Thus MVC helps to split up the the business logic, database access and presentation. This is very useful in most web applications, and now lately into software/desktop applications.
23 :: Tell me an array [1,2,34,5,6,7,8,9], product it using a method?
def productarray()
return array.inject(:*)
end
Read Morereturn array.inject(:*)
end
24 :: Please explain each of the following operators and how and when they should be used: ==, ===, eql?, equal?
== – Checks if the value of two operands are equal (often overridden to provide a class-specific definition of equality).
=== – Specifically used to test equality within the when clause of a case statement (also often overridden to provide meaningful class-specific semantics in case statements).
eql? – Checks if the value and type of two operands are the same (as opposed to the == operator which compares values but ignores types). For example, 1 == 1.0 evaluates to true, whereas 1.eql?(1.0) evaluates to false.
equal? – Compares the identity of two objects; i.e., returns true iff both operands have the same object id (i.e., if they both refer to the same object). Note that this will return false when comparing two identical copies of the same object.
Read More=== – Specifically used to test equality within the when clause of a case statement (also often overridden to provide meaningful class-specific semantics in case statements).
eql? – Checks if the value and type of two operands are the same (as opposed to the == operator which compares values but ignores types). For example, 1 == 1.0 evaluates to true, whereas 1.eql?(1.0) evaluates to false.
equal? – Compares the identity of two objects; i.e., returns true iff both operands have the same object id (i.e., if they both refer to the same object). Note that this will return false when comparing two identical copies of the same object.
25 :: Explain me what is the difference between Symbol and String?
☛ The symbol in Ruby on rails act the same way as the string but the difference is in their behaviors that are opposite to each other.
☛ The difference remains in the object_id, memory and process time for both of them when used together at one time.
☛ Strings are considered as mutable objects. Whereas, symbols, belongs to the category of immutable.
☛ Strings objects are mutable so that it takes only the assignments to change the object information. Whereas, information of, immutable objects gets overwritten.
☛ String objects are written like
p: “string object jack”.object_id #=>2250 or
p: “string object jack”.to_sym.object_id #=> 2260, and
p: “string object jack”. to_s_object_id #=> 2270
☛ Symbols are used to show the values for the actions like equality or non-equality to test the symbols faster then the string values.
Read More☛ The difference remains in the object_id, memory and process time for both of them when used together at one time.
☛ Strings are considered as mutable objects. Whereas, symbols, belongs to the category of immutable.
☛ Strings objects are mutable so that it takes only the assignments to change the object information. Whereas, information of, immutable objects gets overwritten.
☛ String objects are written like
p: “string object jack”.object_id #=>2250 or
p: “string object jack”.to_sym.object_id #=> 2260, and
p: “string object jack”. to_s_object_id #=> 2270
☛ Symbols are used to show the values for the actions like equality or non-equality to test the symbols faster then the string values.