Python Developer Interview Questions & Answers Download PDF
Sharpen your Python Developer interview expertise with our handpicked 77 questions. These questions will test your expertise and readiness for any Python Developer interview scenario. Ideal for candidates of all levels, this collection is a must-have for your study plan. Don't miss out on our free PDF download, containing all 77 questions to help you succeed in your Python Developer interview. It's an invaluable tool for reinforcing your knowledge and building confidence.
77 Python Developer Questions and Answers:
Python Developer Job Interview Questions Table of Contents:
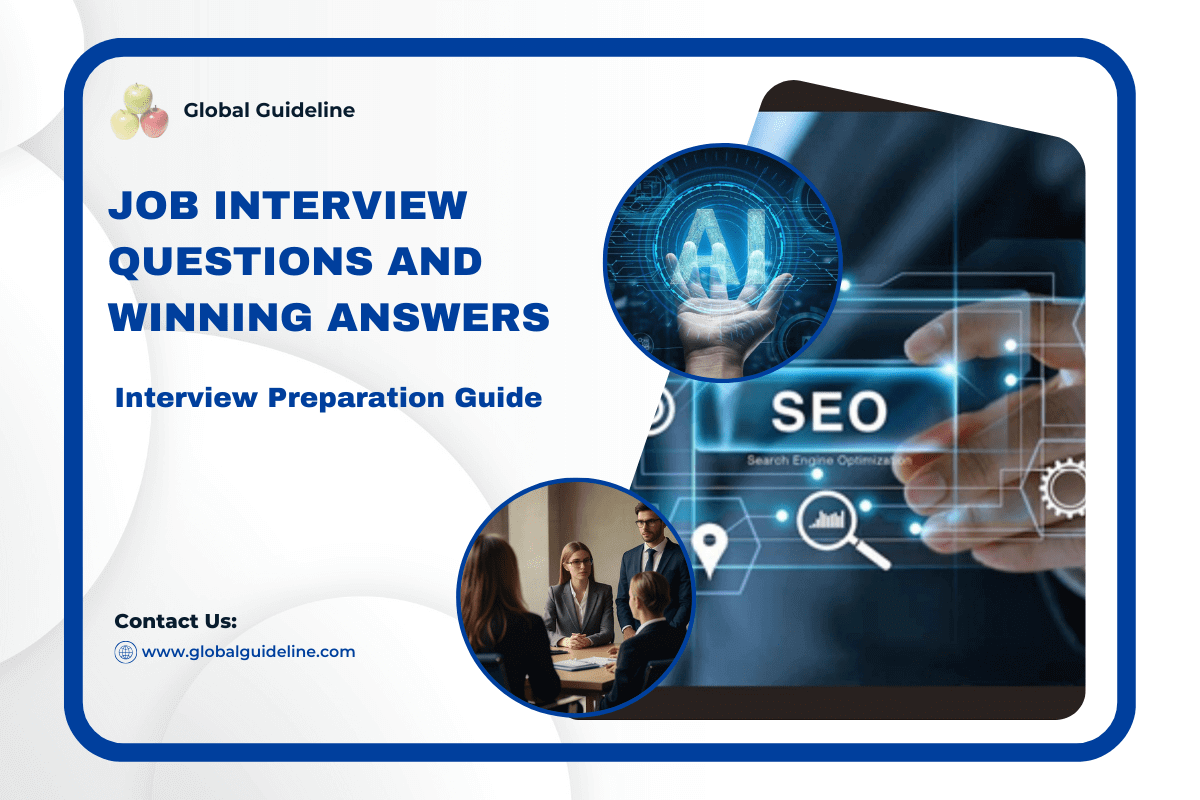
1 :: Tell me what are generators in Python?
The way of implementing iterators are known as generators. It is a normal function except that it yields expression in the function.
2 :: Tell me what is pickling and unpickling?
Pickle module accepts any Python object and converts it into a string representation and dumps it into a file by using dump function, this process is called pickling. While the process of retrieving original Python objects from the stored string representation is called unpickling.
3 :: How to copy an object in Python?
To copy an object in Python, you can try copy.copy () or copy.deepcopy() for the general case. You cannot copy all objects but most of them.
4 :: Tell me what is the difference between list and tuple?
The difference between list and tuple is that list is mutable while tuple is not. Tuple can be hashed for e.g as a key for dictionaries.
5 :: Tell me what is docstring in Python?
A Python documentation string is known as docstring, it is a way of documenting Python functions, modules and classes.
6 :: Explain me what is Python and explain some of its benefits?
Python is a programming language with objects, modules, threads, exceptions and automatic memory management. The benefits of pythons are that it is simple and easy, portable, extensible, build-in data structure and it is an open source.
7 :: Tell me the use of the split function in Python?
The use of the split function in Python is that it breaks a string into shorter strings using the defined separator. It gives a list of all words present in the string.
8 :: Explain me what is unittest in Python?
A unit testing framework in Python is known as unittest. It supports sharing of setups, automation testing, shutdown code for tests, aggregation of tests into collections etc.
9 :: How to convert a number to a string?
In order to convert a number into a string, use the inbuilt function str(). If you want a octal or hexadecimal representation, use the inbuilt function oct() or hex().
10 :: Do you know how Python is interpreted?
Python language is an interpreted language. Python program runs directly from the source code. It converts the source code that is written by the programmer into an intermediate language, which is again translated into machine language that has to be executed.
11 :: Explain me what Is The Purpose Of Doc Strings In Python?
In Python, documentation string is popularly known as doc strings. It sets a process of recording Python functions, modules, and classes.
12 :: How to access sessions in Flask?
A session basically allows you to remember information from one request to another. In a flask, it uses a signed cookie so the user can look at the session contents and modify. The user can modify the session if only it has the secret key Flask.secret_key.
13 :: Tell me what is Dict and List comprehensions are?
They are syntax constructions to ease the creation of a Dictionary or List based on existing iterable.
14 :: Explain me five benefits of using Python?
☛ Python comprises of a huge standard library for most Internet platforms like Email, HTML, etc.
☛ Python does not require explicit memory management as the interpreter itself allocates the memory to new variables and free them automatically
☛ Provide easy readability due to use of square brackets
☛ Easy-to-learn for beginners
☛ Having the built-in data types saves programming time and effort from declaring variables
☛ Python does not require explicit memory management as the interpreter itself allocates the memory to new variables and free them automatically
☛ Provide easy readability due to use of square brackets
☛ Easy-to-learn for beginners
☛ Having the built-in data types saves programming time and effort from declaring variables
15 :: Tell me how are arguments passed by value or by reference?
Everything in Python is an object and all variables hold references to the objects. The references values are according to the functions; as a result you cannot change the value of the references. However, you can change the objects if it is mutable.
16 :: Explain me what Does The <Self> Keyword Do?
The <self> keyword is a variable that holds the instance of an object. In almost, all the object-oriented languages, it is passed to the methods as hidden parameter.
17 :: Explain me what is Flask-WTF and what are their features?
Flask-WTF offers simple integration with WTForms. Features include for Flask WTF are
☛ Integration with wtforms
☛ Secure form with csrf token
☛ Global csrf protection
☛ Internationalization integration
☛ Recaptcha supporting
☛ File upload that works with Flask Uploads
☛ Integration with wtforms
☛ Secure form with csrf token
☛ Global csrf protection
☛ Internationalization integration
☛ Recaptcha supporting
☛ File upload that works with Flask Uploads
18 :: How to access a module written in Python from C?
You can access a module written in Python from C by following method,
Module = =PyImport_ImportModule(“<modulename>”);
Module = =PyImport_ImportModule(“<modulename>”);
19 :: Tell me how Does Python Handle The Memory Management?
☛ Python uses private heaps to maintain its memory. So the heap holds all the Python objects and the data structures. This area is only accessible to the Python interpreter; programmers can’t use it.
☛ And it’s the Python memory manager that handles the Private heap. It does the required allocation of the heap for Python objects.
☛ Python employs a built-in garbage collector, which salvages all the unused memory and offloads it to the heap space.
☛ And it’s the Python memory manager that handles the Private heap. It does the required allocation of the heap for Python objects.
☛ Python employs a built-in garbage collector, which salvages all the unused memory and offloads it to the heap space.
20 :: Do you know how is memory managed in Python?
☛ Python memory is managed by Python private heap space. All Python objects and data structures are located in a private heap. The programmer does not have an access to this private heap and interpreter takes care of this Python private heap.
☛ The allocation of Python heap space for Python objects is done by Python memory manager. The core API gives access to some tools for the programmer to code.
☛ Python also have an inbuilt garbage collector, which recycle all the unused memory and frees the memory and makes it available to the heap space.
☛ The allocation of Python heap space for Python objects is done by Python memory manager. The core API gives access to some tools for the programmer to code.
☛ Python also have an inbuilt garbage collector, which recycle all the unused memory and frees the memory and makes it available to the heap space.
21 :: Tell me how Do You Debug A Program In Python? Is It Possible To Step Through Python Code?
Yes, we can use the Python debugger (<pdb>) to debug any Python program. And if we start a program using <pdb>, then it let us even step through the code.
22 :: Tell me what Do You Think Is The Output Of The Following Code Fragment? Is There Any Error In The Code?
list = ['a', 'b', 'c', 'd', 'e']
print (list[10:])
The result of the above lines of code is []. There won’t be any error like an IndexError.
You should know that trying to fetch a member from the list using an index that exceeds the member count (for example, attempting to access list[10] as given in the question) would yield an IndexError. By the way, retrieving only a slice at an opening index that surpasses the no. of items in the list won’t result in an IndexError. It will just return an empty list.
print (list[10:])
The result of the above lines of code is []. There won’t be any error like an IndexError.
You should know that trying to fetch a member from the list using an index that exceeds the member count (for example, attempting to access list[10] as given in the question) would yield an IndexError. By the way, retrieving only a slice at an opening index that surpasses the no. of items in the list won’t result in an IndexError. It will just return an empty list.
23 :: Please explain when Is The Python Decorator Used?
Python decorator is a relative change that you do in Python syntax to adjust the functions quickly.
24 :: Tell me what Is The Command To Debug A Python Program?
The following command helps run a Python program in debug mode.
$ python -m pdb python-script.py
$ python -m pdb python-script.py
25 :: Do you know why lambda forms in python does not have statements?
A lambda form in python does not have statements as it is used to make new function object and then return them at runtime.
