Operational C++ Operator Overloading Interview Preparation Guide Download PDF
C++ operator overloading job test questions and answers guide. The one who provides the best answers with a perfect presentation is the one who wins the job hunting race. Learn C++ Operator Overloading and get preparation for the new job
26 C++ Operator Overloading Questions and Answers:
Table of Contents:
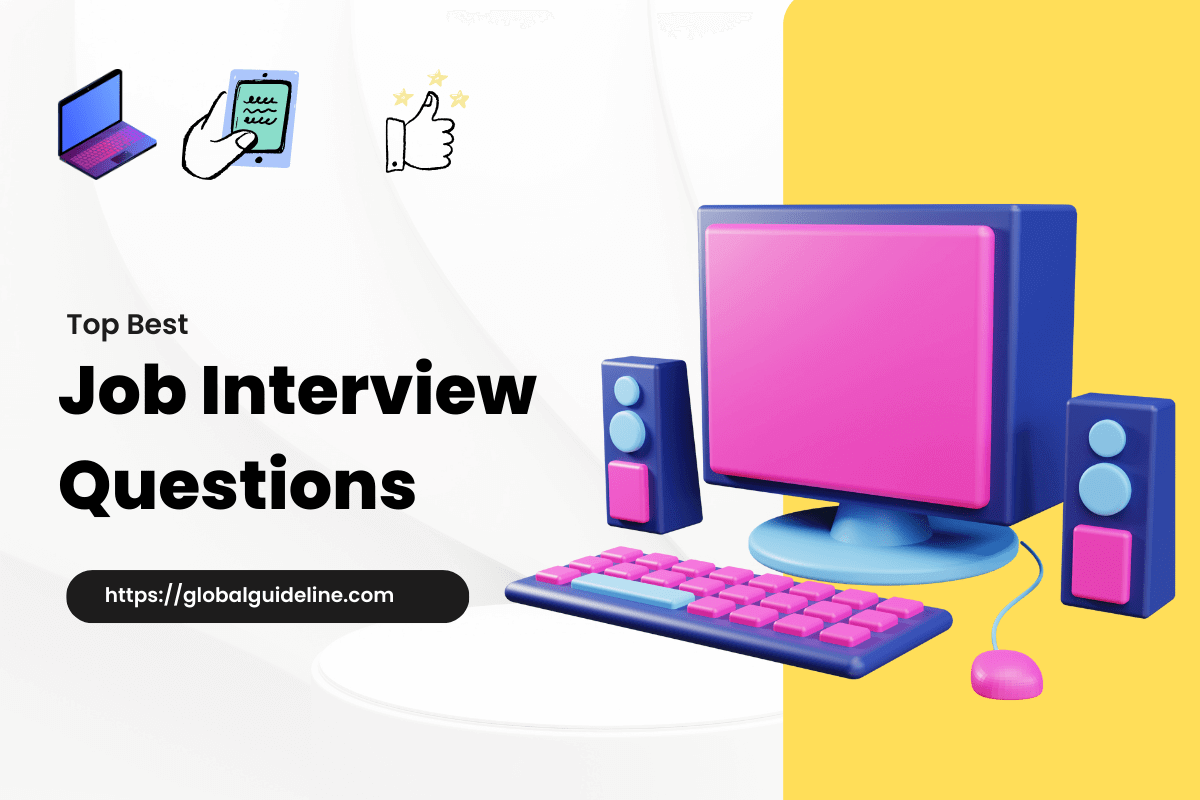
1 :: Pick the other name of operator function.
a) function overloading
b) operator overloading
c) member overloading
d) None of the mentioned
b) operator overloading
2 :: How to declare operator function?
a) operator operator sign
b) operator
c) operator sign
d) None of the mentioned
a) operator operator sign
10 :: Pick out the correct syntax of operator conversion.
a) operator float()const
b) operator float()
c) operator const
d) None of the mentioned
a) operator float()const
17 :: How many parameters does a conversion operator may take?
a) 0
b) 1
c) 2
d) as many as possible
a) 0
18 :: Why we use the "dynamic_cast" type conversion?
a) result of the type conversion is a valid
b) to be used in low memory
c) result of the type conversion is a invalid
d) None of the mentioned
a) result of the type conversion is a valid
19 :: What is the return type of the conversion operator?
a) void
b) int
c) float
d) no return type
d) no return type
20 :: Can you please explain the difference between overloaded functions and overridden functions?
Overloading is a static or compile-time binding and Overriding is dynamic or run-time binding.
Redefining a function in a derived class is called function overriding
A derived class can override a base-class member function by supplying a new version of that function with the same signature (if the signature were different, this would be function overloading rather than function overriding).
Redefining a function in a derived class is called function overriding
A derived class can override a base-class member function by supplying a new version of that function with the same signature (if the signature were different, this would be function overloading rather than function overriding).
21 :: Explain overloading unary operator?
Unary operators are those which operate on a single variable. Overloading unary operator means extending the operator’s original functionality to operate upon object of the class. The declaration of a overloaded unary operator function precedes the word operator.
For example, consider class 3D which has data members x, y and z and overloaded increment operators:
class 3D
{
int x, y, z;
public:
3D (int a=0, int b=0, int c=0)
{
x = a;
y = b;
z = c;
}
3D operator ++() //unary operator ++ overloaded
{
x = x + 1;
y = y + 1;
z = z + 1;
return *this; //this pointer which points to the caller object
}
3D operator ++(int) //use of dummy argument for post increment
operator
{
3D t = *this;
x = x + 1;
y = y + 1;
z = z + 1;
return t; //return the original object
}
3D show()
{
cout<<”The elements are:\n”
cout<<”x:”<<this->x<<”, y:<this->y <<”, z:”<<this->z;
}
};
int main()
{
3D pt1(2,4,5), pt2(7,1,3);
cout<<”Point one’s dimensions before increment are:”<< pt1.show();
++pt1; //The overloaded operator function ++() will return object’s this pointer
cout<<”Point one’s dimensions after increment are:”<< pt1.show();
cout<<”Point two’s dimensions before increment are:”<< pt2.show();
pt2++; //The overloaded operator function ++() will return object’s this pointer
cout<<”Point two’s dimensions after increment are:”<< pt2.show();
return 0;
}
The o/p would be:
Point one’s dimensions before increment are:
x:2, y:4, z:5
Point one’s dimensions after increment are:
x:3, y:5, z:6
Point two’s dimensions before increment are:
x:7, y:1, z:3
Point two’s dimensions after increment are:
x:7, y:1, z:3
Please note in case of post increment, the operator function increments the value; but returns the original value since it is post increment.
For example, consider class 3D which has data members x, y and z and overloaded increment operators:
class 3D
{
int x, y, z;
public:
3D (int a=0, int b=0, int c=0)
{
x = a;
y = b;
z = c;
}
3D operator ++() //unary operator ++ overloaded
{
x = x + 1;
y = y + 1;
z = z + 1;
return *this; //this pointer which points to the caller object
}
3D operator ++(int) //use of dummy argument for post increment
operator
{
3D t = *this;
x = x + 1;
y = y + 1;
z = z + 1;
return t; //return the original object
}
3D show()
{
cout<<”The elements are:\n”
cout<<”x:”<<this->x<<”, y:<this->y <<”, z:”<<this->z;
}
};
int main()
{
3D pt1(2,4,5), pt2(7,1,3);
cout<<”Point one’s dimensions before increment are:”<< pt1.show();
++pt1; //The overloaded operator function ++() will return object’s this pointer
cout<<”Point one’s dimensions after increment are:”<< pt1.show();
cout<<”Point two’s dimensions before increment are:”<< pt2.show();
pt2++; //The overloaded operator function ++() will return object’s this pointer
cout<<”Point two’s dimensions after increment are:”<< pt2.show();
return 0;
}
The o/p would be:
Point one’s dimensions before increment are:
x:2, y:4, z:5
Point one’s dimensions after increment are:
x:3, y:5, z:6
Point two’s dimensions before increment are:
x:7, y:1, z:3
Point two’s dimensions after increment are:
x:7, y:1, z:3
Please note in case of post increment, the operator function increments the value; but returns the original value since it is post increment.
22 :: Can you please explain function overloading?
A feature in C++ that enables several functions of the same name can be defined with different types of parameters or different number of parameters. This feature is called function overloading. The appropriate function will be identified by the compiler by examining the number or the types of parameters / arguments in the overloaded function. Function overloading reduces the investment of different function names and used to perform similar functionality by more than one function.
23 :: Can you please explain operator overloading?
A feature in C++ that enables the redefinition of operators. This feature operates on user defined objects. All overloaded operators provides syntactic sugar for function calls that are equivalent. Without adding to / changing the fundamental language changes, operator overloading provides a pleasant façade.
24 :: What is overloading template?
A template function overloads itself as needed. But we can explicitly overload it too. Overloading a function template means having different sets of function templates which differ in their parameter list. Consider following example:
#include <iostream>
template <class X> void func(X a)
{
// Function code;
cout <<”Inside f(X a) \n”;
}
template <class X, class Y> void func(X a, Y b) //overloading function template func()
{
// Function code;
cout <<”Inside f(X a, Y b) \n”;
}
int main()
{
func(10); // calls func(X a)
func(10, 20); // calls func(X a, Y b)
return 0;
}
#include <iostream>
template <class X> void func(X a)
{
// Function code;
cout <<”Inside f(X a) \n”;
}
template <class X, class Y> void func(X a, Y b) //overloading function template func()
{
// Function code;
cout <<”Inside f(X a, Y b) \n”;
}
int main()
{
func(10); // calls func(X a)
func(10, 20); // calls func(X a, Y b)
return 0;
}
25 :: Explain function overloading?
Function overloading is the process of using the same name for two or more functions. The secret to overloading is that each redefinition of the function must use either different types of parameters or a different number of parameters. It is only through these differences that the compiler knows which function to call in any situation.
Consider following program which overloads MyFunction() by using different types of parameters:
#include <iostream>
Using namespace std;
int MyFunction(int i);
double MyFunction(double d);
int main()
{
cout <<MyFunction(10)<<”\n”; //calls MyFunction(int i);
cout <<MyFunction(5.4)<<”\n”; //calls MyFunction(double d);
return 0;
}
int MyFunction(int i)
{
return i*i;
}
double MyFunction(double d)
{
return d*d;
}
Now the following program overloads MyFunction using different no of parameters
#include <iostream>
Using namespace std;
int MyFunction(int i);
int MyFunction(int i, int j);
int main()
{
cout <<MyFunction(10)<<”\n”; //calls MyFunction(int i);
cout <<MyFunction(1, 2)<<”\n”; //calls MyFunction(int i, int j);
return 0;
}
int MyFunction(int i)
{
return i;
}
double MyFunction(int i, int j)
{
return i*j;
}
Please note, the key point about function overloading is that the functions must differ in regard to the types and/or number of parameters. Two functions differing only in their return types can not be overloaded.
Consider following program which overloads MyFunction() by using different types of parameters:
#include <iostream>
Using namespace std;
int MyFunction(int i);
double MyFunction(double d);
int main()
{
cout <<MyFunction(10)<<”\n”; //calls MyFunction(int i);
cout <<MyFunction(5.4)<<”\n”; //calls MyFunction(double d);
return 0;
}
int MyFunction(int i)
{
return i*i;
}
double MyFunction(double d)
{
return d*d;
}
Now the following program overloads MyFunction using different no of parameters
#include <iostream>
Using namespace std;
int MyFunction(int i);
int MyFunction(int i, int j);
int main()
{
cout <<MyFunction(10)<<”\n”; //calls MyFunction(int i);
cout <<MyFunction(1, 2)<<”\n”; //calls MyFunction(int i, int j);
return 0;
}
int MyFunction(int i)
{
return i;
}
double MyFunction(int i, int j)
{
return i*j;
}
Please note, the key point about function overloading is that the functions must differ in regard to the types and/or number of parameters. Two functions differing only in their return types can not be overloaded.
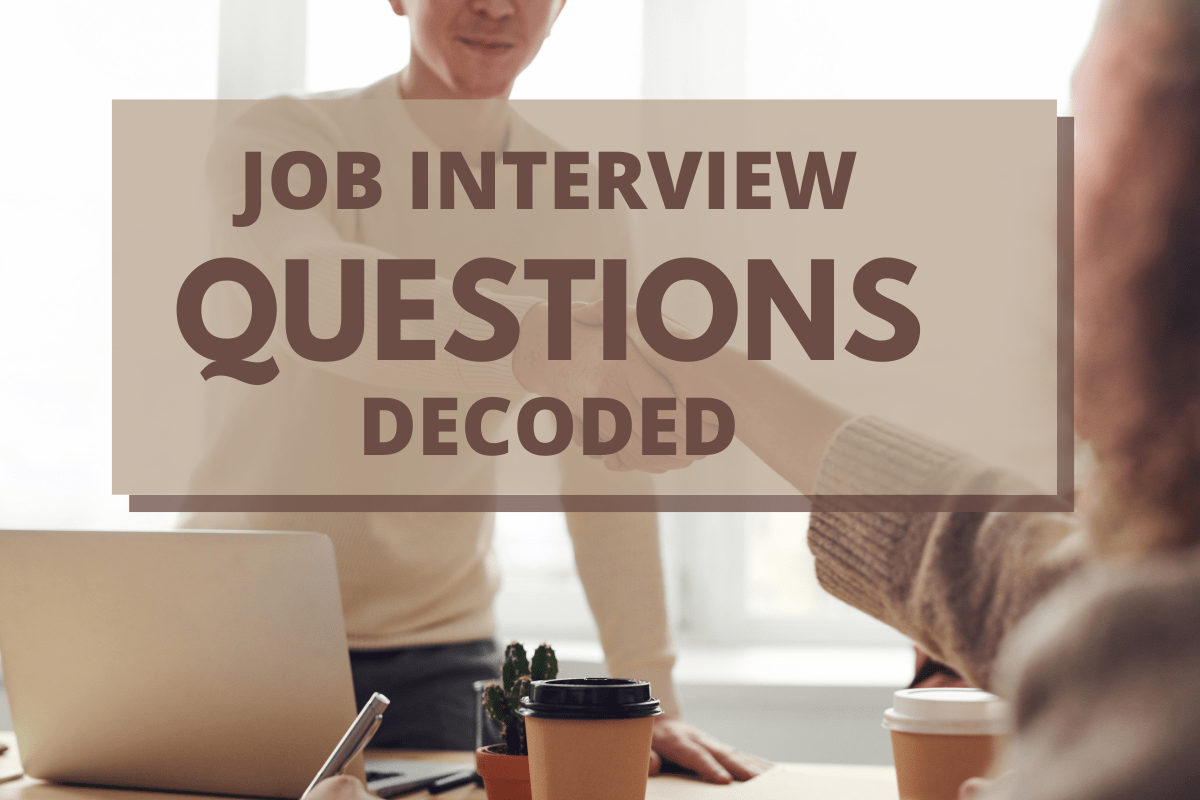