C++ Type Checking Interview Questions And Answers
Refine your C++ Type Checking interview skills with our 27 critical questions. These questions will test your expertise and readiness for any C++ Type Checking interview scenario. Ideal for candidates of all levels, this collection is a must-have for your study plan. Don't miss out on our free PDF download, containing all 27 questions to help you succeed in your C++ Type Checking interview. It's an invaluable tool for reinforcing your knowledge and building confidence.
27 C++ Type Checking Questions and Answers:
C++ Type Checking Job Interview Questions Table of Contents:
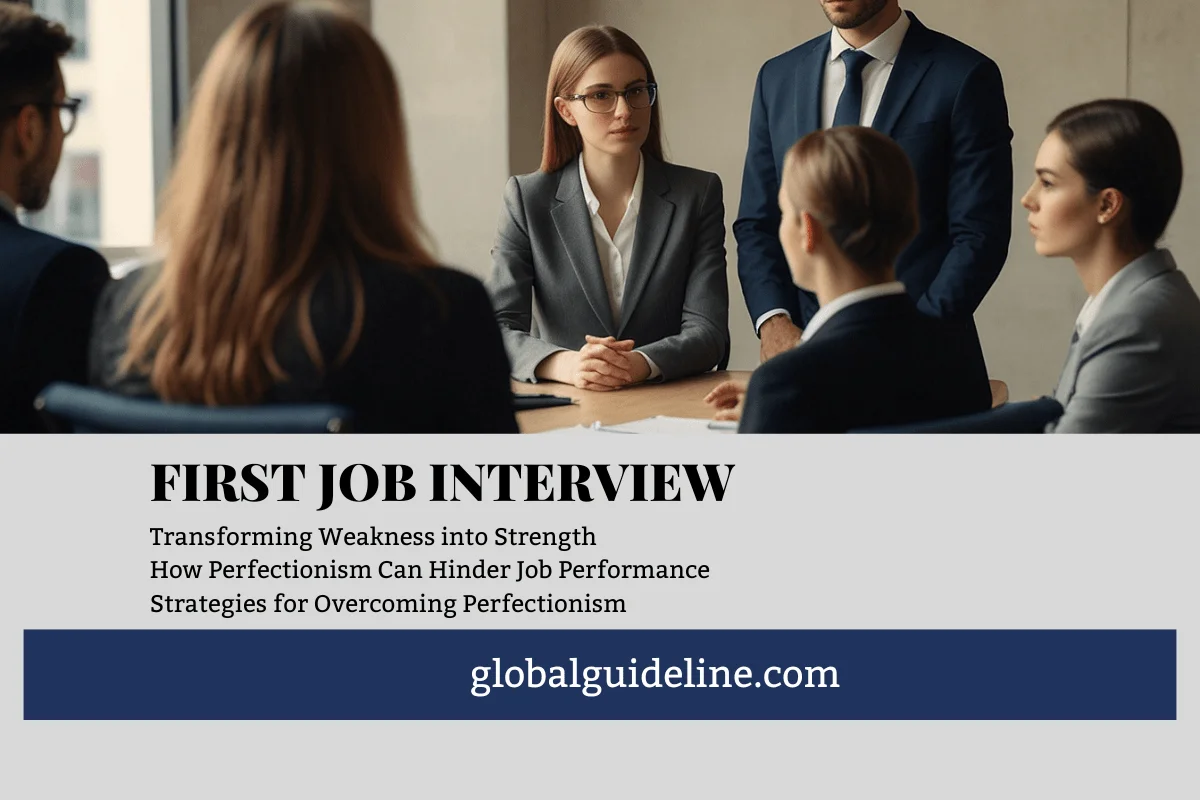
1 :: Can you explain static function?
Static member functions are used to maintain a single copy of a class member function across various objects of the class. Static member functions can be called either by itself, independent of any object, by using class name and :: (scope resolution operator) or in connection with an object.
Restrictions on static member functions are:
1. They can directly refer to other static members of the class.
2. Static member functions do not have this pointer.
3. Static member function can not be virtual.
Though there are several restrictions on static member functions, one good use of them is to initialize private static data members of a class before any object is created.
Consider following example:
#include <iostream>
using namespace std;
class S
{
static int i;
public:
static void init(int x)
{
i = x;
}
void show()
{
cout <<i;
}
};
int S::i;
int main()
{
S::init(100); //initialize static variable i before creating object
S x;
x.show();
return 0;
}
Read MoreRestrictions on static member functions are:
1. They can directly refer to other static members of the class.
2. Static member functions do not have this pointer.
3. Static member function can not be virtual.
Though there are several restrictions on static member functions, one good use of them is to initialize private static data members of a class before any object is created.
Consider following example:
#include <iostream>
using namespace std;
class S
{
static int i;
public:
static void init(int x)
{
i = x;
}
void show()
{
cout <<i;
}
};
int S::i;
int main()
{
S::init(100); //initialize static variable i before creating object
S x;
x.show();
return 0;
}
2 :: Do you know what are function prototypes?
In C++ all functions must be declared before they are used. This is accomplished using function prototype. Prototypes enable complier to provide stronger type checking. When prototype is used, the compiler can find and report any illegal type conversions between the type of arguments used to call a function and the type definition of its parameters. It can also find the difference between the no of arguments used to call a function and the number of parameters in the function. Thus function prototypes help us trap bugs before they occur. In addition, they help verify that your program is working correctly by not allowing functions to be called with mismatched arguments.
A general function prototype looks like following:
return_type func_name(type param_name1, type param_name2, …,type param_nameN);
The type indicates data type. parameter names are optional in prototype.
Following program illustrates the value of function parameters:
void sqr_it(int *i); //prototype of function sqr_it
int main()
{
int num;
num = 10;
sqr_it(num); //type mismatch
return 0;
}
void sqr_it(int *i)
{
*i = *i * *i;
}
Since sqr_it() has pointer to integer as its parameter, the program throws an error when we pass an integer to it.
Read MoreA general function prototype looks like following:
return_type func_name(type param_name1, type param_name2, …,type param_nameN);
The type indicates data type. parameter names are optional in prototype.
Following program illustrates the value of function parameters:
void sqr_it(int *i); //prototype of function sqr_it
int main()
{
int num;
num = 10;
sqr_it(num); //type mismatch
return 0;
}
void sqr_it(int *i)
{
*i = *i * *i;
}
Since sqr_it() has pointer to integer as its parameter, the program throws an error when we pass an integer to it.
3 :: Explain what are associate containers?
Containers are objects that hold other objects. An associative container stores pair of values. It is typically a key-value pair. Given one value (key), we can access the other, called the mapped value. The key needs to be unique. The value associated with that key could be unique or multiple depending upon the type of associative container.
The key-value pair could be of any data type (unlike integer in case of array).
There are various types of associative containers:
Map: It is a traditional associate array, where a single value is associated with each unique pair.
Multimap : This type of associative array allows duplicate elements (value) for a given key.
Read MoreThe key-value pair could be of any data type (unlike integer in case of array).
There are various types of associative containers:
Map: It is a traditional associate array, where a single value is associated with each unique pair.
Multimap : This type of associative array allows duplicate elements (value) for a given key.
4 :: What is static type checking?
Static type checking performs the type checking operation before the execution of the program. To perform this operation, the arguments, expressions, variables must be given a data type.
Read More5 :: What is dynamic type checking?
Dynamic type checking performs the type checking operation at the time of the program execution. To perform this operation, the arguments, expressions, variables must be given a data type.
Read More6 :: Tell me What is when is dynamic checking necessary?
Dynamic checking is necessary in the following scenarios:
► Whenever the definition of a variable is not necessary before its usage.
► When implicit conversion of variables into other types.
► When the program is to be compiled independently as there is no type checking at compile time.
Read More► Whenever the definition of a variable is not necessary before its usage.
► When implicit conversion of variables into other types.
► When the program is to be compiled independently as there is no type checking at compile time.
7 :: How many characters are specified in the ASCII scheme?
a) 64
b) 128
c) 256
d) none of the mentioned?
b) 128
Read More8 :: Select the right option.
Given the variables p, q are of char type and r, s, t are of int type
1. t = (r * s) / (r + s);
2. t = (p * q) / (r + s);
a) 1 is true but 2 is false
b) 1 is false and 2 is true
c) both 1 and 2 are true
d) both 1 and 2 are false
c) both 1 and 2 are true
Read More9 :: Which of the following belongs to the set of character types?
a) char
b) wchar_t
c) only a
d) both a and b
d) both a and b
Read More10 :: What will be the output of this program?
#include <iostream>
using namespace std;
int main()
{
char c = 74;
cout << c;
return 0;
}
a) A
b) N
c) J
d) I
c) J
Read More11 :: How do we represent a wide character of the form wchar_t?
a) L'a'
b) l'a'
c) L[a]
d) la
a) L'a'
Read More12 :: What is the output of this program?
#include <stdio.h>
int main()
{
char a = '12';
printf("%d", a);
return 0;
}
a) Compiler error
b) 12
c) 10
d) Empty
c) 10
Read More13 :: Is the size of character literals different in C and C++?
a) Implementation defined
b) Can't say
c) Yes, they are different
d) No, they are not different
c) Yes, they are different
Read More14 :: Suppose in a hypothetical machine, the size of char is 32 bits. What would sizeof(char) return?
a) 4
b) 1
c) Implementation dependent
d) Machine dependent
b) 1
Read More15 :: What constant defined in <climits> header returns the number of bits in a char?
a) CHAR_SIZE
b) SIZE_CHAR
c) BIT_CHAR
d) CHAR_BIT
d) CHAR_BIT
Read More16 :: What is the size of wchar_t in C++?
a) 2
b) 4
c) 2 or 4
d) based on the number of bits in the system
d) based on the number of bits in the system
Read More17 :: Pick the odd one out:
a) array type
b) character type
c) boolean type
d) integer type
a) array type
Read More18 :: In C++, what is the sign of character data type by default?
a) Signed
b) Unsigned
c) Implementation dependent
d) None of these
c) Implementation dependent
Read More20 :: Which type is best suited to represent the logical values?
a) integer
b) boolean
c) character
d) all of the mentioned
b) boolean
Read More21 :: Which datatype is used to represent the absence of parameters?
a) int
b) short
c) void
d) float
c) void
Read More22 :: Which of the following statements are true?
int f(float)
a) f is a function taking an argument of type int and retruning a floating point number
b) f is a function taking an argument of type float and returning a integer.
c) f is a function of type float
d) none of the mentioned
b) f is a function taking an argument of type float and returning a integer.
Read More23 :: Pick the odd one out.
a) integer, character, boolean, floating
b) enumeration, classes
c) integer, enum, void
d) arrays, pointer, classes
c) integer, enum, void
Read More24 :: When a language has the capability to produce new data type mean, it can be called as
a) overloaded
b) extensible
c) encapsulated
d) reprehensible
b) extensible
Read More25 :: The value 132.54 can represented using which data type?
a) double
b) void
c) int
d) bool
a) double
Read More