C++ Constructors Interview Questions & Answers Download PDF
Prepare comprehensively for your C++ Constructors interview with our extensive list of 51 questions. Our questions cover a wide range of topics in C++ Constructors to ensure you're well-prepared. Whether you're new to the field or have years of experience, these questions are designed to help you succeed. Access the free PDF to get all 51 questions and give yourself the best chance of acing your C++ Constructors interview. This resource is perfect for thorough preparation and confidence building.
51 C++ Constructors Questions and Answers:
C++ Constructors Job Interview Questions Table of Contents:
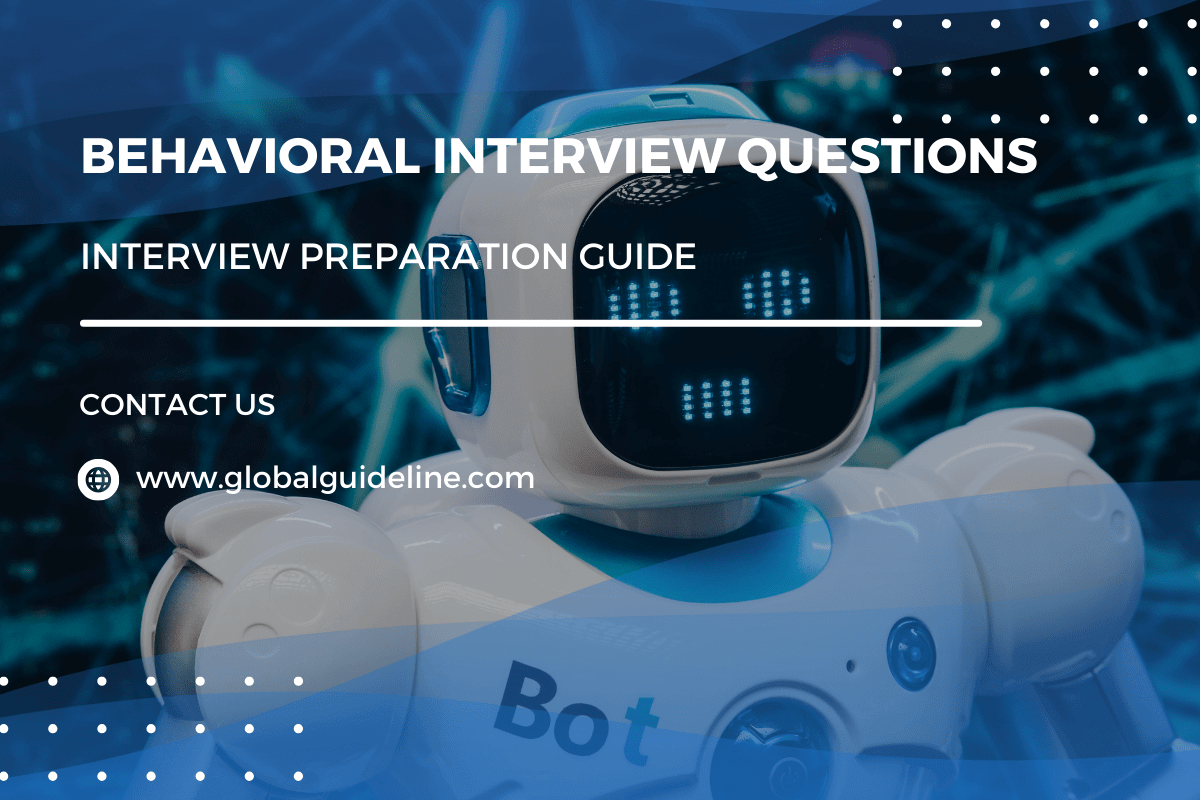
1 :: Which of the following statement is correct?
A. Destructor destroys only integer data members of the object.
B. Destructor destroys only float data members of the object.
C. Destructor destroys only pointer data members of the object.
D. Destructor destroys the complete object.
Option D
Destructor destroys the complete object.
Destructor destroys the complete object.
2 :: How many default constructors per class are possible?
A. Only one
B. Two
C. Three
D. Unlimited
Option A
Only one
Only one
3 :: Which of the following statement is correct about destructors?
A. A destructor has void return type.
B. A destructor has integer return type.
C. A destructor has no return type.
D. A destructors return type is always same as that of main().
Option C
A destructor has no return type.
A destructor has no return type.
4 :: Which of the following cannot be declared as virtual?
A. Constructor
B. Destructor
C. Data Members
D. Both A and C
Option D
Both A and C
Both A and C
5 :: Which of the following are NOT provided by the compiler by default?
A. Zero-argument Constructor
B. Destructor
C. Copy Constructor
D. Copy Destructor
Option D
Copy Destructor
Copy Destructor
6 :: If the copy constructor receives its arguments by value, the copy constructor would
A. call one-argument constructor of the class
B. work without any problem
C. call itself recursively
D. call zero-argument constructor
Option C
call itself recursively
call itself recursively
7 :: Which of the following statement is correct?
A. A destructor has the same name as the class in which it is present.
B. A destructor has a different name than the class in which it is present.
C. A destructor always returns an integer.
D. A destructor can be overloaded.
Option A
A destructor has the same name as the class in which it is present.
A destructor has the same name as the class in which it is present.
8 :: Constructors __________ to allow different approaches of object construction.
A. cannot overloaded
B. can be overloaded
C. can be called
D. can be nested
Option B
can be overloaded
can be overloaded
9 :: A __________ is a constructor that either has no parameters, or if it has parameters, all the parameters have default values.
A. default constructor
B. copy constructor
C. Both A and B
D. None of these
Option A
default constructor
default constructor
11 :: It is a __________ error to pass arguments to a destructor.
A. logical
B. virtual
C. syntax
D. linker
Option C
syntax
syntax
12 :: __________ used to make a copy of one class object from another class object of the same class type.
A. constructor
B. copy constructor
C. destructor
D. default constructor
Option B
copy constructor
copy constructor
15 :: Which of the following never requires any arguments?
A. Member function
B. Friend function
C. Default constructor
D. const function
Option C
Default constructor
Default constructor
16 :: Which of the following gets called when an object goes out of scope?
A. constructor
B. destructor
C. main
D. virtual function
Option B
destructor
destructor
20 :: Which of the following statement is correct?
A. A constructor has the same name as the class in which it is present.
B. A constructor has a different name than the class in which it is present.
C. A constructor always returns an integer.
D. A constructor cannot be overloaded.
Option A
A constructor has the same name as the class in which it is present.
A constructor has the same name as the class in which it is present.
21 :: Copy constructor must receive its arguments by __________ .
A. either pass-by-value or pass-by-reference
B. only pass-by-value
C. only pass-by-reference
D. only pass by address
Option C
only pass-by-reference
only pass-by-reference
22 :: When are the Global objects destroyed?
A. When the control comes out of the block in which they are being used.
B. When the program terminates.
C. When the control comes out of the function in which they are being used.
D. As soon as local objects die.
Option B
When the program terminates.
When the program terminates.
25 :: Which constructor function is designed to copy objects of the same class type?
A. Create constructor
B. Object constructor
C. Dynamic constructor
D. Copy constructor
Option D
Copy constructor
Copy constructor
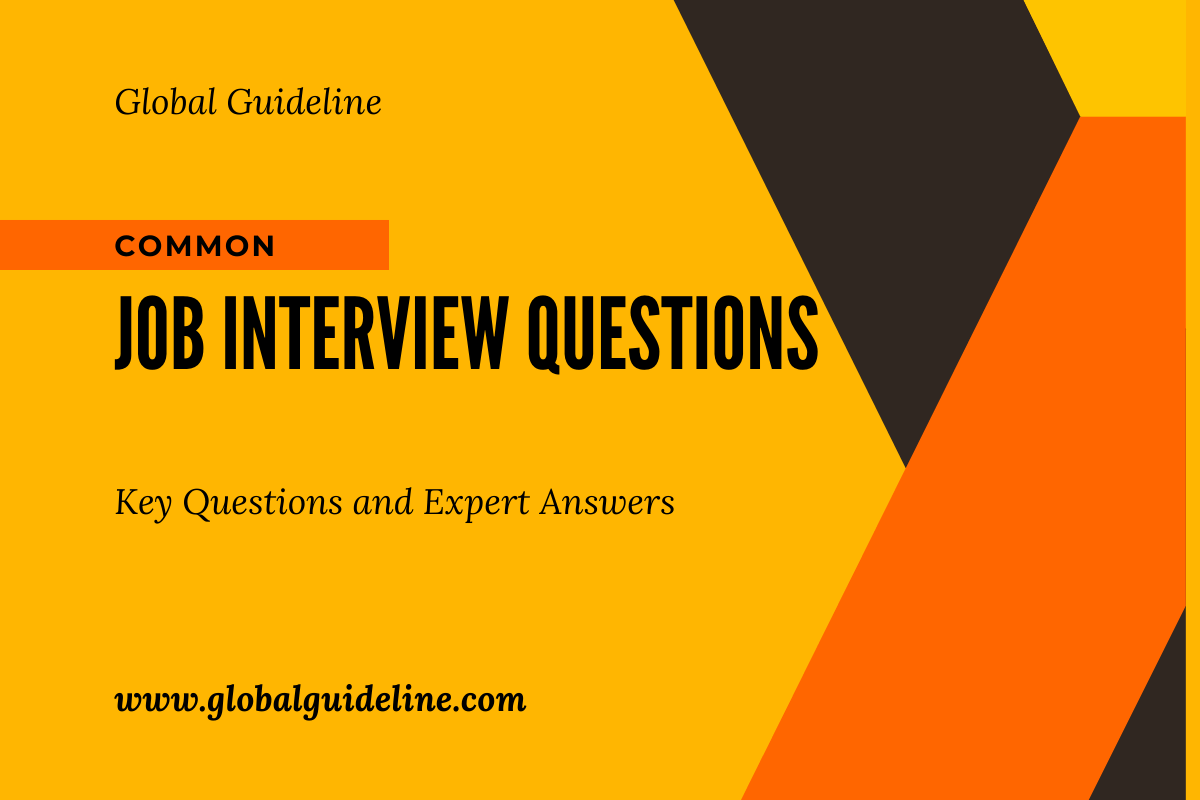