Mostly Asked C++ Friend Interview Preparation Guide Download PDF
C++ Friend frequently Asked Questions in various C++ Friend job Interviews by interviewer. The set of questions here ensures that you offer a perfect answer posed to you. So get preparation for your new job hunting
20 C++ Friend Questions and Answers:
Table of Contents:
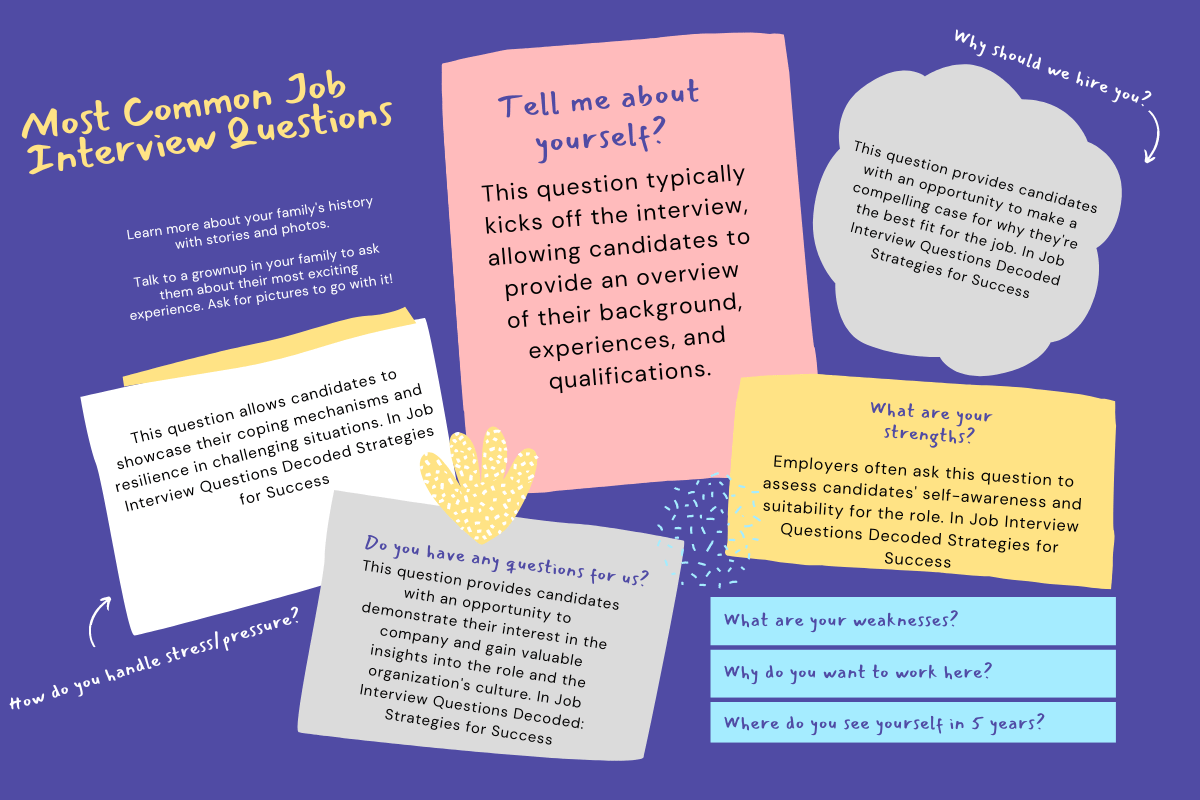
1 :: List the advantages of using friend classes?
There are situations when private data members need to be accessed and used by 2 classes simultaneously. In these kind of situations we can introduce friend functions which have an access to the private data members of both the classes. Friend functions need to be declared as 'friend' in both the classes. They need not be members of either of these classes.
2 :: What are the C++ friend classes? Explain its uses with an example?
A friend class and all its member functions have access to all the private members defined within other class.
#include <iostream>
using namespace std;
class Numbers
{
int a;
int b;
public:
Numbers(int i, int j)
{
a = i;
b = j;
}
friend class Average;
};
class Average
{
public:
int average(Numbers x);
};
int Average:: average(Numbers x)
{
return ((x.a + x.b)/2);
}
int main()
{
Numbers ob(23, 67);
Average avg;
cout<<"The average of the numbers is: "<<avg.average(ob);
return 0;
}
Note the friend class member function average is passed the object of Numbers class as parameter.
#include <iostream>
using namespace std;
class Numbers
{
int a;
int b;
public:
Numbers(int i, int j)
{
a = i;
b = j;
}
friend class Average;
};
class Average
{
public:
int average(Numbers x);
};
int Average:: average(Numbers x)
{
return ((x.a + x.b)/2);
}
int main()
{
Numbers ob(23, 67);
Average avg;
cout<<"The average of the numbers is: "<<avg.average(ob);
return 0;
}
Note the friend class member function average is passed the object of Numbers class as parameter.
3 :: List the characteristics of friend functions?
Friend functions are not a part of the class and are external. This function is a "Friend" of a class. This is to say, it has special privileges to access to the class's private and protected members.
4 :: What is the friend class in C++?
When a class declares another class as its friend, it is giving complete access to all its data and methods including private and protected data and methods to the friend class member methods. Friendship is one way only, which means if A declares B as its friend it does NOT mean that A can access private data of B. It only means that B can access all data of A.
5 :: Pick out the correct statement.
a) A friend function may be a member of another class.
b) A friend function may not be a member of another class.
c) A friend function may or may not be a member of another class.
d) None of the mentioned
c) A friend function may or may not be a member of another class.
6 :: Where does keyword 'friend' should be placed?
a) function declaration
b) function definition
c) main function
d) None of the mentioned
a) function declaration
Explanation:
The keyword friend is placed only in the function declaration of the friend function and not in the function definition because it is used toaccess the member of a class.
Explanation:
The keyword friend is placed only in the function declaration of the friend function and not in the function definition because it is used toaccess the member of a class.
8 :: What is output of this program?
#include <iostream>
using namespace std;
class sample
{
private:
int a, b;
public:
void test()
{
a = 100;
b = 200;
}
friend int compute(sample e1);
};
int compute(sample e1)
{
return int(e1.a + e1.b) - 5;
}
int main()
{
sample e;
e.test();
cout << compute(e);
return 0;
}
a) 100
b) 200
c) 300
d) 295
d) 295
Explanation:
In this program, we are finding a value from the given function by using the friend for compute function.
Output:
$ g++ friend4.cpp
$ a.out
295
Explanation:
In this program, we are finding a value from the given function by using the friend for compute function.
Output:
$ g++ friend4.cpp
$ a.out
295
9 :: What is the output of this program?
#include <iostream>
using namespace std;
class sample;
class sample1
{
int width, height;
public:
int area ()
{
return (width * height);}
void convert (sample a);
};
class sample
{
private:
int side;
public:
void set_side (int a)
{
side = a;
}
friend class sample1;
};
void sample1::convert (sample a)
{
width = a.side;
height = a.side;
}
int main ()
{
sample sqr;
sample1 rect;
sqr.set_side(6);
rect.convert(sqr);
cout << rect.area();
return 0;
}
a) 24
b) 35
c) 16
d) 36
d) 36
Explanation:
In this program, we are using the friend for the class and calculating the area of the square.
Output:
$ g++ friend2.cpp
$ a.out
36
Explanation:
In this program, we are using the friend for the class and calculating the area of the square.
Output:
$ g++ friend2.cpp
$ a.out
36
10 :: What is the output of this program?
#include <iostream>
using namespace std;
class sample
{
int width, height;
public:
void set_values (int, int);
int area () {return (width * height);}
friend sample duplicate (sample);
};
void sample::set_values (int a, int b)
{
width = a;
height = b;
}
sample duplicate (sample rectparam)
{
sample rectres;
rectres.width = rectparam.width * 2;
rectres.height = rectparam.height * 2;
return (rectres);
}
int main ()
{
sample rect, rectb;
rect.set_values (2, 3);
rectb = duplicate (rect);
cout << rectb.area();
return 0;
}
a) 20
b) 16
c) 24
c) 24
Explanation:
In this program, we are using the friend function for duplicate function and calculating the area of the rectangle.
Output:
$ g++ friend1.cpp
$ a.out
24
Explanation:
In this program, we are using the friend function for duplicate function and calculating the area of the rectangle.
Output:
$ g++ friend1.cpp
$ a.out
24
11 :: What is the output of this program?
#include <iostream>
using namespace std;
class Box
{
double width;
public:
friend void printWidth( Box box );
void setWidth( double wid );
};
void Box::setWidth( double wid )
{
width = wid;
}
void printWidth( Box box )
{
box.width = box.width * 2;
cout << "Width of box : " << box.width << endl;
}
int main( )
{
Box box;
box.setWidth(10.0);
printWidth( box );
return 0;
}
a) 40
b) 5
c) 10
d) 20
d) 20
Explanation:
We are using the friend function for printwidth and multiplied the width value by 2, So we got the output as 20
Output:
$ g++ friend.cpp
$ a.out
20
Explanation:
We are using the friend function for printwidth and multiplied the width value by 2, So we got the output as 20
Output:
$ g++ friend.cpp
$ a.out
20
13 :: What is the syntax of friend function?
a) friend class1 Class2;
b) friend class;
c) friend class
d) None of the mentioned
a) friend class1 Class2;
Explanation:
In option a, the class2 is the friend of class1 and it can access all the private and protected members of class1.
Explanation:
In option a, the class2 is the friend of class1 and it can access all the private and protected members of class1.
14 :: Which rule will not affect the friend function?
a) private and protected members of a class cannot be accessed from outside
b) private and protected member can be accessed anywhere
c) both a & b
d) None of the mentioned
a) private and protected members of a class cannot be accessed from outside
Explanation:
Friend is used to access private and protected members of a class from outside the same class.
Explanation:
Friend is used to access private and protected members of a class from outside the same class.
15 :: What are the advantages of using friend classes?
There are situations when private data members need to be accessed and used by 2 classes simultaneously. In these kind of situations we can introduce friend functions which have an access to the private data members of both the classes. Friend functions need to be declared as ‘friend’ in both the classes. They need not be members of either of these classes.
16 :: What is a friend function?
To allow a non-member function the access to private members of a class, it needs to be friend of that class. Friend functions can access private and protected data of the class. To make a non-member function friend of a class, its declaration needs to be made inside the class and it has to be preceded by the keyword friend. We can also have a member function of a class to be friend of certain other class. Even if a function is not a member of any class, it can still be friend of multiple classes.
If we write equivalent friend function for a member function, then friend function has one extra parameter because being a non-member of the class, it does not have the caller object. Therefore, it does not have this pointer.
Friend functions are very useful for overloading certain types of operators. They also make creation of some type of I/O functions easier.
Consider following example of class 3D having data members x, y and z. Overloaded binary operator * for scalar multiplication. It should work in both cases:
ob1 = ob2 * 3;
ob1 = 3 * ob2;
Note that first can be achieved through member or friend function. But for the second case we need to write friend function since there is no caller object.
class 3D
{
int x, y, z;
public:
3D (int a=0, int b=0, int c=0)
{
x = a;
y = b;
z = c;
}
3D show()
{
cout<<”The elements are:\n”
cout<<”x:”<<this->x<<”, y:<<this->y <<”, z:”<<this->z;
}
friend 3D operator * (3D, int);
friend 3D operator * (int, 3D);
};
3D operator * (3D ob, int i) //friend function’s definition is written outside class
{
3D tob;
tob.x = ob.x * i;
tob.y = ob.y * i;
tob.z = ob.z * i;
return tob;
}
3D operator * (int i, 3D ob) //friend function’s definition is written outside class
{
3D tob;
tob.x = ob.x * i;
tob.y = ob.y * i;
tob.z = ob.z * i;
return tob;
}
int main()
{
3D pt1(2,-4,5), pt2, pt3;
pt 2 = pt1 * 3;
pt3 = -2 * pt1
cout << “\n Point one’s dimensions are: \n“<<pt1.show();
cout << “\n Point two’s dimensions are: \n“<<pt2.show();
cout << “\n Point three’s dimensions are: \n“<<pt3.show();
return 0;
}
The o/p would be:
Point one’s dimensions are:
x:2, y:-4, z:5
Point two’s dimensions are:
x:6, y:-12, z:15
Point three’s dimensions are:
x:-4, y:8, z:-10
Thus, friend functions are useful when we have to overload operators which have no caller object.
If we write equivalent friend function for a member function, then friend function has one extra parameter because being a non-member of the class, it does not have the caller object. Therefore, it does not have this pointer.
Friend functions are very useful for overloading certain types of operators. They also make creation of some type of I/O functions easier.
Consider following example of class 3D having data members x, y and z. Overloaded binary operator * for scalar multiplication. It should work in both cases:
ob1 = ob2 * 3;
ob1 = 3 * ob2;
Note that first can be achieved through member or friend function. But for the second case we need to write friend function since there is no caller object.
class 3D
{
int x, y, z;
public:
3D (int a=0, int b=0, int c=0)
{
x = a;
y = b;
z = c;
}
3D show()
{
cout<<”The elements are:\n”
cout<<”x:”<<this->x<<”, y:<<this->y <<”, z:”<<this->z;
}
friend 3D operator * (3D, int);
friend 3D operator * (int, 3D);
};
3D operator * (3D ob, int i) //friend function’s definition is written outside class
{
3D tob;
tob.x = ob.x * i;
tob.y = ob.y * i;
tob.z = ob.z * i;
return tob;
}
3D operator * (int i, 3D ob) //friend function’s definition is written outside class
{
3D tob;
tob.x = ob.x * i;
tob.y = ob.y * i;
tob.z = ob.z * i;
return tob;
}
int main()
{
3D pt1(2,-4,5), pt2, pt3;
pt 2 = pt1 * 3;
pt3 = -2 * pt1
cout << “\n Point one’s dimensions are: \n“<<pt1.show();
cout << “\n Point two’s dimensions are: \n“<<pt2.show();
cout << “\n Point three’s dimensions are: \n“<<pt3.show();
return 0;
}
The o/p would be:
Point one’s dimensions are:
x:2, y:-4, z:5
Point two’s dimensions are:
x:6, y:-12, z:15
Point three’s dimensions are:
x:-4, y:8, z:-10
Thus, friend functions are useful when we have to overload operators which have no caller object.
17 :: Explain the characteristics of friend functions?
A friend function is not in the scope of the class n which it has been declared as friend.
It cannot be called using the object of that class.
It can be invoked like a normal function without any object.
Unlike member functions, it cannot use the member names directly.
It can be declared in public or private part without affecting its meaning.
Usually, it has objects as arguments.
It cannot be called using the object of that class.
It can be invoked like a normal function without any object.
Unlike member functions, it cannot use the member names directly.
It can be declared in public or private part without affecting its meaning.
Usually, it has objects as arguments.
18 :: Explain advantages of using friend classes?
A friend function has the following advantages:
- Provides additional functionality which is kept outside the class.
- Provides functions that need data which is not normally used by the class.
- Allows sharing private class information by a non member function.
- Provides additional functionality which is kept outside the class.
- Provides functions that need data which is not normally used by the class.
- Allows sharing private class information by a non member function.
19 :: What are friend classes?
The friend function is a ‘non member function’ of a class. It can access non public members of the class. A friend function is external to the class definition.
20 :: Explain friend function?
When the application is needed to access a private member of another class, the only way is to utilize the friend functions. The following are the guidelines to handle friend functions.
Declare the function with the keyword ‘friend’ that is followed by return type and followed by the function name.
Specify the class whose private members are to be accessed in the friend function within parenthesis of the friend function.
Write the code of the friend function in the class.
The following code snippet illustrates the use of friend function:
friend void display(car); //Friend of the class 'car'
void display(car myCar)
{
cout<<"\nThe color of my car is : "<<myCar.color;
cout<<"\nThe speed of my car is : "<<myCar.speed;
}
Declare the function with the keyword ‘friend’ that is followed by return type and followed by the function name.
Specify the class whose private members are to be accessed in the friend function within parenthesis of the friend function.
Write the code of the friend function in the class.
The following code snippet illustrates the use of friend function:
friend void display(car); //Friend of the class 'car'
void display(car myCar)
{
cout<<"\nThe color of my car is : "<<myCar.color;
cout<<"\nThe speed of my car is : "<<myCar.speed;
}
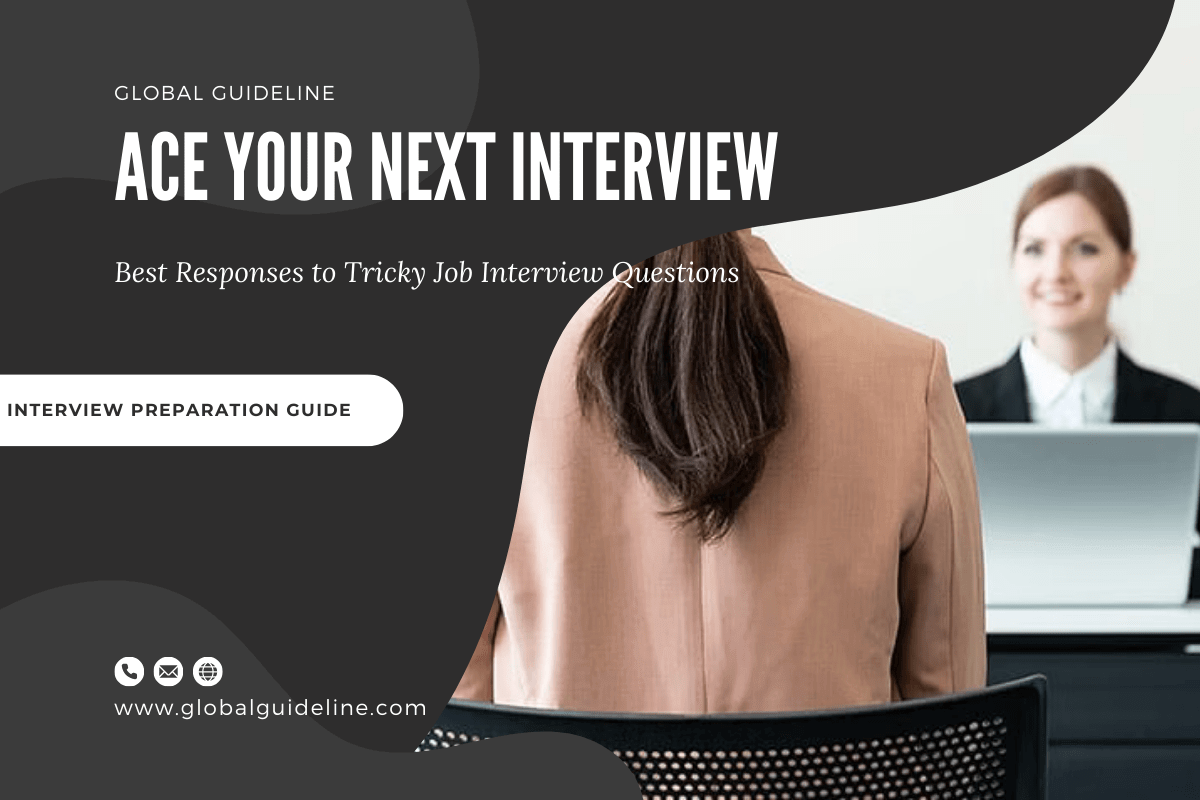