Professional Java GUI Framework Interview Preparation Guide Download PDF
Java GUI Framework frequently Asked Questions by expert members with experience in Java GUI Framework . These interview questions and answers on Java GUI Framework will help you strengthen your technical skills, prepare for the interviews and quickly revise the concepts. So get preparation for the Java GUI Framework job interview
28 Java GUI Framework Questions and Answers:
Table of Contents:
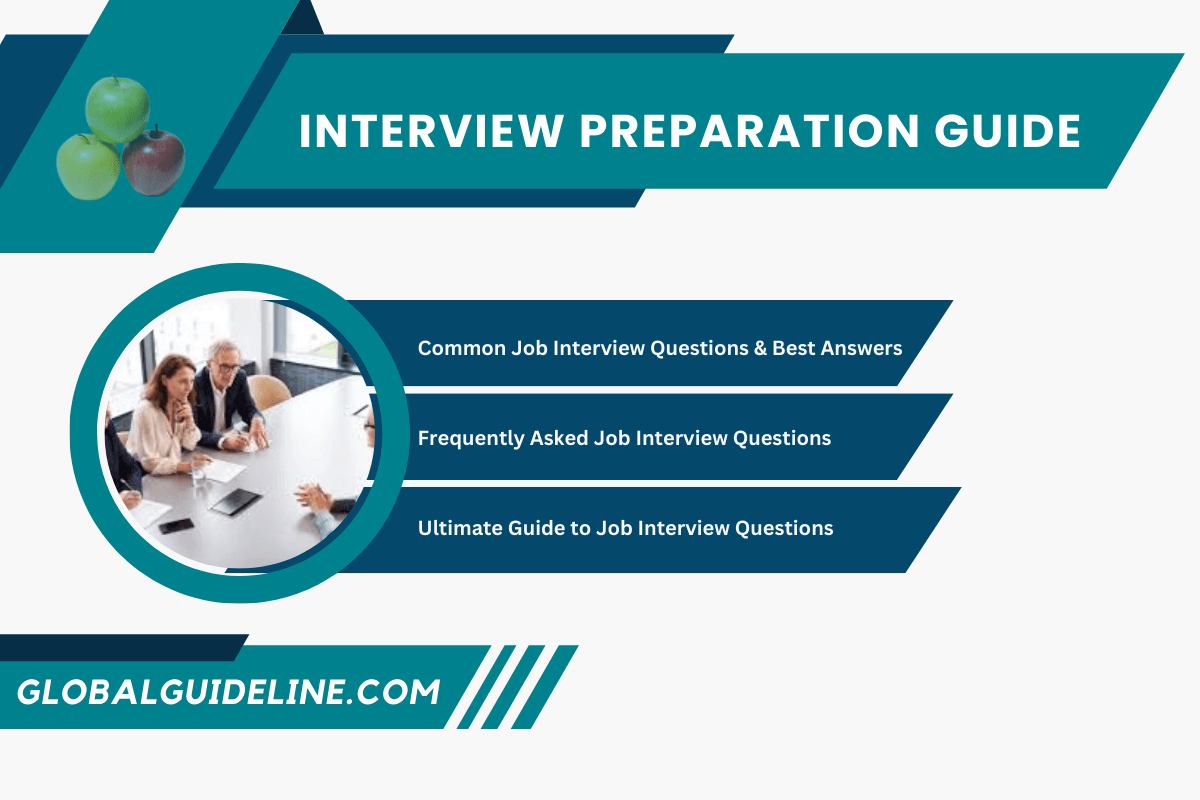
1 :: Explain Prediction of output of code?
This is another category of Swing interview questions asked in IB whether they will give you code and asked what would be the output , how will the GUI look like. This type of question is based upon how well you understand and visualize the code. Whether you are familiar with default layout manager of various component classes or not e.g. default layout of JFrame is BorderLayout. So do some practice of these kinds of java Swing interview questions as well?
2 :: How to handle opening of database, file or network connection on a click of button?
This one is one of the easy java swing interview question. Interviewer is interested on whether you know the basic principle of Java GUI development or not. answer is you should not do this operation in EDT thread instead spawn a new thread from actionListener or button and disable the button until operation gets completed to avoid resubmitting request. Only condition is that your GUI should always be responsive no matter what happens on network connection or database connection because these operations usually take time.
3 :: Write code to print following layout (mainly focused on GridBag layout)?
After JTable second favorite topic of swing interviewer is GridBagLayout. GridBagLayout in swing is most powerful but at same time most complex layout and a clear cut experience and expertise around GridBagLayout is desired for developing Swing GUI for trading systems. No matter whether you are developing GUI for equities trading, futures or options trading or forex trading you always required GridBagLayout. Swing interview question on GridBagLayout will be mostly on writing code for a particular layout just like an example shown here. In which six buttons A, B, C, D, E and F are organized in certain fashion.
4 :: Write code for JTable with custom cell editor and custom cell renderer?
Now comes harder part of swing interviews, questions asked in this part of Swing interview is mostly about writing code and checking developer’s capability of API familiarity, key concept of swing code etc.
JTable is one of favorite topic of all Swing interviews and most popular questions on swing interviews are from JTable why? Because here interviewer will directly asked you to write code another reason is JTable heavily used in all Electronic trading GUI. GUI used for online stock trading uses JTable to show data in tabular format so an in depth knowledge of JTable is required to work on online trading GUI developed in Swing. While this question is just an example questions around JTable are mostly centered around updating table, how do you handle large volume of data in table, using customize cell renderer and editor, sorting table data based on any column etc. so just make sure you have done quite a few handsone exercise on JTable before appearing for any java swing interview in IB.
JTable is one of favorite topic of all Swing interviews and most popular questions on swing interviews are from JTable why? Because here interviewer will directly asked you to write code another reason is JTable heavily used in all Electronic trading GUI. GUI used for online stock trading uses JTable to show data in tabular format so an in depth knowledge of JTable is required to work on online trading GUI developed in Swing. While this question is just an example questions around JTable are mostly centered around updating table, how do you handle large volume of data in table, using customize cell renderer and editor, sorting table data based on any column etc. so just make sure you have done quite a few handsone exercise on JTable before appearing for any java swing interview in IB.
5 :: What is difference between invokeAndWait and invokeLater?
This swing interview question is asked differently at different point. some time interviewer ask how do you update swing component from a thread other than EDT, for such kind of scenario we use SwingUtilities.invokeAndWait(Runnable r) and SwingUtilities.invokeLetter(Runnable r) though there are quite a few differences between these two, major one is invokeAndWait is a blocking call and wait until GUI updates while invokeLater is a non blocking asynchronous call. In my opinion these question has its own value and every swing developer should be familiar with these questions or concept not just for interview point of view but on application perspective. you can read more on my post How InvokeAndWait and InvokeLater works in Swing
6 :: Why Swing components are called lightweight component?
Another popular java swing interview question, I guess the oldest that is generally comes as followup of earlier question based on your answer provided. AWT components are associated with native screen resource and called heavyweight component while Swing components is uses the screen resource of an ancestor instead of having there own and that's why called lightweight or lighter component.
7 :: What are differences between Swing and AWT?
One of the classic java swing interview questions and mostly asked on phone interviews. There is couple of differences between swing and AWT:
1) AWT component are considered to be heavyweight while Swing component are lightweights
2) Swing has plug-gable look and feel.
3) AWT is platform depended same GUI will look different on different platform while Swing is developed in Java and is platform dependent.
1) AWT component are considered to be heavyweight while Swing component are lightweights
2) Swing has plug-gable look and feel.
3) AWT is platform depended same GUI will look different on different platform while Swing is developed in Java and is platform dependent.
8 :: Does Swing is thread safe? What do you mean by swing is not thread-safe?
This swing interview questions is getting very popular now days. Though it’s pretty basic many developer doesn't understand thread-safety issue in Swing. Since Swing components are not thread-safe it means you can not update these components in any thread other than Event-Driven-Thread. If you do so you will get unexpected behavior. Some time interviewer will also ask what are thread-safe methods in swing which can be safely called from any thread only few like repaint() and revalidate().
9 :: What is Event-Driven-Thread (EDT) in Swing?
Event-Driven-Thread or EDT is a special thread in Swing and AWT. Event-Driven Thread is used to draw graphics and listen for events in Swing. You will get a bonus point if you able to highlight that time consuming operations like connecting to database, opening a file or connecting to network should not be done on EDT thread because it could lead to freezing GUI because of blocking and time consuming nature of these operations instead they should be done on separate thread and EDT can just be used to spawn those thread on a button click or mouse click.
10 :: What is an SSCCE?
Short/small, self contained, compilable, example (source code).
It would be best if you provide such short (see the group's charter) example source code in our first request for help. When asked for one, please don't complain that your source code is to large, to tricky, to secret for being cut down to a reasonable size and posted. You have the problem, and you asked in a public forum, so it is in your interest to provide the requested information.
It would be best if you provide such short (see the group's charter) example source code in our first request for help. When asked for one, please don't complain that your source code is to large, to tricky, to secret for being cut down to a reasonable size and posted. You have the problem, and you asked in a public forum, so it is in your interest to provide the requested information.
11 :: How to do this JavaScript thing on my web site?
Java is not JavaScript. Try news:comp.lang.javascript or another suitable JavaScript newsgroup.
12 :: How to draw a tree?
This seems to be a common homework question, so please have a look at the "Which topics are not welcome in the newsgroup?" to understand why this answer is intentionally vague.
If the fixed layout of JTree suits your needs, you could start reading the JTree API documentation.
Or you could use a simple (recursive) algorithm. E.g
x(node) = K * level(node), and
y(node) = M * inorder_rank(node).
gives very ugly trees, but trees. If this doesn't get you started, ask your professor or tutor for more hints. Consult your text book about (inorder) tree traversal, and consult
http://home.earthlink.net/~patricia_shanahan/beginner.html
If the fixed layout of JTree suits your needs, you could start reading the JTree API documentation.
Or you could use a simple (recursive) algorithm. E.g
x(node) = K * level(node), and
y(node) = M * inorder_rank(node).
gives very ugly trees, but trees. If this doesn't get you started, ask your professor or tutor for more hints. Consult your text book about (inorder) tree traversal, and consult
http://home.earthlink.net/~patricia_shanahan/beginner.html
13 :: How to draw lines between JLabels on a JPanel?
You are apparently trying to draw a graph by using normal widgets to draw the nodes of your graph. This is not a good idea. Consider using the Java 2D API (nowadays part of J2SE) to draw the complete graph. Have a look at java.awt.geom for predefined shapes. Also check out Sun's 2D Programmer's Guide
14 :: How to to write a diagram editor. How to start?
Consider using a framework like
http://www.jhotdraw.org/
for a start. You also might want to familiarize yourself with many of the design patterns in
Gamma, E.; Helm, R.; Johnson, R.; Vlissides, J.: Design
Patterns: Elements of reusable object-oriented Software.
Addison-Wesley professional computing series. Brian W.
Kernighan, Consulting Editor. Reading, MA: Addison-Wesley,
1994.
And (if you manage to find documentation), the early work on UniDraw and IDraw by Vlissides is also interesting (in C++).
http://www.jhotdraw.org/
for a start. You also might want to familiarize yourself with many of the design patterns in
Gamma, E.; Helm, R.; Johnson, R.; Vlissides, J.: Design
Patterns: Elements of reusable object-oriented Software.
Addison-Wesley professional computing series. Brian W.
Kernighan, Consulting Editor. Reading, MA: Addison-Wesley,
1994.
And (if you manage to find documentation), the early work on UniDraw and IDraw by Vlissides is also interesting (in C++).
15 :: How to generate some charts / plots in Java?
If you want to do the drawing in Java, consider using a chart drawing library. E.g.
http://www.jfree.org/jfreechart/index.html
gets recommended often. The web site also has a list of other chart libraries.
If you just have to plot some (scientific) data, and if you can live with an external C program, consider using gnuplot
http://www.gnuplot.info/
Use System.exec() to pipe the plot commands and data into gnuplot, or just write the data to a file and use gnuplot separately.
http://www.jfree.org/jfreechart/index.html
gets recommended often. The web site also has a list of other chart libraries.
If you just have to plot some (scientific) data, and if you can live with an external C program, consider using gnuplot
http://www.gnuplot.info/
Use System.exec() to pipe the plot commands and data into gnuplot, or just write the data to a file and use gnuplot separately.
16 :: What is the equivalent of AWTs Canvas in Swing?
JPanel, if you want to have a "complete" component with a UI delegate which handles opaque settings (if paintComponent() is correctly overridden).
JComponent if you intend to always draw every pixel in the area of the component (and break the opaque attribute API contract). If you need to have your own special key and mouse processing, you might also want to start with JComponent and create your own UI delegate.
You could even start higher up in the inheritance chain. java.awt.Component is lightweight since Java 1.1. However, you will not get Swing additions like double-buffering.
If this is all Greek to you, use JPanel. And remember, if you use JComponent or JPanel, override paintComponent(), not paint().
JComponent if you intend to always draw every pixel in the area of the component (and break the opaque attribute API contract). If you need to have your own special key and mouse processing, you might also want to start with JComponent and create your own UI delegate.
You could even start higher up in the inheritance chain. java.awt.Component is lightweight since Java 1.1. However, you will not get Swing additions like double-buffering.
If this is all Greek to you, use JPanel. And remember, if you use JComponent or JPanel, override paintComponent(), not paint().
17 :: How to get the screen size? How Do I center a window on the screen?
Manually, pre 1.4:
Use java.awt.Toolkit.getDefaultToolkit().getScreenSize() to get the screen size, and do the math:
import java.awt.*;
Dimension winSize = win.getSize();
Dimension screenSize =
Toolkit.getDefaultToolkit().getScreenSize();
win.setLocation(
screenSize.width / 2 - winSize.width / 2,
screenSize.height / 2 - winSize.height / 2
);
Since 1.4:
Window.setLocationRelativeTo(null);
Use java.awt.Toolkit.getDefaultToolkit().getScreenSize() to get the screen size, and do the math:
import java.awt.*;
Dimension winSize = win.getSize();
Dimension screenSize =
Toolkit.getDefaultToolkit().getScreenSize();
win.setLocation(
screenSize.width / 2 - winSize.width / 2,
screenSize.height / 2 - winSize.height / 2
);
Since 1.4:
Window.setLocationRelativeTo(null);
18 :: How to replace the icon in the title bar (window decoration) of a [J]Dialog?
There is only a partial solution to this problem, and it is not recommended.
A dialog gets its icon from its parent frame. You can create a dummy frame, set the icon of that dummy frame, and use it in the constructor of the dialog as the dialog's owner:
JFrame dummy = new JFrame();
Image icon = ...
dummyFrame.setIconImage(icon);
JDialog dialog = new JDialog(dummy);
However, this is dangerous. Certain GUI behavior depends on a correct [J]Frame (parent window) <-> [J]Dialog (child window) relation. Introducing a dummy parent breaks this relation. Things which can go wrong include (de)iconising of all windows of an application, and ensuring a modal dialog is always placed on-top of the main window.
A dialog gets its icon from its parent frame. You can create a dummy frame, set the icon of that dummy frame, and use it in the constructor of the dialog as the dialog's owner:
JFrame dummy = new JFrame();
Image icon = ...
dummyFrame.setIconImage(icon);
JDialog dialog = new JDialog(dummy);
However, this is dangerous. Certain GUI behavior depends on a correct [J]Frame (parent window) <-> [J]Dialog (child window) relation. Introducing a dummy parent breaks this relation. Things which can go wrong include (de)iconising of all windows of an application, and ensuring a modal dialog is always placed on-top of the main window.
19 :: How to replace/remove the icon in the title bar (window decoration) of a [J]Frame?
Use setIconImage().
To revert to the platform's default icon use:
frame.setIconImage(null);
On some platforms this might remove the icon. Alternatively you can try a transparent Image if you don't want to have an icon.
To revert to the platform's default icon use:
frame.setIconImage(null);
On some platforms this might remove the icon. Alternatively you can try a transparent Image if you don't want to have an icon.
20 :: How to (de)iconify a window?
Before Java 1.2 you had to revert to native calls.
Since Java 1.2 you can use [J]Frame.setState().
Since Java 1.4 you can use [J]Frame.setExtendedState(), too. setExtendedState() provides more features than setState().
Since Java 1.2 you can use [J]Frame.setState().
Since Java 1.4 you can use [J]Frame.setExtendedState(), too. setExtendedState() provides more features than setState().
21 :: How to make sure a window is always on top of all other windows using AWT or Swing?
Before Java 1.5 you couldn't:
AWT and Swing didn't provide this feature. All you could do was to use a (modal) [J]Dialog, and make sure the [J]Dialog is provided with the correct parent/owner in the constructor.
Since Java 1.5:
Window.setAlwaysOnTop(), which is inherited by the other top-level containers like JFrame.
AWT and Swing didn't provide this feature. All you could do was to use a (modal) [J]Dialog, and make sure the [J]Dialog is provided with the correct parent/owner in the constructor.
Since Java 1.5:
Window.setAlwaysOnTop(), which is inherited by the other top-level containers like JFrame.
22 :: What is Qt Jambi?
A java wrapper to the native qt library which is written in c/c++. Very powerful, widely used and accepted. Has a lot of GUI components and a easy to use API.
23 :: What is Apache Pivot?
It renders UI using Java2D, thus minimizing the impact of (IMO, bloated) legacies of Swing and AWT.
It's main focus seems to be on RIA (Rich internet applications), but it seems it can also be applied to desktop applications. And as a personal comment, Looks very interesting! I Especially like that it's an apache project.
It's main focus seems to be on RIA (Rich internet applications), but it seems it can also be applied to desktop applications. And as a personal comment, Looks very interesting! I Especially like that it's an apache project.
24 :: What is JavaFX?
The latest flagship of Java/Oracle. promising to be the facto standard in developing rich desktop or web applications.
25 :: What is SwingX?
Based on Swing and it's mission is to create rich components for swing. Still under development. (not very active though.) Have a very nice set of components, like for example TreeTable. But the TreeTable does not support filtering and sorting as far as i know. It does however support searching with highlighting.
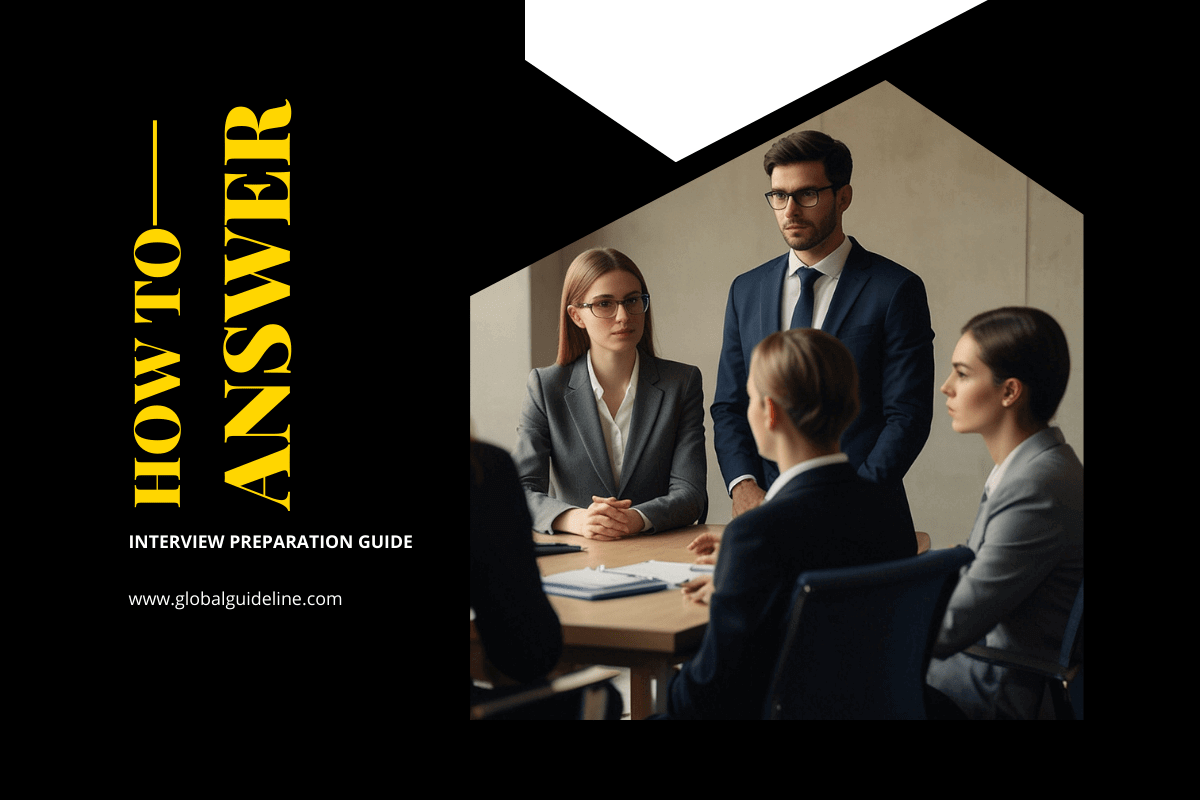