Full Stack Developer (Java) Interview Questions And Answers
Strengthen your Full Stack Developer (Java) interview skills with our collection of 43 important questions. These questions will test your expertise and readiness for any Full Stack Developer (Java) interview scenario. Ideal for candidates of all levels, this collection is a must-have for your study plan. Get the free PDF download to access all 43 questions and excel in your Full Stack Developer (Java) interview. This comprehensive guide is essential for effective study and confidence building.
43 Full Stack Developer (Java) Questions and Answers:
Full Stack Developer (Java) Job Interview Questions Table of Contents:
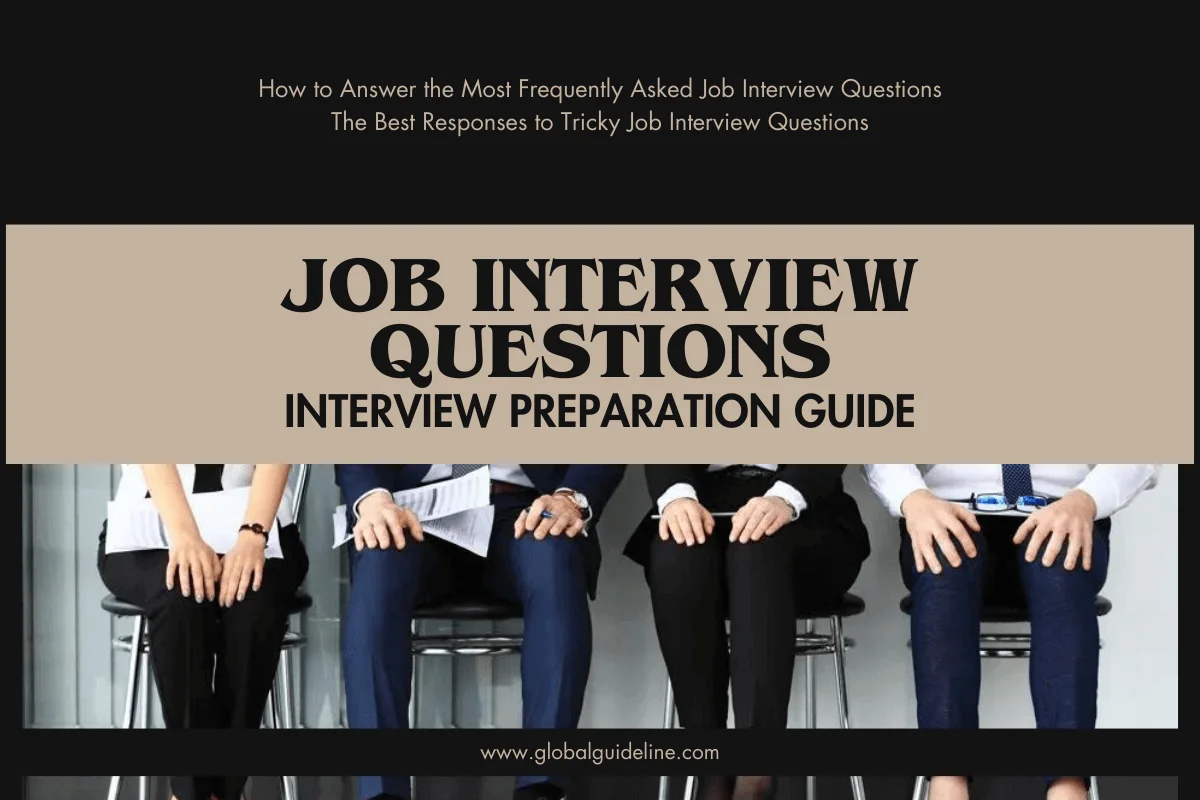
1 :: Tell me what is Bridge pattern?
Bridge pattern is used when we need to decouple an abstraction from its implementation so that the two can vary independently. This type of design pattern comes under structural pattern as this pattern decouples implementation class and abstract class by providing a bridge structure between them.
The bridge pattern is useful when both the class and what it does vary often. The class itself can be thought of as the abstraction and what the class can do as the implementation. The bridge pattern can also be thought of as two layers of abstraction.
Read MoreThe bridge pattern is useful when both the class and what it does vary often. The class itself can be thought of as the abstraction and what the class can do as the implementation. The bridge pattern can also be thought of as two layers of abstraction.
2 :: Can you please explain what is CORS? How does it work?
Cross-origin resource sharing (CORS) is a mechanism that allows many resources (e.g., fonts, JavaScript, etc.) on a web page to be requested from another domain outside the domain from which the resource originated. It’s a mechanism supported in HTML5 that manages XMLHttpRequest access to a domain different.
CORS adds new HTTP headers that provide access to permitted origin domains. For HTTP methods other than GET (or POST with certain MIME types), the specification mandates that browsers first use an HTTP OPTIONS request header to solicit a list of supported (and available) methods from the server. The actual request can then be submitted. Servers can also notify clients whether “credentials” (including Cookies and HTTP Authentication data) should be sent with requests.
Read MoreCORS adds new HTTP headers that provide access to permitted origin domains. For HTTP methods other than GET (or POST with certain MIME types), the specification mandates that browsers first use an HTTP OPTIONS request header to solicit a list of supported (and available) methods from the server. The actual request can then be submitted. Servers can also notify clients whether “credentials” (including Cookies and HTTP Authentication data) should be sent with requests.
3 :: Tell me what is your favorite language and why?
Full Stack Web Developers work with a multitude of languages. Having just one loved coding language might be an alarm signal. Ideally, a candidate must have a few languages that he loves, preferably, some with which he can design the front end and others with which he can take care of the back end. A candidate should be able to demonstrate that well and remember to include the basic most used ones like HTML, CSS, Python etc.
Read More4 :: Tell us are you aware of design patterns?
While answering this question you should show your ability to understand common errors that might creep in while creating web applications. If your knowledge is formal and deep, you must push the employer to gain confidence in the experience you possess in understanding a clean and readable code.
Read More5 :: Explain me what Are The Latest Trends In Full Stack Web Development?
According to industry experts, a candidate who is passionate about full stack development should be aware of the following trends:
☛ The rise of Vue JS Functional, real-time web apps, progressive apps and mobile web development.
☛ Programming benefits from JavaScript improvements
☛ The emergence of more compatible extensions.
This question will help filter those candidates who are not keen on upskilling in technology. If you read blogs or books on web development or attend seminars/webinars on the same topics, don’t forget to mention it.
Displaying how up-to-date you are in the field will give the interviewer enough confidence to hire you.
Read More☛ The rise of Vue JS Functional, real-time web apps, progressive apps and mobile web development.
☛ Programming benefits from JavaScript improvements
☛ The emergence of more compatible extensions.
This question will help filter those candidates who are not keen on upskilling in technology. If you read blogs or books on web development or attend seminars/webinars on the same topics, don’t forget to mention it.
Displaying how up-to-date you are in the field will give the interviewer enough confidence to hire you.
6 :: Tell me how do you run a Java application on the command line and set the classpath with multiple jars?
Some people will be thinking “what!?” but I’ve met a lot of Java developers who’ve not run a Java application outside of an IDE for years.
java -cp /dev/myapp.jar:/dev/mydependency.jar com.codementor.MyApp
Read Morejava -cp /dev/myapp.jar:/dev/mydependency.jar com.codementor.MyApp
7 :: Can you write a function to detect if two strings are anagrams (for example, SAVE and VASE)?
This is my go-to first interview question. It helps me gauge a candidate’s ability to understand a problem and write an algorithm to solve it.
If someone has not solved the problem before, I expect to see some code with loops and if/then’s. Maybe some HashMaps. The things I look for are ability to break down the problem to see what you need to check, what the edge cases are, and whether the code meets those criteria.
The naive solution is often to loop through the letters of the first string and see if they’re all in the second string. The next thing to look for is, the candidate should also do that in reverse too (check string 1 for string 2’s letters)? The next thing to look for is, what about strings with duplicate letters, like VASES?
Read MoreIf someone has not solved the problem before, I expect to see some code with loops and if/then’s. Maybe some HashMaps. The things I look for are ability to break down the problem to see what you need to check, what the edge cases are, and whether the code meets those criteria.
The naive solution is often to loop through the letters of the first string and see if they’re all in the second string. The next thing to look for is, the candidate should also do that in reverse too (check string 1 for string 2’s letters)? The next thing to look for is, what about strings with duplicate letters, like VASES?
8 :: Tell me what's your favorite language, and why?
This is a good question to warm a candidate up before diving into more technical ones
Read More9 :: Do you know a use case for Docker?
☛ Docker a low overhead way to run virtual machines on your local box or in the cloud. Although they're not strictly distinct machines, nor do they need to boot an OS, they give you many of those benefits.
☛ Docker can encapsulate legacy applications, allowing you to deploy them to servers that might not otherwise be easy to setup with older packages & software versions.
☛ Docker can be used to build test boxes, during your deploy process to facilitate continuous integration testing.
☛ Docker can be used to provision boxes in the cloud, and with swarm you can orchestrate clusters too.
Read More☛ Docker can encapsulate legacy applications, allowing you to deploy them to servers that might not otherwise be easy to setup with older packages & software versions.
☛ Docker can be used to build test boxes, during your deploy process to facilitate continuous integration testing.
☛ Docker can be used to provision boxes in the cloud, and with swarm you can orchestrate clusters too.
10 :: Tell me what is the purpose of each of the HTTP request types when used with a RESTful web service?
The purpose of each of the HTTP request types when used with a RESTful web service is as follows:
☛ GET: Retrieves data from the server (should only retrieve data and should have no other effect).
☛ POST: Sends data to the server for a new entity. It is often used when uploading a file or submitting a completed web form.
☛ PUT: Similar to POST, but used to replace an existing entity.
☛ PATCH: Similar to PUT, but used to update only certain fields within an existing entity.
☛ DELETE: Removes data from the server.
☛ TRACE: Provides a means to test what a machine along the network path receives when a request is made. As such, it simply returns what was sent.
☛ OPTIONS: Allows a client to request information about the request methods supported by a service. The relevant response header is Allow and it simply lists the supported methods. (It can also be used to request information about the request methods supported for the server where the service resides by using a * wildcard in the URI.)
☛ HEAD: Same as the GET method for a resource, but returns only the response headers (i.e., with no entity-body).
☛ CONNECT: Primarily used to establish a network connection to a resource (usually via some proxy that can be requested to forward an HTTP request as TCP and maintain the connection). Once established, the response sends a 200 status code and a “Connection Established” message.
Read More☛ GET: Retrieves data from the server (should only retrieve data and should have no other effect).
☛ POST: Sends data to the server for a new entity. It is often used when uploading a file or submitting a completed web form.
☛ PUT: Similar to POST, but used to replace an existing entity.
☛ PATCH: Similar to PUT, but used to update only certain fields within an existing entity.
☛ DELETE: Removes data from the server.
☛ TRACE: Provides a means to test what a machine along the network path receives when a request is made. As such, it simply returns what was sent.
☛ OPTIONS: Allows a client to request information about the request methods supported by a service. The relevant response header is Allow and it simply lists the supported methods. (It can also be used to request information about the request methods supported for the server where the service resides by using a * wildcard in the URI.)
☛ HEAD: Same as the GET method for a resource, but returns only the response headers (i.e., with no entity-body).
☛ CONNECT: Primarily used to establish a network connection to a resource (usually via some proxy that can be requested to forward an HTTP request as TCP and maintain the connection). Once established, the response sends a 200 status code and a “Connection Established” message.
11 :: Tell us what’s the difference between GET and POST?
Both are methods used in HTTP requests. Generally it is said that GET is to download data and PUT is to upload data. But we can do both downloading as well as uploading either by GET/POST.
☛ GET:
If we are sending parameters in a GET request to the server, then those parameters will be visible in the URL, because in GET, parameters are append to the URL. So there’s a lack of security while uploading to the server.
We can only send a limited amount of data in a GET request, because the URL has its max limit and we can not append a long data string to the URL.
☛ POST:
If we are using POST then we are sending parameters in the body section of a request. If we send data after using encryption in the body of an http request, it’s quite a bit more secure.
We can send a lot more data using POST.
Read More☛ GET:
If we are sending parameters in a GET request to the server, then those parameters will be visible in the URL, because in GET, parameters are append to the URL. So there’s a lack of security while uploading to the server.
We can only send a limited amount of data in a GET request, because the URL has its max limit and we can not append a long data string to the URL.
☛ POST:
If we are using POST then we are sending parameters in the body section of a request. If we send data after using encryption in the body of an http request, it’s quite a bit more secure.
We can send a lot more data using POST.
12 :: Tell us can you relate of an experience when you found your colleagues code to be inefficient? How did you deal with it?
This helps to determine a candidate’s quality standards and also gives an impression of his team playing skills. You must demonstrate that you have high-quality assurance standards, and show comfort in pointing out flaws or for that matter bugs to others. However, you must pay focus on portraying that you are good at giving positive feedback, getting the work done without creating resentment.
Read More13 :: Explain me something About Your Favorite Language And Why?
In addition to being an ice-breaker, this is a good question to know how well the candidate enjoys coding and working with programming languages. An ideal candidate for the full stack developer job should have multiple favorite languages rather than just a single one. Your answer should definitely include HTML, CSS, Javascript, Python or Ruby On Rails etc.
Read More14 :: Explain me what is the difference between String, StringBuffer andStringBuilder?
String is an immutable class. In older JDK’s the recommendation when programmatically building a String was to use StringBuffer since this was optimized to concatenate multiple Strings together. However, the methods on StringBuffer were marked as sychronized, which meant that there was a performance penalty, hence StringBuilder was introduced to provide a non-synchronized way to efficiently concatenate and modify Strings.
Read More15 :: Please explain what is the contract between equals and hashCode of an object?
The only obligation is that for any objects o1 and o2 then if o1.equals(o2) is true then o1.hashCode() == o2.hashCode() is true.
Note that this relationship goes only one way: for any o1, o2 of some class C, where none of o1 and o2 are null, then it can happen that o1.hashCode() == o2.hashCode() is true BUT o1.equals(o2) is false.
Read MoreNote that this relationship goes only one way: for any o1, o2 of some class C, where none of o1 and o2 are null, then it can happen that o1.hashCode() == o2.hashCode() is true BUT o1.equals(o2) is false.
16 :: Explain me what’s the difference between a ClassNotFoundException and NoClassDefFoundError?
A ClassNotFoundException means the class file for a requested class is not on the classpath of the application. A NoClassDefFoundErrormeans that the class file existed at runtime, but for some reason the class could not be turned into a Class definition. A common cause is an exception being thrown in static initialization blocks.
Read More17 :: Tell us have You Ever Struggled To Implement Or Debug Something In The Past While Working On Web Development?
This could be easily one among the trickiest questions in an average job interview. Any experienced full stack developer would have struggled at some point to implement or debug something. As a candidate, you should explain in a crisp manner why you faced the issue. Don’t forget to end the answer with how you overcame the roadblock and what you learned from the experience at the level of an individual or team player.
Read More18 :: Tell us what is the biggest mistake you did in any of your projects? How did you rectify it?
As they say, ‘No man is an island’. Similarly, you can’t be working on technology and be right all the time. That is not imaginable. You need to be honest here and talk about a mistake that you think was serious. To add to it, speak about your learnings from this mistake and explain the procedure you adopted to minimize the damage done.
Read More19 :: Explain me which technologies and languages would you need to develop a project from scratch?
This is a hypothetical question geared at understanding the level at which the hiring manager will gauge your readiness to start the job. It is an easy way to distinguish a good full stack developer from someone who is an amateur. People who have difficulty transmitting their thoughts will have bleak chances of getting through at this point.
Read More20 :: Explain me what is an ETag and how does it work?
An ETag is an opaque identifier assigned by a web server to a specific version of a resource found at an URL. If the resource content at that URL ever changes, a new and different ETag is assigned.
In typical usage, when an URL is retrieved the web server will return the resource along with its corresponding ETag value, which is placed in an HTTP “ETag” field:
ETag: "unique_id_of_resource_version"
The client may then decide to cache the resource, along with its ETag. Later, if the client wants to retrieve the same URL again, it will send its previously saved copy of the ETag along with the request in a "If-None-Match" field.
If-None-Match: "unique_id_of_resource_version"
On this subsequent request, the server may now compare the client’s ETag with the ETag for the current version of the resource. If the ETag values match, meaning that the resource has not changed, then the server may send back a very short response with a HTTP 304 Not Modified status. The 304 status tells the client that its cached version is still good and that it should use that.
However, if the ETag values do not match, meaning the resource has likely changed, then a full response including the resource’s content is returned, just as if ETag were not being used. In this case the client may decide to replace its previously cached version with the newly returned resource and the new ETag.
Read MoreIn typical usage, when an URL is retrieved the web server will return the resource along with its corresponding ETag value, which is placed in an HTTP “ETag” field:
ETag: "unique_id_of_resource_version"
The client may then decide to cache the resource, along with its ETag. Later, if the client wants to retrieve the same URL again, it will send its previously saved copy of the ETag along with the request in a "If-None-Match" field.
If-None-Match: "unique_id_of_resource_version"
On this subsequent request, the server may now compare the client’s ETag with the ETag for the current version of the resource. If the ETag values match, meaning that the resource has not changed, then the server may send back a very short response with a HTTP 304 Not Modified status. The 304 status tells the client that its cached version is still good and that it should use that.
However, if the ETag values do not match, meaning the resource has likely changed, then a full response including the resource’s content is returned, just as if ETag were not being used. In this case the client may decide to replace its previously cached version with the newly returned resource and the new ETag.
21 :: General Full Stack Developer (Java) Job Interview Questions:
☛ How can you define such terms as inversion control, virtual function, and dynamic bidding?
☛ How can you define tail recursion?
☛ Describe the working process of the garbage collector. What’s it like?
☛ What is A/B testing and when it is applicable?
☛ What design principles do you know?
☛ If separation of concerns is not used in development, what will be the drawbacks to your mind?
☛ What are the pros of microservices?
☛ What is 3-tier architecture?
☛ What is 3-layer architecture?
☛ Why use an application server? What is it for?
☛ How do you understand the aspect-oriented programming?
Read More☛ How can you define tail recursion?
☛ Describe the working process of the garbage collector. What’s it like?
☛ What is A/B testing and when it is applicable?
☛ What design principles do you know?
☛ If separation of concerns is not used in development, what will be the drawbacks to your mind?
☛ What are the pros of microservices?
☛ What is 3-tier architecture?
☛ What is 3-layer architecture?
☛ Why use an application server? What is it for?
☛ How do you understand the aspect-oriented programming?
22 :: Tell me the main difference between REST and GraphQL?
The main and most important difference between REST and GraphQL is that GraphQL is not dealing with dedicated resources, instead everything is regarded as a graph and therefore is connected and can be queried to app exact needs.
Read More23 :: Tell me how do you stay aware of new technologies related to full stack web development?
This question is asked to find out how involved or uninvolved a candidate is in the technology community. Interviewees should respond by describing a few meetups they are part of related to JavaScript, or personal projects that they have displayed in GitHub
Read More24 :: Please explain what is the best implementation or debugging you have done in the past?
This is a tricky question. This will actually give the hiring manager an idea of both the complexity and the style of projects you have done in the past. You should explicitly mention the issues you faced and the measures you took to overcome that roadblock. You can additionally speak about the learnings you earned from the issue.
Read More25 :: Do you know how does Garbage Collection prevent a Java application from going out of memory?
This is a tricky one… it doesn’t! Garbage Collection simply cleans up unused memory when an object goes out of scope and is no longer needed. However an application could create a huge number of large objects that causes an OutOfMemoryError.
Read More