C++ Programmer Question:
Download Job Interview Questions and Answers PDF
Tell me when you should use virtual inheritance?
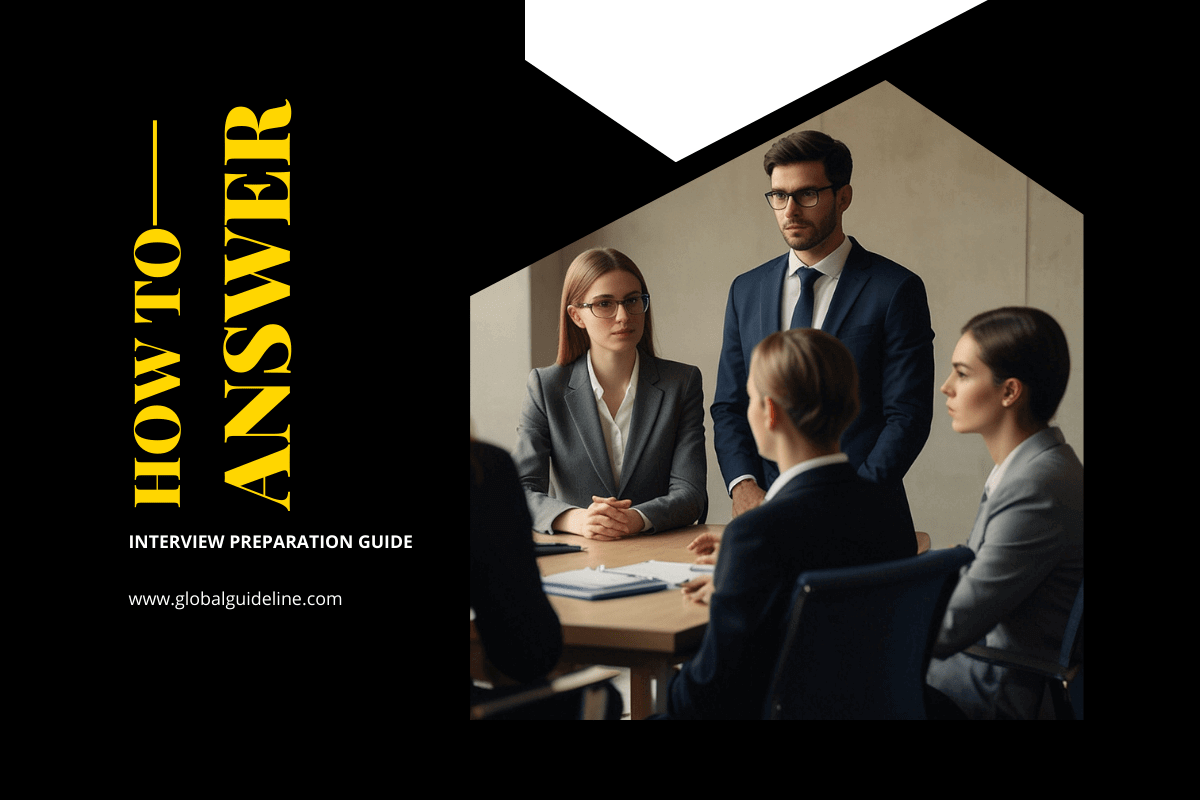
Answer:
While it’s ideal to avoid virtual inheritance altogether (you should know how your class is going to be used) having a solid understanding of how virtual inheritance works is still important:
So when you have a class (class A) which inherits from 2 parents (B and C), both of which share a parent (class D), as demonstrated below:
#include <iostream>
class D {
public:
void foo() {
std::cout << "Foooooo" << std::endl;
}
};
class C: public D {
};
class B: public D {
};
class A: public B, public C {
};
int main(int argc, const char * argv[]) {
A a;
a.foo();
}
If you don’t use virtual inheritance in this case, you will get two copies of D in class A: one from B and one from C. To fix this you need to change the declarations of classes C and B to be virtual, as follows:
class C: virtual public D {
};
class B: virtual public D {
};
So when you have a class (class A) which inherits from 2 parents (B and C), both of which share a parent (class D), as demonstrated below:
#include <iostream>
class D {
public:
void foo() {
std::cout << "Foooooo" << std::endl;
}
};
class C: public D {
};
class B: public D {
};
class A: public B, public C {
};
int main(int argc, const char * argv[]) {
A a;
a.foo();
}
If you don’t use virtual inheritance in this case, you will get two copies of D in class A: one from B and one from C. To fix this you need to change the declarations of classes C and B to be virtual, as follows:
class C: virtual public D {
};
class B: virtual public D {
};
Download C++ Programmer Interview Questions And Answers
PDF
Previous Question | Next Question |
Tell me which compiler switch to be used for compiling the programs using math library with g++ compiler? | Explain me what do you mean by translation unit in c++? |