C++ Exception Handling Question:
Can you please Illustrate Rethrowing exceptions?
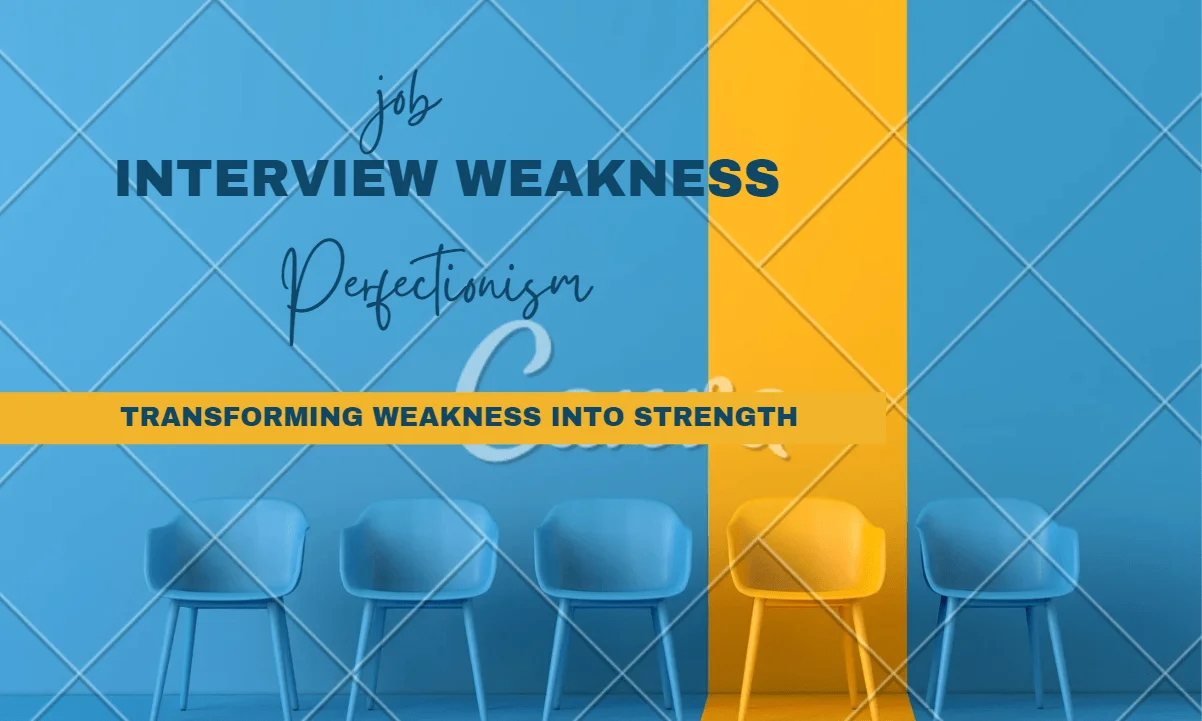
Answer:
Rethrowing an expression from within an exception handler can be done by calling throw, by itself, with no exception. This causes current exception to be passed on to an outer try/catch sequence. An exception can only be rethrown from within a catch block. When an exception is rethrown, it is propagated outward to the next catch block.
Consider following code:
#include <iostream>
using namespace std;
void MyHandler()
{
try
{
throw “hello”;
}
catch (const char*)
{
cout <<”Caught exception inside MyHandler\n”;
throw; //rethrow char* out of function
}
}
int main()
{
cout<< “Main start”;
try
{
MyHandler();
}
catch(const char*)
{
cout <<”Caught exception inside Main\n”;
}
cout << “Main end”;
return 0;
}
O/p:
Main start
Caught exception inside MyHandler
Caught exception inside Main
Main end
Thus, exception rethrown by the catch block inside MyHandler() is caught inside main();
Consider following code:
#include <iostream>
using namespace std;
void MyHandler()
{
try
{
throw “hello”;
}
catch (const char*)
{
cout <<”Caught exception inside MyHandler\n”;
throw; //rethrow char* out of function
}
}
int main()
{
cout<< “Main start”;
try
{
MyHandler();
}
catch(const char*)
{
cout <<”Caught exception inside Main\n”;
}
cout << “Main end”;
return 0;
}
O/p:
Main start
Caught exception inside MyHandler
Caught exception inside Main
Main end
Thus, exception rethrown by the catch block inside MyHandler() is caught inside main();
Previous Question | Next Question |
Explain Exception handling for a class with an example? | Generic catch handler is represented by ______________. a. catch(..,) b. catch(---) c. catch(…) d. catch( void x) |