JQuery Programmer Interview Questions And Answers
Download JQuery Programmer Interview Questions and Answers PDF
Refine your JQuery Programmer interview skills with our 201 critical questions. Each question is designed to test and expand your JQuery Programmer expertise. Suitable for all experience levels, these questions will help you prepare thoroughly. Secure the free PDF to access all 201 questions and guarantee your preparation for your JQuery Programmer interview. This guide is crucial for enhancing your readiness and self-assurance.
201 JQuery Programmer Questions and Answers:
JQuery Programmer Job Interview Questions Table of Contents:
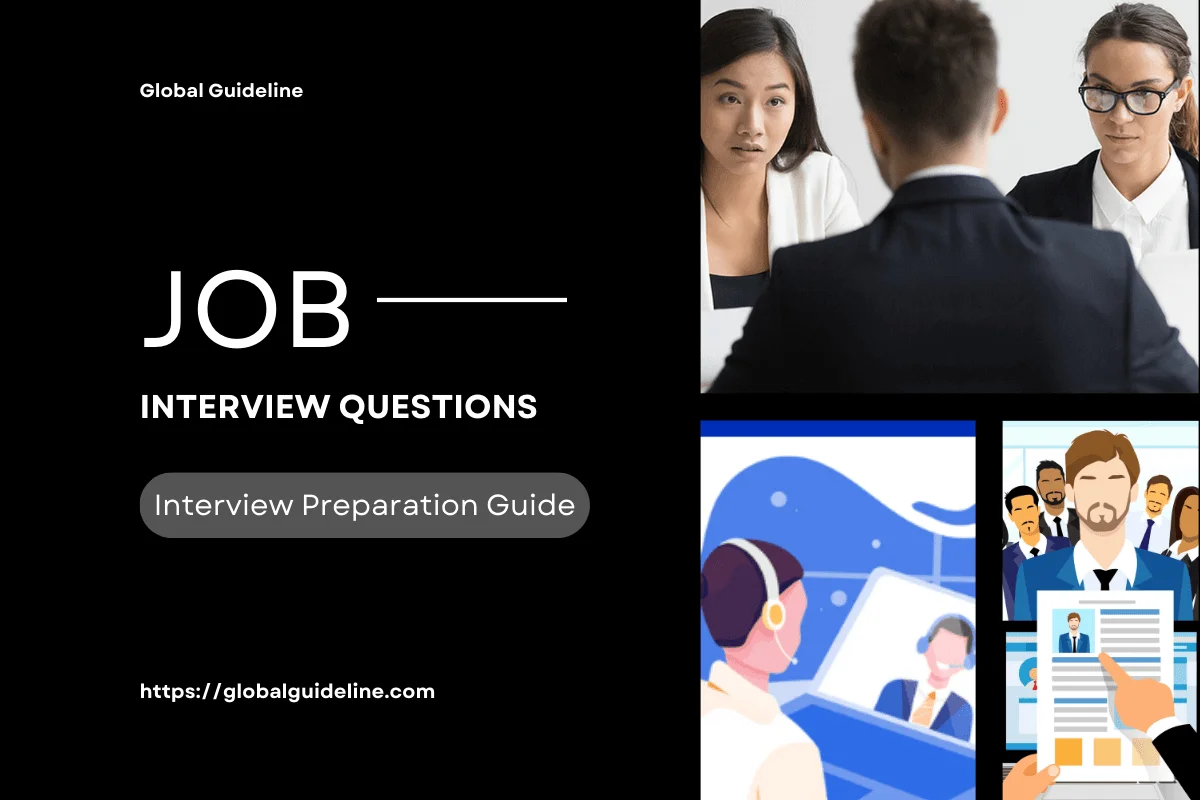
1 :: Explain what is jQuery?
jQuery is a fast and concise JavaScript Library created by John Resig in 2006 with a nice motto - Write less, do more. jQuery simplifies HTML document traversing, event handling, animating, and Ajax interactions for rapid web development. jQuery is a JavaScript toolkit designed to simplify various tasks by writing less code.
jQuery is not a programming language but a well written JavaScript code. It is a JavaScript code, which do document traversing, event handling, Ajax interactions and Animations.
Read MorejQuery is not a programming language but a well written JavaScript code. It is a JavaScript code, which do document traversing, event handling, Ajax interactions and Animations.
2 :: In what scenarios jQuery can be used?
jQuery can be used in following scenarios:
☛ Apply CSS static or dynamic
☛ Calling functions on events
☛ Manipulation purpose
☛ Mainly for Animation effects
Read More☛ Apply CSS static or dynamic
☛ Calling functions on events
☛ Manipulation purpose
☛ Mainly for Animation effects
3 :: Tell me what is jQuery connect?
A 'jQuery connect' is a plugin used to connect or bind a function with another function. Connect is used to execute function from any other function or plugin is executed.
Read More4 :: How to use connect?
Connect can be used by downloading jQuery connect file from jQuery.com and then include that file in the HTML file. Use $.connect function to connect a function to another function.
Read More5 :: What are the basic selectors in jQuery?
Following are the basic selectors in jQuery:
► Element ID
► CSS Name
► Tag Name
► DOM hierarchy
Read More► Element ID
► CSS Name
► Tag Name
► DOM hierarchy
6 :: What is the use of jQuery load method?
jQuery load method is a powerful AJAX method which is used to load the data from a server and assign the data into the element without loading the page.
Read More7 :: What is CDN?
CDN is abbreviated as Content Distribution network and it is said to be a group of companies in different location with network containing copies of data files to maximize bandwidth in accessing the data.
Read More8 :: How can we debug jQuery?
There are two ways to debug jQuery:
Debugger keyword
► Add the debugger to the line from where we have to start debugging and then run Visual Studio in Debug mode with F5 function key.
► Insert a break point after attaching the process
Read MoreDebugger keyword
► Add the debugger to the line from where we have to start debugging and then run Visual Studio in Debug mode with F5 function key.
► Insert a break point after attaching the process
9 :: What is the use of jQuery.data() method?
jQuery data method is used to associate data with DOM nodes and JavaScript objects. This method will make a code very concise and neat.
Read More10 :: Why jQuery is better than JavaScript?
jQuery is a library used for developing Ajax application and it helps to write the code clean and concise. It also handles events, animation and Ajax support applications.
Read More11 :: How method can be called inside code behind using jQuery?
$.ajax can be called and by declaring WebMethod inside code behind using jQuery.
Read More12 :: Tell us what are the advantages of jQuery?
Following are the advantages of jQuery:
► Just a JavaScript enhancement
► Coding is simple, clear, reusable
► Removal of writing more complex conditions and loops
Read More► Just a JavaScript enhancement
► Coding is simple, clear, reusable
► Removal of writing more complex conditions and loops
14 :: What are the four parameters used for jQuery Ajax method?
The four parameters are
► URL - Need to specify the URL to send the request
► type - Specifies type of request(Get or Post)
► data - Specifies data to be sent to server
► Cache - Whether the browser should cache the requested page
Read More► URL - Need to specify the URL to send the request
► type - Specifies type of request(Get or Post)
► data - Specifies data to be sent to server
► Cache - Whether the browser should cache the requested page
15 :: Whether we need to add jQuery file in both Master and Content page?
jQuery file should be added to the Master page and can use access from the content page directly without having any reference to it.
Read More16 :: What are the methods used to provide effects?
Some of the effects methods are:
☷ Show()
☷ Hide()
☷ Toggle()
☷ FadeIn() and
☷ FadeOut()
Read More☷ Show()
☷ Hide()
☷ Toggle()
☷ FadeIn() and
☷ FadeOut()
17 :: Why jQuery is needed?
jQuery is needed for the following list:
► Used to develop browser compatible web applications
► Improve the performance of an application
► Very fast and extensible
► UI related functions are written in minimal lines of codes
Read More► Used to develop browser compatible web applications
► Improve the performance of an application
► Very fast and extensible
► UI related functions are written in minimal lines of codes
18 :: What are the browser related issues for jQuery?
Browser compatibility of jQuery plugin is an issue and needs lot of time to fix it.
Read More19 :: Which program is useful for testing jQuery?
QUnit is used to test jQuery and it is very easy and efficient.
Read More20 :: What is called chaining?
Chaining is used to connect multiple events and functions in a selector.
Read More21 :: What is the method used to define the specific character in place of $ sign?
'NoConflict' method is used to reference a jQuery and save it in a variable. That variable can be used instead of Sign.
Read More22 :: What are the core features of jQuery?
Following is the list of important core features supported by jQuery −
► DOM manipulation − The jQuery made it easy to select DOM elements, traverse them and modifying their content by using cross-browser open source selector engine called Sizzle.
► Event handling − The jQuery offers an elegant way to capture a wide variety of events, such as a user clicking on a link, without the need to clutter the HTML code itself with event handlers.
► AJAX Support − The jQuery helps you a lot to develop a responsive and feature-rich site using AJAX technology.
► Animations − The jQuery comes with plenty of built-in animation effects which you can use in your websites.
► Lightweight − The jQuery is very lightweight library - about 19KB in size ( Minified and gzipped ).
► Cross Browser Support − The jQuery has cross-browser support, and works well in IE 6.0+, FF 2.0+, Safari 3.0+, Chrome and Opera 9.0+.
► Latest Technology − The jQuery supports CSS3 selectors and basic XPath syntax.
Read More► DOM manipulation − The jQuery made it easy to select DOM elements, traverse them and modifying their content by using cross-browser open source selector engine called Sizzle.
► Event handling − The jQuery offers an elegant way to capture a wide variety of events, such as a user clicking on a link, without the need to clutter the HTML code itself with event handlers.
► AJAX Support − The jQuery helps you a lot to develop a responsive and feature-rich site using AJAX technology.
► Animations − The jQuery comes with plenty of built-in animation effects which you can use in your websites.
► Lightweight − The jQuery is very lightweight library - about 19KB in size ( Minified and gzipped ).
► Cross Browser Support − The jQuery has cross-browser support, and works well in IE 6.0+, FF 2.0+, Safari 3.0+, Chrome and Opera 9.0+.
► Latest Technology − The jQuery supports CSS3 selectors and basic XPath syntax.
23 :: What is closure?
Closures are created whenever a variable that is defined outside the current scope is accessed from within some inner scope.
Read More24 :: What is a jQuery selector?
A jQuery Selector is a function which makes use of expressions to find out matching elements from a DOM based on the given criteria. Simply you can say, selectors are used to select one or more HTML elements using jQuery. Once an element is selected then we can perform various operations on that selected element. jQuery selectors start with the dollar sign and parentheses - $().
Read More25 :: How to select single element using jQuery with the given element id some-id?
$('#some-id') selects the single element in the document that has an ID of some-id.
Read More