Expert JavaScript Developer Interview Questions And Answers
Download Expert JavaScript Developer Interview Questions and Answers PDF
Strengthen your Expert JavaScript Developer interview skills with our collection of 58 important questions. These questions are specifically selected to challenge and enhance your knowledge in Expert JavaScript Developer. Perfect for all proficiency levels, they are key to your interview success. Get the free PDF download to access all 58 questions and excel in your Expert JavaScript Developer interview. This comprehensive guide is essential for effective study and confidence building.
58 Expert JavaScript Developer Questions and Answers:
Expert JavaScript Developer Job Interview Questions Table of Contents:
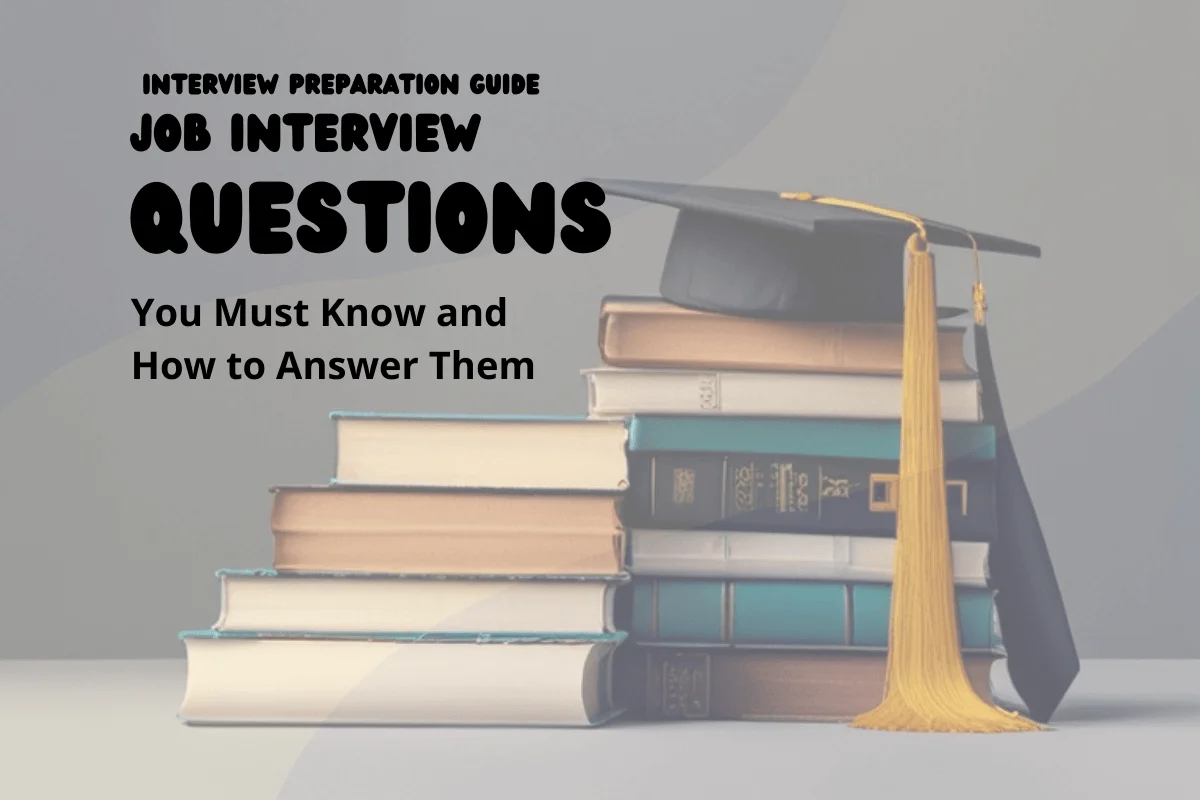
1 :: Explain JavaScript?
JavaScript is a general-purpose programming language designed to let programmers of all skill levels control the behavior of software objects. The language is used most widely today in Web browsers whose software objects tend to represent a variety of HTML elements in a document and the document itself. But the language can be--and is--used with other kinds of objects in other environments. For example, Adobe Acrobat Forms uses JavaScript as its underlying scripting language to glue together objects that are unique to the forms generated by Adobe Acrobat. Therefore, it is important to distinguish JavaScript, the language, from the objects it can communicate with in any particular environment. When used for Web documents, the scripts go directly inside the HTML documents and are downloaded to the browser with the rest of the HTML tags and content.
JavaScript is a platform-independent, event-driven, interpreted client-side scripting and programming language developed by Netscape Communications Corp. and Sun Microsystems.
Read MoreJavaScript is a platform-independent, event-driven, interpreted client-side scripting and programming language developed by Netscape Communications Corp. and Sun Microsystems.
2 :: How to submit a form using JavaScript?
Use document.forms[0].submit()
(0 refers to the index of the form - if we have more than one form in a page, then the first one has the index 0, second has index 1 and so on).
Read More(0 refers to the index of the form - if we have more than one form in a page, then the first one has the index 0, second has index 1 and so on).
3 :: What's relationship between JavaScript and ECMAScript?
ECMAScript is yet another name for JavaScript (other names include LiveScript). The current JavaScript that you see supported in browsers is ECMAScript revision 3.
Read More4 :: How to detect the operating system on the client machine?
In order to detect the operating system on the client machine, the navigator.appVersion string (property) should be used.
Read More5 :: Do you convert numbers between different bases in JavaScript?
Use the parseInt() function, that takes a string as the first parameter, and the base as a second parameter. So to convert hexadecimal 3F to decimal, use parseInt (" 3F" , 16).
Read More6 :: How to create arrays in JavaScript?
We can declare an array like this
var scripts = new Array()
We can add elements to this array like this
scripts[0] = " PHP"
scripts[1] = " ASP"
scripts[2] = " JavaScript"
scripts[3] = " HTML"
Now our array scripts have 4 elements inside it and we can print or access them by using their index number. Note that index number starts from 0. To get the third element of the array we have to use the index number 2. Here is the way to get the third element of an array.
document.write(scripts[2])
We also can create an array like this
var no_array = new Array(21, 22, 23, 24, 25)
Read Morevar scripts = new Array()
We can add elements to this array like this
scripts[0] = " PHP"
scripts[1] = " ASP"
scripts[2] = " JavaScript"
scripts[3] = " HTML"
Now our array scripts have 4 elements inside it and we can print or access them by using their index number. Note that index number starts from 0. To get the third element of the array we have to use the index number 2. Here is the way to get the third element of an array.
document.write(scripts[2])
We also can create an array like this
var no_array = new Array(21, 22, 23, 24, 25)
7 :: Do you target a specific frame from a hyperlink in JavaScript?
Include the name of the frame in the target attribute of the hyperlink in JavaScript. < a href="http://www.globalguideline.com" target="myframe"> Global Guide Line< /a>
Read More8 :: What are fixed-width table and its advantages in JavaScript?
Fixed width tables are rendered by the browser based on the widths of the columns in the first row, in JavaScript resulting in a faster display in case of large tables. Use the CSS style table-layout:fixed to specify a fixed width table.
If the table is not specified to be of fixed width in JavaScript, the browser has to wait till all data is downloaded and then infer the best width for each of the columns. This process can be very slow for large tables.
Read MoreIf the table is not specified to be of fixed width in JavaScript, the browser has to wait till all data is downloaded and then infer the best width for each of the columns. This process can be very slow for large tables.
9 :: Example of using Regular Expressions for syntax checking in JavaScript?
var re = new RegExp(" ^(&[A-Za-z_0-9]{1,}=[A-Za-z_0-9]{1,})*$" )
var text = myWidget.value
var OK = re.test(text)
if( ! OK ) {
alert(" The extra parameters need some work. Should be something like: " &a=1&c=4" " )
}
Read Morevar text = myWidget.value
var OK = re.test(text)
if( ! OK ) {
alert(" The extra parameters need some work. Should be something like: " &a=1&c=4" " )
}
10 :: How to add Buttons in JavaScript?
The most basic and ancient use of buttons are the " submit" and " clear" , which appear slightly before the Pleistocene period. Notice when the " GO!" button is pressed it submits itself to itself and appends the name in the URL.
< form action=" " name=" buttonsGalore" method=" get" >
Your Name: < input type=" text" name=" mytext" />
< br />
< input type=" submit" value=" GO!" />
< input type=" reset" value=" Clear All" />
< /form>
Another useful approach is to set the " type" to " button" and use the " onclick" event.
< script type=" text/javascript" >
function displayHero(button) {
alert(" Your hero is " " +button.value+" " ." )
}
< /script>
< form action=" " name=" buttonsGalore" method=" get" >
< fieldset style=" margin: 1em text-align: center " >
< legend> Select a Hero< /legend>
< input type=" button" value=" Agamemnon" onclick=" displayHero(this)" />
< input type=" button" value=" Achilles" onclick=" displayHero(this)" />
< input type=" button" value=" Hector" onclick=" displayHero(this)" />
< div style=" height: 1em " />
Read More< form action=" " name=" buttonsGalore" method=" get" >
Your Name: < input type=" text" name=" mytext" />
< br />
< input type=" submit" value=" GO!" />
< input type=" reset" value=" Clear All" />
< /form>
Another useful approach is to set the " type" to " button" and use the " onclick" event.
< script type=" text/javascript" >
function displayHero(button) {
alert(" Your hero is " " +button.value+" " ." )
}
< /script>
< form action=" " name=" buttonsGalore" method=" get" >
< fieldset style=" margin: 1em text-align: center " >
< legend> Select a Hero< /legend>
< input type=" button" value=" Agamemnon" onclick=" displayHero(this)" />
< input type=" button" value=" Achilles" onclick=" displayHero(this)" />
< input type=" button" value=" Hector" onclick=" displayHero(this)" />
< div style=" height: 1em " />
11 :: What can JavaScript programs do?
Generation of HTML pages on-the-fly without accessing the Web server. The user can be given control over the browser like User input validation Simple computations can be performed on the client's machine The user's browser, OS, screen size, etc. can be detected Date and Time Handling.
Read More12 :: How to set a HTML document's background color?
document.bgcolor property can be set to any appropriate color.
Read More13 :: Where are cookies actually stored on the hard disk?
This depends on the user's browser and OS.
In the case of Netscape with Windows OS, all the cookies are stored in a single file called
cookies.txt
c:Program FilesNetscapeUsersusernamecookies.txt
In the case of IE,each cookie is stored in a separate file namely username@website.txt.
c:WindowsCookiesusername@Website.txt
Read MoreIn the case of Netscape with Windows OS, all the cookies are stored in a single file called
cookies.txt
c:Program FilesNetscapeUsersusernamecookies.txt
In the case of IE,each cookie is stored in a separate file namely username@website.txt.
c:WindowsCookiesusername@Website.txt
14 :: Explain JavaScript Data Types?
JavaScript Data Types are Number, String, Boolean, Function, Object, Null, Undefined.
Read More15 :: How to read and write a file using JavaScript?
I/O operations like reading or writing a file is not possible with client-side JavaScript. However, this can be done by coding a Java applet that reads files for the script.
Read More16 :: Are you concerned to that older browsers don't support JavaScript and thus exclude a set of Web users? individual users?
Fragmentation of the installed base of browsers will only get worse. By definition, it can never improve unless absolutely everyone on the planet threw away their old browsers and upgraded to the latest gee-whiz versions. But even then, there are plenty of discrepancies between the script ability of the latest Netscape Navigator and Microsoft Internet Explorer.
The situation makes scripting a challenge, especially for newcomers who may not be aware of the limitations of earlier browsers. A lot of effort in my books and ancillary material goes toward helping scripter know what features work in which browsers and how to either workaround limitations in earlier browsers or raise the compatibility common denominator.
Designing scripts for a Web site requires making some hard decisions about if, when, and how to implement the advantages scripting offers a page to your audience. For public Web sites, I recommend using scripting in an additive way: let sufficient content stand on its own, but let scriptable browser users receive an enhanced EXPERIENCE, preferably with the same HTML document.
Read MoreThe situation makes scripting a challenge, especially for newcomers who may not be aware of the limitations of earlier browsers. A lot of effort in my books and ancillary material goes toward helping scripter know what features work in which browsers and how to either workaround limitations in earlier browsers or raise the compatibility common denominator.
Designing scripts for a Web site requires making some hard decisions about if, when, and how to implement the advantages scripting offers a page to your audience. For public Web sites, I recommend using scripting in an additive way: let sufficient content stand on its own, but let scriptable browser users receive an enhanced EXPERIENCE, preferably with the same HTML document.
17 :: Can JavaScript be used to improve the "look and feel" of a Web site? By the same token, how can JavaScript be used to improve the user interface?
On their own, Web pages tend to be lifeless and flat unless you add animated images or more bandwidth-intensive content such as Java applets or other content requiring plug-ins to operate (Shockwave and Flash, for example).
Embedding JavaScript into an HTML page can bring the page to life in any number of ways. Perhaps the most visible features built into pages recently with the help of JavaScript are the so-called image rollovers: roll the cursor atop a graphic image and its appearance changes to a highlighted version as a feedback mechanism to let you know precisely what you're about to click on. But there are less visible yet more powerful enhancements to pages that JavaScript offers.
Interactive forms validation is an extremely useful application of JavaScript. While a user is entering data into form fields, scripts can examine the validity of the data--did the user type any letters into a phone number field?, for instance. Without scripting, the user has to submit the form and let a server program (CGI) check the field entry and then report back to the user. This is usually done in a batch mode (the entire form at once), and the extra transactions take a lot of time and server processing power. Interactive validation scripts can check each form field immediately after the user has entered the data, while the information is fresh in the mind.
Read MoreEmbedding JavaScript into an HTML page can bring the page to life in any number of ways. Perhaps the most visible features built into pages recently with the help of JavaScript are the so-called image rollovers: roll the cursor atop a graphic image and its appearance changes to a highlighted version as a feedback mechanism to let you know precisely what you're about to click on. But there are less visible yet more powerful enhancements to pages that JavaScript offers.
Interactive forms validation is an extremely useful application of JavaScript. While a user is entering data into form fields, scripts can examine the validity of the data--did the user type any letters into a phone number field?, for instance. Without scripting, the user has to submit the form and let a server program (CGI) check the field entry and then report back to the user. This is usually done in a batch mode (the entire form at once), and the extra transactions take a lot of time and server processing power. Interactive validation scripts can check each form field immediately after the user has entered the data, while the information is fresh in the mind.
18 :: How can JavaScript make a Web site easier to use? That is, are there certain JavaScript techniques that make it easier for people to use a Web site?
JavaScript's greatest potential gift to a Web site is that scripts can make the page more immediately interactive, that is, interactive without having to submit every little thing to the server for a server program to re-render the page and send it back to the client. For example, consider a top-level navigation panel that has, say, six primary image map links into subsections of the Web site. With only a little bit of scripting, each map area can be instructed to pop up a more detailed list of links to the contents within a subsection whenever the user rolls the cursor atop a map area. With the help of that popup list of links, the user with a scriptable browser can bypass one intermediate menu page. The user without a scriptable browser (or who has disabled JavaScript) will have to drill down through a more traditional and time-consuming path to the desired content.
Read More19 :: How is JavaScript different from Java?
JavaScript was developed by Brendan Eich of Netscape Java was developed at Sun Microsystems. While the two languages share some common syntax, they were developed independently of each other and for different audiences. Java is a full-fledged programming language tailored for network computing it includes hundreds of its own objects, including objects for creating user interfaces that appear in Java applets (in Web browsers) or standalone Java applications. In contrast, JavaScript relies on whatever environment it's operating in for the user interface, such as a Web document's form elements.
JavaScript was initially called LiveScript at Netscape while it was under development. A licensing deal between Netscape and Sun at the last minute let Netscape plug the "Java" name into the name of its scripting language. Programmers use entirely different tools for Java and JavaScript. It is also not uncommon for a programmer of one language to be ignorant of the other. The two languages don't rely on each other and are intended for different purposes. In some ways, the "Java" name on JavaScript has confused the world's understanding of the differences between the two. On the other hand, JavaScript is much easier to learn than Java and can offer a gentle introduction for newcomers who want to graduate to Java and the kinds of applications you can develop with it.
Read MoreJavaScript was initially called LiveScript at Netscape while it was under development. A licensing deal between Netscape and Sun at the last minute let Netscape plug the "Java" name into the name of its scripting language. Programmers use entirely different tools for Java and JavaScript. It is also not uncommon for a programmer of one language to be ignorant of the other. The two languages don't rely on each other and are intended for different purposes. In some ways, the "Java" name on JavaScript has confused the world's understanding of the differences between the two. On the other hand, JavaScript is much easier to learn than Java and can offer a gentle introduction for newcomers who want to graduate to Java and the kinds of applications you can develop with it.
20 :: How JavaScript be used to personalize or tailor a Web site to fit individual users?
JavaScript allows a Web page to perform "if-then" kinds of decisions based on browser version, operating system, user input, and, in more recent browsers, details about the screen size in which the browser is running. While a server CGI program can make some of those same kinds of decisions, not everyone has access to or the expertise to create CGI programs. For example, an experienced CGI programmer can examine information about the browser whenever a request for a page is made thus a server so equipped might serve up one page for Navigator users and a different page for Internet Explorer users. Beyond browser and operating system version, a CGI program can't know more about the environment. But a JavaScript-enhanced page can instruct the browser to render only certain content based on the browser, operating system, and even the screen size.
Scripting can even go further if the page author desires. For example, the author may include a preference screen that lets the user determine the desired background and text color combination. A script can save this information on the client in a well-regulated local file called a cookie. The next time the user comes to the site, scripts in its pages look to the cookie info and render the page in the color combination selected previously. The server is none the wiser, nor does it have to store any visitor-specific information.
Read MoreScripting can even go further if the page author desires. For example, the author may include a preference screen that lets the user determine the desired background and text color combination. A script can save this information on the client in a well-regulated local file called a cookie. The next time the user comes to the site, scripts in its pages look to the cookie info and render the page in the color combination selected previously. The server is none the wiser, nor does it have to store any visitor-specific information.
22 :: Explain Methods GET and POST in HTML forms - what's the difference?
GET: Parameters are passed in the query string. Maximum amount of data that can be sent via the GET method is limited to about 2kb.
POST: Parameters are passed in the request body. There is no limit to the amount of data that can be transferred using POST. However, there are limits on the maximum amount of data that can be transferred in one name/value pair.
Read MorePOST: Parameters are passed in the request body. There is no limit to the amount of data that can be transferred using POST. However, there are limits on the maximum amount of data that can be transferred in one name/value pair.
23 :: Explain data type of variables of in JavaScript?
All variables are of object type in JavaScript.
Read More24 :: In a pop-up browser window, how do you refer to the main browser window that opened it?
Use window.opener to refer to the main window from pop-ups.
Read More25 :: Explain negative infinity?
It's a number in JavaScript, derived by dividing negative number by zero.
Read More