Expert Developer JavaScript Interview Questions And Answers
Sharpen your Expert JavaScript Developer interview expertise with our handpicked 150 questions. Each question is crafted to challenge your understanding and proficiency in Expert JavaScript Developer. Suitable for all skill levels, these questions are essential for effective preparation. Access the free PDF to get all 150 questions and give yourself the best chance of acing your Expert JavaScript Developer interview. This resource is perfect for thorough preparation and confidence building.
150 Expert JavaScript Developer Questions and Answers:
Expert JavaScript Developer Job Interview Questions Table of Contents:
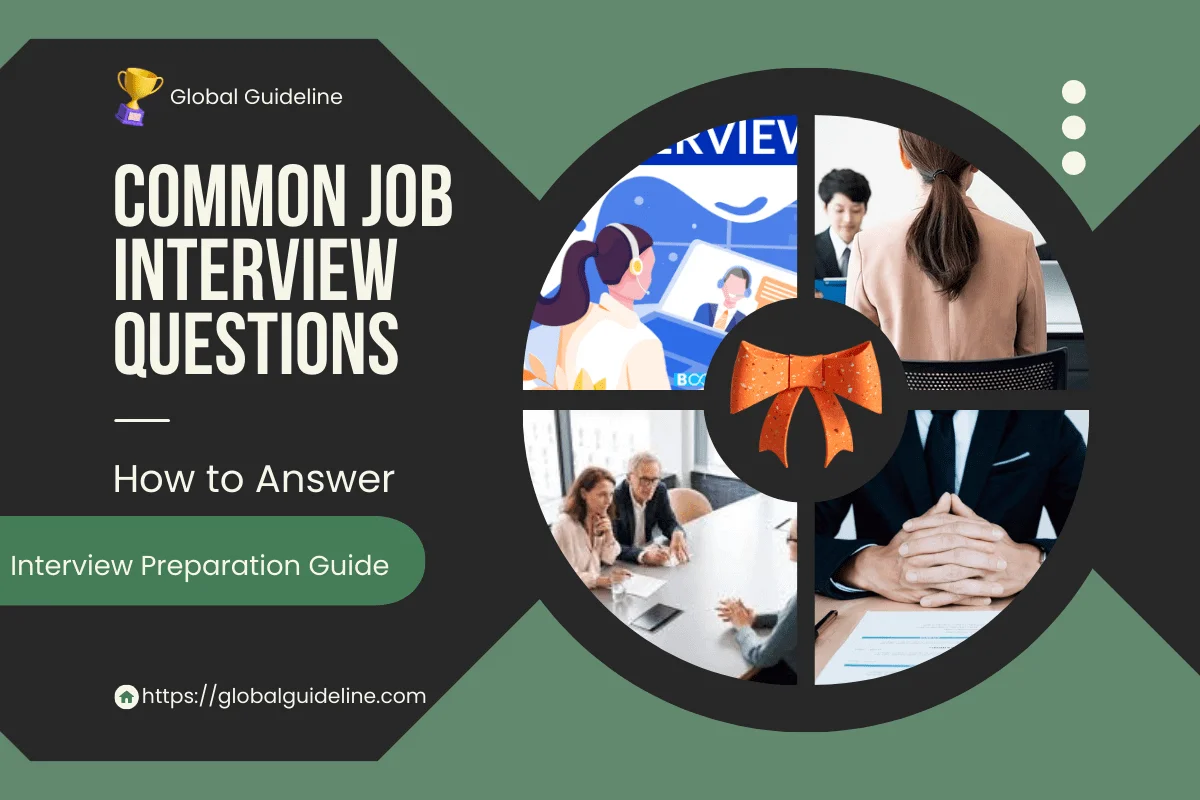
1 :: What is the use of isNaN function?
isNan function returns true if the argument is not a number otherwise it is false.
Read More2 :: Which company developed JavaScript?
Netscape is the software company who developed JavaScript.
Read More3 :: What is a prompt box in JavaScript?
A prompt box is a box which allows the user to enter input by providing a text box. Label and box will be provided to enter the text or number.
Read More4 :: Which symbol is used for comments in Javascript?
// for Single line comments and
/* Multi
Line
Comment
*/
Read More/* Multi
Line
Comment
*/
5 :: What is === operator in JavaScript?
=== is called as strict equality operator which returns true when the two operands are having the same value without any type conversion.
Read More6 :: What would be the result of 3+2+"7″?
Since 3 and 2 are integers, they will be added numerically. And since 7 is a string, its concatenation will be done. So the result would be 57.
Read More7 :: What is an undefined value in JavaScript?
Undefined value means the
► Variable used in the code doesn't exist
► Variable is not assigned to any value
► Property doesn't exist
Read More► Variable used in the code doesn't exist
► Variable is not assigned to any value
► Property doesn't exist
8 :: What are escape characters?
Escape characters (Backslash) is used when working with special characters like single quotes, double quotes, apostrophes and ampersands. Place backslash before the characters to make it display.
Example:
document.write "I m a "good" boy"
document.write "I m a "good" boy"
Read MoreExample:
document.write "I m a "good" boy"
document.write "I m a "good" boy"
9 :: What is the use of type of operator?
'Typeof' is an operator which is used to return a string description of the type of a variable.
Read More10 :: Tell me what is JavaScript?
JavaScript is a client-side scripting language that can be inserted into HTML pages and is understood by web browsers.
Read More11 :: Write the code for adding new elements dynamically?
<html>
<head> <title>t1</title>
<script type="text/javascript">
function addNode() { var newP = document.createElement("p");
var textNode = document.createTextNode(" This is a new text node");
newP.appendChild(textNode); document.getElementById("firstP").appendChild(newP); }
</script> </head>
<body> <p id="firstP">firstP<p> </body>
</html>
Read More<head> <title>t1</title>
<script type="text/javascript">
function addNode() { var newP = document.createElement("p");
var textNode = document.createTextNode(" This is a new text node");
newP.appendChild(textNode); document.getElementById("firstP").appendChild(newP); }
</script> </head>
<body> <p id="firstP">firstP<p> </body>
</html>
12 :: How can the style/class of an element be changed?
It can be done in the following way:
JavaScript
document.getElementById("myText").style.fontSize = "20?;
or
document.getElementById("myText").className = "anyclass";
Read MoreJavaScript
document.getElementById("myText").style.fontSize = "20?;
or
document.getElementById("myText").className = "anyclass";
13 :: Explain how to detect the operating system on the client machine?
In order to detect the operating system on the client machine, the navigator.appVersion string (property) should be used.
Read More14 :: What is the data type of variables in JavaScript?
All variables in the JavaScript are object data types.
Read More15 :: Whether JavaScript has concept level scope?
No. JavaScript does not have concept level scope. The variable declared inside the function has scope inside the function.
Read More16 :: How generic objects can be created?
Generic objects can be created as:
var I = new object();
Read Morevar I = new object();
17 :: How to find operating system in the client machine using JavaScript?
The 'Navigator.appversion' is used to find the name of the operating system in the client machine.
Read More18 :: How are object properties assigned?
Properties are assigned to objects in the following way -
obj["class"] = 12;
or
obj.class = 12;
Read Moreobj["class"] = 12;
or
obj.class = 12;
19 :: What are JavaScript types?
Following are the JavaScript types:
Number
String
Boolean
Function
Object
Null
Undefined
Read MoreNumber
String
Boolean
Function
Object
Null
Undefined
20 :: What are undeclared and undefined variables?
Undeclared variables are those that do not exist in a program and are not declared. If the program tries to read the value of an undeclared variable, then a runtime error is encountered.
Undefined variables are those that are declared in the program but have not been given any value. If the program tries to read the value of an undefined variable, an undefined value is returned.
Read MoreUndefined variables are those that are declared in the program but have not been given any value. If the program tries to read the value of an undefined variable, an undefined value is returned.
21 :: Does JavaScript support automatic type conversion?
Yes JavaScript does support automatic type conversion, it is the common way of type conversion used by JavaScript developers
Read More22 :: How can you convert the string of any base to integer in JavaScript?
The parseInt() function is used to convert numbers between different bases. parseInt() takes the string to be converted as its first parameter, and the second parameter is the base of the given string.
In order to convert 4F (of base 16) to integer, the code used will be -
parseInt ("4F", 16);
Read MoreIn order to convert 4F (of base 16) to integer, the code used will be -
parseInt ("4F", 16);
23 :: What is the use of Void(0)?
Void(0) is used to prevent the page from refreshing and parameter "zero" is passed while calling.
Void(0) is used to call another method without refreshing the page.
Read MoreVoid(0) is used to call another method without refreshing the page.
24 :: Mention what is the disadvantage of using innerHTML in JavaScript?
If you use innerHTML in JavaScript the disadvantage is
► Content is replaced everywhere
► We cannot use like "appending to innerHTML"
► Even if you use +=like "innerHTML = innerHTML + 'html'" still the old content is replaced by html
► The entire innerHTML content is re-parsed and build into elements, therefore its much slower
► The innerHTML does not provide validation and therefore we can potentially insert valid and broken HTML in the document and break it
Read More► Content is replaced everywhere
► We cannot use like "appending to innerHTML"
► Even if you use +=like "innerHTML = innerHTML + 'html'" still the old content is replaced by html
► The entire innerHTML content is re-parsed and build into elements, therefore its much slower
► The innerHTML does not provide validation and therefore we can potentially insert valid and broken HTML in the document and break it
25 :: Which keyword is used to print the text in the screen?
document.write("Welcome") is used to print the text - Welcome in the screen.
Read More