Front End Developer (AngularJS) Question:
Download Job Interview Questions and Answers PDF
Explain me how would you organize your Javascript code?
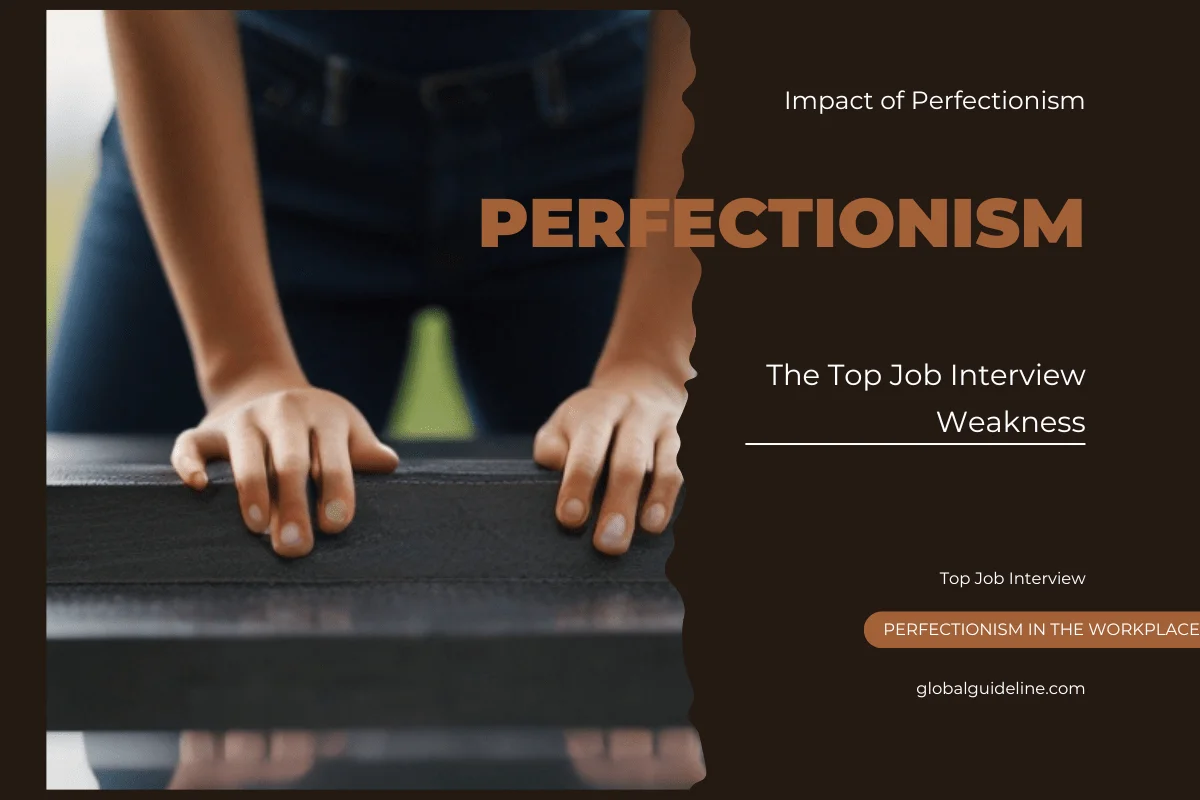
Answer:
The following pattern is the one that I personally prefer and is called 'module pattern', where we separate our javascript into logical modules, or namespaces. What you'll see below is an example of how I would separate my user module.
// Declaring my main namespace
var myapplication = myapplication || {};
// Declaring modules usermodule
myapplication.usermodule = (function() {
// createMessage: only accessible inside this module
var createMessage = function(message) {
return "Hello! " + message;
}
return {
// sayHello is a public method
sayHello: function(message) {
return createMessage(message);
}
}
})();
// Declaring another module
myapplication.adminmodule = (function(){
// your code here
})()
// This is how we call sayHello
myapplication.usermodule.sayHello("This is my module");
Some explanation on the code above
Take a look at the previous code and notice how I create my module using the notation below. It makes the function to be executed immediately because of the parenthesis at the end of the command. The result of the execution will be an object which will be set to my variable myapplication.usermodule.
...
myapplication.usermodule = (function() {
// code to be executed immediately
})();
So applying this pattern to your code you may have multiple modules and you have the control over what you want to make public and what to keep private. Besides your code will be more organized therefore easy to maintain.
// Declaring my main namespace
var myapplication = myapplication || {};
// Declaring modules usermodule
myapplication.usermodule = (function() {
// createMessage: only accessible inside this module
var createMessage = function(message) {
return "Hello! " + message;
}
return {
// sayHello is a public method
sayHello: function(message) {
return createMessage(message);
}
}
})();
// Declaring another module
myapplication.adminmodule = (function(){
// your code here
})()
// This is how we call sayHello
myapplication.usermodule.sayHello("This is my module");
Some explanation on the code above
Take a look at the previous code and notice how I create my module using the notation below. It makes the function to be executed immediately because of the parenthesis at the end of the command. The result of the execution will be an object which will be set to my variable myapplication.usermodule.
...
myapplication.usermodule = (function() {
// code to be executed immediately
})();
So applying this pattern to your code you may have multiple modules and you have the control over what you want to make public and what to keep private. Besides your code will be more organized therefore easy to maintain.
Download Front End Developer (AngularJS) Interview Questions And Answers
PDF