jQuery Tutorial plus Interview Questions And Answers
Prepare comprehensively for your jQuery interview with our extensive list of 91 questions. Our questions cover a wide range of topics in jQuery to ensure you're well-prepared. Whether you're new to the field or have years of experience, these questions are designed to help you succeed. Don't miss out on our free PDF download, containing all 91 questions to help you succeed in your jQuery interview. It's an invaluable tool for reinforcing your knowledge and building confidence.
91 jQuery Questions and Answers:
jQuery Job Interview Questions Table of Contents:
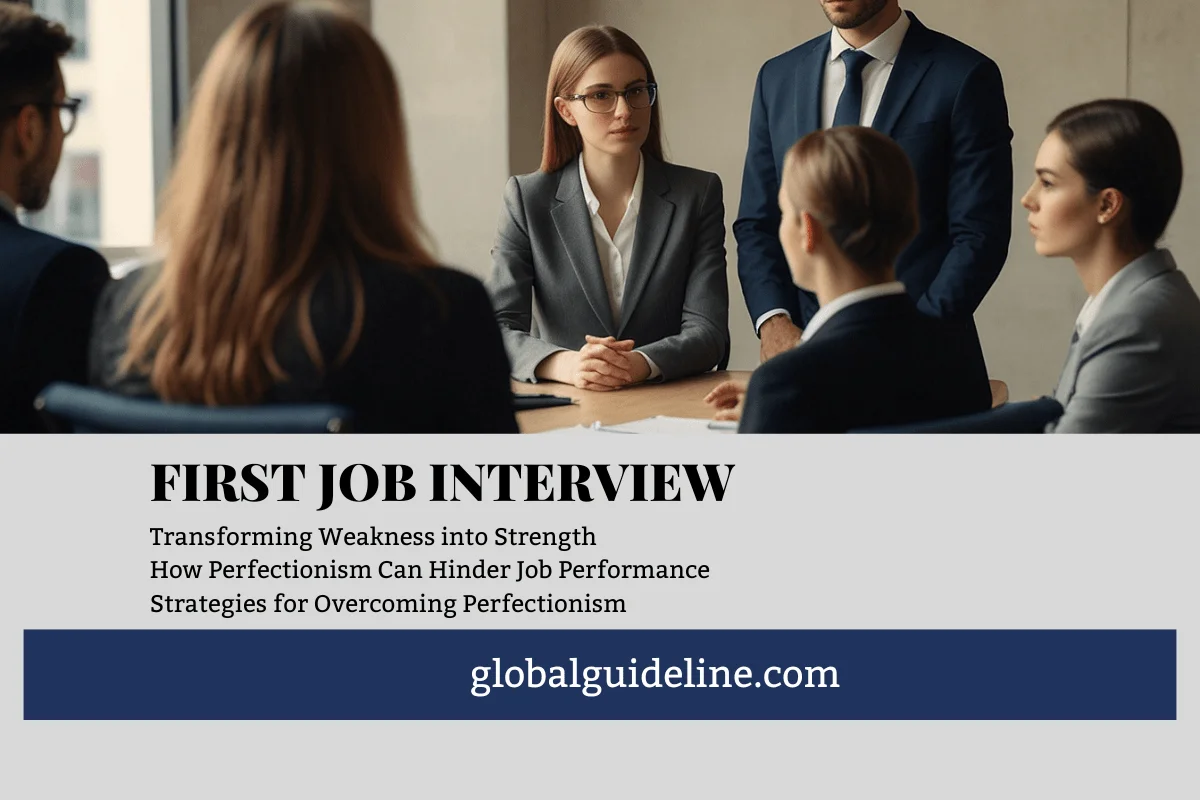
1 :: What is jQuery?
jQuery is a light weight JavaScript library which provides fast and easy way of HTML DOM traversing and manipulation,its event handling,its client side animations, etc. One of the greatest features of jQuery is that jQuery supports an efficient way to implement AJAX applications because of its light weight nature and make normalize and efficient web programs.
Read More2 :: How to use jQuery?
jQuery can be easily used with other libraries so it should work out of the box with simple and complex JavaScript and Ajax..
Read More3 :: How to use jQuery library in our ASP.Net project?
Download the latest jQuery library from jQuery.com and include the reference to the jQuery library file in our ASPX page.
<script src="_scripts/jQuery-1.2.6.js" type="text/javascript"></script>
<script language="javascript">
$(document).ready(function() {
alert('test');
});
</script>
Read More<script src="_scripts/jQuery-1.2.6.js" type="text/javascript"></script>
<script language="javascript">
$(document).ready(function() {
alert('test');
});
</script>
4 :: Can you give me a brief history of your programming days? Where did it all start?
Although it’s a bit embarrassing, my first programming experience was trying to build a customized and skinnable mediaplayer with Visual Basic 6. This probably was around 1999 or so, and although the media player was quite nice in terms of features, it had countless timers and totally killed every CPU by just playing a simple song.
After this experiment I soon realized that I wanted to do something with the internet. One of my hobbies was (and still is) japanese anime and manga, and there wasn’t really a great community around it in germany at that time, so I took the challenge and created a community called Anime-Domain as my first big web project. It was my little playground, and after 10 revisions and designs it grew so popular that it was featured in german print magazines and had some 10k unique visitors a day. This was the time where I knew that by doing web development, you could reach many people very quickly with what you do, so I decided to continue to go that route.
Read MoreAfter this experiment I soon realized that I wanted to do something with the internet. One of my hobbies was (and still is) japanese anime and manga, and there wasn’t really a great community around it in germany at that time, so I took the challenge and created a community called Anime-Domain as my first big web project. It was my little playground, and after 10 revisions and designs it grew so popular that it was featured in german print magazines and had some 10k unique visitors a day. This was the time where I knew that by doing web development, you could reach many people very quickly with what you do, so I decided to continue to go that route.
5 :: How did you become the creator of jQuery UI? Whats the story behind that?
I initially came to the jQuery project while searching for a good solution to power the web applications of a big german client. As I was specifically responsible for building out a lot of the frontend logic and interaction, I quickly found out about Interface, a collection of interface plugins developed by Stefan Petre. I soon realized there was a lot of work involved to make it stable for our environment, so I invested a lot of time into bugfixes and feature stabilization, and later even planned the next generation of Interface, Interface 2 with Stefan. However, it was then that Stefan moved on with founding his own business and ran out of time, so Interface was discontinued.
I would build the first version of an official jQuery interface addition, since I already had quite some experience from working on Interface. He and the jQuery community wanted to have it done in three months for the Ajax Experience conference in Boston, which was nearly impossible after a quick analysis of my workload. I had a day job, and I estimated I would need to work on it 3-4 hours everyday. After some days of consideration, I finally said ‘yes’, and for a three month period woke up every day at 6am to work for 3 hours on jQuery UI and then go to my day job. Now I can say that it was worth it
Read MoreI would build the first version of an official jQuery interface addition, since I already had quite some experience from working on Interface. He and the jQuery community wanted to have it done in three months for the Ajax Experience conference in Boston, which was nearly impossible after a quick analysis of my workload. I had a day job, and I estimated I would need to work on it 3-4 hours everyday. After some days of consideration, I finally said ‘yes’, and for a three month period woke up every day at 6am to work for 3 hours on jQuery UI and then go to my day job. Now I can say that it was worth it
6 :: With jQuery UI 1.7 being released in the last few days, what do you see as the key parts of jQuery UI 1.7? What are you most proud of out of that release?
The one thing I’m most proud of is that our framework has matured so much since 1.5. We have taken great care to unify our API, fix hundreds of compatibility and behavior issues, and now we truly have a foundation to build upon without needing to rewrite the core functionality again and again. This will allow us to push our features in the next releases in literally no time.
Read More7 :: What were the biggest challenges of getting the 1.7 release out there?
Our test coverage is still incomplete, and with every fixed bug, we introduced 2 others, which made the arrival at a stable level extremely difficult. Every week, there were some 50-100 bugs entered in the bugtracker, and there was literally no end. Luckily, we were able to triage the bugs to critical and blockers and solve these in time for a release. You have to have a lot of guts to push out a release that’s still imperfect - but an imperfect release is better than one that gets delayed for months. You can always roll out 1.7.1.
Read More8 :: Jumping off of jQuery UI for a second, Do you “release early, release often” with all of your projects? What are your thoughts on that strategy?
Speaking for myself, I often release too early. That has been a problem in the past, when we released versions as stable that weren’t, for instance. So it’s important to find a combination of both - a stable release must be stable, while development cannot be halted or blocked through stubborn processes. A labs section is great in that way - it allows developers to contribute freely and plan on an open canvas, with early preview releases, and the work can later be merged back.
Read More9 :: Have any of your startup projects failed dismally - if so, why and how did you learn from them?
A lot of my side projects failed, as a matter of fact, while others succeeded. Usually, the reason why some project fails is not a technical one, but the fact that one didn’t build meta data around it. That means a dedicated site, documentation, instructions.
Read More10 :: Mac, Windows or Linux? Why do you love this platform while using jQuery?
I switched to Mac hardware around a year ago and I’m totally in love with it. All components work together nicely, and so far, I never had to return my Macbook Pro to the Apple Store because of an issue. However, I’m still using Windows through Parallels because OSX, while visually nice and stable, has fundamental usability flaws.
One of these flaws is the Finder. I recently worked on the jQuery UI Selectables in the labs version, and once again saw that the Finder had great flaws when it comes down to selection. For instance, if you select multiple items and click on one of them, the multiple selection isn’t cleared. Also, my tools that I love for windows simply don’t have an alternative yet
Read MoreOne of these flaws is the Finder. I recently worked on the jQuery UI Selectables in the labs version, and once again saw that the Finder had great flaws when it comes down to selection. For instance, if you select multiple items and click on one of them, the multiple selection isn’t cleared. Also, my tools that I love for windows simply don’t have an alternative yet
11 :: What are your tools of choice to get the jQuery job done?
For editing files, I love the e texteditor (yep, the name is “e”). It basically started as a textmate clone for windows, but since then grew into something much greater. One of the features I can’t live without now is there great multiple selection support. Hold down CTRL, select a couple words through double clicking and then type over all of them. Is that cool or what? On a sidenote, on one of my talks in Japan the audience asked why I’m so insane to use a Textmate clone through an emulated Windows on OSX - I showed them this exact feature, and they were all amazed.
Other than that, I like TortoiseSVN, even if it slows down the Explorer, I love Photoshop, especially the new version with hardware acceleration, Trillian Astra for instant messaging, Gmail for email, Firefox 3 for browsing, VideoLAN for playing video, Keynote for preparing presentations, and WinSCP/Putty to do server administration. Phew, I guess that’s about it
Read MoreOther than that, I like TortoiseSVN, even if it slows down the Explorer, I love Photoshop, especially the new version with hardware acceleration, Trillian Astra for instant messaging, Gmail for email, Firefox 3 for browsing, VideoLAN for playing video, Keynote for preparing presentations, and WinSCP/Putty to do server administration. Phew, I guess that’s about it
12 :: Back onto jQuery UI… Can you see jQuery UI making more of an impression in the future, lead by the current successes of jQuery?
This question is difficult to answer because jQuery, other than jQuery UI, can be useful almost everytime. jQuery UI gives you a specific set of user interface widgets and behaviours, and many people think of it as loosing a kind of freedom. On the other hand, there’s definitely some connection - if you’re using Prototype already, and you’re looking for an UI framework, your choice is most often script.aculo.us. If you’re using jQuery, why not use the official side project?
Read More13 :: Whats next on the hitlist with jQuery UI?
There’s a lot of movement right now. We finally pushed out 1.7 3 days ago, which we believe is a solid foundation for everything that’s coming in in the next couple of months. While 1.7 was a stability and foundation release, the next releases will concentrate around features, so expect to see many more components soon. Some examples are the colorpicker, menu, grid, tooltip and tree widgets.
Additionally, my personal goal is to target more platforms, for example the iPhone. Early test implementations I did show that it’s fairly doable to support the touch events, and therefore make all jQuery UI interaction compatible with mobile devices.
Additionally, I’m working on a brand new lab section to be able to push feature development without any restrictions. This allows us to work on anything we find is cool, but maybe not on the roadmap.
Read MoreAdditionally, my personal goal is to target more platforms, for example the iPhone. Early test implementations I did show that it’s fairly doable to support the touch events, and therefore make all jQuery UI interaction compatible with mobile devices.
Additionally, I’m working on a brand new lab section to be able to push feature development without any restrictions. This allows us to work on anything we find is cool, but maybe not on the roadmap.
14 :: Is it too early to discuss jQuery UI 1.8?
There’s a lot on our list for the year. Filament Group did a great job to start a list of all widgets they could imagine being part of jQuery UI, which can be found and discussed in the jQuery UI planning wiki at http://wiki.jqueryui.com. There’s no definite roadmap for 1.8 yet, but some components are likely to make it into the next release. For instance, widgets that are already being worked on (grid, menu), as well as widgets that we had to kick out of 1.6/1.7 because they weren’t stable enough (spinner, colorpicker, autocomplete). It will be a huge feature release!
Read More15 :: And finally, If you could give one tip to any new budding jQuery UI developers, what would it be?
Think different. No, seriously. For jQuery UI, we’re trying to take the same path than jQuery, and people that are restricted to thinking in classical OOP patterns will have a problem. If you think about it freely and give the functional, event-driven and progressive approach a try, you’ll find yourself getting the work done with jQuery UI in a fraction of the time needed than with other frameworks.
Read More16 :: what is jQuery connect?
It is a jquery plugin which enables us to connect a function to another function. It is like assigning a handler for another function. This situation happens when you are using any javascript plugins and you want to execute some function when ever some function is executed from the plugin. This we can solve using jquery connect function.
Read More17 :: How to use jQuery.connect?
download jquery.connect.js file
include this file in your html file.
and use $.connect function to connect a function to another function.
Read Moreinclude this file in your html file.
and use $.connect function to connect a function to another function.
18 :: Different ways of using $.connect function in jQuery?
The syntax of connect function is
$.connect(sourceObj/*object*/, sourceFunc/*string*/, callObj/*object*/, callFunc/*string or Func*/)
sourceObj(optional) is the object of the source function to which we want to connect
sourceFunc is the function name to which we want to connect
callObj(optional) is the object which we want to use for the handler function
callFunc is the function that we want to execute when sourceFunc is executed.
Here sourceObj, callObj are optional for the global functions.
suppose if your sourceFunc is global function then no need to pass the sourceObj or you can use null or self
suppose if your callObj is global function then no need to pass the callObj or you can use null or self
ex:
// fun1, fun2 are global functions
1. $.connect('fun1',fun2)
2. $.connect(null,'fun1',fun2)
3. $.connect(self,'fun1',fun2)
4. $.connect('fun1',null,fun2)
5. $.connect('fun1',self,fun2)
6. $.connect(self,'fun1',null,fun2)
Read More$.connect(sourceObj/*object*/, sourceFunc/*string*/, callObj/*object*/, callFunc/*string or Func*/)
sourceObj(optional) is the object of the source function to which we want to connect
sourceFunc is the function name to which we want to connect
callObj(optional) is the object which we want to use for the handler function
callFunc is the function that we want to execute when sourceFunc is executed.
Here sourceObj, callObj are optional for the global functions.
suppose if your sourceFunc is global function then no need to pass the sourceObj or you can use null or self
suppose if your callObj is global function then no need to pass the callObj or you can use null or self
ex:
// fun1, fun2 are global functions
1. $.connect('fun1',fun2)
2. $.connect(null,'fun1',fun2)
3. $.connect(self,'fun1',fun2)
4. $.connect('fun1',null,fun2)
5. $.connect('fun1',self,fun2)
6. $.connect(self,'fun1',null,fun2)
19 :: Do you have plans for a new jQuery plug-in or something else we should look out for? Where to from here for the imgPreview plugin?
I'm not sure, I'm largely unpredictable when it comes to jQuery; I'm still learning! If anyone has any requests feel free to forward me them! As for the imgPreview plugin I hope to keep it under constant development; any contributions or suggestions are welcomed!
Read More20 :: Explain the features of jQuery?
Features of jQuery are :
* Effects and animations
* Ajax
* Extensibility
* DOM element selections functions
* Events
* CSS manipulation
* Utilities - such as browser version and the each function.
* JavaScript Plugins
* DOM traversal and modification
Read More* Effects and animations
* Ajax
* Extensibility
* DOM element selections functions
* Events
* CSS manipulation
* Utilities - such as browser version and the each function.
* JavaScript Plugins
* DOM traversal and modification
21 :: Explain the concepts of "$ function" in jQuery with an example?
The type of a function is "function".
There are a lot of anonymous functions is jquery.
$(document).ready(function() {});
$("a").click(function() {});
$.ajax({
url: "someurl.php",
success: function() {}
});
Read MoreThere are a lot of anonymous functions is jquery.
$(document).ready(function() {});
$("a").click(function() {});
$.ajax({
url: "someurl.php",
success: function() {}
});
22 :: Why is jQuery better than JavaScript?
* jQuery is great library for developing ajax based application.
* It helps the programmers to keep code simple and concise and reusable.
* jQuery library simplifies the process of traversal of HTML DOM tree.
* jQuery can also handle events, perform animation, and add the Ajax support in web applications.
Read More* It helps the programmers to keep code simple and concise and reusable.
* jQuery library simplifies the process of traversal of HTML DOM tree.
* jQuery can also handle events, perform animation, and add the Ajax support in web applications.
23 :: Explain how jQuery Works?
<html>
<head>
<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript">
// You can write the code here
</script>
</head>
<body>
<a href="http://www.globalguideline.com/">Global GuideLine</a>
</body>
</html>
Read More<head>
<script type="text/javascript" src="jquery.js"></script>
<script type="text/javascript">
// You can write the code here
</script>
</head>
<body>
<a href="http://www.globalguideline.com/">Global GuideLine</a>
</body>
</html>
24 :: When can you use jQuery?
JQuery can be used to
apply CSS
call functions on events
traverse the documents
manipulation purpose and
to add effects too.
Read Moreapply CSS
call functions on events
traverse the documents
manipulation purpose and
to add effects too.
25 :: Advantages of jQuery.
The advantages of using jQuery are:
* JavaScript enhancement without the overhead of learning new syntax
* Ability to keep the code simple, clear, readable and reusable
* Eradication of the requirement of writing repetitious and complex loops and DOM scripting library calls
Read More* JavaScript enhancement without the overhead of learning new syntax
* Ability to keep the code simple, clear, readable and reusable
* Eradication of the requirement of writing repetitious and complex loops and DOM scripting library calls