Operational Debugging Interview Preparation Guide Download PDF
Linux Debugging frequently Asked Questions by expert members with experience in Debugging. These questions and answers will help you strengthen your technical skills, prepare for the new job test and quickly revise the concepts
80 Linux Debugging Questions and Answers:
Table of Contents:
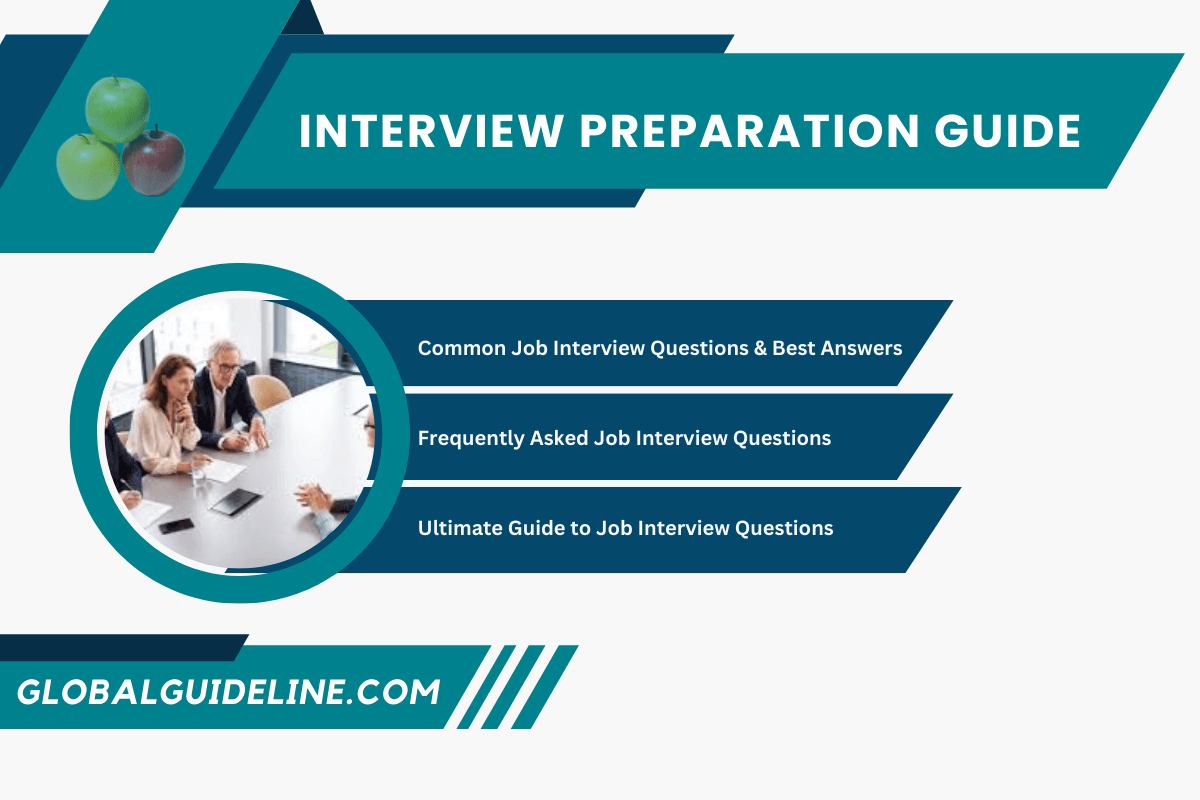
1 :: Which one of the following command saves the command history of GDB in a file?
a) history
b) set history
c) set history save on
d) none of the mentioned
c) set history save on
3 :: The command "show commands" of GDB
a) displays the last 10 commands in the command history
b) displays all commands of the command history
c) displays all the commands available in GDB
d) none of the mentioned
a) displays the last 10 commands in the command history
4 :: The GDB command "show output-radix"
a) sets the default base for numeric display
b) displays the current default base for numeric display
c) both (a) and (b)
d) none of the mentioned
b) displays the current default base for numeric display
13 :: Which one of the following variables is used within GDB to hold on to a value and refer to it later?
a) convenience variables
b) environment variables
c) temporary variables
d) none of the mentioned
a) convenience variables
18 :: Inside GDB, a program may stop because of
a) a signal
b) a breakpoint
c) step command
d) all of the mentioned
d) all of the mentioned
20 :: What is the output of this program no 16?
#include<stdio.h>
#include<sys/types.h>
#include<netinet/in.h>
#include<sys/socket.h>
#include<errno.h>
int main()
{
struct sockaddr_in addr;
int fd;
fd = socket(AF_UNIX,SOCK_STREAM,0);
if (fd == -1)
perror("socket");
addr.sun_family = AF_UNIX;
strcpy(addr.sun_path,"san_sock");
if (bind(4,(struct sockaddr*)&addr,sizeof(addr)) == -1)
printf("Sanfoudnryn");
return 0;
}
a) error
b) "google"
c) segmentation fault
d) none of the mentioned
a) error
Explanation:
The structure used for AF_UNIX if sockaddr_un.
Output:
[root@localhost google]# gcc -o san san.c
san.c: In function 'main':
san.c:14:6: error: 'struct sockaddr_in' has no member named 'sun_family'
san.c:15:2: warning: incompatible implicit declaration of built-in function 'strcpy' [enabled by default]
san.c:15:13: error: 'struct sockaddr_in' has no member named 'sun_path'
[root@localhost google]#
Explanation:
The structure used for AF_UNIX if sockaddr_un.
Output:
[root@localhost google]# gcc -o san san.c
san.c: In function 'main':
san.c:14:6: error: 'struct sockaddr_in' has no member named 'sun_family'
san.c:15:2: warning: incompatible implicit declaration of built-in function 'strcpy' [enabled by default]
san.c:15:13: error: 'struct sockaddr_in' has no member named 'sun_path'
[root@localhost google]#
21 :: What is the output of this program no 15?
#include<stdio.h>
#include<sys/types.h>
#include<sys/un.h>
#include<sys/socket.h>
int main()
{
struct sockaddr_un add_server, add_client;
int fd_server, fd_client;
int len;
char ch;
fd_server = socket(AF_UNIX,SOCK_STREAM,0);
if(fd_server == -1)
perror("socket");
add_server.sun_family = AF_UNIX;
strcpy(add_server.sun_path,"san_sock");
if( bind(fd_server,(struct sockaddr*)&add_server,sizeof(add_server)) != 0)
perror("bind");
len = sizeof(add_client);
fd_client = connect(fd_server,(struct sockaddr*)&add_client,&len);
printf("googlen");
return 0;
}
a) this program will print the string "google"
b) segmentation fault
c) error
d) none of the mentioned
c) error
Explanation:
The syntax of the connect() is wrong. connect() should be used in client program only.
Ouptut:
[root@localhost google]# gcc -o san san.c
san.c: In function 'main':
san.c:20:46: warning: passing argument 3 of 'connect' makes integer from pointer without a cast [enabled by default]
/usr/include/sys/socket.h:129:12: note: expected 'socklen_t' but argument is of type 'int *'
[root@localhost google]#
Explanation:
The syntax of the connect() is wrong. connect() should be used in client program only.
Ouptut:
[root@localhost google]# gcc -o san san.c
san.c: In function 'main':
san.c:20:46: warning: passing argument 3 of 'connect' makes integer from pointer without a cast [enabled by default]
/usr/include/sys/socket.h:129:12: note: expected 'socklen_t' but argument is of type 'int *'
[root@localhost google]#
23 :: What is the output of this program no 14?
#include<stdio.h>
#include<sys/types.h>
#include<sys/un.h>
#include<sys/socket.h>
#include<errno.h>
int main()
{
struct sockaddr_un addr;
int fd;
fd = socket(AF_UNIX,SOCK_STREAM,0);
if (fd == -1)
perror("socket");
addr.sun_family = AF_UNIX;
strcpy(addr.sun_path,"san_sock");
if (bind(4,(struct sockaddr*)&addr,sizeof(addr)) == -1)
printf("Sanfoudnryn");
return 0;
}
a) this program will print the string "google"
b) this program will not print the string "google"
c) segmentation fault
d) none of the mentioned
a) this program will print the string "google"
Explanation:
The first argument of the bind() is not a valid file descriptor in this program.
Output:
[root@localhost google]# gcc -o san san.c
[root@localhost google]# ./san
Sanfoudnry
[root@localhost google]#
Explanation:
The first argument of the bind() is not a valid file descriptor in this program.
Output:
[root@localhost google]# gcc -o san san.c
[root@localhost google]# ./san
Sanfoudnry
[root@localhost google]#
24 :: What is the output of this program?
#include<stdio.h>
#include<sys/types.h>
#include<sys/socket.h>
int main()
{
int fd;
fd = socket(AF_UNIX,SOCK_STREAM,0);
printf("%dn",fd);
return 0;
}
a) 0
b) 1
c) 2
d) 3
d) 3
Explanation:
The socket() returns the lowest available file descriptor and in this program i.e. 3.
Output:
[root@localhost google]# gcc -o san san.c
[root@localhost google]# ./san
3
[root@localhost google]#
Explanation:
The socket() returns the lowest available file descriptor and in this program i.e. 3.
Output:
[root@localhost google]# gcc -o san san.c
[root@localhost google]# ./san
3
[root@localhost google]#
25 :: What is the output of the program no 13?
#include<stdio.h>
#include<sys/types.h>
#include<sys/un.h>
#include<sys/socket.h>
int main()
{
struct sockaddr_un add_server, add_client;
int fd_server, fd_client;
int len;
char ch;
fd_server = socket(AF_UNIX,SOCK_STREAM,0);
if(fd_server == -1)
perror("socket");
add_server.sun_family = AF_UNIX;
strcpy(add_server.sun_path,"san_sock");
if( bind(fd_server,(struct sockaddr*)&add_server,sizeof(add_server)) != 0)
perror("bind");
if( listen(fd_server,3) != 0)
perror("listen");
len = sizeof(add_client);
fd_client = accept(fd_server,(struct sockaddr*)&add_client,&len);
printf("googlen");
return 0;
}
a) the program will print the string "google"
b) the process will remain block
c) segmentation fault
d) none of the mentioned
b) the process will remain block
Explanation:
There is no peding request in the queue for listening socket "san_sock".
Output:
[root@localhost google]# gcc -o san san.c
[root@localhost google]# ./san
^Z
[4]+ Stopped ./san
[root@localhost google]#
Explanation:
There is no peding request in the queue for listening socket "san_sock".
Output:
[root@localhost google]# gcc -o san san.c
[root@localhost google]# ./san
^Z
[4]+ Stopped ./san
[root@localhost google]#
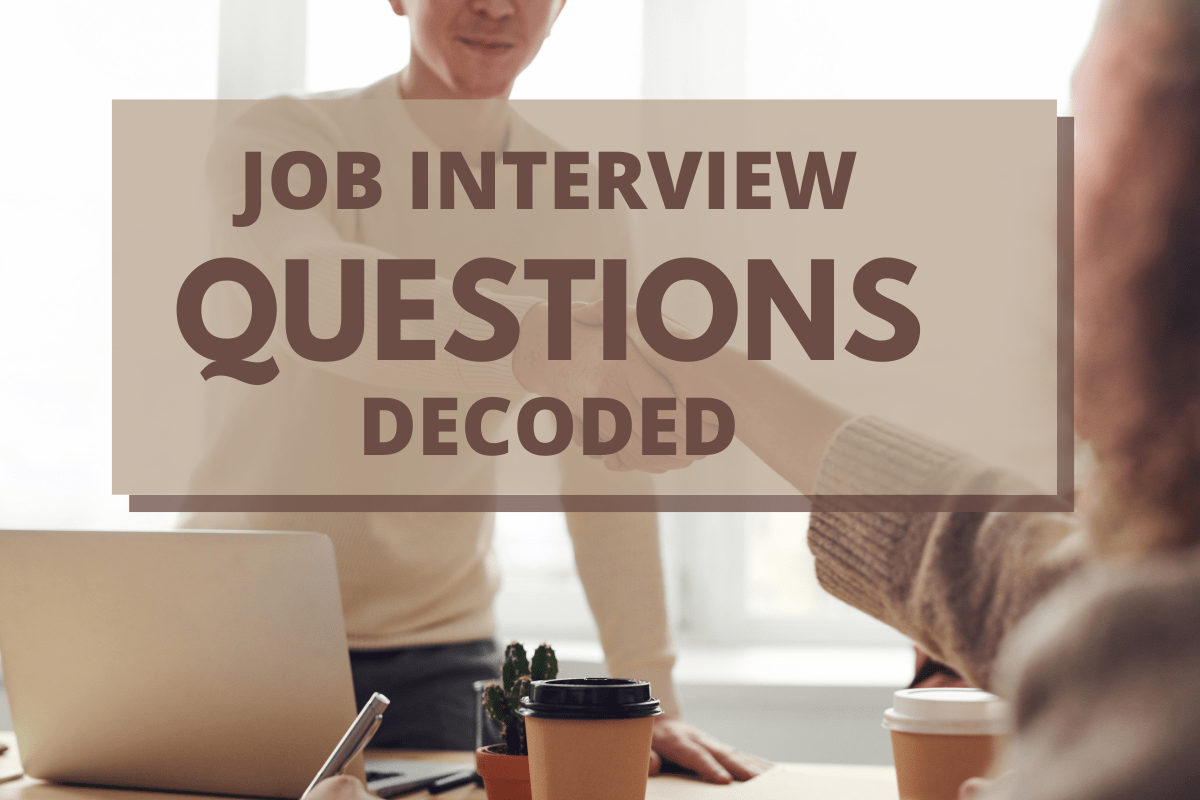