Debugging Question: Download Questions PDF
Which one of the following statement is not true about this program?
#include<stdio.h>
#include<pthread.h>
void *fun_t(void *arg);
void *fun_t(void *arg)
{
printf("%dn",getpid());
pthread_exit("Bye");
}
int main()
{
pthread_t pt;
void *res_t;
if(pthread_create(&pt,NULL,fun_t,NULL) != 0)
perror("pthread_create");
if(pthread_join(pt,&res_t) != 0)
perror("pthread_join");
printf("%dn",getpid());
return 0;
}
a) both printf statements will print the same value
b) both printf statements will print the different values
c) this program will print nothing
d) none of the mentioned
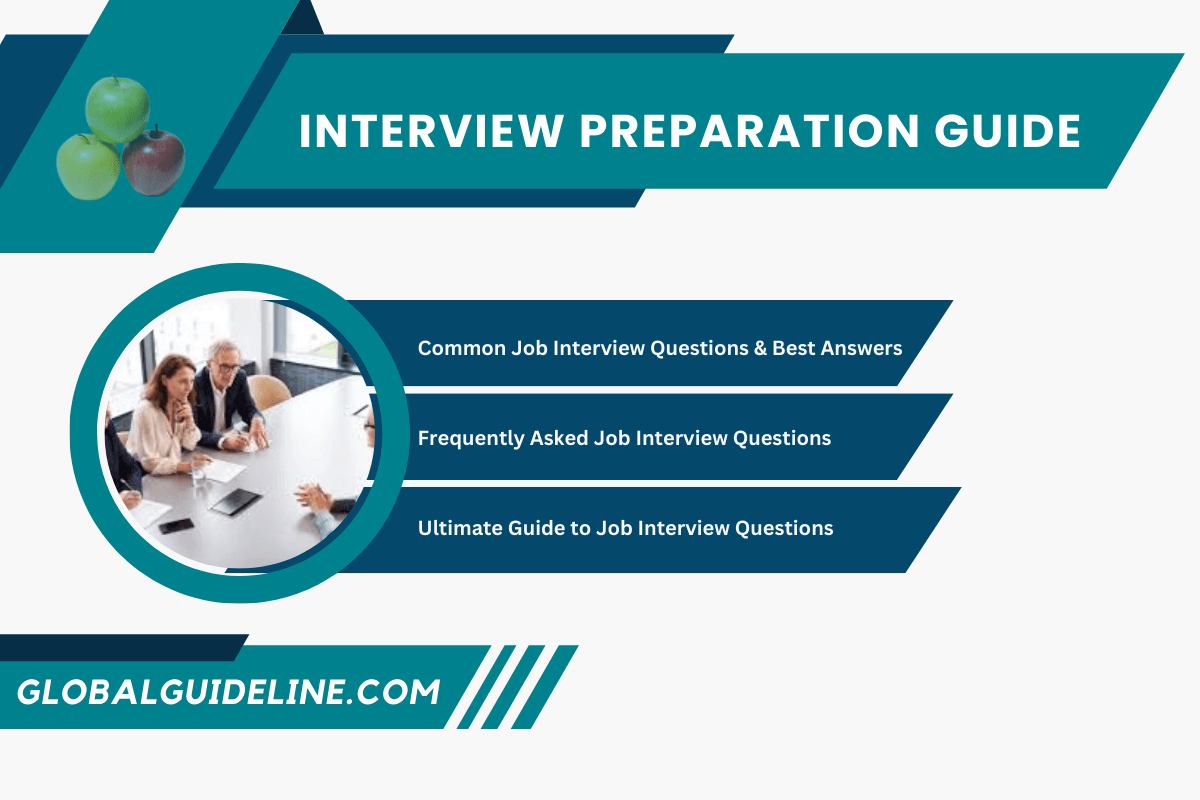
Answer:
a) both printf statements will print the same value
Explanation:
All the threads of the same process have same PID.
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
12981
12981
[root@localhost google]#
Explanation:
All the threads of the same process have same PID.
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
12981
12981
[root@localhost google]#
Download Linux Debugging Interview Questions And Answers
PDF