MySQL Programming Interview Questions And Answers
Prepare comprehensively for your MySQL Programming interview with our extensive list of 110 questions. Each question is crafted to challenge your understanding and proficiency in MySQL Programming. Suitable for all skill levels, these questions are essential for effective preparation. Access the free PDF to get all 110 questions and give yourself the best chance of acing your MySQL Programming interview. This resource is perfect for thorough preparation and confidence building.
110 MySQL Programming Questions and Answers:
MySQL Programming Job Interview Questions Table of Contents:
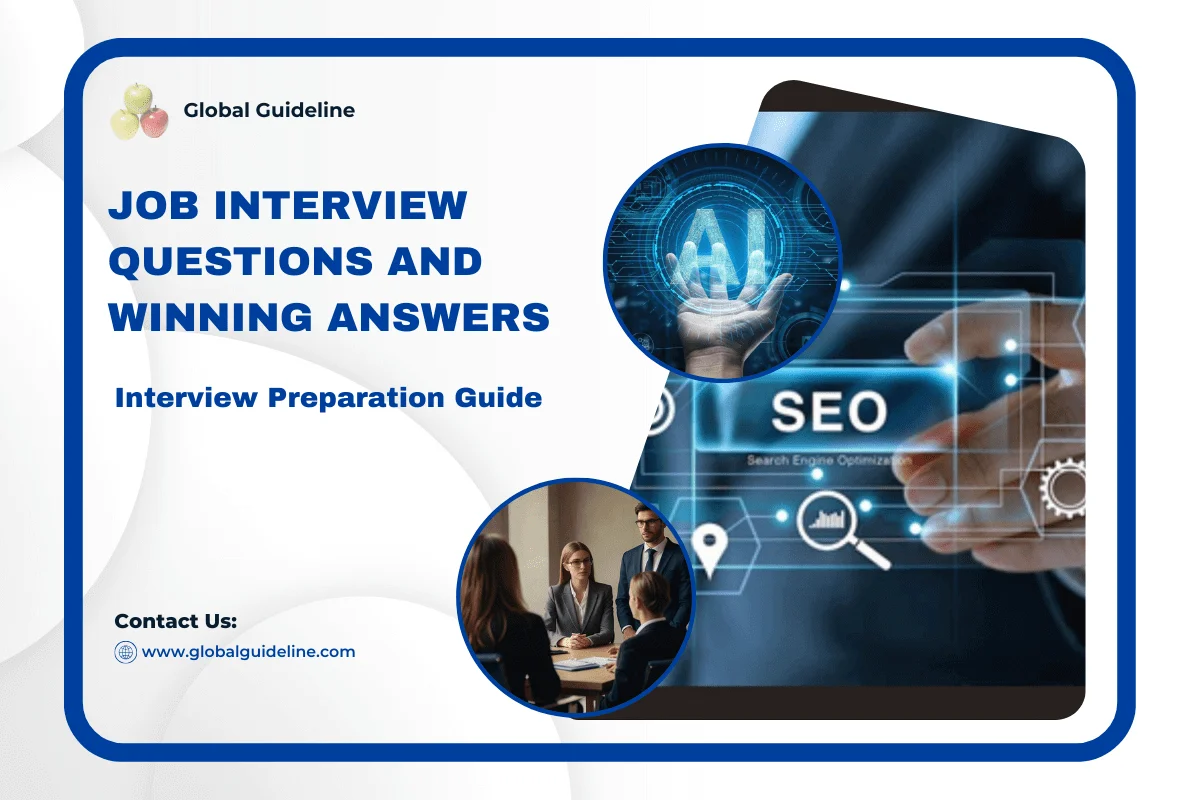
1 :: What Is MySQL?
MySQL is an open source database management system developed by MySQL AB, http://www.mysql.com.
Read More2 :: What Is mSQL?
Mini SQL (mSQL) is a light weight relational database management system capable of providing rapid access to your data with very little overhead. mSQL is developed by Hughes Technologies Pty Ltd.
MySQL was started from mSQL and shared the same API.
Read MoreMySQL was started from mSQL and shared the same API.
3 :: What Is SQL?
SQL, SEQUEL (Structured English Query Language), is a language for RDBMS (Relational Database Management Systems). SQL was developed by IBM Corporation.
Read More6 :: What Is Row?
A row is a unit of data with related data items stored as one item in one column in a table.
Read More7 :: What Is Primary Key?
A primary key is a single column or multiple columns defined to have unique values that can be used as row identifications.
Read More8 :: What Is Foreign Key?
A foreign key is a single column or multiple columns defined to have values that can be mapped to a primary key in another table.
Read More9 :: What Is Index?
An index is a single column or multiple columns defined to have values pre-sorted to speed up data retrieval speed.
Read More11 :: What Is Join?
Join is data retrieval operation that combines rows from multiple tables under certain matching conditions to form a single row.
Read More12 :: What Is Union?
Join is data retrieval operation that combines multiple query outputs of the same structure into a single output.
Read More13 :: What Is ISAM?
ISAM (Indexed Sequential Access Method) was developed by IBM to store and retrieve data on secondary storage systems like tapes.
Read More14 :: What Is MyISAM?
MyISAM is a storage engine used as the default storage engine for MySQL database. MyISAM is based on the ISAM (Indexed Sequential Access Method) concept and offers fast data storage and retrieval. But it is not transaction safe.
Read More15 :: What Is InnoDB?
InnoDB is a transaction safe storage engine developed by Innobase Oy (an Oracle company now).
Read More16 :: What Is BDB (BerkeleyDB)?
BDB (BerkeleyDB) is transaction safe storage engine originally developed at U.C. Berkeley. It is now developed by Sleepycat Software, Inc. (an Oracle company now).
Read More17 :: What Is CSV?
CSV (Comma Separated Values) is a file format used to store database table contents, where one table row is stored as one line in the file, and each data field is separated with comma.
Read More18 :: What Is Transaction?
A transaction is a logical unit of work requested by a user to be applied to the database objects. MySQL server introduces the transaction concept to allow users to group one or more SQL statements into a single transaction, so that the effects of all the SQL statements in a transaction can be either all committed (applied to the database) or all rolled back (undone from the database).
Read More19 :: What Is Commit?
Commit is a way to terminate a transaction with all database changes to be saved permanently to the database server.
Read More20 :: What Is Rollback?
Rollback is a way to terminate a transaction with all database changes not saving to the database server.
Read More21 :: Explain what Is MySQL?
MySQL is an open source database management system developed by MySQL AB, http://www.mysql.com. MySQL has the following main features:
* Works on many different platforms.
* APIs for C, C++, Eiffel, Java, Perl, PHP, Python, Ruby, and Tcl are available.
* Fully multi-threaded using kernel threads. It can easily use multiple CPUs if they are available.
* Provides transactional and non-transactional storage engines.
* Uses very fast B-tree disk tables (MyISAM) with index compression.
* A very fast thread-based memory allocation system.
* Very fast joins using an optimized one-sweep multi-join.
* In-memory hash tables, which are used as temporary tables.
* SQL functions are implemented using a highly optimized class library and should be as fast as possible. Usually there is no memory allocation at all after query initialization.
* The server is available as a separate program for use in a client/server networked environment. It is also available as a library that can be embedded (linked) into standalone applications. Such applications can be used in isolation or in environments where no network is available.
Read More* Works on many different platforms.
* APIs for C, C++, Eiffel, Java, Perl, PHP, Python, Ruby, and Tcl are available.
* Fully multi-threaded using kernel threads. It can easily use multiple CPUs if they are available.
* Provides transactional and non-transactional storage engines.
* Uses very fast B-tree disk tables (MyISAM) with index compression.
* A very fast thread-based memory allocation system.
* Very fast joins using an optimized one-sweep multi-join.
* In-memory hash tables, which are used as temporary tables.
* SQL functions are implemented using a highly optimized class library and should be as fast as possible. Usually there is no memory allocation at all after query initialization.
* The server is available as a separate program for use in a client/server networked environment. It is also available as a library that can be embedded (linked) into standalone applications. Such applications can be used in isolation or in environments where no network is available.
22 :: How To Install MySQL?
MySQL is an open source database management system developed by MySQL AB, http://www.mysql.com. You can download a copy and install it on your local computer very easily. Here is how you can do this:
* Go to http://dev.mysql.com/downloads/mysql/5.0.html.
* Select the "Windows" and "Without installer" version.
* Find a mirror site and download "mysql-noinstall-5.0.24-win32.zip".
* Unzip the file, you will get a new sub-directory, ".mysql-5.0.24-win32".
* Move and rename this sub-directory to mysql.
* The installation is done and your MySQL server is ready.
Read More* Go to http://dev.mysql.com/downloads/mysql/5.0.html.
* Select the "Windows" and "Without installer" version.
* Find a mirror site and download "mysql-noinstall-5.0.24-win32.zip".
* Unzip the file, you will get a new sub-directory, ".mysql-5.0.24-win32".
* Move and rename this sub-directory to mysql.
* The installation is done and your MySQL server is ready.
23 :: How To Start MySQL Server?
If you want to start the MySQL server, you can run the "mysqld" program in a command window as shown in the following tutorial:
>cd mysqlin
>mysqld
"mysqld" will run quietly without printing any message in you command window. So you will see nothing after entering the "mysqld" command. You should trust "mysqld" and believe that MySQL server is running ok on your local computer now.
Another way to start the MySQL server is double-click mysqlinmysqld.exe on your file explorer window.
Read More>cd mysqlin
>mysqld
"mysqld" will run quietly without printing any message in you command window. So you will see nothing after entering the "mysqld" command. You should trust "mysqld" and believe that MySQL server is running ok on your local computer now.
Another way to start the MySQL server is double-click mysqlinmysqld.exe on your file explorer window.
24 :: How Do You Know If Your MySQL Server Is Alive?
If you want to know whether your MySQL server is alive, you can use the "mysqladmin" program in a command window as shown in the following tutorial:
>cd mysqlin
>mysqladmin -u root ping
mysqld is alive
The "mysqld is alive" message tells you that your MySQL server is running ok. If your MySQL server is not running, you will get a "connect ... failed" message.
Read More>cd mysqlin
>mysqladmin -u root ping
mysqld is alive
The "mysqld is alive" message tells you that your MySQL server is running ok. If your MySQL server is not running, you will get a "connect ... failed" message.
25 :: How Do You Know the Version of Your MySQL Server?
If you want to know the version number of your MySQL server, you can use the "mysqladmin" program in a command window as shown in the following tutorial:
>cd mysqlin
>mysqladmin -u root version
mysqladmin Ver 8.41 Distrib 5.0.24, for Win32 on ia32
Copyright (C) 2000 MySQL AB & MySQL Finland AB
& TCX DataKonsult AB
...
<pre>Server version 5.0.24-community
Protocol version 10
Connection localhost via TCP/IP
TCP port 3306
Uptime: 25 min 9 sec</pre>
Threads: 1 Questions: 2 Slow queries: 0 Opens: 12
Flush tables: 1 Open tables: 6
Queries per second avg: 0.001
The output in the above example tells you that the version number is 5.0.24.
Read More>cd mysqlin
>mysqladmin -u root version
mysqladmin Ver 8.41 Distrib 5.0.24, for Win32 on ia32
Copyright (C) 2000 MySQL AB & MySQL Finland AB
& TCX DataKonsult AB
...
<pre>Server version 5.0.24-community
Protocol version 10
Connection localhost via TCP/IP
TCP port 3306
Uptime: 25 min 9 sec</pre>
Threads: 1 Questions: 2 Slow queries: 0 Opens: 12
Flush tables: 1 Open tables: 6
Queries per second avg: 0.001
The output in the above example tells you that the version number is 5.0.24.