JUnit Interview Questions And Answers
Prepare comprehensively for your JUnit interview with our extensive list of 45 questions. These questions are specifically selected to challenge and enhance your knowledge in JUnit. Perfect for all proficiency levels, they are key to your interview success. Secure the free PDF to access all 45 questions and guarantee your preparation for your JUnit interview. This guide is crucial for enhancing your readiness and self-assurance.
45 JUnit Questions and Answers:
JUnit Job Interview Questions Table of Contents:
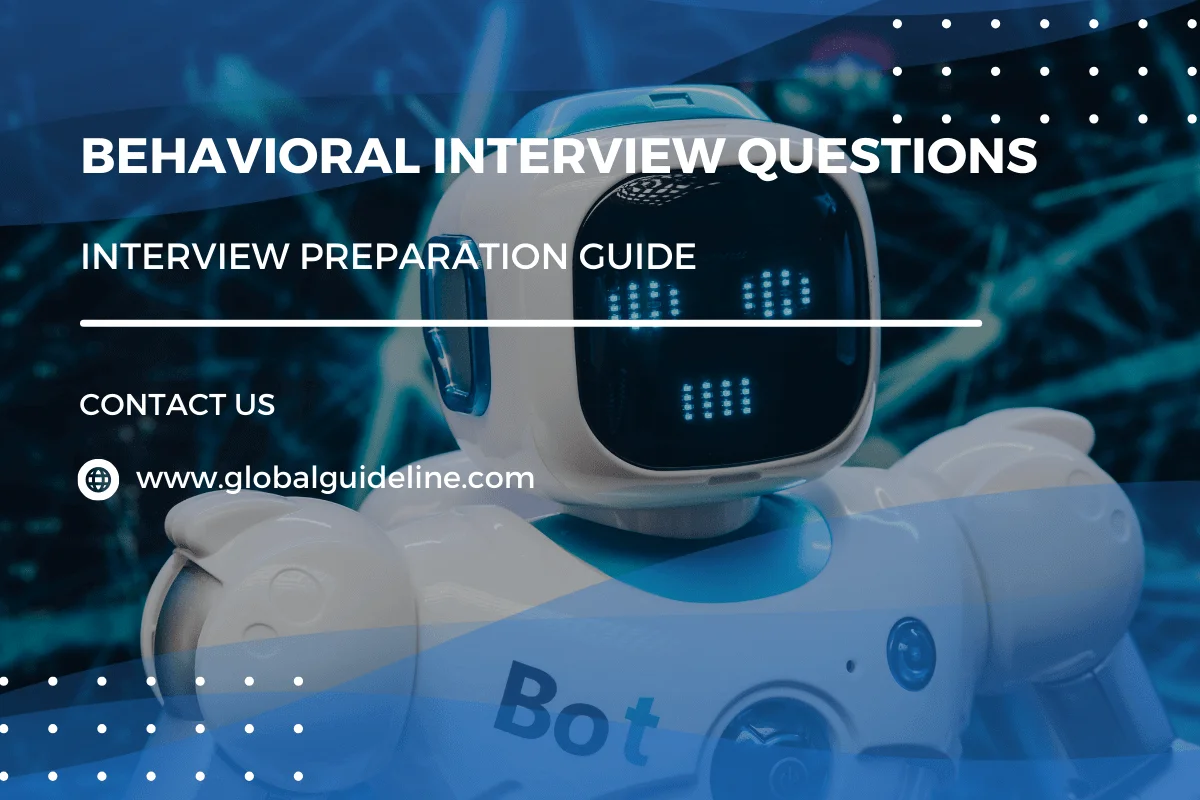
1 :: What Is JUnit?
What is JUnit? You need to remember the following points when answering this question:
► It is a software testing framework to for unit testing.
► It is written in Java and designed to test Java applications.
► It is an Open Source Software maintained by the JUnit.org community.
Answer from the JUnit FAQ:
JUnit is a simple, open source framework to write and run repeatable tests. It is an instance of the xUnit architecture for unit testing frameworks. JUnit features include:
► Assertions for testing expected results
► Test fixtures for sharing common test data
► Test runners for running tests
JUnit was originally written by Erich Gamma and Kent Beck.
Read More► It is a software testing framework to for unit testing.
► It is written in Java and designed to test Java applications.
► It is an Open Source Software maintained by the JUnit.org community.
Answer from the JUnit FAQ:
JUnit is a simple, open source framework to write and run repeatable tests. It is an instance of the xUnit architecture for unit testing frameworks. JUnit features include:
► Assertions for testing expected results
► Test fixtures for sharing common test data
► Test runners for running tests
JUnit was originally written by Erich Gamma and Kent Beck.
2 :: Where Do You Download JUnit?
Where do I download JUnit? I don't think anyone will ask this question in a job interview. But the answer is simple. You should follow the download instructions from the JUnit official Website: JUnit.org.
Read More3 :: Who Should Use JUnit, Developers or Testers?
I should say that JUnit is mostly used by developers. JUnit is designed for unit testing, which is really a coding process, not a testing process.
But many testers or QA engineers, are also required to use JUnit for unit testing. For example, I found this job title on the Internet: Lead QA Engineer - Java / J2EE / whitebox / SAP / Junit
Read MoreBut many testers or QA engineers, are also required to use JUnit for unit testing. For example, I found this job title on the Internet: Lead QA Engineer - Java / J2EE / whitebox / SAP / Junit
4 :: Why Do You Use JUnit to Test Your Code?
This is a commonly asked question in a job interview. Your answer should have these points:
► I believe that writing more tests will make me more productive, not less productive.
► I believe that tests should be done as soon as possible at the code unit level.
► I believe that using JUnit makes unit testing easier and faster.
Read More► I believe that writing more tests will make me more productive, not less productive.
► I believe that tests should be done as soon as possible at the code unit level.
► I believe that using JUnit makes unit testing easier and faster.
5 :: How Do You Install JUnit?
How do I install JUnit? First I will download the lastest version of JUnit.
Then I will extract all files from the downloaded file.
The most important file should be the JUnit JAR file: junit-4.4.jar, which contains all JUnit class packages.
To verify junit-4.4.jar, I will run the org.junit.runner.JUnitCore class:
java -cp junit-4.4.jar org.junit.runner.JUnitCore
JUnit version 4.4
Time: 0
OK (0 tests)
Read MoreThen I will extract all files from the downloaded file.
The most important file should be the JUnit JAR file: junit-4.4.jar, which contains all JUnit class packages.
To verify junit-4.4.jar, I will run the org.junit.runner.JUnitCore class:
java -cp junit-4.4.jar org.junit.runner.JUnitCore
JUnit version 4.4
Time: 0
OK (0 tests)
6 :: How To Wirte a Simple JUnit Test Class?
This is a common test in a job interview. You should be able to write this simple test class with one test method:
import org.junit.*;
public class HelloTest {
@Test public void testHello() {
String message = "Hello World!";
Assert.assertEquals(12, message.length());
}
}
Read Moreimport org.junit.*;
public class HelloTest {
@Test public void testHello() {
String message = "Hello World!";
Assert.assertEquals(12, message.length());
}
}
7 :: How To Compile a JUnit Test Class?
Compiling a JUnit test class is like compiling any other Java classes. The only thing you need watch out is that the JUnit JAR file must be included in the classpath. For example, to compile the test class HelloTest.java described previously, you should do this:
javac -cp junit-4.4.jar HelloTest.java
dir HelloTest.*
453 HelloTest.class
183 HelloTest.java
The compilation is ok, if you see the HelloTest.class file.
Read Morejavac -cp junit-4.4.jar HelloTest.java
dir HelloTest.*
453 HelloTest.class
183 HelloTest.java
The compilation is ok, if you see the HelloTest.class file.
8 :: How To Run a JUnit Test Class?
A JUnit test class usually contains a number of test methods. You can run all test methods in a JUnit test class with the JUnitCore runner class. For example, to run the test class HelloTest.java described previously, you should do this:
java -cp .;
junit-4.4.jar org.junit.runner.JUnitCore HelloTest
JUnit version 4.4
.
Time: 0.015
OK (1 test)
This output says that 1 tests performed and passed.
Read Morejava -cp .;
junit-4.4.jar org.junit.runner.JUnitCore HelloTest
JUnit version 4.4
.
Time: 0.015
OK (1 test)
This output says that 1 tests performed and passed.
9 :: What CLASSPATH Settings Are Needed to Run JUnit?
It doesn't matter if you run your JUnit tests from a command line, from an IDE, or from "ant", you must define your CLASSPATH settings correctly. Here is what recommended by the JUnit FAQ with some minor changes:
To run your JUnit tests, you'll need the following elemements in your CLASSPATH:
* The JUnit JAR file.
* Location of your JUnit test classes.
* Location of classes to be tested.
* JAR files of class libraries that are required by classes to be tested.
If attempting to run your tests results in a NoClassDefFoundError, then something is missing from your CLASSPATH.
If you are running your JUnit tests from a command line on a Windows system:
set CLASSPATH=c:Ajunit-4.4.jar;c:Btest_classes;
c:Btarget_classes;c:D3rd_party.jar
If you are running your JUnit tests from a command line on a Unix (bash) system:
export CLASSPATH=/A/junit-4.4.jar:/B/test_classes:
/C/target_classes:/D/3rd_party.jar
Read MoreTo run your JUnit tests, you'll need the following elemements in your CLASSPATH:
* The JUnit JAR file.
* Location of your JUnit test classes.
* Location of classes to be tested.
* JAR files of class libraries that are required by classes to be tested.
If attempting to run your tests results in a NoClassDefFoundError, then something is missing from your CLASSPATH.
If you are running your JUnit tests from a command line on a Windows system:
set CLASSPATH=c:Ajunit-4.4.jar;c:Btest_classes;
c:Btarget_classes;c:D3rd_party.jar
If you are running your JUnit tests from a command line on a Unix (bash) system:
export CLASSPATH=/A/junit-4.4.jar:/B/test_classes:
/C/target_classes:/D/3rd_party.jar
10 :: How Do I Run JUnit Tests from Command Window?
To run JUnit tests from a command window, you need to check the following list:
1. Make sure that JDK is installed and the "java" command program is accessible through the PATH setting. Type "java -version" at the command prompt, you should see the JVM reports you back the version string.
2. Make sure that the CLASSPATH is defined as shown in the previous question.
3. Invoke the JUnit runner by entering the following command:
java org.junit.runner.JUnitCore <test class name>
Read More1. Make sure that JDK is installed and the "java" command program is accessible through the PATH setting. Type "java -version" at the command prompt, you should see the JVM reports you back the version string.
2. Make sure that the CLASSPATH is defined as shown in the previous question.
3. Invoke the JUnit runner by entering the following command:
java org.junit.runner.JUnitCore <test class name>
11 :: How Do You Uninstall JUnit?
Uninstalling JUnit is easy. Just remember these:
* Delete the directory that contains the JUnit JAR file and other JUnit files.
* Remove the JUnit JAR file from the CLASSPATH environment variable.
* No need to stop any background processes, because JUnit does not use background process.
* No need to remove any registry settings, because JUnit does not use Windows registry.
Read More* Delete the directory that contains the JUnit JAR file and other JUnit files.
* Remove the JUnit JAR file from the CLASSPATH environment variable.
* No need to stop any background processes, because JUnit does not use background process.
* No need to remove any registry settings, because JUnit does not use Windows registry.
12 :: How To Write a JUnit Test Method?
This interview question is to check if you know the basic rules about writing a JUnit test method:
* You need to mark the method as a JUnit test method with the JUnit annotation: @org.junit.Test.
* A JUnit test method must be a "public" method. This allows the runner class to access this method.
* A JUnit test method must be a "void" method. The runner class does not check any return values.
* A JUnit test should perform one JUnit assertion - calling an org.junit.Assert.assertXXX() method.
Here is a simple JUnit test method:
import org.junit.*;
...
@Test public void testHello() {
String message = "Hello World!";
Assert.assertEquals(12, message.length());
}
Read More* You need to mark the method as a JUnit test method with the JUnit annotation: @org.junit.Test.
* A JUnit test method must be a "public" method. This allows the runner class to access this method.
* A JUnit test method must be a "void" method. The runner class does not check any return values.
* A JUnit test should perform one JUnit assertion - calling an org.junit.Assert.assertXXX() method.
Here is a simple JUnit test method:
import org.junit.*;
...
@Test public void testHello() {
String message = "Hello World!";
Assert.assertEquals(12, message.length());
}
13 :: Can You Provide a List of Assertion Methods Supported by JUnit 4.4?
You should be able to answer this question by looking up the org.junit.Assert class API document. Here is a list of assertino methods supported by JUnit 4.4:
* assertEquals(expected, actual)
* assertEquals(message, expected, actual)
* assertEquals(expected, actual, delta)
* assertEquals(message, expected, actual, delta)
* assertFalse(condition)
* assertFalse(message, condition)
* assertNotNull(object)
* assertNotNull(message, object)
* assertNotSame(expected, actual)
* assertNotSame(message, expected, actual)
* assertNull(object)
* assertNull(message, object)
* assertSame(expected, actual)
* assertSame(message, expected, actual)
* assertTrue(condition)
* assertTrue(message, condition)
* fail()
* fail(message)
* failNotEquals(message, expected, actual)
* failNotSame(message, expected, actual)
* failSame(message)
Read More* assertEquals(expected, actual)
* assertEquals(message, expected, actual)
* assertEquals(expected, actual, delta)
* assertEquals(message, expected, actual, delta)
* assertFalse(condition)
* assertFalse(message, condition)
* assertNotNull(object)
* assertNotNull(message, object)
* assertNotSame(expected, actual)
* assertNotSame(message, expected, actual)
* assertNull(object)
* assertNull(message, object)
* assertSame(expected, actual)
* assertSame(message, expected, actual)
* assertTrue(condition)
* assertTrue(message, condition)
* fail()
* fail(message)
* failNotEquals(message, expected, actual)
* failNotSame(message, expected, actual)
* failSame(message)
14 :: Why Does Poeple Import org.junit.Assert Statically?
Poeple use the static import statement on org.junit.Assert to save coding time on calling its assetion methods. With a normal import statement, assertion method names must qualified with the class name like this:
import org.junit.Assert;
...
Assert.assertEquals(12, message.length());
With a static import statement, assertion method names can be used directly like this:
import static org.junit.Assert.*;
...
assertEquals(12, message.length());
Read Moreimport org.junit.Assert;
...
Assert.assertEquals(12, message.length());
With a static import statement, assertion method names can be used directly like this:
import static org.junit.Assert.*;
...
assertEquals(12, message.length());
15 :: What Is the @SuiteClasses Annotation?
"@SuiteClasses" is a class annotation defined in JUnit 4.4 in org.junit.runners.Suite.SuiteClasses. It allows you to define a suite class as described in the previous question.
By the way, the API document of JUnit 4.4 has a major typo for the org.junit.runners.Suite class (Suite.html).
Using Suite as a runner allows you to manually build a suite containing tests from many classes. It is the JUnit 4 equivalent of the JUnit 3.8.x static Test suite() method. To use it, annotate a class with @RunWith(Suite.class) and @SuiteClasses(TestClass1.class, ...). When you run this class, it will run all the tests in all the suite classes.
"@SuiteClasses(TestClass1.class, ...)" should be changed to "@Suite.SuiteClasses({TestClass1.class, ...})".
Someone provided wrong information on build test suite in JUnit 4.4. Do not follow this:
JUnit provides tools to define the suite to be run and to display its results. To run tests and see the results on the console, run:
Read MoreBy the way, the API document of JUnit 4.4 has a major typo for the org.junit.runners.Suite class (Suite.html).
Using Suite as a runner allows you to manually build a suite containing tests from many classes. It is the JUnit 4 equivalent of the JUnit 3.8.x static Test suite() method. To use it, annotate a class with @RunWith(Suite.class) and @SuiteClasses(TestClass1.class, ...). When you run this class, it will run all the tests in all the suite classes.
"@SuiteClasses(TestClass1.class, ...)" should be changed to "@Suite.SuiteClasses({TestClass1.class, ...})".
Someone provided wrong information on build test suite in JUnit 4.4. Do not follow this:
JUnit provides tools to define the suite to be run and to display its results. To run tests and see the results on the console, run:
16 :: Why Not Just Use a Debugger for Unit Testing?
This is a common question in a job interview. You should answer it with these points:
* A debugger is designed for manual debugging and manual unit testing, not for automated unit testing.
* JUnit is designed for automated unit testing.
* Automated unit testing requires extra time to setup initially. But it will save your time, if your code requires changes many times in the future.
Here is how the JUnit FAQ answers this question:
Debuggers are commonly used to step through code and inspect that the variables along the way contain the expected values. But stepping through a program in a debugger is a manual process that requires tedious visual inspections. In essence, the debugging session is nothing more than a manual check of expected vs. actual results. Moreover, every time the program changes we must manually step back through the program in the debugger to ensure that nothing broke.
It generally takes less time to codify expectations in the form of an automated JUnit test that retains its value over time. If it's difficult to write a test to assert expected values, the tests may be telling you that shorter and more cohesive methods would improve your design.
Read More* A debugger is designed for manual debugging and manual unit testing, not for automated unit testing.
* JUnit is designed for automated unit testing.
* Automated unit testing requires extra time to setup initially. But it will save your time, if your code requires changes many times in the future.
Here is how the JUnit FAQ answers this question:
Debuggers are commonly used to step through code and inspect that the variables along the way contain the expected values. But stepping through a program in a debugger is a manual process that requires tedious visual inspections. In essence, the debugging session is nothing more than a manual check of expected vs. actual results. Moreover, every time the program changes we must manually step back through the program in the debugger to ensure that nothing broke.
It generally takes less time to codify expectations in the form of an automated JUnit test that retains its value over time. If it's difficult to write a test to assert expected values, the tests may be telling you that shorter and more cohesive methods would improve your design.
17 :: Why Not Just Write a main() Method for Unit Testing?
It is possible to write a main() method in each class that need to be tested for unit testing. In the main() method, you could create test object of the class itself, and write some tests to test its methods.
However, this is not a recommended approach because of the following points:
* Your classes will be cluttered with test code in main method. All those test codes will be packaged into the final product.
* If you have a lots of classes to test, you need to run the main() method of every class. This requires some extra coding effort.
* If you want the test results to be displayed in a GUI, you will have to write code for that GUI.
* If you want to log the results of tests in HTML format or text format, you will have to write additional code.
* If one method call fails, next method calls won?t be executed. You will have to work-around this.
* If you start working on a project created by some other team in your organization, you may see an entirely different approach for testing. That will increase your learning time and things won?t be standard.
Read MoreHowever, this is not a recommended approach because of the following points:
* Your classes will be cluttered with test code in main method. All those test codes will be packaged into the final product.
* If you have a lots of classes to test, you need to run the main() method of every class. This requires some extra coding effort.
* If you want the test results to be displayed in a GUI, you will have to write code for that GUI.
* If you want to log the results of tests in HTML format or text format, you will have to write additional code.
* If one method call fails, next method calls won?t be executed. You will have to work-around this.
* If you start working on a project created by some other team in your organization, you may see an entirely different approach for testing. That will increase your learning time and things won?t be standard.
18 :: Why Not Just Use System.out.println() for Unit Testing?
Inserting debug statements into code is a low-tech method for debugging it. It usually requires that output be scanned manually every time the program is run to ensure that the code is doing what's expected.
It generally takes less time in the long run to codify expectations in the form of an automated JUnit test that retains its value over time. If it's difficult to write a test to assert expectations, the tests may be telling you that shorter and more cohesive methods would improve your design.
Read MoreIt generally takes less time in the long run to codify expectations in the form of an automated JUnit test that retains its value over time. If it's difficult to write a test to assert expectations, the tests may be telling you that shorter and more cohesive methods would improve your design.
19 :: Under What Conditions Should You Test set() and get() Methods?
This is a good question for a job interview. It shows your experience with test design and data types.
Tests should be designed to target areas that might break. set() and get() methods on simple data types are unlikely to break. So no need to test them.
set() and get() methods on complex data types are likely to break. So you should test them.
Read MoreTests should be designed to target areas that might break. set() and get() methods on simple data types are unlikely to break. So no need to test them.
set() and get() methods on complex data types are likely to break. So you should test them.
20 :: Do You Need to Write a Test Class for Every Class That Need to Be Tested?
This is a simple question. But the answer shows your organization skills.
The technical answer is no. There is no need to write one test class for each every class that need to be tested. One test class can contain many tests for many test target classes.
But the practical answer is yes. You should design one test class per test target class for low level basic tests. This makes your test classes much easier to manage and maintain.
You should write separate test classes for high level tests that requires multiple target classes working together.
Read MoreThe technical answer is no. There is no need to write one test class for each every class that need to be tested. One test class can contain many tests for many test target classes.
But the practical answer is yes. You should design one test class per test target class for low level basic tests. This makes your test classes much easier to manage and maintain.
You should write separate test classes for high level tests that requires multiple target classes working together.
21 :: What Is JUnit TestCase?
JUnit TestCase is the base class, junit.framework.TestCase, used in JUnit 3.8 that allows you to create a test case. TestCase class is no longer supported in JUnit 4.4.
A test case defines the fixture to run multiple tests. To define a test case
* Implement a subclass of TestCase
* Define instance variables that store the state of the fixture
* Initialize the fixture state by overriding setUp
* Clean-up after a test by overriding tearDown
Each test runs in its own fixture so there can be no side effects among test runs. Here is an example:
import junit.framework.*;
public class MathTest extends TestCase {
protected double fValue1;
protected double fValue2;
protected void setUp() {
fValue1= 2.0;
fValue2= 3.0;
}
public void testAdd() {
double result= fValue1 + fValue2;
assertTrue(result == 5.0);
}
}
Read MoreA test case defines the fixture to run multiple tests. To define a test case
* Implement a subclass of TestCase
* Define instance variables that store the state of the fixture
* Initialize the fixture state by overriding setUp
* Clean-up after a test by overriding tearDown
Each test runs in its own fixture so there can be no side effects among test runs. Here is an example:
import junit.framework.*;
public class MathTest extends TestCase {
protected double fValue1;
protected double fValue2;
protected void setUp() {
fValue1= 2.0;
fValue2= 3.0;
}
public void testAdd() {
double result= fValue1 + fValue2;
assertTrue(result == 5.0);
}
}
22 :: How to Run Your JUnit 4.4 Tests with a JUnit 3.8 Runner?
I am not sure why you have to do this. But if you want to, you can use the junit.framework.JUnit4TestAdapter class included in JUnit 4.4 JAR file. Here is sample code:
import junit.framework.Test;
import junit.textui.TestRunner;
import junit.framework.JUnit4TestAdapter;
public class JUnit3Adapter {
public static void main (String[] args) {
Test adaptedTest = new JUnit4TestAdapter(HelloTest.class);
TestRunner.run(adaptedTest);
}
}
Classes junit.framework.Test, junit.textui.TestRunner and junit.framework.JUnit4TestAdapter are included in the JUnit 4.4 JAR file. You don't need to include the JUnit 3.8 JAR file in your CLASSPATH.
Read Moreimport junit.framework.Test;
import junit.textui.TestRunner;
import junit.framework.JUnit4TestAdapter;
public class JUnit3Adapter {
public static void main (String[] args) {
Test adaptedTest = new JUnit4TestAdapter(HelloTest.class);
TestRunner.run(adaptedTest);
}
}
Classes junit.framework.Test, junit.textui.TestRunner and junit.framework.JUnit4TestAdapter are included in the JUnit 4.4 JAR file. You don't need to include the JUnit 3.8 JAR file in your CLASSPATH.
23 :: What Are JUnit 3.8 Naming Conventions?
JUnit 3.8 suggests the following naming conventions:
Test Case Class: Named as [classname]Test.java, where "classname" is the name of the class that is being tested. A test case class define the fixture to run multiple tests. A test case class must be subclass of junit.framework.TestCase.
Test Method: Named test[XXX], where "XXX" is any unique name for this test. A test method name should be prefixed with "test" to allow the TestSuite class to extract it automatically. A test method must be declared as "public".
Test Suite: Can be named any way you want to. But Eclipse uses AllTests.java as the name. A test suite is a collection of test cases.
Read MoreTest Case Class: Named as [classname]Test.java, where "classname" is the name of the class that is being tested. A test case class define the fixture to run multiple tests. A test case class must be subclass of junit.framework.TestCase.
Test Method: Named test[XXX], where "XXX" is any unique name for this test. A test method name should be prefixed with "test" to allow the TestSuite class to extract it automatically. A test method must be declared as "public".
Test Suite: Can be named any way you want to. But Eclipse uses AllTests.java as the name. A test suite is a collection of test cases.
24 :: How Many Test Runners Are Supported in JUnit 3.8?
JUnit 3.8 supports 3 test runners:
junit.textui.TestRunner - A command line based tool to run tests. TestRunner expects the name of a TestCase class as argument. If this class defines a static suite method it will be invoked and the returned test is run. Otherwise all the methods starting with "test" having no arguments are run.
junit.awtgui.TestRunner - An AWT based user interface to run tests. Enter the name of a class which either provides a static suite method or is a subclass of TestCase. TestRunner takes as an optional argument the name of the testcase class to be run.
junit.swingui.TestRunner - A Swing based user interface to run tests. Enter the name of a class which either provides a static suite method or is a subclass of TestCase. TestRunner takes as an optional argument the name of the testcase class to be run.
All 3 runners can be executed directly by JVM with a class name as an argument
Read Morejunit.textui.TestRunner - A command line based tool to run tests. TestRunner expects the name of a TestCase class as argument. If this class defines a static suite method it will be invoked and the returned test is run. Otherwise all the methods starting with "test" having no arguments are run.
junit.awtgui.TestRunner - An AWT based user interface to run tests. Enter the name of a class which either provides a static suite method or is a subclass of TestCase. TestRunner takes as an optional argument the name of the testcase class to be run.
junit.swingui.TestRunner - A Swing based user interface to run tests. Enter the name of a class which either provides a static suite method or is a subclass of TestCase. TestRunner takes as an optional argument the name of the testcase class to be run.
All 3 runners can be executed directly by JVM with a class name as an argument
25 :: What Is a JUnit Test Fixture?
A test fixture is a fixed state of a set of objects used as a baseline for running tests. The purpose of a test fixture is to ensure that there is a well known and fixed environment in which tests are run so that results are repeatable. Examples of fixtures:
* Loading a database with a specific, known set of data
* Copying a specific known set of files
* Preparation of input data and setup/creation of fake or mock objects
In other word, creating a test fixture is to create a set of objects initialized to certain states.
If a group of tests requires diferent test fixtures, you can write code inside the test method to create its own test fixture.
If a group of tests shares the same fixtures, you should write a separate setup code to create the common test fixture.
Read More* Loading a database with a specific, known set of data
* Copying a specific known set of files
* Preparation of input data and setup/creation of fake or mock objects
In other word, creating a test fixture is to create a set of objects initialized to certain states.
If a group of tests requires diferent test fixtures, you can write code inside the test method to create its own test fixture.
If a group of tests shares the same fixtures, you should write a separate setup code to create the common test fixture.