Android Developer Interview Questions And Answers
Refine your Android Developer interview skills with our 56 critical questions. Each question is crafted to challenge your understanding and proficiency in Android Developer. Suitable for all skill levels, these questions are essential for effective preparation. Get the free PDF download to access all 56 questions and excel in your Android Developer interview. This comprehensive guide is essential for effective study and confidence building.
56 Android Developer Questions and Answers:
Android Developer Job Interview Questions Table of Contents:
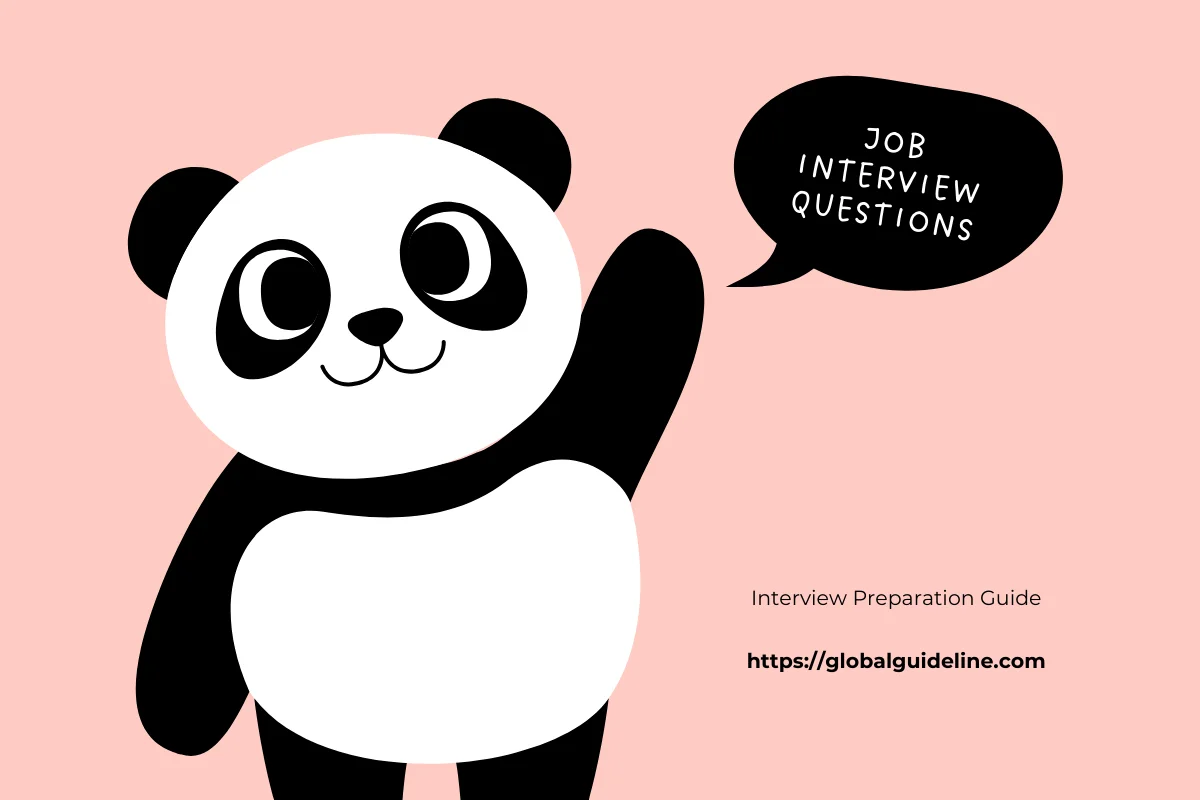
1 :: Explain me what is Context?
A Context is a handle to the system; it provides services like resolving resources, obtaining access to databases and preferences, and so on. An Android app has activities. Context is like a handle to the environment your application is currently running in.
Read More2 :: Explain me what is the Android Application Architecture?
Android application architecture has the following components:
☛ Services − It will perform background functionalities
☛ Intent − It will perform the inter connection between activities and the data passing mechanism
☛ Resource Externalization − strings and graphics
☛ Notification − light, sound, icon, notification, dialog box and toast
☛ Content Providers − It will share the data between applications
Read More☛ Services − It will perform background functionalities
☛ Intent − It will perform the inter connection between activities and the data passing mechanism
☛ Resource Externalization − strings and graphics
☛ Notification − light, sound, icon, notification, dialog box and toast
☛ Content Providers − It will share the data between applications
3 :: Explain me scenario in which only onDestroy is called for an activity without onPause() and onStop()?
If finish() is called in the OnCreate method of an activity, the system will invoke onDestroy() method directly.
Read More4 :: Explain me how does the activity respond when the user rotates the screen?
When the screen is rotated, the current instance of activity is destroyed a new instance of the Activity is created in the new orientation. The onRestart() method is invoked first when a screen is rotated. The other lifecycle methods get invoked in the similar flow as they were when the activity was first created.
Read More5 :: What are content providers?
A ContentProvider provides data from one application to another, when requested. It manages access to a structured set of data. It provides mechanisms for defining data security. ContentProvider is the standard interface that connects data in one process with code running in another process.
When you want to access data in a ContentProvider, you must instead use the ContentResolver object in your application’s Context to communicate with the provider as a client. The provider object receives data requests from clients, performs the requested action, and returns the results.
Read MoreWhen you want to access data in a ContentProvider, you must instead use the ContentResolver object in your application’s Context to communicate with the provider as a client. The provider object receives data requests from clients, performs the requested action, and returns the results.
6 :: Explain me difference between AsyncTasks & Threads?
☛ Thread should be used to separate long running operations from main thread so that performance is improved. But it can’t be cancelled elegantly and it can’t handle configuration changes of Android. You can’t update UI from Thread.
☛ AsyncTask can be used to handle work items shorter than 5ms in duration. With AsyncTask, you can update UI unlike java Thread. But many long running tasks will choke the performance.
Read More☛ AsyncTask can be used to handle work items shorter than 5ms in duration. With AsyncTask, you can update UI unlike java Thread. But many long running tasks will choke the performance.
7 :: Please explain what is a Job Scheduling?
Job Scheduling api, as the name suggests, allows to schedule jobs while letting the system optimize based on memory, power, and connectivity conditions. The JobScheduler supports batch scheduling of jobs. The Android system can combine jobs so that battery consumption is reduced. JobManager makes handling uploads easier as it handles automatically the unreliability of the network. It also survives application restarts. Some scenarios:
☛ Tasks that should be done once the device is connect to a power supply
☛ Tasks that require network access or a Wi-Fi connection.
☛ Task that are not critical or user facing
☛ Tasks that should be running on a regular basis as batch where the timing is not critical
☛ You can click on this link to learn more about Job Schedulers.
Read More☛ Tasks that should be done once the device is connect to a power supply
☛ Tasks that require network access or a Wi-Fi connection.
☛ Task that are not critical or user facing
☛ Tasks that should be running on a regular basis as batch where the timing is not critical
☛ You can click on this link to learn more about Job Schedulers.
8 :: Explain me what is an intent?
Intents are messages that can be used to pass information to the various components of android. For instance, launch an activity, open a webview etc. Two types of intents-
☛ Implicit: Implicit intent is when you call system default intent like send email, send SMS, dial number.
☛ Explicit: Explicit intent is when you call an application activity from another activity of the same application.
Read More☛ Implicit: Implicit intent is when you call system default intent like send email, send SMS, dial number.
☛ Explicit: Explicit intent is when you call an application activity from another activity of the same application.
9 :: What are fragments?
Fragment is a UI entity attached to Activity. Fragments can be reused by attaching in different activities. Activity can have multiple fragments attached to it. Fragment must be attached to an activity and its lifecycle will depend on its host activity.
Read More10 :: Do you know what is Application?
The Application class in Android is the base class within an Android app that contains all other components such as activities and services. The Application class, or any subclass of the Application class, is instantiated before any other class when the process for your application/package is created.
Read More11 :: Explain me what are the advantages of Android Operating System?
It is an open-source and platform independent. It supports various technologies like Bluetooth, Wi-Fi, etc
Read More12 :: Explain me what is a broadcast receiver?
The broadcast receiver communicates with the operation system messages such as “check whether an internet connection is available,” what the battery label should be, etc.
Read More13 :: Please explain what is difference between Serializable and Parcelable ? Which is best approach in Android?
Serializable is a standard Java interface. You simply mark a class Serializable by implementing the interface, and Java will automatically serialize it in certain situations.
Parcelable is an Android specific interface where you implement the serialization yourself. It was created to be far more efficient than Serializable, and to get around some problems with the default Java serialization scheme.
Read MoreParcelable is an Android specific interface where you implement the serialization yourself. It was created to be far more efficient than Serializable, and to get around some problems with the default Java serialization scheme.
14 :: Explain me three common use cases for using an Intent?
Common use cases for using an Intent include:
☛ To start an activity: You can start a new instance of an Activity by passing an Intent to startActivity() method.
☛ To start a service: You can start a service to perform a one-time operation (such as download a file) by passing an Intent to startService().
☛ To deliver a broadcast: You can deliver a broadcast to other apps by passing an Intent to sendBroadcast(), sendOrderedBroadcast(), or sendStickyBroadcast().
Read More☛ To start an activity: You can start a new instance of an Activity by passing an Intent to startActivity() method.
☛ To start a service: You can start a service to perform a one-time operation (such as download a file) by passing an Intent to startService().
☛ To deliver a broadcast: You can deliver a broadcast to other apps by passing an Intent to sendBroadcast(), sendOrderedBroadcast(), or sendStickyBroadcast().
15 :: Please explain what are intent Filters?
Specifies the type of intent that the activity/service can respond to.
Read More16 :: What is difference between Serializable and Parcelable?
Serialization is the process of converting an object into a stream of bytes in order to store an object into memory, so that it can be recreated at a later time, while still keeping the object’s original state and data.
Read More17 :: Tell us difference between Service, Intent Service, AsyncTask & Threads?
☛ Android service is a component that is used to perform operations on the background such as playing music. It doesn’t has any UI (user interface). The service runs in the background indefinitely even if application is destroyed.
☛ AsyncTask allows you to perform asynchronous work on your user interface. It performs the blocking operations in a worker thread and then publishes the results on the UI thread, without requiring you to handle threads and/or handlers yourself.
☛ IntentService is a base class for Services that handle asynchronous requests (expressed as Intents) on demand. Clients send requests through startService(Intent) calls; the service is started as needed, handles each Intent in turn using a worker thread, and stops itself when it runs out of work.
☛ A thread is a single sequential flow of control within a program. Threads can be thought of as mini-processes running within a main process.
Read More☛ AsyncTask allows you to perform asynchronous work on your user interface. It performs the blocking operations in a worker thread and then publishes the results on the UI thread, without requiring you to handle threads and/or handlers yourself.
☛ IntentService is a base class for Services that handle asynchronous requests (expressed as Intents) on demand. Clients send requests through startService(Intent) calls; the service is started as needed, handles each Intent in turn using a worker thread, and stops itself when it runs out of work.
☛ A thread is a single sequential flow of control within a program. Threads can be thought of as mini-processes running within a main process.
18 :: Tell me two ways to clear the back stack of Activities when a new Activity is called using intent?
The first approach is to use a FLAG_ACTIVITY_CLEAR_TOP flag. The second way is by using FLAG_ACTIVITY_CLEAR_TASK and FLAG_ACTIVITY_NEW_TASK in conjunction.
Read More19 :: Do you know launch modes in Android?
☛ Standard: It creates a new instance of an activity in the task from which it was started. Multiple instances of the activity can be created and multiple instances can be added to the same or different tasks.
☛ Eg: Suppose there is an activity stack of A -> B -> C.
☛ Now if we launch B again with the launch mode as “standard”, the new stack will be A -> B -> C -> B.
☛ SingleTop: It is the same as the standard, except if there is a previous instance of the activity that exists in the top of the stack, then it will not create a new instance but rather send the intent to the existing instance of the activity.
☛ Eg: Suppose there is an activity stack of A -> B.
☛ Now if we launch C with the launch mode as “singleTop”, the new stack will be A -> B -> C as usual.
☛ Now if there is an activity stack of A -> B -> C.
☛ If we launch C again with the launch mode as “singleTop”, the new stack will still be A -> B -> C.
☛ SingleTask: A new task will always be created and a new instance will be pushed to the task as the root one. So if the activity is already in the task, the intent will be redirected to onNewIntent() else a new instance will be created. At a time only one instance of activity will exist.
☛ Eg: Suppose there is an activity stack of A -> B -> C -> D.
☛ Now if we launch D with the launch mode as “singleTask”, the new stack will be A -> B -> C -> D as usual.
☛ Now if there is an activity stack of A -> B -> C -> D.
☛ If we launch activity B again with the launch mode as “singleTask”, the new activity stack will be A -> B. Activities C and D will be destroyed.
☛ SingleInstance: Same as single task but the system does not launch any activities in the same task as this activity. If new activities are launched, they are done so in a separate task.
☛ Eg: Suppose there is an activity stack of A -> B -> C -> D. If we launch activity B again with the launch mode as “singleInstance”, the new activity stack will be:
☛ Task1 — A -> B -> C
☛ Task2 — D
Read More☛ Eg: Suppose there is an activity stack of A -> B -> C.
☛ Now if we launch B again with the launch mode as “standard”, the new stack will be A -> B -> C -> B.
☛ SingleTop: It is the same as the standard, except if there is a previous instance of the activity that exists in the top of the stack, then it will not create a new instance but rather send the intent to the existing instance of the activity.
☛ Eg: Suppose there is an activity stack of A -> B.
☛ Now if we launch C with the launch mode as “singleTop”, the new stack will be A -> B -> C as usual.
☛ Now if there is an activity stack of A -> B -> C.
☛ If we launch C again with the launch mode as “singleTop”, the new stack will still be A -> B -> C.
☛ SingleTask: A new task will always be created and a new instance will be pushed to the task as the root one. So if the activity is already in the task, the intent will be redirected to onNewIntent() else a new instance will be created. At a time only one instance of activity will exist.
☛ Eg: Suppose there is an activity stack of A -> B -> C -> D.
☛ Now if we launch D with the launch mode as “singleTask”, the new stack will be A -> B -> C -> D as usual.
☛ Now if there is an activity stack of A -> B -> C -> D.
☛ If we launch activity B again with the launch mode as “singleTask”, the new activity stack will be A -> B. Activities C and D will be destroyed.
☛ SingleInstance: Same as single task but the system does not launch any activities in the same task as this activity. If new activities are launched, they are done so in a separate task.
☛ Eg: Suppose there is an activity stack of A -> B -> C -> D. If we launch activity B again with the launch mode as “singleInstance”, the new activity stack will be:
☛ Task1 — A -> B -> C
☛ Task2 — D
20 :: Tell us why bytecode cannot be run in Android?
Android uses DVM (Dalvik Virtual Machine ) rather using JVM(Java Virtual Machine).
Read More21 :: Tell us how do you supply construction arguments into a Fragment?
Construction arguments for a Fragment are passed via Bundle using the Fragment#setArgument(Bundle) method. The passed-in Bundle can then be retrieved through the Fragment#getArguments() method in the appropriate Fragment lifecycle method.
It is a common mistake to pass in data through a custom constructor. Non-default constructors on a Fragment are not advisable because the Fragment may be destroyed and recreated due to a configuration change (e.g. orientation change). Using #setArguments()/getArguments() ensures that when the Fragment needs to be recreated, the Bundle will be appropriately serialized/deserialized so that construction data is restored.
Read MoreIt is a common mistake to pass in data through a custom constructor. Non-default constructors on a Fragment are not advisable because the Fragment may be destroyed and recreated due to a configuration change (e.g. orientation change). Using #setArguments()/getArguments() ensures that when the Fragment needs to be recreated, the Bundle will be appropriately serialized/deserialized so that construction data is restored.
22 :: Tell us what is the difference between a fragment and an activity? Explain the relationship between the two?
An activity is typically a single, focused operation that a user can perform (such as dial a number, take a picture, send an email, view a map, etc.). Yet at the same time, there is nothing that precludes a developer from creating an activity that is arbitrarily complex.
Activity implementations can optionally make use of the Fragment class for purposes such as producing more modular code, building more sophisticated user interfaces for larger screens, helping scale applications between small and large screens, and so on. Multiple fragments can be combined within a single activity and, conversely, the same fragment can often be reused across multiple activities. This structure is largely intended to foster code reuse and facilitate economies of scale.
A fragment is essentially a modular section of an activity, with its own lifecycle and input events, and which can be added or removed at will. It is important to remember, though, that a fragment’s lifecycle is directly affected by its host activity’s lifecycle; i.e., when the activity is paused, so are all fragments in it, and when the activity is destroyed, so are all of its fragments.
Read MoreActivity implementations can optionally make use of the Fragment class for purposes such as producing more modular code, building more sophisticated user interfaces for larger screens, helping scale applications between small and large screens, and so on. Multiple fragments can be combined within a single activity and, conversely, the same fragment can often be reused across multiple activities. This structure is largely intended to foster code reuse and facilitate economies of scale.
A fragment is essentially a modular section of an activity, with its own lifecycle and input events, and which can be added or removed at will. It is important to remember, though, that a fragment’s lifecycle is directly affected by its host activity’s lifecycle; i.e., when the activity is paused, so are all fragments in it, and when the activity is destroyed, so are all of its fragments.
23 :: What is fragment lifecycle?
☛ onAttach() : The fragment instance is associated with an activity instance.The fragment and the activity is not fully initialized. Typically you get in this method a reference to the activity which uses the fragment for further initialization work.
☛ onCreate() : The system calls this method when creating the fragment. You should initialize essential components of the fragment that you want to retain when the fragment is paused or stopped, then resumed.
☛ onCreateView() : The system calls this callback when it’s time for the fragment to draw its user interface for the first time. To draw a UI for your fragment, you must return a View component from this method that is the root of your fragment’s layout. You can return null if the fragment does not provide a UI.
☛ onActivityCreated() : The onActivityCreated() is called after the onCreateView() method when the host activity is created. Activity and fragment instance have been created as well as the view hierarchy of the activity. At this point, view can be accessed with the findViewById() method. example. In this method you can instantiate objects which require a Context object
☛ onStart() : The onStart() method is called once the fragment gets visible.
☛ onResume() : Fragment becomes active.
☛ onPause() : The system calls this method as the first indication that the user is leaving the fragment. This is usually where you should commit any changes that should be persisted beyond the current user session.
☛ onStop() : Fragment going to be stopped by calling onStop()
☛ onDestroyView() : Fragment view will destroy after call this method
☛ onDestroy() :called to do final clean up of the fragment’s state but Not guaranteed to be called by the Android platform.
Read More☛ onCreate() : The system calls this method when creating the fragment. You should initialize essential components of the fragment that you want to retain when the fragment is paused or stopped, then resumed.
☛ onCreateView() : The system calls this callback when it’s time for the fragment to draw its user interface for the first time. To draw a UI for your fragment, you must return a View component from this method that is the root of your fragment’s layout. You can return null if the fragment does not provide a UI.
☛ onActivityCreated() : The onActivityCreated() is called after the onCreateView() method when the host activity is created. Activity and fragment instance have been created as well as the view hierarchy of the activity. At this point, view can be accessed with the findViewById() method. example. In this method you can instantiate objects which require a Context object
☛ onStart() : The onStart() method is called once the fragment gets visible.
☛ onResume() : Fragment becomes active.
☛ onPause() : The system calls this method as the first indication that the user is leaving the fragment. This is usually where you should commit any changes that should be persisted beyond the current user session.
☛ onStop() : Fragment going to be stopped by calling onStop()
☛ onDestroyView() : Fragment view will destroy after call this method
☛ onDestroy() :called to do final clean up of the fragment’s state but Not guaranteed to be called by the Android platform.
24 :: Tell us what is the relationship between the life cycle of an AsyncTask and an Activity? What problems can this result in? How can these problems be avoided?
An AsyncTask is not tied to the life cycle of the Activity that contains it. So, for example, if you start an AsyncTask inside an Activity and the user rotates the device, the Activity will be destroyed (and a new Activity instance will be created) but the AsyncTask will not die but instead goes on living until it completes.
Then, when the AsyncTask does complete, rather than updating the UI of the new Activity, it updates the former instance of the Activity (i.e., the one in which it was created but that is not displayed anymore!). This can lead to an Exception (of the type java.lang.IllegalArgumentException: View not attached to window manager if you use, for instance, findViewById to retrieve a view inside the Activity).
There’s also the potential for this to result in a memory leak since the AsyncTask maintains a reference to the Activity, which prevents the Activity from being garbage collected as long as the AsyncTask remains alive.
For these reasons, using AsyncTasks for long-running background tasks is generally a bad idea . Rather, for long-running background tasks, a different mechanism (such as a service) should be employed.
Read MoreThen, when the AsyncTask does complete, rather than updating the UI of the new Activity, it updates the former instance of the Activity (i.e., the one in which it was created but that is not displayed anymore!). This can lead to an Exception (of the type java.lang.IllegalArgumentException: View not attached to window manager if you use, for instance, findViewById to retrieve a view inside the Activity).
There’s also the potential for this to result in a memory leak since the AsyncTask maintains a reference to the Activity, which prevents the Activity from being garbage collected as long as the AsyncTask remains alive.
For these reasons, using AsyncTasks for long-running background tasks is generally a bad idea . Rather, for long-running background tasks, a different mechanism (such as a service) should be employed.
25 :: Please explain the difference between FLAG_ACTIVITY_CLEAR_TASK and FLAG_ACTIVITY_CLEAR_TOP?
☛ FLAG_ACTIVITY_CLEAR_TASK is used to clear all the activities from the task including any existing instances of the class invoked. The Activity launched by intent becomes the new root of the otherwise empty task list. This flag has to be used in conjunction with FLAG_ ACTIVITY_NEW_TASK.
☛ FLAG_ACTIVITY_CLEAR_TOP on the other hand, if set and if an old instance of this Activity exists in the task list then barring that all the other activities are removed and that old activity becomes the root of the task list. Else if there’s no instance of that activity then a new instance of it is made the root of the task list. Using FLAG_ACTIVITY_NEW_TASK in conjunction is a good practice, though not necessary.
Read More☛ FLAG_ACTIVITY_CLEAR_TOP on the other hand, if set and if an old instance of this Activity exists in the task list then barring that all the other activities are removed and that old activity becomes the root of the task list. Else if there’s no instance of that activity then a new instance of it is made the root of the task list. Using FLAG_ACTIVITY_NEW_TASK in conjunction is a good practice, though not necessary.