Debugging Question:
Download Job Interview Questions and Answers PDF
What is the the response of this server for this client if both programs are running on the same system?
/*This is server.c*/
#include<stdio.h>
#include<stdlib.h>
#include<netinet/in.h>
#include<sys/types.h>
#include<sys/socket.h>
int main()
{
int fd_server, fd_client, len, len_client;
struct sockaddr_in add_server, add_client;
char buff[10];
fd_server = socket(AF_INET,SOCK_STREAM,0);
if (fd_server == -1){
perror("fd_sock");
exit(1);
}
add_server.sin_family = AF_INET;
add_server.sin_port = htons(4001);
add_server.sin_addr.s_addr = inet_addr("127.0.0.1");
len = sizeof(add_server);
len = sizeof(add_client);
if( bind(fd_server,(struct sockaddr*)&add_server,len) != 0)
perror("bind");
if(listen(fd_server,5) != 0)
perror("listen");
fd_client = accept(fd_server,(struct sockaddr*)&add_client,&len_client);
if(fd_client == -1)
perror("accept");
read(fd_client,buff,10);
return 0;
}
/*This is the client.c*/
#include<stdio.h>
#include<netinet/in.h>
#include<sys/types.h>
#include<sys/socket.h>
int main()
{
int fd_client,fd, len;
struct sockaddr_in add_server;
fd_client = socket(AF_INET,SOCK_STREAM,0);
if (fd_client == -1){
perror("fd_sock");
exit(1);
}
add_server.sin_family = AF_INET;
add_server.sin_port = ntohs(4001);
add_server.sin_addr.s_addr = inet_addr("127.0.0.1");
len = sizeof(add_server);
fd = connect(fd_client,(struct sockaddr*)&add_server,len);
if(fd == -1)
perror("connect");
write(fd,"Hellon",6);
return 0;
}
a) the server will write back to the client whatever the clinet will write to the server
b) the client server communication will not work
c) the response can not be determined
d) none of the mentioned
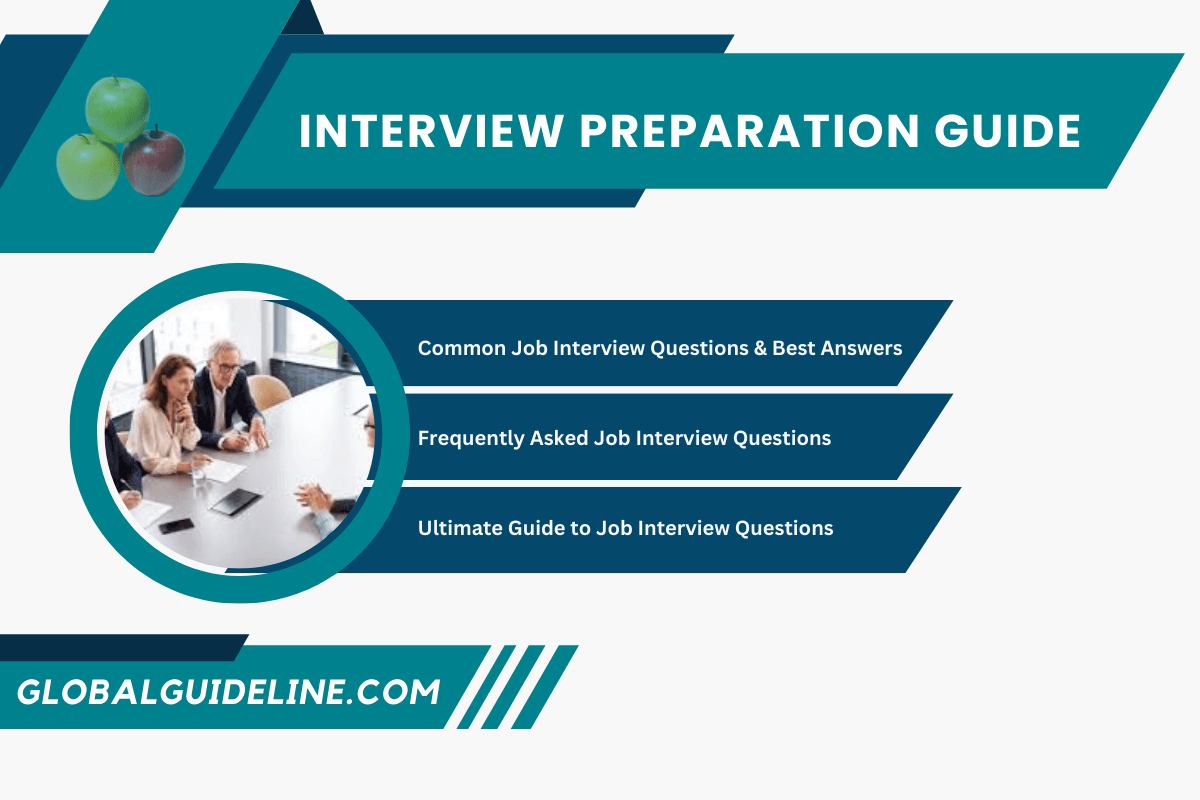
Answer:
a) the server will write back to the client whatever the client will write to the server
Explanation:
The loopback address is used as IP address in both the programs.
Explanation:
The loopback address is used as IP address in both the programs.
Download Linux Debugging Interview Questions And Answers
PDF