Front End Programmer Interview Questions And Answers
Download Front End Developer Interview Questions and Answers PDF
Optimize your Front End Developer interview preparation with our curated set of 67 questions. Each question is crafted to challenge your understanding and proficiency in Front End Developer. Suitable for all skill levels, these questions are essential for effective preparation. Get the free PDF download to access all 67 questions and excel in your Front End Developer interview. This comprehensive guide is essential for effective study and confidence building.
67 Front End Developer Questions and Answers:
Front End Developer Job Interview Questions Table of Contents:
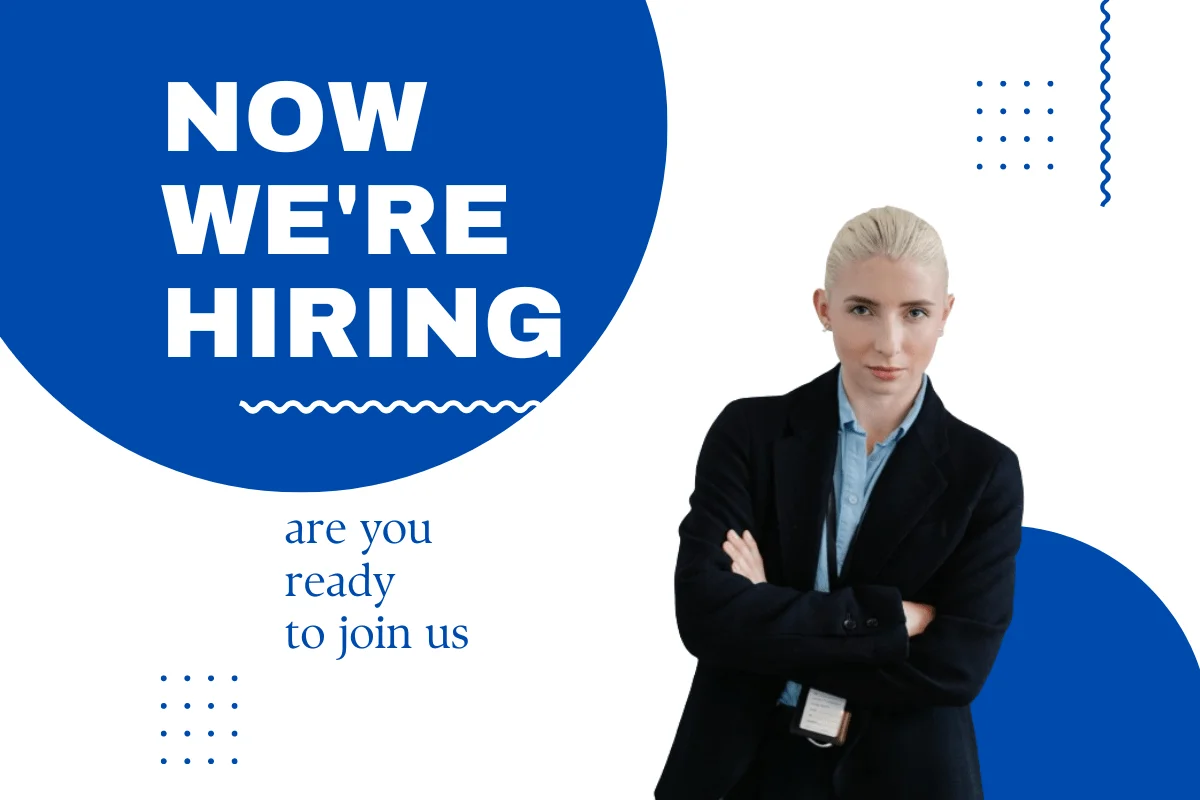
1 :: What is the difference between a host object and a native object?
Native - existing in JavaScript. Host - existing in the environment.
Read More2 :: Tell me are you familiar with Jasmine or QUnit?
Jasmine and QUnit are JavaScript testing frameworks. I would familiarize yourself with the basics.
Read More3 :: Explain what is lazy loading?
Lazy loading is a design pattern commonly used in computer programming to defer initialization of an object until the point at which it is needed.
Lazy loading is loading code only once user needs it. For Example, there is a button on the page, which shows different layout once user pressed it. So there is no need to load code for that layout on initial page load.
Read MoreLazy loading is loading code only once user needs it. For Example, there is a button on the page, which shows different layout once user pressed it. So there is no need to load code for that layout on initial page load.
4 :: Do you know what is the difference between == and === ?
== is equal to
=== is exactly equal to (value and type)
Read More=== is exactly equal to (value and type)
5 :: Do you know what is a closure?
Closures are expressions, usually functions, which can work with variables set within a certain context. Or, to try and make it easier, inner functions referring to local variables of its outer function create closures.
Read More6 :: Explain the difference between == and === ?
The 3 equal signs mean "equality without type coercion". Using the triple equals, the values must be equal in type as well.
== is equal to
=== is exactly equal to (value and type)
0==false // true
0===false // false, because they are of a different type
1=="1" // true, auto type coercion
1==="1" // false, because they are of a different type
Read More== is equal to
=== is exactly equal to (value and type)
0==false // true
0===false // false, because they are of a different type
1=="1" // true, auto type coercion
1==="1" // false, because they are of a different type
7 :: Tell me what is the difference between form get and form post?
Get:
With GET the form data is encoded into a URL by the browser. The form data is visible in the URL allowing it to be bookmarked and stored in web history. The form data is restricted to ASCII codes. Because URL lengths are limited there can be limitations on how much form data can be sent.
Post:
With POST all the name value pairs are submitted in the message body of the HTTP request which has no restrictions on the length of the string. The name value pairs cannot be seen in the web browser bar.
POST and GET correspond to different HTTP requests and they differ in how they are submitted. Since the data is encoded in differently, different decoding may be needed.
Read MoreWith GET the form data is encoded into a URL by the browser. The form data is visible in the URL allowing it to be bookmarked and stored in web history. The form data is restricted to ASCII codes. Because URL lengths are limited there can be limitations on how much form data can be sent.
Post:
With POST all the name value pairs are submitted in the message body of the HTTP request which has no restrictions on the length of the string. The name value pairs cannot be seen in the web browser bar.
POST and GET correspond to different HTTP requests and they differ in how they are submitted. Since the data is encoded in differently, different decoding may be needed.
8 :: Explain what is a javascript object?
A collection of data containing both properties and methods. Each element in a document is an object. Using the DOM you can get at each of these elements/objects and do some cool sh*t.
Read More9 :: Tell us what is the difference between HTML and XHTML?
HTML is HyperText Markup Language used to develop the website.
XHTML is modern version of HTML 4. XHTML is an HTML that follows the XML rules which should be well-formed.
Read MoreXHTML is modern version of HTML 4. XHTML is an HTML that follows the XML rules which should be well-formed.
10 :: What is a clear?
A clear is used when you don't want an element to wrap around another element, such as a float.
Read More11 :: Explain some common IE6 bugs and how you dealt with them?
Ie6 is not dead, just ask China which represents a nice chunk of the worlds online population. Your pages should at least be functional on IE6, unless you dont care about half the worlds population.
Read More13 :: Tell me what are some of the online tools and resources you use when you have a problem? Where do you go to ask questions?
This question really just looks for how resourceful the candidate is, it also reflects on their problem solving process and may lead you to ask more questions.
Read More14 :: Explain the difference between static, fixed, absolute and relative positioning?
static is the default.
fixed is positioned relative to the browser.
absolute is positioned relative to its parent or ancestor element.
relative is positioned relative to normal positioning/the item itself. Used alone it accomplishes nothing.
Read Morefixed is positioned relative to the browser.
absolute is positioned relative to its parent or ancestor element.
relative is positioned relative to normal positioning/the item itself. Used alone it accomplishes nothing.
15 :: Do you know what is a sprite? How is it applied using CSS? What is the benefit?
- A image sprite is a collection of images put into one single image.
- Using css positioning you can show and hide different parts of the sprite depending on what you need.
- Sprites reduces the number of http requsts thus reducing load time of page and bandwidth
Buy Buttons using Sprite as background:
Both buttons use the same background image. The only differece is in the positioning.
BUY BUY
Here is the actual background image:
And the CSS.
<style>
.orangeBuyBtn {
background: url('buyButtons-bg.gif') repeat-x 0 0;
border-color: #5B5752 #6B6B6B #808080;
border-style: solid;
border-width: 1px;
color: #FFFFFF;
cursor: pointer;
font-size: 14px;
font-weight: bold;
}
.greenBuyBtn {
background: url('buyButtons-bg.gif') repeat-x 0 -24px;
border-color: #5B5752 #6B6B6B #808080;
border-style: solid;
border-width: 1px;
color: #FFFFFF;
cursor: pointer;
font-size: 14px;
font-weight: bold;
}
</style>
Learn more about sprites at Smashing Magazine. Also see the Site Point article or this W3School Article.
Read More- Using css positioning you can show and hide different parts of the sprite depending on what you need.
- Sprites reduces the number of http requsts thus reducing load time of page and bandwidth
Buy Buttons using Sprite as background:
Both buttons use the same background image. The only differece is in the positioning.
BUY BUY
Here is the actual background image:
And the CSS.
<style>
.orangeBuyBtn {
background: url('buyButtons-bg.gif') repeat-x 0 0;
border-color: #5B5752 #6B6B6B #808080;
border-style: solid;
border-width: 1px;
color: #FFFFFF;
cursor: pointer;
font-size: 14px;
font-weight: bold;
}
.greenBuyBtn {
background: url('buyButtons-bg.gif') repeat-x 0 -24px;
border-color: #5B5752 #6B6B6B #808080;
border-style: solid;
border-width: 1px;
color: #FFFFFF;
cursor: pointer;
font-size: 14px;
font-weight: bold;
}
</style>
Learn more about sprites at Smashing Magazine. Also see the Site Point article or this W3School Article.
16 :: Using jQuery how would you hide the image when the user clicks it for the following code snippet?
<div class="content">
<p>Some Content Here</p>
<p class="loader"><img src="ajax-loader.gif"></p>
</div>
$(document).ready(function(){
$('.loader img').click(function() {
$('.loader img').hide();
$('.loader img').hide();
});
});
Read More$('.loader img').click(function() {
$('.loader img').hide();
$('.loader img').hide();
});
});
17 :: How to increase page performance?
(1) Sprites, compressed images, smaller images;
(2) include JavaScript at the bottom of the page;
(3) minify or concatenate your CSS and JavaScript; and
(4) caching.
Read More(2) include JavaScript at the bottom of the page;
(3) minify or concatenate your CSS and JavaScript; and
(4) caching.
18 :: Tell me the difference between visibility:hidden; and display:none;?
Visibility:Hidden; - It is not visible but takes up it's original space.
Display:None; - It is hidden and takes up absolutely no space as if it was never there.
Read MoreDisplay:None; - It is hidden and takes up absolutely no space as if it was never there.
19 :: Explain the difference between visibility:hidden; and display:none?
Visibility:Hidden; - It is not visible but takes up it's original space.
Display:None; - It is hidden and takes no space.
Read MoreDisplay:None; - It is hidden and takes no space.
20 :: Tell me what is the difference between call and apply?
apply lets you invoke the function with arguments as an array. call requires the parameters to be listed explicitly. Also, check out this stackoverflow answer.
Read More21 :: Explain what is the difference between a prototype and a class?
Prototype-based inheritance allows you to create new objects with a single operator; class-based inheritance allows you to create new objects through instantiation. Prototypes are more concrete than classes, as they are examples of objects rather than descriptions of format and instantiation.
Prototypes are important in JavaScript because JavaScript does not have classical inheritance based on classes; all inheritances happen through prototypes. If the JavaScript runtime can't find an object's property, it looks to the object's prototype, and continues up the prototype chain until the property is found.
Read MorePrototypes are important in JavaScript because JavaScript does not have classical inheritance based on classes; all inheritances happen through prototypes. If the JavaScript runtime can't find an object's property, it looks to the object's prototype, and continues up the prototype chain until the property is found.
22 :: What are the difference between GET and POST?
A GET request is typically used for things like AJAX calls to an API (insignificant changes), whereas a POST request is typically used to store data in a database or submit data via a form (significant changes). GET requests are less secure and can be seen by the user in the URL, whereas POST requests are processed in two steps and are not seen by the user. Therefore, POST requests are more secure.
Read More23 :: Explain what is the importance of the HTML DOCTYPE?
The doctype declaration should be the very first thing in an HTML document, before the html tag.
The doctype declaration is not an HTML tag; it is an instruction to the web browser about what version of the markup language the page is written in.
The doctype declaration refers to a Document Type Definition (DTD). The DTD specifies the rules for the markup language, so that the browsers can render the content correctly.
Read MoreThe doctype declaration is not an HTML tag; it is an instruction to the web browser about what version of the markup language the page is written in.
The doctype declaration refers to a Document Type Definition (DTD). The DTD specifies the rules for the markup language, so that the browsers can render the content correctly.
24 :: Explain three ways to define a color in html?
1) Hex
2) RGB
3) Name (ie red)
.colorMe {
color:red;
color:#ff0000;
color:rgb(0,0,255);
}
Read More2) RGB
3) Name (ie red)
.colorMe {
color:red;
color:#ff0000;
color:rgb(0,0,255);
}
25 :: Fresh Front End Developer Interview Questions:
☛ What are the limitations when serving XHTML pages?
☛ Are there any problems with serving pages as application/xhtml+xml?
☛ How do you serve a page with content in multiple languages?
☛ What are the different ways to visually hide content (and make it available only for screen readers)?
☛ Any familiarity with styling SVG?
☛ What are some of the "gotchas" for writing efficient CSS?
☛ What's the difference between inline and inline-block?
☛ Explain how this works in JavaScript (Yes, I admit this. I think I know it, but I don't think I have a 100% lock down on the answer here and I want a 100% lock down.)
☛ AMD vs. CommonJS?
☛ What's the difference between host objects and native objects?
Read More☛ Are there any problems with serving pages as application/xhtml+xml?
☛ How do you serve a page with content in multiple languages?
☛ What are the different ways to visually hide content (and make it available only for screen readers)?
☛ Any familiarity with styling SVG?
☛ What are some of the "gotchas" for writing efficient CSS?
☛ What's the difference between inline and inline-block?
☛ Explain how this works in JavaScript (Yes, I admit this. I think I know it, but I don't think I have a 100% lock down on the answer here and I want a 100% lock down.)
☛ AMD vs. CommonJS?
☛ What's the difference between host objects and native objects?