Project Planning Question:
Download Job Interview Questions and Answers PDF
Write the InStr function. Write the test cases for this function?
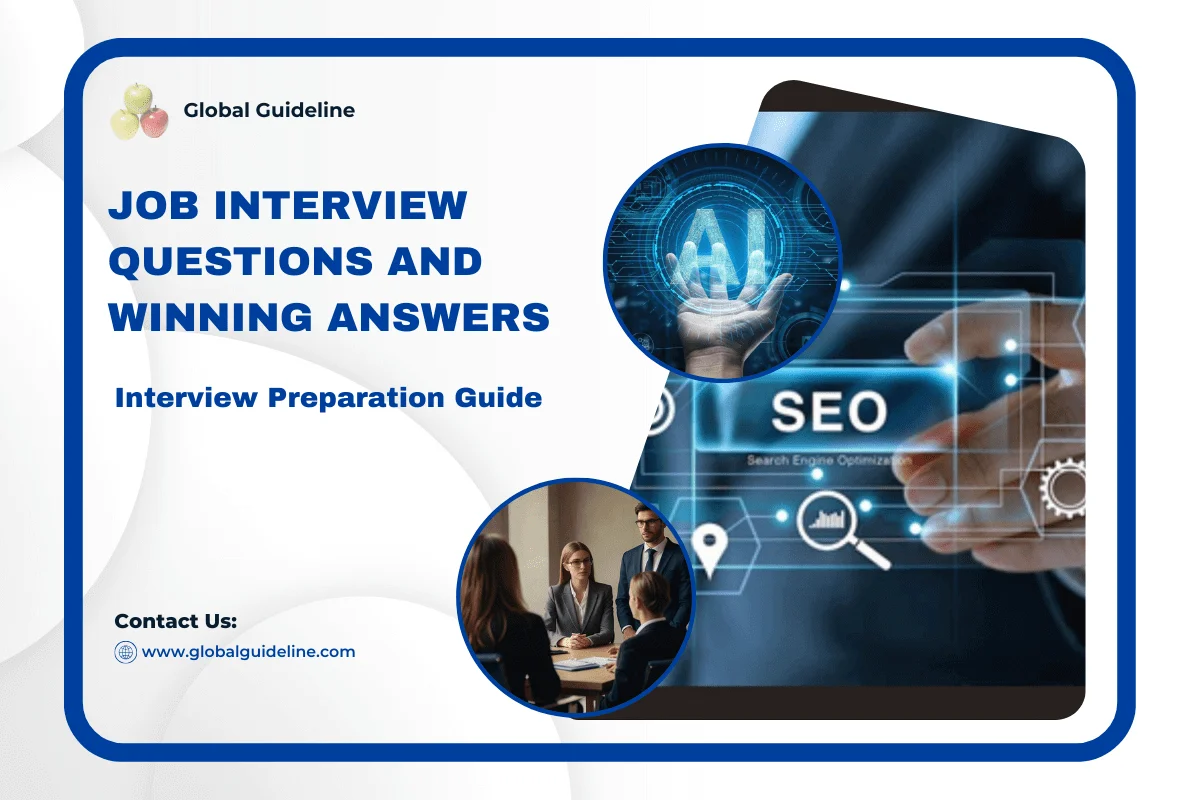
Answer:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace InStr
{
class Program
{
static void Main(string[] args)
{
//test case 1, should return -1
string txt = "this is a test";
string searchString = "ae";
Console.WriteLine(InStr(txt, searchString));
//cast case 2, should return 2
txt = "this is a test";
searchString = "is";
Console.WriteLine(InStr(txt, searchString));
Console.Read();
}
//return the position of the first occurrence of one
string "searchString" within another "txt"
//return -1 means cannot find
static int InStr(string txt, string searchString)
{
return txt.IndexOf(searchString);
}
}
}
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace InStr
{
class Program
{
static void Main(string[] args)
{
//test case 1, should return -1
string txt = "this is a test";
string searchString = "ae";
Console.WriteLine(InStr(txt, searchString));
//cast case 2, should return 2
txt = "this is a test";
searchString = "is";
Console.WriteLine(InStr(txt, searchString));
Console.Read();
}
//return the position of the first occurrence of one
string "searchString" within another "txt"
//return -1 means cannot find
static int InStr(string txt, string searchString)
{
return txt.IndexOf(searchString);
}
}
}
Download Project Planning Interview Questions And Answers
PDF