Debugging Interview Questions And Answers
Download Linux Debugging Interview Questions and Answers PDF
Elevate your Linux Debugging interview readiness with our detailed compilation of 80 questions. These questions are specifically selected to challenge and enhance your knowledge in Linux Debugging. Perfect for all proficiency levels, they are key to your interview success. Download the free PDF now to get all 80 questions and ensure you're well-prepared for your Linux Debugging interview. This resource is perfect for in-depth preparation and boosting your confidence.
80 Linux Debugging Questions and Answers:
Linux Debugging Job Interview Questions Table of Contents:
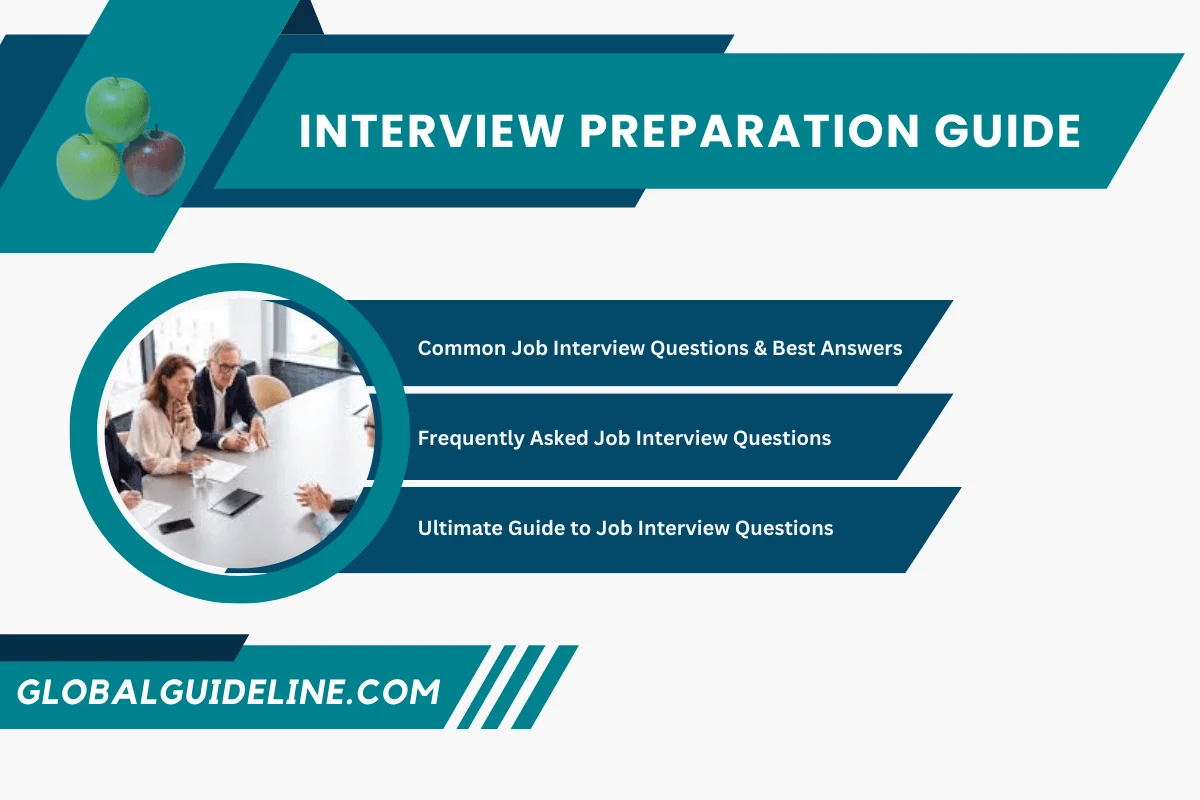
1 :: Which one of the following string will print first by this program?
#include<stdio.h>
#include<pthread.h>
void *fun_t(void *arg);
void *fun_t(void *arg)
{
printf("Googlen");
pthread_exit("Bye");
}
int main()
{
pthread_t pt;
void *res_t;
if(pthread_create(&pt,NULL,fun_t,NULL) != 0)
perror("pthread_create");
printf("Linuxn");
if(pthread_join(pt,&res_t) != 0)
perror("pthread_join");
return 0;
}
a) Linux
b) Google
c) it can not be predicted
d) none of the mentioned
b) Google
Explanation:It depends upon the scheduler.
Output:
[root@localhost Google]# gcc -o san san.c -lpthread
[root@localhost Google]# ./san
Google
Linux
[root@localhost threads]#
Read MoreExplanation:It depends upon the scheduler.
Output:
[root@localhost Google]# gcc -o san san.c -lpthread
[root@localhost Google]# ./san
Linux
[root@localhost threads]#
2 :: What is the output of this program?
#include<stdio.h>
#include<pthread.h>
void *fun_t(void *arg);
void *fun_t(void *arg)
{
int ret;
ret = pthread_exit("Bye");
printf("%dn",ret);
}
int main()
{
pthread_t pt;
void *res_t;
if(pthread_create(&pt,NULL,fun_t,NULL) != 0)
perror("pthread_create");
if(pthread_join(pt,&res_t) != 0)
perror("pthread_join");
return 0;
}
a) 0
b) 1
c) -1
d) none of the mentioned
d) none of the mentioned
Explanation:
The function pthread_exit() does not return any value. Hence this program will give an error.
Output:
[root@localhost Google]# gcc -o san san.c -lpthread
san.c: In function 'fun_t':
san.c:8:6: error: void value not ignored as it ought to be
[root@localhost google]#
Read MoreExplanation:
The function pthread_exit() does not return any value. Hence this program will give an error.
Output:
[root@localhost Google]# gcc -o san san.c -lpthread
san.c: In function 'fun_t':
san.c:8:6: error: void value not ignored as it ought to be
[root@localhost google]#
3 :: What is the output of this program no 1?
#include<stdio.h>
#include<pthread.h>
void *fun_t(void *arg);
void *fun_t(void *arg)
{
printf("googlen");
pthread_exit("Bye");
}
int main()
{
pthread_t pt;
void *res_t;
if(pthread_create(&pt,NULL,fun_t,NULL) != 0)
perror("pthread_create");
return 0;
}
a) this program will print the string "google"
b) this program will print nothing
c) segmentation fault
d) run time error
b) this program will print nothing
Explanation:The pthread_join() function waits for the thread to terminate.
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
[root@localhost google]#
Read MoreExplanation:The pthread_join() function waits for the thread to terminate.
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
[root@localhost google]#
4 :: What is the output of this program no 2?
#include<stdio.h>
#include<pthread.h>
void *fun_t(void *arg);
void *fun_t(void *arg)
{
printf("%dn",a);
pthread_exit("Bye");
}
int main()
{
int a;
pthread_t pt;
void *res_t;
a = 10;
if(pthread_create(&pt,NULL,fun_t,NULL) != 0)
perror("pthread_create");
if(pthread_join(pt,&res_t) != 0)
perror("pthread_join");
return 0;
}
a) 10
b) 0
c) -1
d) none of the mentioned
d) none of the mentioned
Explanation:
Each thread has its own stack so local variables are not shared among thread. Hence this program will give an error.
Output:
[root@localhost google]# gcc -o san san.c -lpthread
san.c: In function 'fun_t':
san.c:7:16: error: 'a' undeclared (first use in this function)
san.c:7:16: note: each undeclared identifier is reported only once for each function it appears in
[root@localhost google]#
Read MoreExplanation:
Each thread has its own stack so local variables are not shared among thread. Hence this program will give an error.
Output:
[root@localhost google]# gcc -o san san.c -lpthread
san.c: In function 'fun_t':
san.c:7:16: error: 'a' undeclared (first use in this function)
san.c:7:16: note: each undeclared identifier is reported only once for each function it appears in
[root@localhost google]#
5 :: What is the output of this program no 3?
#include<stdio.h>
#include<pthread.h>
int a;
void *fun_t(void *arg);
void *fun_t(void *arg)
{
printf("%dn",a);
pthread_exit("Bye");
}
int main()
{
pthread_t pt;
void *res_t;
a = 10;
if(pthread_create(&pt,NULL,fun_t,NULL) != 0)
perror("pthread_create");
if(pthread_join(pt,&res_t) != 0)
perror("pthread_join");
return 0;
}
a) 10
b) 0
c) -1
d) none of the mentioned
a) 10
Explanation:
Thread of the same process shares the global variables.
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
10
[root@localhost google]#
Read MoreExplanation:
Thread of the same process shares the global variables.
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
10
[root@localhost google]#
6 :: What is the output of this program no 4?
#include<stdio.h>
#include<pthread.h>
int a;
void *fun_t(void *arg);
void *fun_t(void *arg)
{
a = 20;
pthread_exit("Bye");
}
int main()
{
pthread_t pt;
void *res_t;
a = 10;
if(pthread_create(&pt,NULL,fun_t,NULL) != 0)
perror("pthread_create");
if(pthread_join(pt,&res_t) != 0)
perror("pthread_join");
printf("%dn",a);
return 0;
}
a) 10
b) 20
c) segmentation fault
d) none of the mentioned
b) 20
Explanation:
In this program the value of variable "a" is changed by the thread "fun_t".
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
20
[root@localhost google]#
Read MoreExplanation:
In this program the value of variable "a" is changed by the thread "fun_t".
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
20
[root@localhost google]#
7 :: Which one of the following statement is not true about this program?
#include<stdio.h>
#include<pthread.h>
void *fun_t(void *arg);
void *fun_t(void *arg)
{
printf("%dn",getpid());
pthread_exit("Bye");
}
int main()
{
pthread_t pt;
void *res_t;
if(pthread_create(&pt,NULL,fun_t,NULL) != 0)
perror("pthread_create");
if(pthread_join(pt,&res_t) != 0)
perror("pthread_join");
printf("%dn",getpid());
return 0;
}
a) both printf statements will print the same value
b) both printf statements will print the different values
c) this program will print nothing
d) none of the mentioned
a) both printf statements will print the same value
Explanation:
All the threads of the same process have same PID.
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
12981
12981
[root@localhost google]#
Read MoreExplanation:
All the threads of the same process have same PID.
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
12981
12981
[root@localhost google]#
8 :: What is the output of this program no 6?
#include<stdio.h>
#include<pthread.h>
#include<fcntl.h>
int fd;
void *fun_t(void *arg);
void *fun_t(void *arg)
{
char buff[10];
int count;
count = read(fd,buff,10);
printf("%dn",count);
pthread_exit("Bye");
}
int main()
{
pthread_t pt;
void *res_t;
fd = open("san.c",O_RDONLY);
if(pthread_create(&pt,NULL,fun_t,NULL) != 0)
perror("pthread_create");
if(pthread_join(pt,&res_t) != 0)
perror("pthread_join");
return 0;
}
a) 10
b) 0
c) -1
d) segmentation fault
a) 10
Explanation:
Open file descritpors can be shares between threads of the same process
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
10
[root@localhost google]#
Read MoreExplanation:
Open file descritpors can be shares between threads of the same process
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
10
[root@localhost google]#
9 :: What is the output of this program no 7?
#include<stdio.h>
#include<pthread.h>
#include<fcntl.h>
void *fun_t(void *arg);
void *fun_t(void *arg)
{
pthread_exit("Bye");
printf("googlen");
}
int main()
{
pthread_t pt;
void *res_t;
if(pthread_create(&pt,NULL,fun_t,NULL) != 0)
perror("pthread_create");
if(pthread_join(pt,&res_t) != 0)
perror("pthread_join");
printf("%sn",res_t);
return 0;
}
a) google
b) Bye
c) segementation fault
d) run time error
b) Bye
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
Bye
[root@localhost google]#
Read MoreOutput:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
Bye
[root@localhost google]#
10 :: What is the output of this program no 8?
#include<stdio.h>
#include<pthread.h>
void *fun_t(void *arg);
void *fun_t(void *arg)
{
sleep(1);
}
int main()
{
pthread_t pt;
void *res_t;
if(pthread_create(&pt,NULL,fun_t,NULL) != 0)
perror("pthread_create");
if(pthread_join(pt,&res_t) != 0)
perror("pthread_join");
printf("%sn",res_t);
return 0;
}
a) this process will pause
b) segmentation fault
c) run time error
d) none of the mentioned
b) segmentation fault
Explanation:
This program is trying to print the return value of the thread, but pthread_exit() function is not present in the thread.
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
Segmentation fault (core dumped)
[root@localhost google]#
Read MoreExplanation:
This program is trying to print the return value of the thread, but pthread_exit() function is not present in the thread.
Output:
[root@localhost google]# gcc -o san san.c -lpthread
[root@localhost google]# ./san
Segmentation fault (core dumped)
[root@localhost google]#
11 :: For debugging with GDB, the file "google" can be created with the command:
a) gcc -g -o google google.c
b) gcc -g google.c
c) gdb google
d) none of the mentioned
a) gcc -g -o google google.c
Read More12 :: For debugging with GDB, the compiled program can be run by the command
a) run
b) execute
c) ./
d) none of the mentioned
a) run
Read More13 :: In GDB, breakpoints can be set by the command:
a) break
b) b
c) both (a) and (b)
d) none of the mentioned
c) both (a) and (b)
Read More14 :: GDB stands for:
a) GNU debugger
b) general debugging breakpoint
c) general debugger
d) none of the mentioned
a) GNU debugger
Read More15 :: GDB can be used for:
a) c language
b) c++ language
c) both (a) and (b)
d) none of the mentioned
c) both (a) and (b)
Read More16 :: The command "gdb google"
a) will start debugging for the file "google" if the file is compiled with -g option with GCC
b) will create executable for debugging
c) will provide all errors present in the file "google"
d) none of the mentioned
a) will start debugging for the file "google" if the file is compiled with -g option with GCC
Read More17 :: In debugging with GDB, break points can be set to:
a) any line
b) any function
c) both (a) and (b)
d) none of the mentioned
c) both (a) and (b)
Read More18 :: In GDB debugging, we can proceed to the next break-point with command:
a) next
b) continue
c) both (a) and (b)
d) none of the mentioned
b) continue
Read More19 :: At the time of debugging with GDB, if we just press ENTER:
a) GDB will repeat the same command you just gave it
b) GDB will do nothing
c) GDB will exit
d) none of the mentioned
a) GDB will repeat the same command you just gave it
Read More20 :: To print the value of a variable while debugging with GDB, ______ command can be used.
a) printf
b) print
c) show
d) none of the mentioned
b) print
Read More21 :: Which GDB command prints the value of a variable in hex.
a) print/x
b) print/h
c) print/e
d) none of the mentioned
a) print/x
Read More22 :: Which GDB command interrupts the program whenever the value of a variable is modified and prints the value old and new values of the variable?
a) watch
b) show
c) trace
d) none of the mentioned
a) watch
Read More23 :: Which GDB command produces a stack trace of the function calls that lead to a segmentation fault?
a) trace
b) backtrace
c) forwardtrace
d) none of the mentioned
b) backtrace
Read More24 :: The specific break point can be deleted by _____ command in GDB.
a) delete
b) del
c) remove
d) none of the mentioned
a) delete
Read More25 :: The "step" command of GDB:
a) executes the current line of the program
b) stops the next statement to be executed
c) both (a) and (b)
d) none of the mentioned
c) both (a) and (b)
Read More