Senior .Net Developer Interview Questions And Answers
Download Senior .Net Developer Interview Questions and Answers PDF
Enhance your Senior .Net Developer interview preparation with our set of 60 carefully chosen questions. Our questions cover a wide range of topics in Senior .Net Developer to ensure you're well-prepared. Whether you're new to the field or have years of experience, these questions are designed to help you succeed. Download the free PDF to have all 60 questions at your fingertips. This resource is designed to boost your confidence and ensure you're interview-ready.
60 Senior .Net Developer Questions and Answers:
Senior .Net Developer Job Interview Questions Table of Contents:
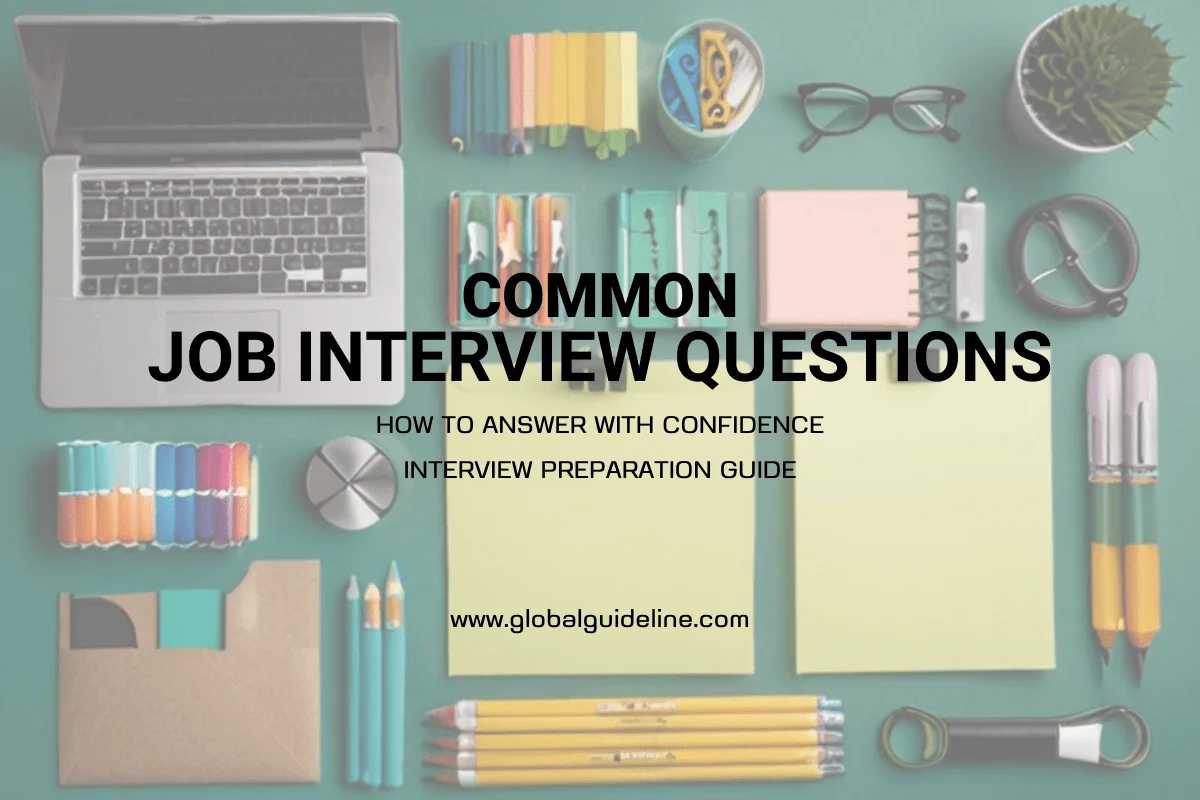
1 :: Do you know what is Garbage Collector?
Garbage Collector is an automatic process of memory release. When memory goes low, it goes through the Heap and eliminates the objects no longer in use. It frees up memory, reorganizes remaining threads and adjusts pointers to these objects, both in Heap and Stack.
Read More2 :: Do you know what is the difference between an abstract class and an interface?
☛ An abstract class can contain both public and private constructors, methods, and fields. On the contrary, the interface contains only methods and public properties.
☛ You can only inherit from an abstract class, but implement many interfaces.
☛ An interface defines behavior, something that the class that implements it can do. Contrary, an abstract class defines what the class is and what it represents.
☛ You can’t instantiate anyone.
☛ An abstract class is useful when creating components, making a partial initial implementation and a specific definition. This leaves you free to implement other methods.
Read More☛ You can only inherit from an abstract class, but implement many interfaces.
☛ An interface defines behavior, something that the class that implements it can do. Contrary, an abstract class defines what the class is and what it represents.
☛ You can’t instantiate anyone.
☛ An abstract class is useful when creating components, making a partial initial implementation and a specific definition. This leaves you free to implement other methods.
3 :: Please tell us what is the difference between Override and Overload in a method?
Override is to overwrite the method with the same signature (parameters and return type) but different functionality. Overwriting requires a “virtual” declaration of the method.
On the other hand, overloading refers to coding several versions of the same method. Though the “virtual” declaration for a method is not necessary to overload, it requires a different signature (parameters and/or return value).
Read MoreOn the other hand, overloading refers to coding several versions of the same method. Though the “virtual” declaration for a method is not necessary to overload, it requires a different signature (parameters and/or return value).
4 :: Tell us why do we use the “using” statement?
We use the “using” statement to make sure that we release the resources of the object in use. It always calls “Dispose of” when it finishes its block of code.
Read More5 :: Do you know what is .NET Standard?
☛ .NET Standard solves the code sharing problem for .NET developers across all platforms by bringing all the APIs that you expect and love across the environments that you need: desktop applications, mobile apps & games, and cloud services
☛ .NET Standard is a set of APIs that all .NET platforms have to implement. This unifies the .NET platforms and prevents future fragmentation.
☛ .NET Standard 2.0 will be implemented by .NET Framework, .NET Core, and Xamarin. For .NET Core, this will add many of the existing APIs that have been requested.
☛ .NET Standard 2.0 includes a compatibility shim for .NET Framework binaries, significantly increasing the set of libraries that you can reference from your .NET Standard libraries.
☛ .NET Standard will replace Portable Class Libraries (PCLs) as the tooling story for building multi-platform .NET libraries.
Read More☛ .NET Standard is a set of APIs that all .NET platforms have to implement. This unifies the .NET platforms and prevents future fragmentation.
☛ .NET Standard 2.0 will be implemented by .NET Framework, .NET Core, and Xamarin. For .NET Core, this will add many of the existing APIs that have been requested.
☛ .NET Standard 2.0 includes a compatibility shim for .NET Framework binaries, significantly increasing the set of libraries that you can reference from your .NET Standard libraries.
☛ .NET Standard will replace Portable Class Libraries (PCLs) as the tooling story for building multi-platform .NET libraries.
6 :: Tell us the difference between managed and unmanaged code?
Managed code is a code created by the .NET compiler. It does not depend on the architecture of the target machine because it is executed by the CLR (Common Language Runtime), and not by the operating system itself. CLR and managed code offers developers few benefits, like garbage collection, type checking and exceptions handling.
On the other hand, unmanaged code is directly compiled to native machine code and depends on the architecture of the target machine. It is executed directly by the operating system. In the unmanaged code, the developer has to make sure he is dealing with memory usage and allocation (especially because of memory leaks), type safety and exceptions manually.
In .NET, Visual Basic and C# compiler creates managed code. To get unmanaged code, the application has to be written in C or C++.
Read MoreOn the other hand, unmanaged code is directly compiled to native machine code and depends on the architecture of the target machine. It is executed directly by the operating system. In the unmanaged code, the developer has to make sure he is dealing with memory usage and allocation (especially because of memory leaks), type safety and exceptions manually.
In .NET, Visual Basic and C# compiler creates managed code. To get unmanaged code, the application has to be written in C or C++.
7 :: Please explain what inheritance is, and why it’s important?
Inheritance is one of the most important concepts in object-oriented programming, together with encapsulation and polymorphism. Inheritance allows developers to create new classes that reuse, extend, and modify the behavior defined in other classes. This enables code reuse and speeds up development. With inheritance, developers can write and debug one class only once, and then reuse that same code as the basis for the new classes. The class whose members are inherited is called the base class, and the class that inherits those members is called the derived class. By default, all classes in .NET are inheritable.
Read More8 :: What is implement a generic action in WebAPI?
It’s not possible, as the WebAPI runtime needs to know the method signatures in advance.
Read More9 :: What is the difference between a stack and a queue?
This .NET interview question tests candidates’ basic knowledge of collections. Along with stacks and queues in this category are hash tables, bags, dictionaries and lists. A stack keeps track of what is executing and contains stored value types to be accessed and processed as LIFO (Last-In, First-Out), with elements inserted and deleted from the top end.
A queue, on the other hand, lists items on a FIFO (First-In, First-Out) basis in terms of both insertion and deletion, with items inserted from the rear end and deleted from the front end of the queue.
Read MoreA queue, on the other hand, lists items on a FIFO (First-In, First-Out) basis in terms of both insertion and deletion, with items inserted from the rear end and deleted from the front end of the queue.
10 :: Do you know what are three common acronyms used in .NET, and what do they stand for?
This one should be easy for .NET developer candidates to answer. The question allows them some flexibility in choosing terms with which they are most familiar. Three frequently used acronyms in .NET are IL, CIL and CLI:
☛ IL stands for Intermediate Language, which is an object-oriented programming language that is a partially compiled code that .NET developers will then compile to native machine code.
☛ CIL stands for Common Intermediate Language, formerly known as Microsoft Intermediate Language (MSIL). This is another programming language that .NET developers use, and it represents the lowest possible level for a language that humans can still read.
☛ CLI stands for Common Language Infrastructure. This is a compiled code library that Microsoft developed as an open specification. Developers use CLI for security, versioning and deployment purposes.
Read More☛ IL stands for Intermediate Language, which is an object-oriented programming language that is a partially compiled code that .NET developers will then compile to native machine code.
☛ CIL stands for Common Intermediate Language, formerly known as Microsoft Intermediate Language (MSIL). This is another programming language that .NET developers use, and it represents the lowest possible level for a language that humans can still read.
☛ CLI stands for Common Language Infrastructure. This is a compiled code library that Microsoft developed as an open specification. Developers use CLI for security, versioning and deployment purposes.
11 :: Explain me what are the deferred execution and the immediate execution in LINQ?
A deferred execution encapsulates a query’s definition without executing it till the data is used at runtime. However, an immediate implementation calls the query at the same moment of its definition.
By default, the executions are deferred but we can do them immediately by calling “To List ()”. For example, in this way, a list of objects will be executed and returned to us when we define it.
Read MoreBy default, the executions are deferred but we can do them immediately by calling “To List ()”. For example, in this way, a list of objects will be executed and returned to us when we define it.
13 :: Tell us what is the difference between struct and class?
A class is a definition of an object and is inheritable. A structure, on the other hand, defines a type of data and is non-inheritable.
Read More14 :: Explain me what is an anonymous method and how is it different from a lambda expression?
For an anonymous method, the declaration comes before its use and implementation through a delegate. Also, this method doesn’t require a name.
A lambda expression refers to an anonymous method in a single line, elegantly replacing the representative for this function.
Read MoreA lambda expression refers to an anonymous method in a single line, elegantly replacing the representative for this function.
15 :: Explain me why do we use MSMQ?
Microsoft Message Queuing, or MSMQ, is technology for asynchronous messaging. Whenever there's need for two or more applications (processes) to send messages to each other without having to immediately know results, MSMQ can be used. MSMQ can communicate between remote machines, even over Internet. It's free and comes with Windows, but is not installed by default.
This mainly addresses the common use case of asynchronous message processing: you have a service Service1 that communicates (send messages) with another part of your software architecture, say Service2.
Main problem: what if Service2 becomes suddenly unavailable? Will messages be lost? If you use MSMQ it won't: Service1 will send messages into a queue, and Service2 will dequeue when it is available.
Read MoreThis mainly addresses the common use case of asynchronous message processing: you have a service Service1 that communicates (send messages) with another part of your software architecture, say Service2.
Main problem: what if Service2 becomes suddenly unavailable? Will messages be lost? If you use MSMQ it won't: Service1 will send messages into a queue, and Service2 will dequeue when it is available.
16 :: Tell us the difference between the while and for loop. Provide a .NET syntax for both loops?
Both loops are used when a unit of code needs to execute repeatedly. The difference is that the for loop is used when you know how many times you need to iterate through the code. On the other hand, the while loop is used when you need to repeat something until a given statement is true.
The syntax of the while loop in C# is:
while (condition [is true])
{
// statements
}
The syntax of the while loop in VB.NET is:
While condition [is True]
' statements
End While
The syntax of the for loop in C# is:
for (initializer; condition; iterator)
{
// statements
}
The syntax of the for loop in VB.NET is:
For counter [ As datatype ] = start To end [ Step step ]
' statements
Next [ counter ]
Read MoreThe syntax of the while loop in C# is:
while (condition [is true])
{
// statements
}
The syntax of the while loop in VB.NET is:
While condition [is True]
' statements
End While
The syntax of the for loop in C# is:
for (initializer; condition; iterator)
{
// statements
}
The syntax of the for loop in VB.NET is:
For counter [ As datatype ] = start To end [ Step step ]
' statements
Next [ counter ]
17 :: Tell us what do the following acronyms in .NET stand for: IL, CIL, MSIL, CLI and JIT?
IL, or Intermediate Language, is a CPU independent partially compiled code. IL code will be compiled to native machine code using current environmental properties by Just-In-Time compiler (JIT). JIT compiler translates the IL code to an assembly code and uses the CPU architecture of the target machine to execute a .NET application. In .NET, IL is called Common Intermediate Language (CIL), and in the early .NET days it was called Microsoft Intermediate Language (MSIL).
CLI, or Common Language Infrastructure, is an open specification developed by Microsoft. It is a compiled code library used for deployment, versioning, and security. In .NET there are two CLI types: process assemblies (EXE) and library assemblies (DLL). CLI assemblies contain code in CIL, and as mentioned, during compilation of CLI programming languages, the source code is translated into CIL code rather than into platform or processor specific object code.
To summarize:
☛ When compiled, source code is first translated to IL (in .NET, that is CIL, and previously called MSIL).
☛ CIL is then assembled into a bytecode and a CLI assembly is created.
☛ Before code execution, CLI code is passed through the runtime’s JIT compiler to generate native machine code.
☛ The computer’s processor executes the native machine code.
Read MoreCLI, or Common Language Infrastructure, is an open specification developed by Microsoft. It is a compiled code library used for deployment, versioning, and security. In .NET there are two CLI types: process assemblies (EXE) and library assemblies (DLL). CLI assemblies contain code in CIL, and as mentioned, during compilation of CLI programming languages, the source code is translated into CIL code rather than into platform or processor specific object code.
To summarize:
☛ When compiled, source code is first translated to IL (in .NET, that is CIL, and previously called MSIL).
☛ CIL is then assembled into a bytecode and a CLI assembly is created.
☛ Before code execution, CLI code is passed through the runtime’s JIT compiler to generate native machine code.
☛ The computer’s processor executes the native machine code.
18 :: Explain me what is the difference between a class and an object, and how do these terms relate to each other?
A class is a comprehensive data type that is the primary building block, or template, of OOP. Class defines attributes and methods of objects, and contains an object’s behavior and data. An object, however, represents an instance of class. As a basic unit of a system, objects have identity and behavior as well as attributes.
Make sure candidates respond to the second part of this .NET interview question, addressing how these terms are related to each other. Answer: The relationship is based on the fact that a class defines the states and properties that are common to a range of objects.
Read MoreMake sure candidates respond to the second part of this .NET interview question, addressing how these terms are related to each other. Answer: The relationship is based on the fact that a class defines the states and properties that are common to a range of objects.
19 :: As you know read-only variables and constants have many similarities, but what is at least one way that they differ?
Here are two possible answers to .NET interview questions of this nature:
☛ Read-only variables can support reference-type variables. Constants can hold only value-type variables.
☛ Developers evaluate read-only variables at the runtime. They evaluate constants at the compile time.
Read More☛ Read-only variables can support reference-type variables. Constants can hold only value-type variables.
☛ Developers evaluate read-only variables at the runtime. They evaluate constants at the compile time.
20 :: Explain me what is the .Net framework and how does it work?
It is a virtual machine that executes a managed code. The code is compiled from C# or VB .NET and is executed by the CLR (Common Language Runtime).
Its working is as follows:
☛ You create a program in C # or VB.Net and compile it. The code is then translated to CIL (Common Intermediate Language).
☛ The program is assembled into bytecode to generate a CLI (Common Language Infrastructure) assembly file of.exe or .dll format.
☛ When you run the program (or the DLL), it is executed by the .Net framework CLR (Common Language Runtime). Since the code isn’t directly run by the operating system, it is called “Managed Code”.
☛ The .Net Framework CLR, through the JIT (Just-In-Time) Compiler, is responsible for compiling this code managed in the intermediate language. The compiled code is then sent to the native machine language assembler for the CPU to execute it.
Read MoreIts working is as follows:
☛ You create a program in C # or VB.Net and compile it. The code is then translated to CIL (Common Intermediate Language).
☛ The program is assembled into bytecode to generate a CLI (Common Language Infrastructure) assembly file of.exe or .dll format.
☛ When you run the program (or the DLL), it is executed by the .Net framework CLR (Common Language Runtime). Since the code isn’t directly run by the operating system, it is called “Managed Code”.
☛ The .Net Framework CLR, through the JIT (Just-In-Time) Compiler, is responsible for compiling this code managed in the intermediate language. The compiled code is then sent to the native machine language assembler for the CPU to execute it.
21 :: Tell me what is serialization?
Serialization converts an object to a data stream. However, for this, you must implement ISerialize.
Read More22 :: Please explain what is the difference between encrypting a password and applying a hashing?
It is quite difficult (almost impossible) to decrypt a hashing (MD5 or SHA1, for example). The process of password validation compares the password in plain text with a hash to the stored one.
Conversely, one can decrypt an encrypted password with access to the keys and the encryption algorithms (such as Triple-DES).
Read MoreConversely, one can decrypt an encrypted password with access to the keys and the encryption algorithms (such as Triple-DES).
23 :: What is the difference between Task and Thread in .NET?
☛ Thread represents an actual OS-level thread, with its own stack and kernel resources. Thread allows the highest degree of control; you can Abort() or Suspend() or Resume() a thread, you can observe its state, and you can set thread-level properties like the stack size, apartment state, or culture. ThreadPool is a wrapper around a pool of threads maintained by the CLR.
☛ The Task class from the Task Parallel Library offers the best of both worlds. Like the ThreadPool, a task does not create its own OS thread. Instead, tasks are executed by a TaskScheduler; the default scheduler simply runs on the ThreadPool. Unlike the ThreadPool, Task also allows you to find out when it finishes, and (via the generic Task) to return a result.
Read More☛ The Task class from the Task Parallel Library offers the best of both worlds. Like the ThreadPool, a task does not create its own OS thread. Instead, tasks are executed by a TaskScheduler; the default scheduler simply runs on the ThreadPool. Unlike the ThreadPool, Task also allows you to find out when it finishes, and (via the generic Task) to return a result.
24 :: What is the difference between boxing and unboxing?
Boxing is the process of converting a value type to the type object, and unboxing is extracting the value type from the object. While the boxing is implicit, unboxing is explicit.
Example (written in C#):
int i = 13;
object myObject = i; // boxing
i = (int)myObject; // unboxing
Read MoreExample (written in C#):
int i = 13;
object myObject = i; // boxing
i = (int)myObject; // unboxing
25 :: What is deferred execution vs. immediate execution in LINQ?
In LINQ, deferred execution simply means that the query is not executed at the time it is specified. Specifically, this is accomplished by assigning the query to a variable. When this is done, the query definition is stored in the variable but the query is not executed until the query variable is iterated over. For example:
DataContext productContext = new DataContext();
var productQuery = from product in productContext.Products
where product.Type == "SOAPS"
select product; // Query is NOT executed here
foreach (var product in productQuery) // Query executes HERE
{
Console.WriteLine(product.Name);
}
You can also force immediate execution of a query. This can be useful, for example, if the database is being updated frequently, and it is important in the logic of your program to ensure that the results you’re accessing are those returned at the point in your code where the query was specified. Immediate execution is often forced using a method such as Average, Sum, Count, List, ToList, or ToArray. For example:
DataContext productContext = new DataContext();
var productCountQuery = (from product in productContext.Products
where product.Type == "SOAPS"
select product).Count(); // Query executes HERE
Read MoreDataContext productContext = new DataContext();
var productQuery = from product in productContext.Products
where product.Type == "SOAPS"
select product; // Query is NOT executed here
foreach (var product in productQuery) // Query executes HERE
{
Console.WriteLine(product.Name);
}
You can also force immediate execution of a query. This can be useful, for example, if the database is being updated frequently, and it is important in the logic of your program to ensure that the results you’re accessing are those returned at the point in your code where the query was specified. Immediate execution is often forced using a method such as Average, Sum, Count, List, ToList, or ToArray. For example:
DataContext productContext = new DataContext();
var productCountQuery = (from product in productContext.Products
where product.Type == "SOAPS"
select product).Count(); // Query executes HERE