Data Structure Arrays Interview Questions And Answers
Sharpen your Arrays interview expertise with our handpicked 31 questions. These questions are specifically selected to challenge and enhance your knowledge in Arrays. Perfect for all proficiency levels, they are key to your interview success. Get the free PDF download to access all 31 questions and excel in your Arrays interview. This comprehensive guide is essential for effective study and confidence building.
31 Arrays Questions and Answers:
Arrays Job Interview Questions Table of Contents:
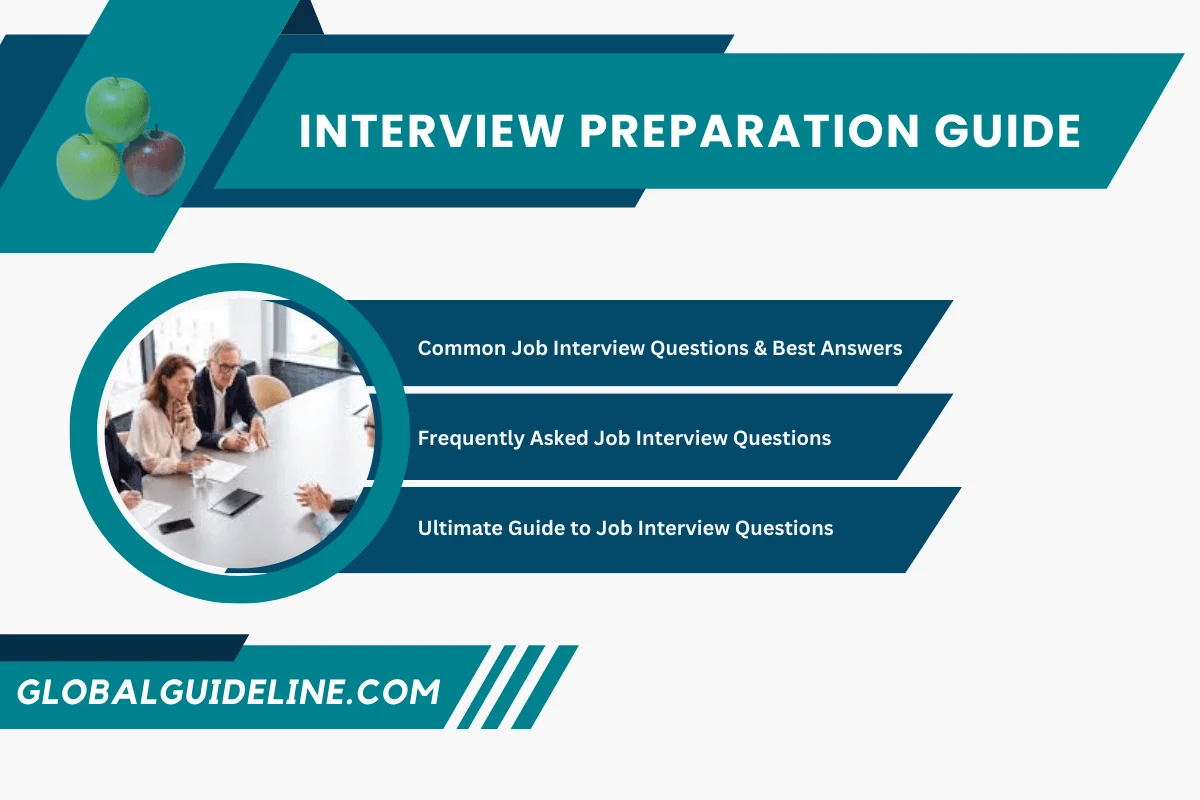
1 :: What is Array?
An array is a series of elements. These elements are of the same type. Each element can be individually accessed using an index. For e.g an array of integers. Array elements are stored one after another (contiguous) in the memory. An array can have more than one dimension. First element in an array starts with 0.
Read More2 :: What is two-dimensional array?
An array with two dimensions is called as a two-dimensional array. It is also called as a matrix. In C, a two dimensional array is initialized as int arr[nb_of_rows] [nb_of_columns]. Hence, two dimensional arrays can be considered as a grid. An element in a two dimensional can be accessed by mentioning its row and column. If the array has 20 integer values, it will occupy 80 bytes in memory (assuming 4 bytes for an integer). All of the bytes are in consecutive memory locations, the first row occupying the first 20 bytes, the second the next 20, and so on.
Read More3 :: Explain Array of pointers?
An array of pointers is an array consisting of pointers. Here, each pointer points to a row of the matrix or an element. E.g char *array [] = {“a”, “b”}. This is an array of pointers to to characters.
Read More4 :: What will be output if you will execute following c code?
#include<stdio.h>
void main(){
char arr[7]="Network";
printf("%s",arr);
Garbage value.
Read More5 :: What will be output if you will execute following c code?
#include<stdio.h>
void main(){
char arr[11]="The African Queen";
printf("%s",arr);
null.
Read More6 :: What will be output if you will execute following c code?
#include<stdio.h>
void main(){
char arr[20]="MysticRiver";
printf("%d",sizeof(arr));
20
Read More7 :: What will be output if you will execute following c code?
#include<stdio.h>
void main(){
int const SIZE=5;
int expr;
double value[SIZE]={2.0,4.0,6.0,8.0,10.0};
expr=1|2|3|4;
printf("%f",value[expr]);
Compilation error
Read More8 :: What will be output if you will execute following c code?
#include<stdio.h>
enum power{
Dalai,
Vladimir=3,
Barack,
Hillary
};
void main(){
float leader[Dalai+Hillary]={1.f,2.f,3.f,4.f,5.f};
enum power p=Barack;
printf("%0.f",leader[p>>1+1]);
2
Read More9 :: What will be output if you will execute following c code?
#include<stdio.h>
#define var 3
void main(){
char *cricket[var+~0]={"clarke","kallis"};
char *ptr=cricket[1+~0];
printf("%c",*++ptr);
l
Read More10 :: What will be output if you will execute following c code?
#include<stdio.h>
#define var 3
void main(){
char data[2][3][2]={0,1,2,3,4,5,6,7,8,9,10,11};
printf("%o",data[0][2][1]);
5
Read More11 :: What will be output if you will execute following c code?
#include<stdio.h>
#define var 3
void main(){
short num[3][2]={3,6,9,12,15,18};
printf("%d %d",*(num+1)[1],**(num+2));
15 15
Read More12 :: What will be output if you will execute following c code?
#include<stdio.h>
#define var 3
void main(){
char *ptr="cquestionbank";
printf("%d",-3[ptr]);
-101
Read More13 :: What will be output if you will execute following c code?
#include<stdio.h>
void main(){
long myarr[2][4]={0l,1l,2l,3l,4l,5l,6l,7l};
printf("%ldt",myarr[1][2]);
printf("%ld%ldt",*(myarr[1]+3),3[myarr[1]]);
printf("%ld%ld%ldt" ,*(*(myarr+1)+2),*(1[myarr]+2),3[1[myarr]]);
6 77 667
Read More14 :: What will be output if you will execute following c code?
#include<stdio.h>
void main(){
int array[2][3]={5,10,15,20,25,30};
int (*ptr)[2][3]=&array;
printf("%dt",***ptr);
printf("%dt",***(ptr+1));
printf("%dt",**(*ptr+1));
printf("%dt",*(*(*ptr+1)+2));
5 Garbage value 20 30
Read More15 :: What will be output if you will execute following c code?
#include<stdio.h>
void main(){
static int a=2,b=4,c=8;
static int *arr1[2]={&a,&b};
static int *arr2[2]={&b,&c};
int* (*arr[2])[2]={&arr1,&arr2};
printf("%d %dt",*(*arr[0])[1], *(*(**(arr+1)+1)));
4 8
Read More16 :: What will be output if you will execute following c code?
#include<stdio.h>
#include<math.h>
double myfun(double);
void main(){
double(*array[3])(double);
array[0]=exp;
array[1]=sqrt;
array[2]=myfun; printf("%.1ft",(*array)((*array[2])((**(array+1))(4))));
}
double myfun(double d){
d-=1;
return d;
}
2.7
Read More17 :: What will be output if you will execute following c code?
#include<stdio.h>
#include<math.h>
typedef struct{
char *name;
double salary;
}job;
void main(){
static job a={"TCS",15000.0};
static job b={"IBM",25000.0};
static job c={"Google",35000.0};
int x=5;
job * arr[3]={&a,&b,&c};
printf("%s %ft",(3,x>>5-4)[*arr]);
}
double myfun(double d){
d-=1;
return d;
Google 35000.000000
Read More18 :: What will be output if you will execute following c code?
#include<stdio.h>
union group{
char xarr[2][2];
char yarr[4];
};
void main(){
union group x={'A','B','C','D'};
printf("%c",x.xarr[x.yarr[2]-67][x.yarr[3]-67]);
}
B
Read More19 :: What will be output if you will execute following c code?
#include<stdio.h>
void main(){
int a=5,b=10,c=15;
int *arr[3]={&a,&b,&c};
printf("%d",*arr[*arr[1]-8]);
Compilation error
Read More20 :: What will be output if you will execute following c code?
#include<stdio.h>
void main(){
int arr[][3]={{1,2},{3,4,5},{5}};
printf("%d %d %d",sizeof(arr),arr[0][2],arr[1][2]);
}
18 0 5
Read More21 :: What will be output if you will execute following c code?
#include<stdio.h>
void main(){
int xxx[10]={5};
printf("%d %d",xxx[1],xxx[9]);
0 0
Read More22 :: What will be output if you will execute following c code?
#include<stdio.h>
#define WWW -1
enum {cat,rat};
void main(){
int Dhoni[]={2,'b',0x3,01001,'x1d','111',rat,WWW};
int i;
for(i=0;i<8;i++)
printf(" %d",Dhoni[i]);
2 98 3 513 29 73 1 -1
Read More23 :: What will be output if you will execute following c code?
#include<stdio.h>
void main(){
long double a;
signed char b;
int arr[sizeof(!a+b)];
printf("%d",sizeof(arr))
}
4
Read More24 :: Tell me do array subscripts always start with zero?
Yes. If you have an array a[MAX] (in which MAX is some value known at compile time), the first element is a[0], and the last element is a[MAX-1]. This arrangement is different from what you would find in some other languages. In some languages, such as some versions of BASIC, the elements would be a[1] through a[MAX], and in other languages, such as Pascal, you can have it either way.
Read More25 :: Explain is it valid to address one element beyond the end of an array?
It's valid to address it, but not to see what's there. (The really short answer is, "Yes, so don't worry about it.") With most compilers, if you say
int i, a[MAX], j;
then either i or j is at the part of memory just after the last element of the array. The way to see whether i or j follows the array is to compare their addresses with that of the element following the array. The way to say this in C is that either
& i == & a[ MAX ]
is true or
& a[ MAX ] == & j
is true. This isn't guaranteed; it's just the way it usually works.
Read Moreint i, a[MAX], j;
then either i or j is at the part of memory just after the last element of the array. The way to see whether i or j follows the array is to compare their addresses with that of the element following the array. The way to say this in C is that either
& i == & a[ MAX ]
is true or
& a[ MAX ] == & j
is true. This isn't guaranteed; it's just the way it usually works.