C Pointers Interview Questions & Answers Download PDF
C Pointers frequently Asked Questions by expert members with experience in C pointers. These questions and answers will help you strengthen your technical skills, prepare for the new job test and quickly revise the concepts
31 C Pointers Questions and Answers:
C Pointers Interview Questions Table of Contents:
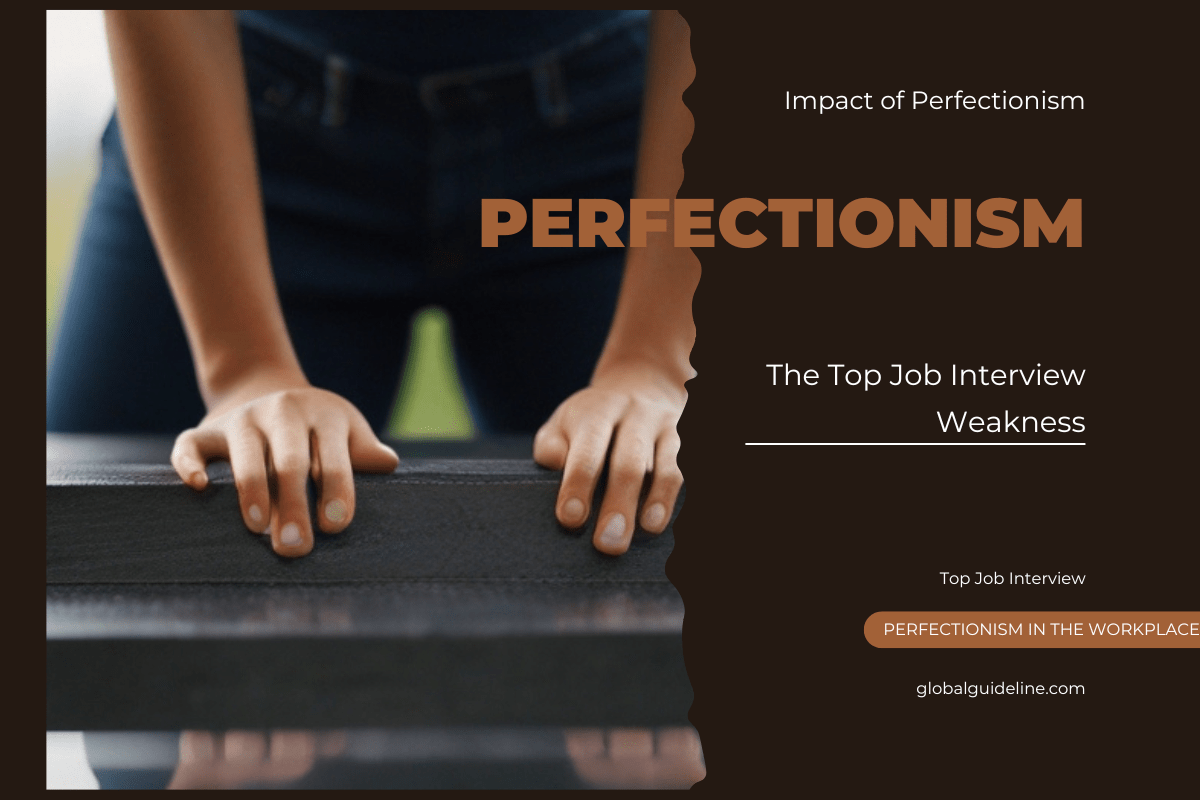
1 :: Explain null pointer?
There are times when it's necessary to have a pointer that doesn't point to anything. The macro NULL, defined in <stddef.h>, has a value that's guaranteed to be different from any valid pointer. NULL is a literal zero, possibly cast to void* or char*. Some people, notably C++ programmers, prefer to use 0 rather than NULL.<stddef.h>
2 :: Explain void pointer?
A void pointer is a C convention for "a raw address." The compiler has no idea what type of object a void pointer "really points to." If you write
int *ip;
ip points to an int. If you write
void *p;
p doesn't point to a void!
int *ip;
ip points to an int. If you write
void *p;
p doesn't point to a void!
3 :: The operator used to get value at address stored in a pointer variable is
A. *
B. &
C. &&
D. ||
Option A
(*)
(*)
4 :: A pointer is
A. A keyword used to create variables
B. A variable that stores address of an instruction
C. A variable that stores address of other variable
D. All of the above
Option C
(A variable that stores address of other variable)
(A variable that stores address of other variable)
5 :: What would be the equivalent pointer expression for referring the array element a[i][j][k][l]
A. ((((a+i)+j)+k)+l)
B. *(*(*(*(a+i)+j)+k)+l)
C. (((a+i)+j)+k+l)
D. ((a+i)+j+k+l)
Option B
*(*(*(*(a+i)+j)+k)+l)
*(*(*(*(a+i)+j)+k)+l)
7 :: How many bytes are occupied by near, far and huge pointers (DOS)?
A. near=2 far=4 huge=4
B. near=4 far=8 huge=8
C. near=2 far=4 huge=8
D. near=4 far=4 huge=8
Option A
(near=2 far=4 huge=4)
(near=2 far=4 huge=4)
8 :: In which header file is the NULL macro defined?
A. stdio.h
B. stddef.h
C. stdio.h and stddef.h
D. math.h
Option C
(stdio.h and stddef.h)
(stdio.h and stddef.h)
9 :: Can you combine the following two statements into one?
char *p;
p = (char*) malloc(100);
A. char p = *malloc(100);
B. char *p = (char) malloc(100);
C. char *p = (char*)malloc(100);
D. char *p = (char *)(malloc*)(100);
Option C
(char *p = (char*)malloc(100);)
(char *p = (char*)malloc(100);)
10 :: What is (void*)0?
A. Representation of NULL pointer
B. Representation of void pointer
C. Error
D. None of above
Option A
(Representation of NULL pointer)
(Representation of NULL pointer)
11 :: Tell me can the size of an array be declared at runtime?
No. In an array declaration, the size must be known at compile time. You can't specify a size that's known only at runtime.
12 :: Tell me when would you use a pointer to a function?
Pointers to functions are interesting when you pass them to other functions. A function that takes function pointers says, in effect, "Part of what I do can be customized. Give me a pointer to a function, and I'll call it when that part of the job needs to be done. That function can do its part for me." This is known as a "callback." It's used a lot in graphical user interface libraries, in which the style of a display is built into the library but the contents of the display are part of the application.
13 :: Can we add pointers together?
No, you can't add pointers together. If you live at 1332 Lakeview Drive, and your neighbor lives at 1364 Lakeview, what's 1332+1364? It's a number, but it doesn't mean anything. If you try to perform this type of calculation with pointers in a C program, your compiler will complain.
14 :: Tell me is NULL always defined as 0(zero)?
NULL is defined as either 0 or (void*)0. These values are almost identical; either a literal zero or a void pointer is converted automatically to any kind of pointer, as necessary, whenever a pointer is needed (although the compiler can't always tell when a pointer is needed).
15 :: Tell me when is a void pointer used?
A void pointer is used for working with raw memory or for passing a pointer to an unspecified type.
Some C code operates on raw memory. When C was first invented, character pointers (char *) were used for that. Then people started getting confused about when a character pointer was a string, when it was a character array, and when it was raw memory.
Some C code operates on raw memory. When C was first invented, character pointers (char *) were used for that. Then people started getting confused about when a character pointer was a string, when it was a character array, and when it was raw memory.
16 :: For what purpose null pointer used?
The null pointer is used in three ways:
► To stop indirection in a recursive data structure.
► As an error value.
► As a sentinel value.
► To stop indirection in a recursive data structure.
► As an error value.
► As a sentinel value.
17 :: How many levels of pointers have?
The answer depends on what you mean by "levels of pointers." If you mean "How many levels of indirection can you have in a single declaration?" the answer is "At least 12."
18 :: Explain indirection?
If you declare a variable, its name is a direct reference to its value. If you have a pointer to a variable, or any other object in memory, you have an indirect reference to its value. If p is a pointer, the value of p is the address of the object. *p means "apply the indirection operator to p"; its value is the value of the object that p points to. (Some people would read it as "Go indirect on p.")
19 :: Tell me with an example the self-referential structure?
A self referential structure is used to create data structures like linked lists, stacks, etc. Following is an example of this kind of structure:
struct struct_name
{
datatype datatypename;
struct_name * pointer_name;
};
struct struct_name
{
datatype datatypename;
struct_name * pointer_name;
};
20 :: Do you know NULL pointer?
A null pointer does not point to any object.
NULL and 0 are interchangeable in pointer contexts.Usage of NULL should be considered a gentle reminder that a pointer is involved.
It is only in pointer contexts that NULL and 0 are equivalent. NULL should not be used when another kind of 0 is required.
NULL and 0 are interchangeable in pointer contexts.Usage of NULL should be considered a gentle reminder that a pointer is involved.
It is only in pointer contexts that NULL and 0 are equivalent. NULL should not be used when another kind of 0 is required.
21 :: Can you please compare array with pointer?
A pointer is an address location of another variable. It is a value that designates the address or memory location of some other value (usually value of a variable). The value of a variable can be accessed by a pointer which points to that variable. To do so, the reference operator (&) is pre-appended to the variable that holds the value. A pointer can hold any data type, including functions.
22 :: What are the advantages of using macro in C Language?
In modular programming, using functions is advisable when a certain code is repeated several times in a program. However, everytime a function is called the control gets transferred to that function and then back to the calling function. This consumes a lot of execution time. One way to save this time is by using macros. Macros substitute a function call by the definition of that function. This saves execution time to a great extent.
23 :: What is the difference between exit() and _exit() function?
The following are the differences between exit() and _exit() functions:
- io buffers are flushed by exit() and executes some functions those are registered by atexit().
- _exit() ends the process without invoking the functions which are registered by atexit().
- io buffers are flushed by exit() and executes some functions those are registered by atexit().
- _exit() ends the process without invoking the functions which are registered by atexit().
24 :: What is the C Language function prototype?
The basic definition of a function is known as function prototype. The signature of the function is same that of a normal function, except instead of containing code, it always ends with semicolon.
When the compiler makes a single pass over each and every file that is compiled. If a function call is encountered by the compiler, which is not yet been defined, the compiler throws an error.
One of the solution for the above is to restructure the program, in which all the functions appear only before they are called in another function.
Another solution is writing the function prototypes at the beginning of the file, which ensures the C compiler to read and process the function definitions, before there is a change of calling the function. If prototypes are declared, it is convenient and comfortable for the developer to write the code of those functions which are just the needed ones.
When the compiler makes a single pass over each and every file that is compiled. If a function call is encountered by the compiler, which is not yet been defined, the compiler throws an error.
One of the solution for the above is to restructure the program, in which all the functions appear only before they are called in another function.
Another solution is writing the function prototypes at the beginning of the file, which ensures the C compiler to read and process the function definitions, before there is a change of calling the function. If prototypes are declared, it is convenient and comfortable for the developer to write the code of those functions which are just the needed ones.
25 :: Do you know the difference between malloc() and calloc() function?
The following are the differences between malloc() and calloc():
- Byte of memory is allocated by malloc(), whereas block of memory is allocated by calloc().
- malloc() takes a single argument, the size of memory, where as calloc takes two parameters, the number of variables to allocate memory and size of bytes of a single variable
- Memory initialization is not performed by malloc() , whereas memory is initialized by calloc().
- malloc(s) returns a pointer with enough storage with s bytes, where as calloc(n,s) returns a pointer with enough contiguous storage each with s bytes.
- Byte of memory is allocated by malloc(), whereas block of memory is allocated by calloc().
- malloc() takes a single argument, the size of memory, where as calloc takes two parameters, the number of variables to allocate memory and size of bytes of a single variable
- Memory initialization is not performed by malloc() , whereas memory is initialized by calloc().
- malloc(s) returns a pointer with enough storage with s bytes, where as calloc(n,s) returns a pointer with enough contiguous storage each with s bytes.
